Published December 7, 2022
C# Send PDF to Printer (Step-By-Step Tutorial)
PDF means "Portable Document Format". There are many scenarios where a developer needs to print PDF files programmatically in an application. In C#, this can be a very tedious task, but thanks to IronPDF, it has become very easy to do with just a few lines of code. This tool allows us to print PDF documents with default printer settings as well as with custom printing options. In this tutorial, you will learn how to print PDFs using the C# language.
Topics Covered In This Tutorial
The following topics will be covered here:
- The IronPDF Library
- Creating a C# Console Project
- Installing IronPDF
- NuGet Package Manager
- NuGet Package Manager Console
- Using DLL files
- Adding the IronPDF Namespace
- Printing PDF documents
- Create a PDF document and print PDFs
- Create a PDF document from URLs and print
- Advanced printing
- Summary
How to send PDF to Printer in C#
- Install C# library to send PDF to printer
- Print PDF with default printer setting utilizing
Print
method - Send to specific printer by setting
PrinterName
property - Set
PrinterResolution
property to customize printer's resolution - Keep trace of printed pages quantity in C#
IronPDF
IronPDF is a PDF Library for the .NET Framework that allows developers to create PDF files easily. IronPDF's rendering is "pixel-perfect" for desktop versions of Google Chrome. IronPDF easily creates PDF documents using a single line of code. It can process PDF documents without Acrobat Reader or other PDF viewers.
IronPDF can be used for creating PDF files from HTML strings, from HTML files, or from URLs. Afterward, it can send these files to a default printer for printing.
A free trial of IronPDF is available.
Some important features of the IronPDF library
- Create PDF documents from HTML 4 and 5, CSS, and JavaScript
- Generate PDF documents from URLs
- Print a PDF to a default, physical printer
- Set print job settings (for printing specific pages, etc.)
- Load URLs with custom-network login credentials, user agents, proxies, cookies, HTTP headers, and form fields or variables, thereby allowing access to web pages behind HTML login forms
- Read and fill PDF (Portable Document Format) form-field data
- Extract images and texts from PDF files
- Digitally sign PDF documents
- No third-party library required
1. Create a C# Project
This tutorial will use Visual Studio 2022, but you may also use earlier versions.
- Open Visual Studio 2022.
- Create a new C# .NET console project. Select the .NET Core console application.
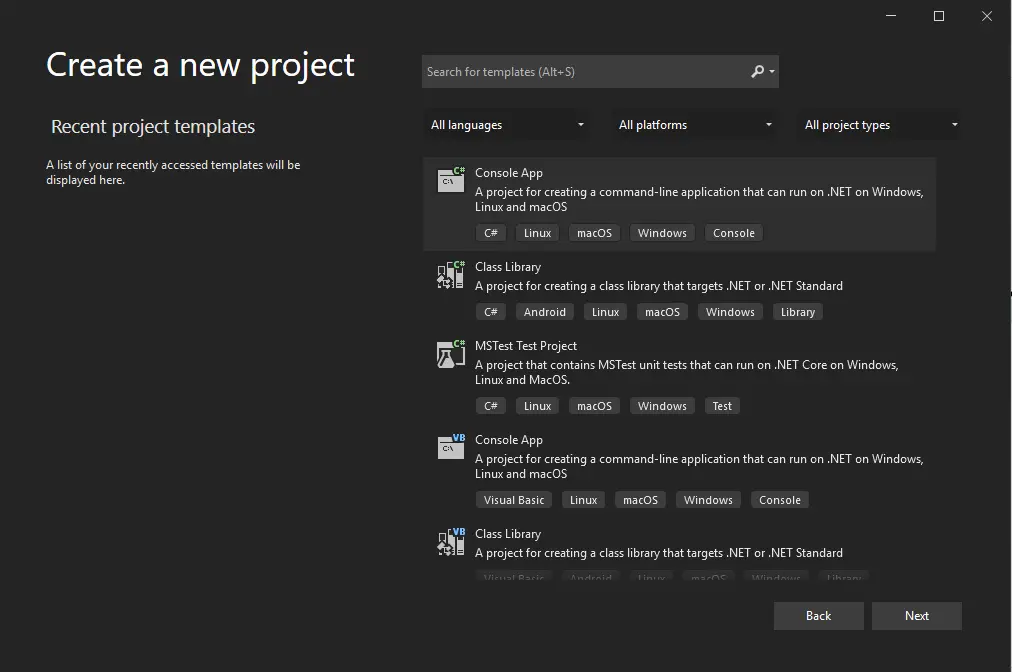
Console Application
- Give a name to the project. e.g. DemoApp.
