Published February 27, 2023
How to Print PDF Files in C# Without Using Adobe
1. Introduction
Are you looking for a way to print PDF files without using Adobe Acrobat? The increased use of PDFs in the modern world means that it has become important to have a reliable and efficient solution for printing these kinds of files. Many people use Adobe Acrobat for this purpose, but it is not always available, or affordable.
In this article, we will discuss how you can print PDF documents using C# .NET applications without using Adobe Reader. For this purpose, we will use the IronPDF C# PDF library.
2. The IronPDF C# PDF Library
IronPDF is a C# PDF library that enables developers to generate, convert, and manipulate PDF documents with ease. It provides a simple API that allows developers to create and edit PDF files with just a few lines of code. With IronPDF, developers can easily add headers and footers, split and merge PDFs, and perform other common PDF operations. The library supports a wide range of file formats, including HTML, images, and Microsoft Office documents, making it easy to convert these formats to PDF. IronPDF is a powerful and flexible solution for C# developers looking to work with PDFs.
Using IronPDF, printing PDFs without a PDF reader is a piece of cake — just follow the instructions below.
3. Prerequisites
Before we get to the stage of printing documents, some requirements first need to be fulfilled.
- Create a C# .NET project in Visual Studio.
- Install IronPDF using NuGet.
3.1. Create a New Project
First, open Visual Studio.
A start-up window will appear — click on "Create a new project".
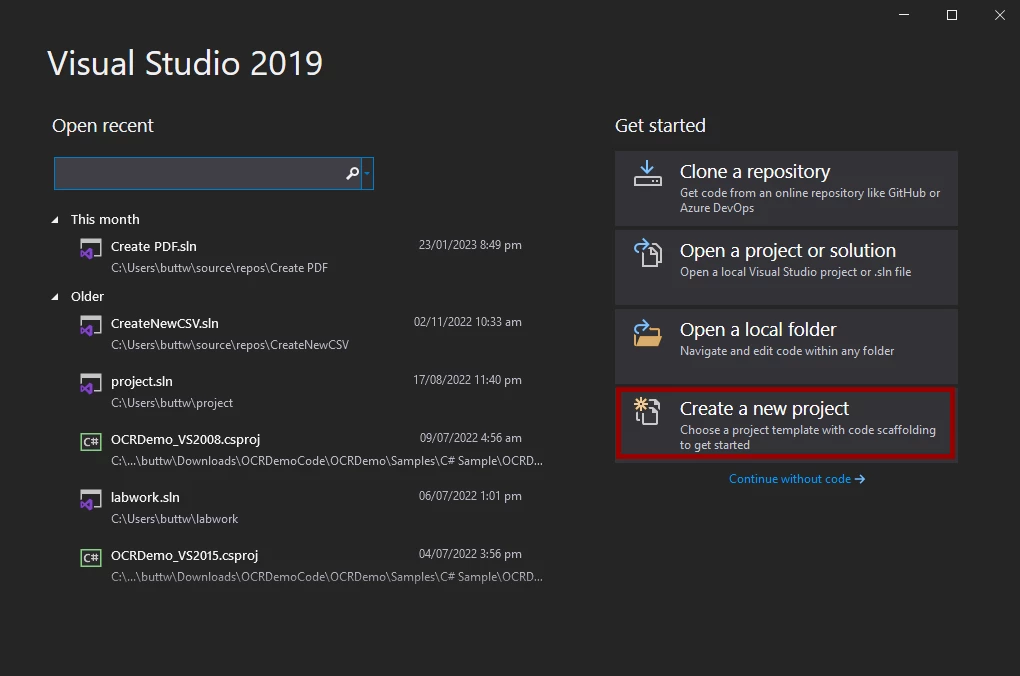
Creating a New Visual Studio Project for the Print PDF Application
In the next window, select "Console Application" and click the "Next" button in the bottom right corner.
