How to Edit a PDF in C#
Introduction
Iron Software has simplified many different PDF edit functions into easy to read and understand methods in the IronPDF library. Be it adding signatures, adding HTML footers, stamping watermarks, or adding annotations. IronPDF is the tool for you which allows you to have readable code, programmatic PDF generation, easy debugging, and easy deployment to any supported environment or platform.
IronPDF has countless features when it comes to editing PDFs. In this tutorial article, we will walk through some of the major ones with code examples and some explanations.
With this article you will have a good understanding of how to use IronPDF to edit your PDFs in C#.
Table of Contents
- Edit Document Structure
- Edit Document Properties
- Edit PDF Content
- Using Forms in PDFs
Install with NuGet
Install-Package IronPdf
Edit Document Structure
Manipulate Pages
Adding PDFs at specific indexes, copying pages out as a range or one by one, and deleting pages from any PDF is a walk in the park with IronPDF which takes care of everything behind the scenes.
Add Pages
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-1.cs
var pdf = new PdfDocument("report.pdf");
var renderer = new ChromePdfRenderer();
var coverPagePdf = renderer.RenderHtmlAsPdf("<h1>Cover Page</h1><hr>");
pdf.PrependPdf(coverPagePdf);
pdf.SaveAs("report_with_cover.pdf");
Dim pdf = New PdfDocument("report.pdf")
Dim renderer = New ChromePdfRenderer()
Dim coverPagePdf = renderer.RenderHtmlAsPdf("<h1>Cover Page</h1><hr>")
pdf.PrependPdf(coverPagePdf)
pdf.SaveAs("report_with_cover.pdf")
Copy Pages
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-2.cs
var pdf = new PdfDocument("report.pdf");
// Copy pages 5 to 7 and save them as a new document.
pdf.CopyPages(4, 6).SaveAs("report_highlight.pdf");
Dim pdf = New PdfDocument("report.pdf")
' Copy pages 5 to 7 and save them as a new document.
pdf.CopyPages(4, 6).SaveAs("report_highlight.pdf")
Delete Pages
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-3.cs
var pdf = new PdfDocument("report.pdf");
// Remove the last page from the PDF and save again
pdf.RemovePage(pdf.PageCount - 1);
pdf.SaveAs("report_minus_one_page.pdf");
Dim pdf = New PdfDocument("report.pdf")
' Remove the last page from the PDF and save again
pdf.RemovePage(pdf.PageCount - 1)
pdf.SaveAs("report_minus_one_page.pdf")
Merge and Split PDFs
Merging PDFs into one PDF or Splitting up an existing PDF is easily achieved with IronPDF's intuitive API.
Join Multiple Existing PDFs into a Single PDF Document
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-4.cs
var pdfs = new List<PdfDocument>
{
PdfDocument.FromFile("A.pdf"),
PdfDocument.FromFile("B.pdf"),
PdfDocument.FromFile("C.pdf")
};
PdfDocument mergedPdf = PdfDocument.Merge(pdfs);
mergedPdf.SaveAs("merged.pdf");
foreach (var pdf in pdfs)
{
pdf.Dispose();
}
Dim pdfs = New List(Of PdfDocument) From {PdfDocument.FromFile("A.pdf"), PdfDocument.FromFile("B.pdf"), PdfDocument.FromFile("C.pdf")}
Dim mergedPdf As PdfDocument = PdfDocument.Merge(pdfs)
mergedPdf.SaveAs("merged.pdf")
For Each pdf In pdfs
pdf.Dispose()
Next pdf
To see joining two or more PDFs on our Code Examples page, please visit here.
Splitting a PDF and Extracting Pages
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-5.cs
var pdf = new PdfDocument("sample.pdf");
// Take the first page
var pdf_page1 = pdf.CopyPage(0);
pdf_page1.SaveAs("Split1.pdf");
// Take the pages 2 & 3
var pdf_page2_3 = pdf.CopyPages(1, 2);
pdf_page2_3.SaveAs("Spli2t.pdf");
Dim pdf = New PdfDocument("sample.pdf")
' Take the first page
Dim pdf_page1 = pdf.CopyPage(0)
pdf_page1.SaveAs("Split1.pdf")
' Take the pages 2 & 3
Dim pdf_page2_3 = pdf.CopyPages(1, 2)
pdf_page2_3.SaveAs("Spli2t.pdf")
To see Splitting and Extracting Pages on our Code Examples page, please visit here.