- The .NET Framework 6.0 is the latest and most stable version that we are going to use. Click the "Create" button.
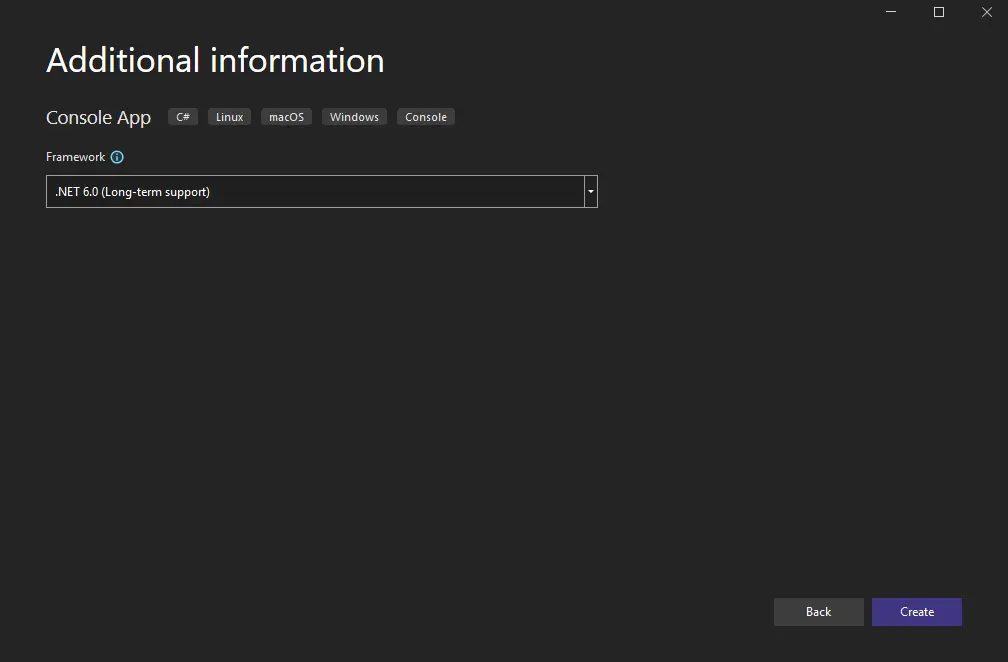
.NET Framework
2. Install the IronPDF Library
To install the IronPDF Library we can use any of the methods listed below:
2.1. NuGet Package Manager
We can install the IronPDF C# .NET Core Library from the NuGet Package Manager.
Open the Package Manager by clicking on Tools > NuGet Package Manager > Manage NuGet Packages for Solution.