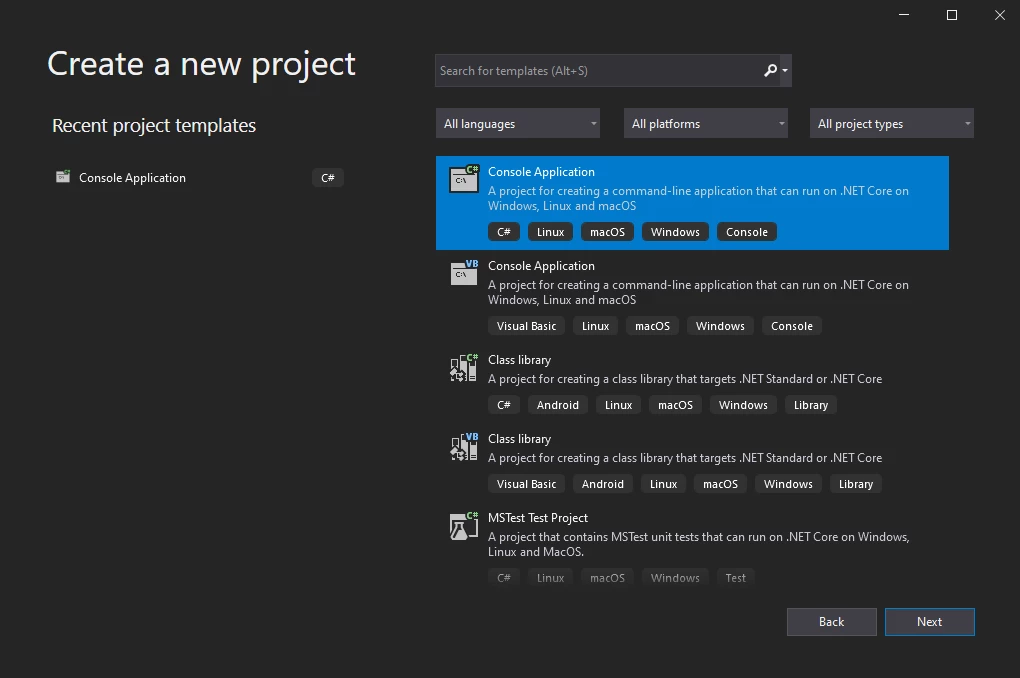
We will be creating a New Console Application in this tutorial. IronPDF can print PDF documents within any Visual Studio project template.
Another window will now appear — write the name of your project, select its location, and click the "Next" button.
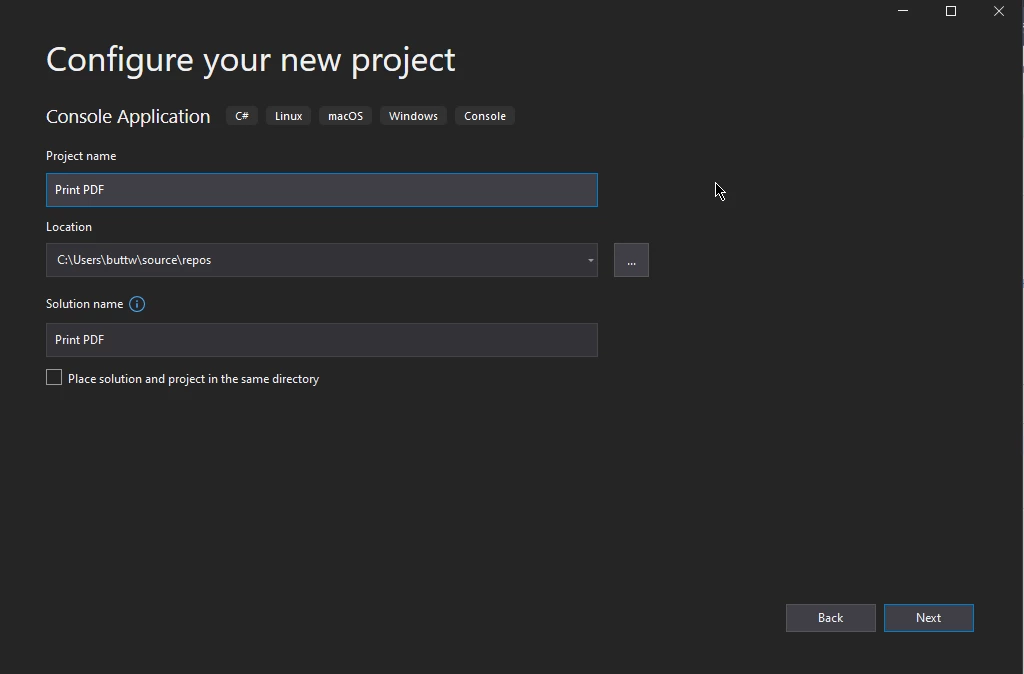
Give the new Visual Studio Project an appropriate name of your choosing.
Your new C# console application project is now created and ready to use.
