How to Set Password and Permissions on a PDF
IronPDF supports everything you need for Password and Permissions for your existing and new PDF files. Granular meta-data and security settings can be applied, this includes the ability to limit PDF documents to be unprintable, read only, and encrypted, 128 bit encryption, decryption, and password protection are all supported.
How to Protect PDF with Password and Permissions in C#
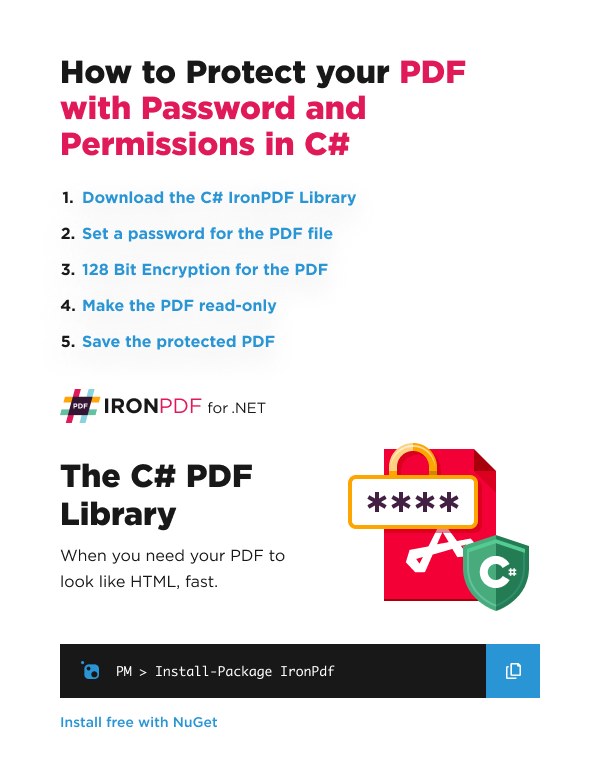
- Download C# library to protect PDF with password
- Set OwnerPassword property to prevent PDF file from being edited
- Set UserPassword property to prevent PDF file from being opened
- Encrypt PDF file with 128 Bit Encryption
- Supply the password to
FromFile
method to open PDF document
Install with NuGet
Install-Package IronPdf
Set a Password for a PDF
Here we have an example of a PDF which we want to protect with IronPDF:
Let's run the following code to add a password to the PDF. We will use the password password123
as an example:
:path=/static-assets/pdf/content-code-examples/how-to/pdf-permissions-passwords-add-password.cs
using IronPdf;
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderHtmlAsPdf("<h1>Secret Information:</h1> Hello World");
pdf.SecuritySettings.OwnerPassword = "123password"; // password to edit the pdf
pdf.SecuritySettings.UserPassword = "password123"; // password to open the pdf
pdf.SaveAs("protected.pdf");
Imports IronPdf
Private renderer = New ChromePdfRenderer()
Private pdf = renderer.RenderHtmlAsPdf("<h1>Secret Information:</h1> Hello World")
pdf.SecuritySettings.OwnerPassword = "123password" ' password to edit the pdf
pdf.SecuritySettings.UserPassword = "password123" ' password to open the pdf
pdf.SaveAs("protected.pdf")
The result is the following PDF which you can view by typing the password password123
.
Open a PDF that has a Password
This is how we can open a PDF that has a password. The PdfDocument.FromFile
method has a second optional parameter which is the password:
:path=/static-assets/pdf/content-code-examples/how-to/pdf-permissions-passwords-open-password.cs
using IronPdf;
var pdf = PdfDocument.FromFile("protected.pdf", "password123");
//... perform PDF-tasks
pdf.SaveAs("protected_2.pdf"); // Saved as another file
Imports IronPdf
Private pdf = PdfDocument.FromFile("protected.pdf", "password123")
'... perform PDF-tasks
pdf.SaveAs("protected_2.pdf") ' Saved as another file
Advanced Security and Permissions Settings
The PdfDocument
object also has MetaData fields you may set such as Author
and ModifiedDate
. You can also disable User Annotations, User Printing, and many more as shown below:
:path=/static-assets/pdf/content-code-examples/how-to/pdf-permissions-passwords-advanced.cs
using IronPdf;
// Open an Encrypted File, alternatively create a new PDF from HTML
var pdf = PdfDocument.FromFile("protected.pdf", "password");
// Edit file metadata
pdf.MetaData.Author = "Satoshi Nakamoto";
pdf.MetaData.Keywords = "SEO, Friendly";
pdf.MetaData.ModifiedDate = System.DateTime.Now;
// Edit file security settings
// The following code makes a PDF read only and will disallow copy & paste and printing
pdf.SecuritySettings.RemovePasswordsAndEncryption();
pdf.SecuritySettings.MakePdfDocumentReadOnly("secret-key");
pdf.SecuritySettings.AllowUserAnnotations = false;
pdf.SecuritySettings.AllowUserCopyPasteContent = false;
pdf.SecuritySettings.AllowUserFormData = false;
pdf.SecuritySettings.AllowUserPrinting = IronPdf.Security.PdfPrintSecurity.FullPrintRights;
// Change or set the document encryption password
pdf.SecuritySettings.OwnerPassword = "top-secret"; // password to edit the pdf
pdf.SecuritySettings.UserPassword = "sharable"; // password to open the pdf
// Save the secure PDF
pdf.SaveAs("secured.pdf");
Imports System
Imports IronPdf
' Open an Encrypted File, alternatively create a new PDF from HTML
Private pdf = PdfDocument.FromFile("protected.pdf", "password")
' Edit file metadata
pdf.MetaData.Author = "Satoshi Nakamoto"
pdf.MetaData.Keywords = "SEO, Friendly"
pdf.MetaData.ModifiedDate = DateTime.Now
' Edit file security settings
' The following code makes a PDF read only and will disallow copy & paste and printing
pdf.SecuritySettings.RemovePasswordsAndEncryption()
pdf.SecuritySettings.MakePdfDocumentReadOnly("secret-key")
pdf.SecuritySettings.AllowUserAnnotations = False
pdf.SecuritySettings.AllowUserCopyPasteContent = False
pdf.SecuritySettings.AllowUserFormData = False
pdf.SecuritySettings.AllowUserPrinting = IronPdf.Security.PdfPrintSecurity.FullPrintRights
' Change or set the document encryption password
pdf.SecuritySettings.OwnerPassword = "top-secret" ' password to edit the pdf
pdf.SecuritySettings.UserPassword = "sharable" ' password to open the pdf
' Save the secure PDF
pdf.SaveAs("secured.pdf")