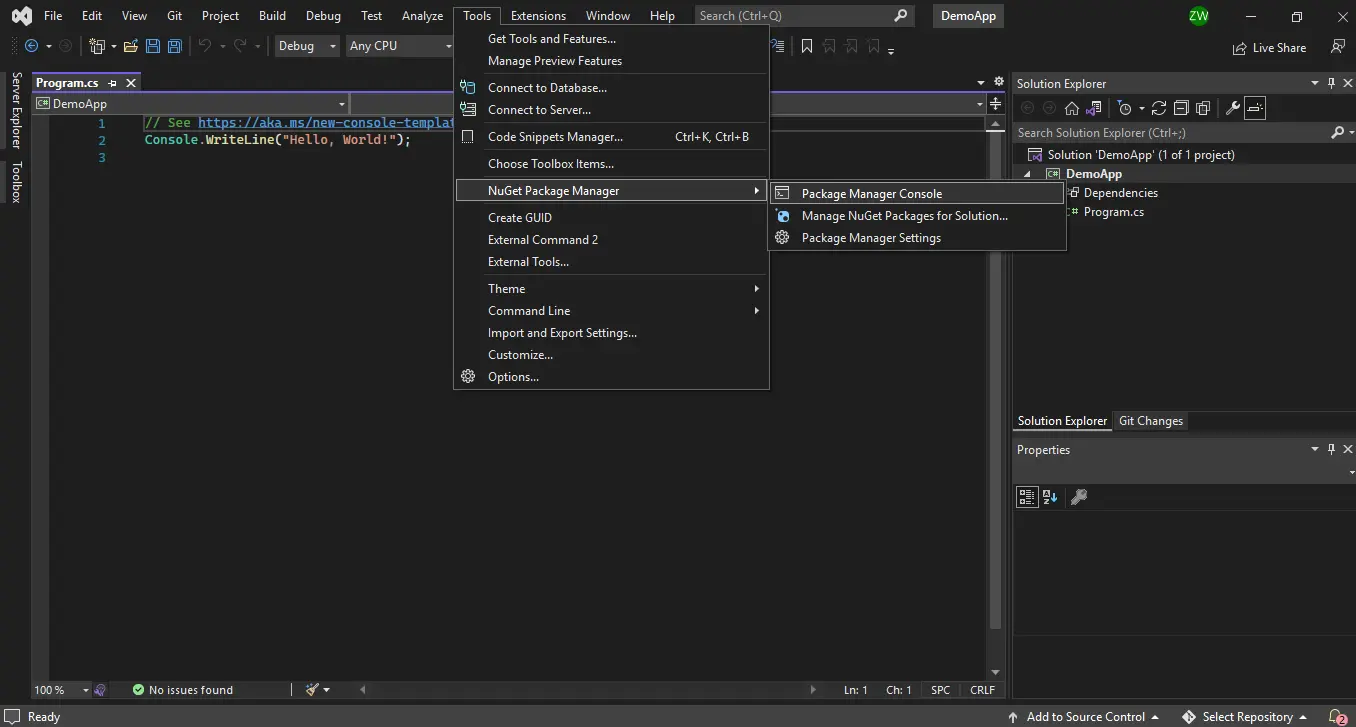
Package Manager
Or, right-click on the project in Solution Explorer and click Manage NuGet Packages.
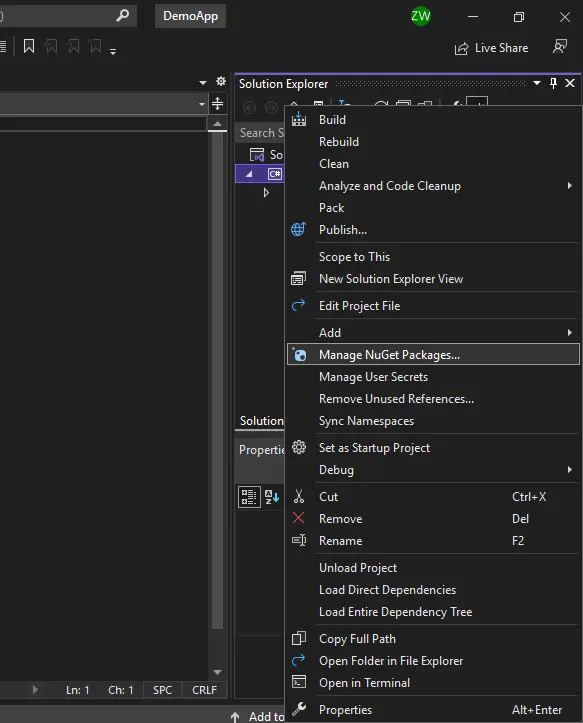
NuGet Package Manager - Solution Explorer
Search for IronPDF. Select IronPDF and click on Install. The library will begin installing.