Edit Document Properties
Add and Use PDF Metadata
You can easily use IronPDF to browse and edit PDF metadata:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-6.cs
// Open an Encrypted File, alternatively create a new PDF from Html
var pdf = PdfDocument.FromFile("encrypted.pdf", "password");
// Edit file metadata
pdf.MetaData.Author = "Satoshi Nakamoto";
pdf.MetaData.Keywords = "SEO, Friendly";
pdf.MetaData.ModifiedDate = System.DateTime.Now;
// Edit file security settings
// The following code makes a PDF read only and will disallow copy & paste and printing
pdf.SecuritySettings.RemovePasswordsAndEncryption();
pdf.SecuritySettings.MakePdfDocumentReadOnly("secret-key");
pdf.SecuritySettings.AllowUserAnnotations = false;
pdf.SecuritySettings.AllowUserCopyPasteContent = false;
pdf.SecuritySettings.AllowUserFormData = false;
pdf.SecuritySettings.AllowUserPrinting = IronPdf.Security.PdfPrintSecurity.FullPrintRights;
// Change or set the document encryption password
pdf.SecuritySettings.OwnerPassword = "top-secret"; // password to edit the pdf
pdf.SecuritySettings.UserPassword = "shareable"; // password to open the pdf
pdf.SaveAs("secured.pdf");
Imports System
' Open an Encrypted File, alternatively create a new PDF from Html
Dim pdf = PdfDocument.FromFile("encrypted.pdf", "password")
' Edit file metadata
pdf.MetaData.Author = "Satoshi Nakamoto"
pdf.MetaData.Keywords = "SEO, Friendly"
pdf.MetaData.ModifiedDate = DateTime.Now
' Edit file security settings
' The following code makes a PDF read only and will disallow copy & paste and printing
pdf.SecuritySettings.RemovePasswordsAndEncryption()
pdf.SecuritySettings.MakePdfDocumentReadOnly("secret-key")
pdf.SecuritySettings.AllowUserAnnotations = False
pdf.SecuritySettings.AllowUserCopyPasteContent = False
pdf.SecuritySettings.AllowUserFormData = False
pdf.SecuritySettings.AllowUserPrinting = IronPdf.Security.PdfPrintSecurity.FullPrintRights
' Change or set the document encryption password
pdf.SecuritySettings.OwnerPassword = "top-secret" ' password to edit the pdf
pdf.SecuritySettings.UserPassword = "shareable" ' password to open the pdf
pdf.SaveAs("secured.pdf")
Digital Signatures
IronPDF has options to digitally sign new or existing PDF files using .pfx and .p12 X509Certificate2 digital certificates.
Once a PDF file is signed, it can not be modified without the certificate being validated. This ensures fidelity.
To generate a signing certificate for free using Adobe Reader, please read https://helpx.adobe.com/acrobat/using/digital-ids.html
In addition to cryptographic signing, a hand written signature image or company stamp image may also be used to sign using IronPDF.
Cryptographically sign an existing PDF in 1 line of code!
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-7.cs
using IronPdf;
using IronPdf.Signing;
new IronPdf.Signing.PdfSignature("Iron.p12", "123456").SignPdfFile("any.pdf");
Imports IronPdf
Imports IronPdf.Signing
Call (New IronPdf.Signing.PdfSignature("Iron.p12", "123456")).SignPdfFile("any.pdf")
More advanced example which has more control:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-8.cs
using IronPdf;
// Step 1. Create a PDF
var renderer = new ChromePdfRenderer();
var doc = renderer.RenderHtmlAsPdf("<h1>Testing 2048 bit digital security</h1>");
// Step 2. Create a Signature.
// You may create a .pfx or .p12 PDF signing certificate using Adobe Acrobat Reader.
// Read: https://helpx.adobe.com/acrobat/using/digital-ids.html
var signature = new IronPdf.Signing.PdfSignature("Iron.pfx", "123456")
{
// Step 3. Optional signing options and a handwritten signature graphic
SigningContact = "support@ironsoftware.com",
SigningLocation = "Chicago, USA",
SigningReason = "To show how to sign a PDF"
};
//Step 4. Sign the PDF with the PdfSignature. Multiple signing certificates may be used
doc.Sign(signature);
//Step 5. The PDF is not signed until saved to file, steam or byte array.
doc.SaveAs("signed.pdf");
Imports IronPdf
' Step 1. Create a PDF
Private renderer = New ChromePdfRenderer()
Private doc = renderer.RenderHtmlAsPdf("<h1>Testing 2048 bit digital security</h1>")
' Step 2. Create a Signature.
' You may create a .pfx or .p12 PDF signing certificate using Adobe Acrobat Reader.