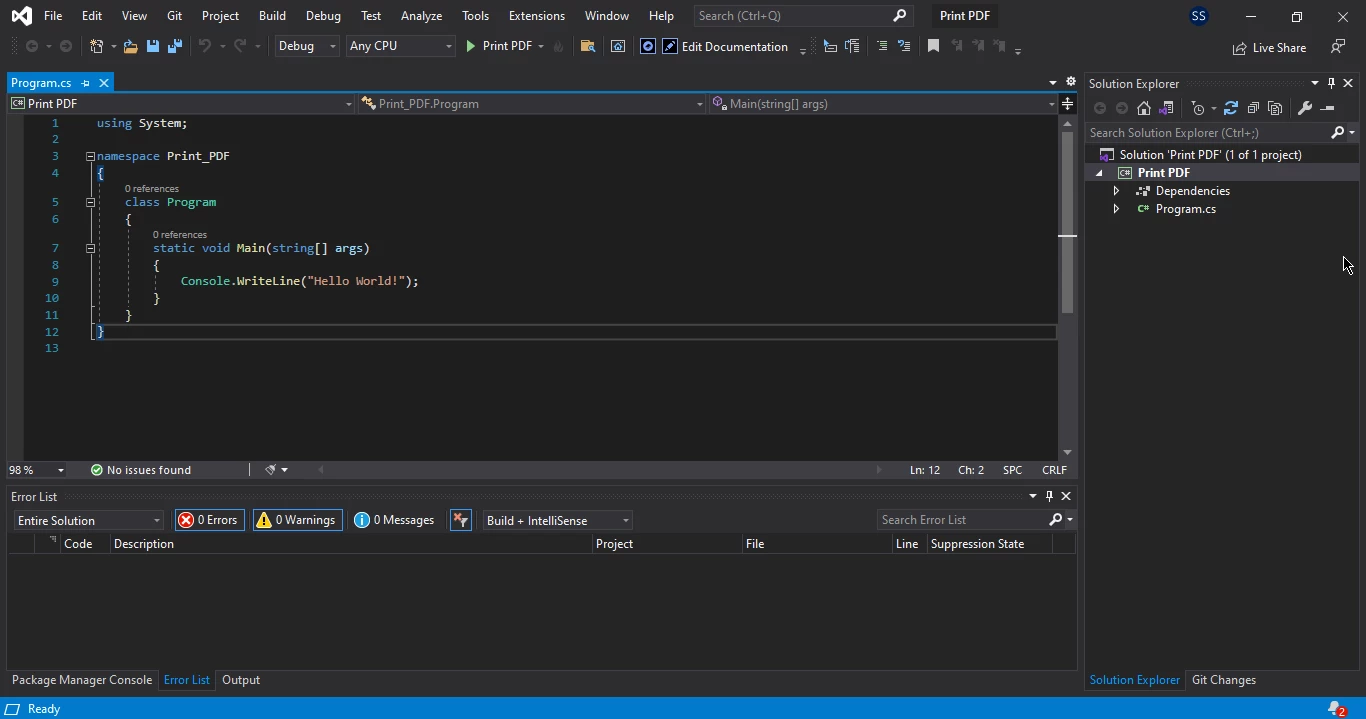
Visual Studio generates a new Project Solution based on specified options.
3.2. Install IronPDF using NuGet
Once the project is created, all that is left to do before you can print PDFs without Adobe is to install the IronPDF C# library in your project.
There are many different ways to install IronPDF, but, to keep this article short, we will only be demonstrating one of them — the NuGet Package Manager.
In your newly-created project, click on Tools in the menu bar and a dropdown menu will appear.