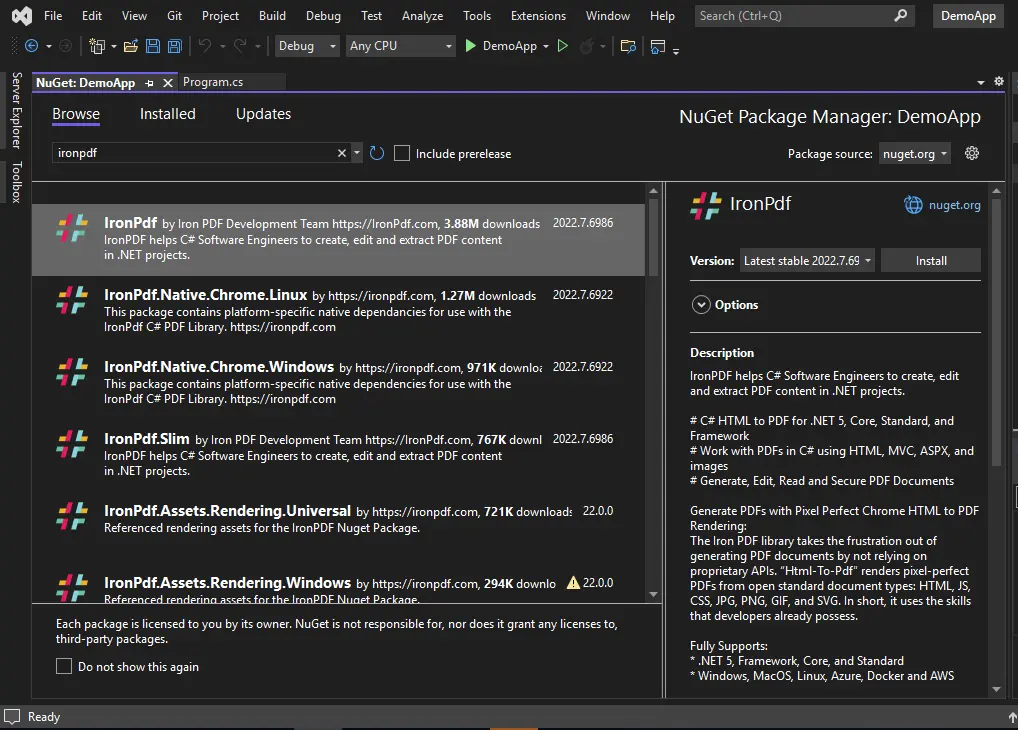
Install IronPDF
2.2. NuGet Package Manager Console
Open the NuGet Package Manager Console by clicking on Tools > NuGet Package Manager > Package Manager Console.
Type the following command in the command line:
Install-Package IronPrint

Package Manager Console
2.3. Using a DLL File
Another way to use IronPDF in your project is to add a DLL file from the IronPDF library. You can download the DLL file from this link.
- Download the DLL zip file. Extract it to a specific folder.
- Open a project in Visual Studio. In the Solution Explorer, right-click on "References" and browse for the IronPDF DLL file.
2.4. Add the IronPDF Namespace
Once the installation is done, add the IronPDF and System.Drawing.DLL
namespace to your program file.
using IronPdf;
using System.Drawing.dll;
using IronPdf;
using System.Drawing.dll;
Imports IronPdf
Imports System.Drawing.dll
Note: You must add these references to every file where you wish to use IronPDF's features.
IronPDF is installed and ready! We can now create our first PDF document for our .NET Core applications and send it to the default printer for printing. Let's have a look at some of them below using code examples.
3. Printing PDF Documents
3.1. Create and Print a PDF Document from HTML
It is very easy to process HTML strings and convert them to PDF format. This newly created file can be then printed using IronPDF. Here is the code that easily creates PDFs.
// Render any HTML fragment or document to HTML
var html= new ChromePdfRenderer();
using var PDF = html.RenderHtmlAsPdf("<h1>Hello IronPdf</h1><p>This tutorial will help to print this text to PDF file");
// Send the PDF to the default printer to print
Pdf.Print();
System.Drawing.Printing.PrintDocument PrintDocYouCanWorkWith = Pdf.GetPrintDocument();
// Render any HTML fragment or document to HTML
var html= new ChromePdfRenderer();
using var PDF = html.RenderHtmlAsPdf("<h1>Hello IronPdf</h1><p>This tutorial will help to print this text to PDF file");
// Send the PDF to the default printer to print
Pdf.Print();
System.Drawing.Printing.PrintDocument PrintDocYouCanWorkWith = Pdf.GetPrintDocument();
' Render any HTML fragment or document to HTML
Dim html= New ChromePdfRenderer()
Dim PDF = html.RenderHtmlAsPdf("<h1>Hello IronPdf</h1><p>This tutorial will help to print this text to PDF file")
' Send the PDF to the default printer to print
Pdf.Print()
Dim PrintDocYouCanWorkWith As System.Drawing.Printing.PrintDocument = Pdf.GetPrintDocument()
This code will create a PDF file with the HTML content passed in the RenderHtmlAsPdf
function. This function performs the conversion of HTML fragments to a PDF document.
You must be familiar with HTML tags to generate PDF files or PDF pages using the IronPDF library. We use the Print
function to send the output of the PDF file to the printer. The printer dialog will appear, allowing you to confirm the print job.
3.2. Create and Print a PDF Document from URL
You can also create PDF documents using a URL:
var Render = new ChromePdfRenderer();
var PDF = Render.RenderUrlAsPdf("https://ironpdf.com/");
// Send the PDF to the default printer to print
Pdf.Print();
System.Drawing.Printing.PrintDocument PrintDoc = Pdf.GetPrintDocument();
var Render = new ChromePdfRenderer();
var PDF = Render.RenderUrlAsPdf("https://ironpdf.com/");
// Send the PDF to the default printer to print
Pdf.Print();
System.Drawing.Printing.PrintDocument PrintDoc = Pdf.GetPrintDocument();
Dim Render = New ChromePdfRenderer()
Dim PDF = Render.RenderUrlAsPdf("https://ironpdf.com/")
' Send the PDF to the default printer to print
Pdf.Print()
Dim PrintDoc As System.Drawing.Printing.PrintDocument = Pdf.GetPrintDocument()
The PDF will be printed as shown below:
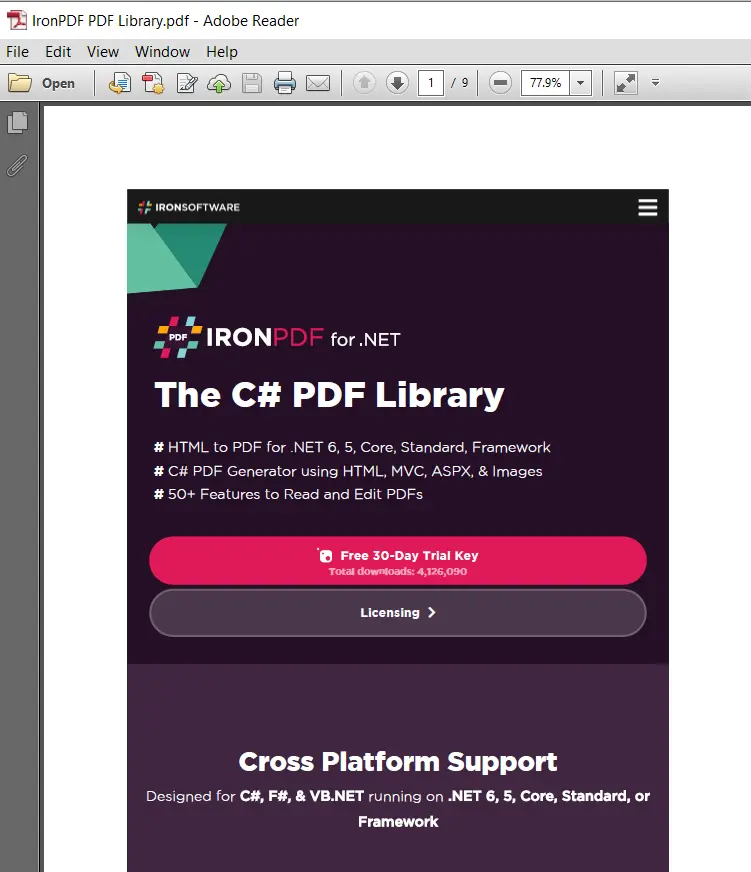
Package Manager Console
4. Advanced Printing Options
IronPDF is versatile and quite capable of handling printing features such as finding a printer and setting print resolution.