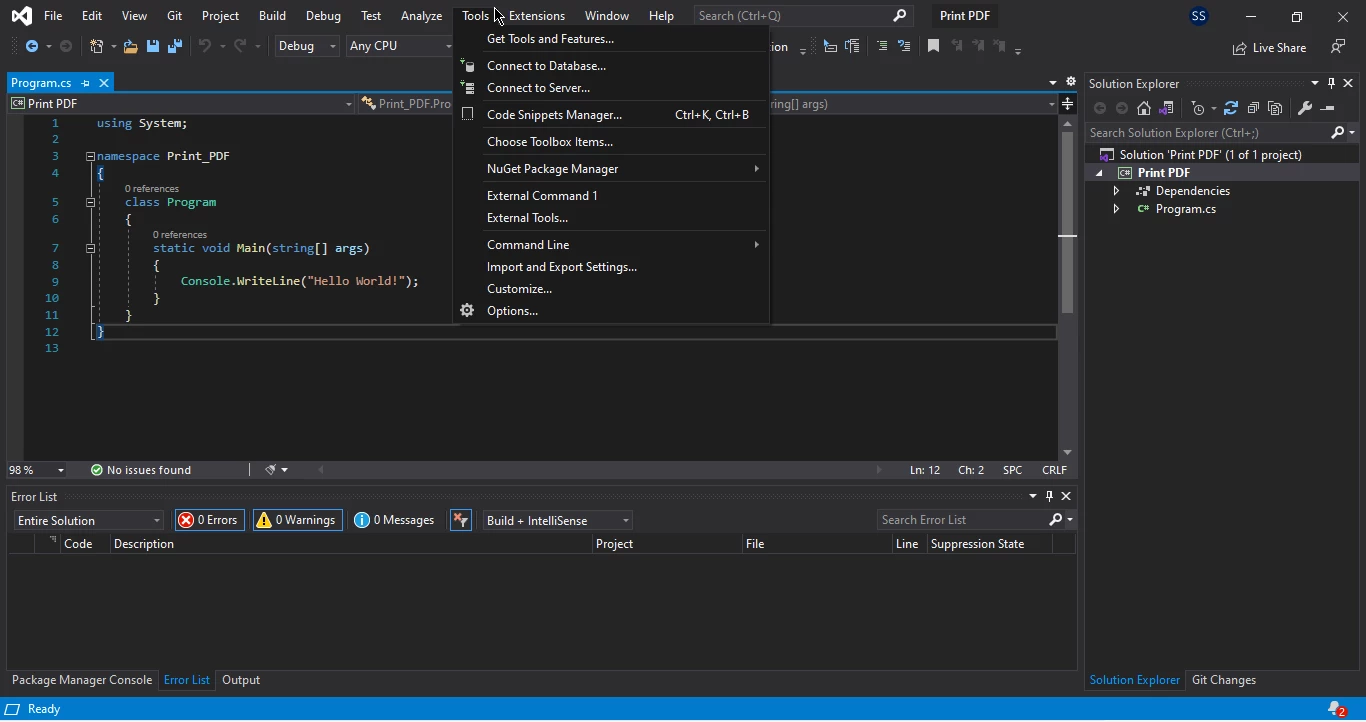
Access the NuGet Package Manager Window in Visual Studio from the Tools menu
From this dropdown menu, hover your mouse over "NuGet Package Manager", and a side menu will appear. From this menu, click on "Manage NuGet Packages for solutions...".
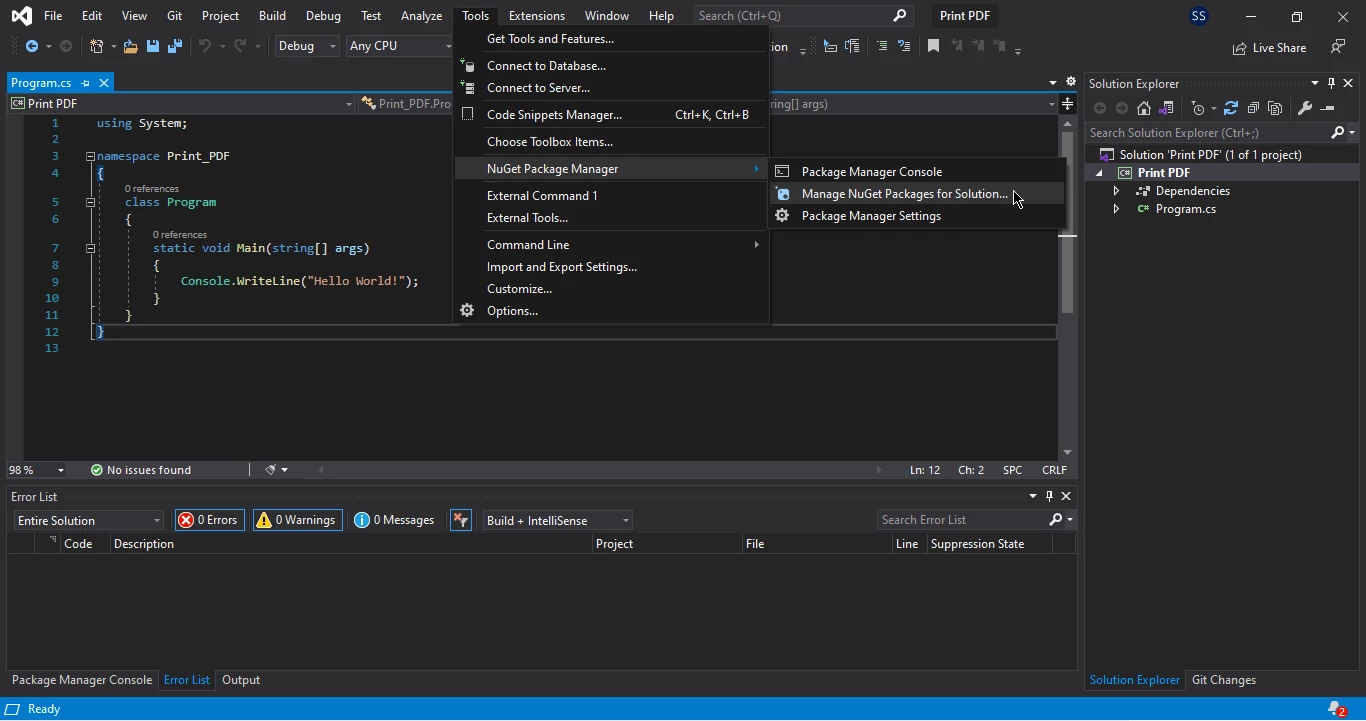
From the Tools Menu, select NuGet Package Manager > Manage Nuget Packages for solutions... to enter the NuGet Package Manager Window
A new page will open where you can navigate and browse pages.
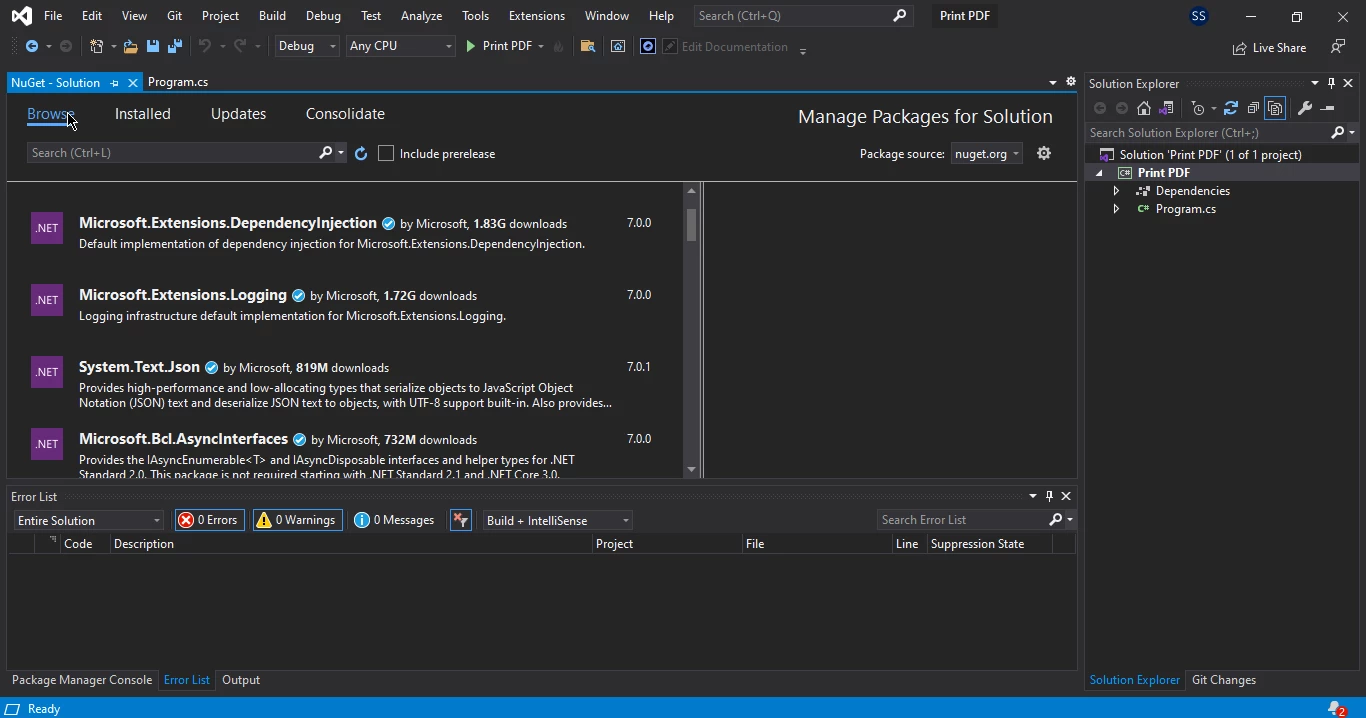
A view of the Visual Studio NuGet Package Manager Window/UI
In the search bar, write IronPDF, and you will see the list of IronPDF packages. Simply click on the most recent package and install it.