4.1 Specify the Printer
To specify the printer, all you need to do is to get the current printing document object (with the help of the GetPrintDocument
method), then use the PrinterSettings.PrinterName
property. You can choose any available printer.
using (var printDocument = PDF.GetPrintDocument())
{
printDocument.PrinterSettings.PrinterName = "Microsoft Print to PDF";
printDocument.Print();
}
using (var printDocument = PDF.GetPrintDocument())
{
printDocument.PrinterSettings.PrinterName = "Microsoft Print to PDF";
printDocument.Print();
}
Using printDocument = PDF.GetPrintDocument()
printDocument.PrinterSettings.PrinterName = "Microsoft Print to PDF"
printDocument.Print()
End Using
In the code sample above, I choose "Microsoft Print to PDF". More information about setting specific print settings can be found in the Documentation pages.
4.2 Set printer resolution
You can also set the resolution for printing a PDF. The resolution refers to the number of pixels being printed, or displayed, depending on your output. You can also set the resolution of your printing document through IronPDF with the help of the DefaultPageSettings.PrinterResolution
property of the PDF document.
printDocument.DefaultPageSettings.PrinterResolution = new PrinterResolution
{
Kind = PrinterResolutionKind.Custom,
X = 1200,
Y = 1200
};
printDocument.DefaultPageSettings.PrinterResolution = new PrinterResolution
{
Kind = PrinterResolutionKind.Custom,
X = 1200,
Y = 1200
};
printDocument.DefaultPageSettings.PrinterResolution = New PrinterResolution With {
.Kind = PrinterResolutionKind.Custom,
.X = 1200,
.Y = 1200
}
4.3 Tracing Printing Processes Using C##
In the following code example, you will see how to change the printer name, and the resolution, and how to get a count of the pages that were printed.
using (var printDocument = PDF.GetPrintDocument())
{
printDocument.PrinterSettings.PrinterName = "Microsoft Print to PDF";
printDocument.DefaultPageSettings.PrinterResolution = new PrinterResolution
{
Kind = PrinterResolutionKind.Custom,
X = 1200,
Y = 1200
};
var printedPages = 0;
printDocument.PrintPage += (sender, args) => printedPages++;
printDocument.Print();
}
using (var printDocument = PDF.GetPrintDocument())
{
printDocument.PrinterSettings.PrinterName = "Microsoft Print to PDF";
printDocument.DefaultPageSettings.PrinterResolution = new PrinterResolution
{
Kind = PrinterResolutionKind.Custom,
X = 1200,
Y = 1200
};
var printedPages = 0;
printDocument.PrintPage += (sender, args) => printedPages++;
printDocument.Print();
}
Using printDocument = PDF.GetPrintDocument()
printDocument.PrinterSettings.PrinterName = "Microsoft Print to PDF"
printDocument.DefaultPageSettings.PrinterResolution = New PrinterResolution With {
.Kind = PrinterResolutionKind.Custom,
.X = 1200,
.Y = 1200
}
Dim printedPages = 0
'INSTANT VB WARNING: An assignment within expression was extracted from the following statement:
'ORIGINAL LINE: printDocument.PrintPage += (sender, args) => printedPages++;
AddHandler printDocument.PrintPage, Sub(sender, args) printedPages
printedPages += 1
printDocument.Print()
End Using
5. Summary
IronPDF is a complete solution for working with PDF documents. It provides the ability to convert from different formats to PDF. The manipulation and formatting of PDF files become very easy with the IronPDF library function. All that is required is just a few lines of code to create and format the PDF file. It can also print PDFs programmatically. It prints a PDF by sending it to the computer's default printer. We can either display print dialog windows to the users, or we can print silently using the overloads of the Print
method.
A free trial of IronPDF is also available to test its full potential to generate and print PDF documents in your applications. More information about licensing can be found at this link.
Additionally, the current special offer allows you to obtain five Iron Software products for the price of just two.