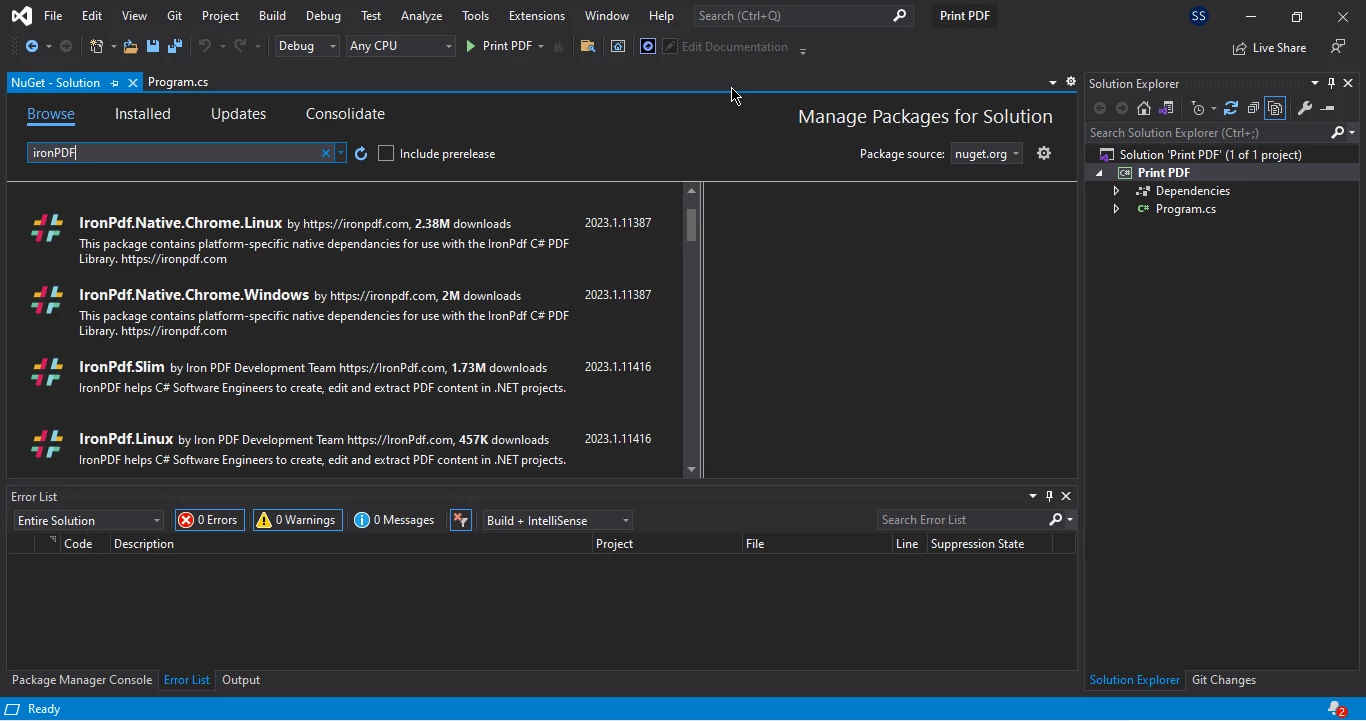
In the NuGet Package Manager window, click on the Browse button and search for "IronPdf" in the provided search field. IronPDF usually appears first in the search results.
It will only take a couple of minutes to install. and it will then be ready to use for printing PDF files.
4. Print PDF Documents using C#
To print PDF documents using C#, you can use IronPDF, a C# PDF library that supports printing. With IronPDF, you can easily print a PDF document directly from code by using the Print method. Using IronPDF, you can convert HTML files to PDF files, and print PDF documents in runtime using a single line of code. You can also print PDF documents directly from URLs.
4.1. Print PDF files from HTML files
With IronPDF, you can easily convert an HTML file to a PDF document and then print it. Here is an example of how you can print a PDF file from an HTML file in C# using IronPDF:
using IronPdf;
// Create a new PDFdocument and print it
var renderer = new IronPdf.HtmlToPdf();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Hello World</h1>");
// Send the PDF to the default printer to print
// 300 DPI, no user dialog this time ... many overloads to this method
pdf.Print(300,true);
using IronPdf;
// Create a new PDFdocument and print it
var renderer = new IronPdf.HtmlToPdf();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Hello World</h1>");
// Send the PDF to the default printer to print
// 300 DPI, no user dialog this time ... many overloads to this method
pdf.Print(300,true);
Imports IronPdf
' Create a new PDFdocument and print it
Private renderer = New IronPdf.HtmlToPdf()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Hello World</h1>")
' Send the PDF to the default printer to print
' 300 DPI, no user dialog this time ... many overloads to this method
pdf.Print(300,True)
The above sample code is for the silent printing of PDF documents. If you want to save the created PDF files and open them in a PDF viewer, you need to change PDF.Print(300, true)
to PDF.Print(300, false)
. This small change allows you to save the PDF files before printing.
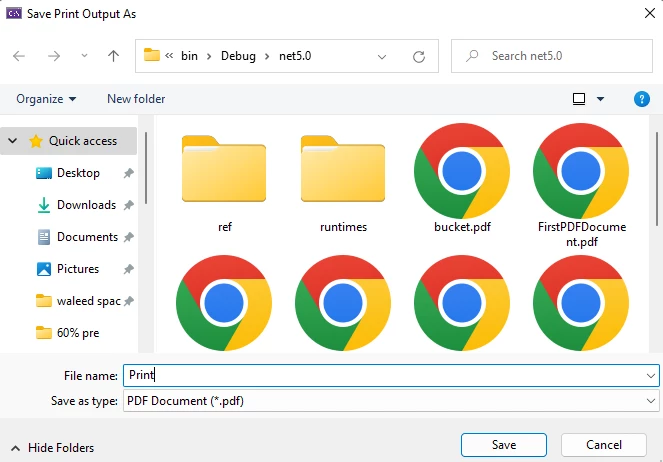
IronPDF can print documents using any installed printer, including the Microsoft Print to PDf print driver.
Below is the output from printing the PDF document.
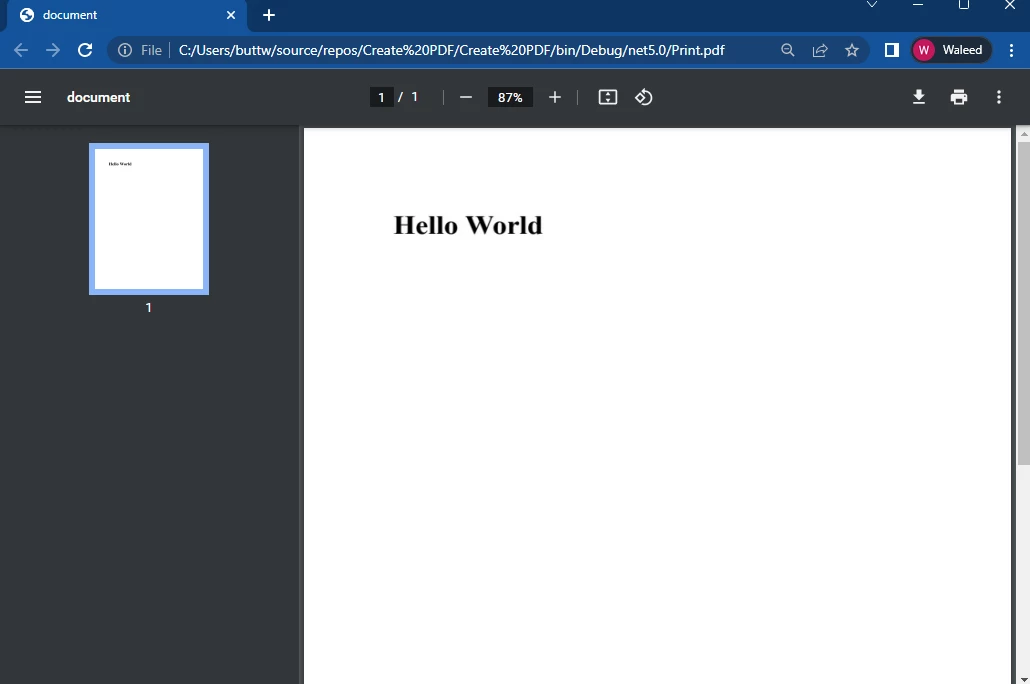
Printing a PDF document with the Microsoft Print to PDF driver using IronPDF
4.2. Print PDF Documents from URLs
Using IronPDF, you can print PDF documents directly from URLs without using Adobe Acrobat Reader, just by using a few lines of code. Below is the code for printing PDF documents directly from URLs.
using IronPdf;
// Create a new PDF and print it
var renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf");
// Send the PDF to the default printer to print
// 300 DPI, no user dialog this time ... many overloads to this method
pdf.Print(300, false);
using IronPdf;
// Create a new PDF and print it
var renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf");
// Send the PDF to the default printer to print
// 300 DPI, no user dialog this time ... many overloads to this method
pdf.Print(300, false);
Imports IronPdf
' Create a new PDF and print it
Private renderer = New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf")
' Send the PDF to the default printer to print
' 300 DPI, no user dialog this time ... many overloads to this method
pdf.Print(300, False)
Just run the above code and your URL will be converted to a PDF document and printing will begin. You can also save this file to read later.
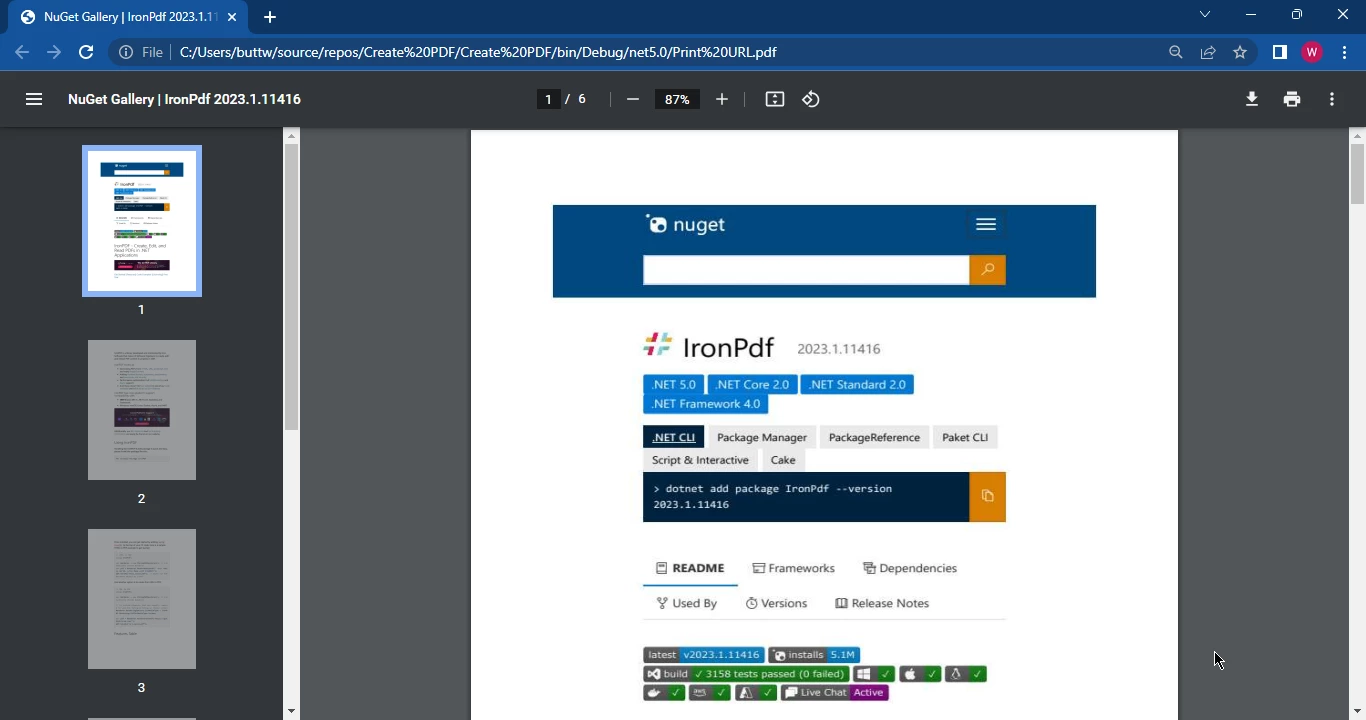
IronPDF can print HTML documents and webpages after they have been converted into PDF documents.
Below is the output of the saved file that was printed.

IronPDF Print PDF output
5. Conclusion
This article has demonstrated how you can print PDF documents without using Adobe Acrobat by using IronPDF, a C# PDF library.
IronPDF provides a straightforward API that allows developers to create and edit PDF files with just a few lines of code. The library supports a wide range of file formats, including HTML, images, and Microsoft Office documents, making it easy to convert these formats to PDF. With IronPDF, you can easily print a PDF document directly from code, convert HTML files to PDF, and print PDF documents directly from URLs. The article has demonstrated how to install IronPDF using NuGet and provided code examples of how to print PDF documents from HTML files and URLs. For more advanced and detailed C# printing tutorials, please refer to the library documentation pages.
IronPDF is free for development purposes but requires a license for commercial use. You can get additional information on licensing from our Licensing page.