' Read: https://helpx.adobe.com/acrobat/using/digital-ids.html
Private signature = New IronPdf.Signing.PdfSignature("Iron.pfx", "123456") With {
.SigningContact = "support@ironsoftware.com",
.SigningLocation = "Chicago, USA",
.SigningReason = "To show how to sign a PDF"
}
'Step 4. Sign the PDF with the PdfSignature. Multiple signing certificates may be used
doc.Sign(signature)
'Step 5. The PDF is not signed until saved to file, steam or byte array.
doc.SaveAs("signed.pdf")
PDF Attachments
IronPDF fully supports adding and removing attachments to and from your PDF documents.
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-9.cs
var Renderer = new ChromePdfRenderer();
var myPdf = Renderer.RenderHtmlFileAsPdf("my-content.html");
// Here we can add an attachment with a name and byte[]
var attachment1 = myPdf.Attachments.AddAttachment("attachment_1", example_attachment);
// And here is an example of removing an attachment
myPdf.Attachments.RemoveAttachment(attachment1);
myPdf.SaveAs("my-content.pdf");
Dim Renderer = New ChromePdfRenderer()
Dim myPdf = Renderer.RenderHtmlFileAsPdf("my-content.html")
' Here we can add an attachment with a name and byte[]
Dim attachment1 = myPdf.Attachments.AddAttachment("attachment_1", example_attachment)
' And here is an example of removing an attachment
myPdf.Attachments.RemoveAttachment(attachment1)
myPdf.SaveAs("my-content.pdf")
Compress PDFs
IronPDF supports compression of PDFs. One way a PDF file size can be reduced is by reducing the size of embedded images within the PdfDocument. In IronPDF, we can call the CompressImages
method.
The way resizing JPEGs works, 100% quality has almost no loss, and 1% is a very low quality output image. Generally speaking, 90% and above are considered high quality, 80%-90% is considered medium quality, and 70%-80% is considered low quality. Reducing below 70% will produce low quality images, but doing this may reduce the total file size of the PdfDocument dramatically.
Please experiment with different values to get a feel for the range of quality versus the file size to get the optimal balance for your requirements. Obviousness of quality reduction ultimately depends on the type of image that was input, and some images may reduce in clarity more significantly than others.
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-10.cs
var pdf = new PdfDocument("document.pdf");
// Quality parameter can be 1-100, where 100 is 100% of original quality
pdf.CompressImages(60);
pdf.SaveAs("document_compressed.pdf");
Dim pdf = New PdfDocument("document.pdf")
' Quality parameter can be 1-100, where 100 is 100% of original quality
pdf.CompressImages(60)
pdf.SaveAs("document_compressed.pdf")
There is a second optional parameter that can scale down the image resolution according to its visible size in the PDF document. Note that this may cause distortion with some image configurations:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-11.cs
var pdf = new PdfDocument("document.pdf");
pdf.CompressImages(90, true);
pdf.SaveAs("document_scaled_compressed.pdf");
Dim pdf = New PdfDocument("document.pdf")
pdf.CompressImages(90, True)
pdf.SaveAs("document_scaled_compressed.pdf")
Editing PDF Content
Add Headers and Footers
You can easily add headers and footers to your PDF. IronPDF has two different kinds of "HeaderFooters" which are TextHtmlFooter
and HtmlHeaderFooter
. TextHeaderFooter is better for header and footers that only have text in them and may want to use merge fields such as "{page} of {total-pages}"
. HtmlHeaderFooter is an advanced header and footer which works with any HTML in it and formats neatly.
HTML Header and Footer
HTML Header Footer will use the rendered version of your HTML as a Header or Footer on your PDF document for pixel perfect layout.
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-12.cs
var renderer = new ChromePdfRenderer();
// Build a footer using html to style the text
// mergeable fields are:
// {page} {total-pages} {url} {date} {time} {html-title} & {pdf-title}
renderer.RenderingOptions.HtmlFooter = new HtmlHeaderFooter()
{
MaxHeight = 15, //millimeters
HtmlFragment = "<center><i>{page} of {total-pages}<i></center>",
DrawDividerLine = true
};
// Build a header using an image asset
// Note the use of BaseUrl to set a relative path to the assets
renderer.RenderingOptions.HtmlHeader = new HtmlHeaderFooter()
{
MaxHeight = 20, //millimeters
HtmlFragment = "<img src='logo.png'>",
BaseUrl = new System.Uri(@"C:\assets\images").AbsoluteUri
};
Dim renderer = New ChromePdfRenderer()
' Build a footer using html to style the text
' mergeable fields are:
' {page} {total-pages} {url} {date} {time} {html-title} & {pdf-title}
renderer.RenderingOptions.HtmlFooter = New HtmlHeaderFooter() With {
.MaxHeight = 15,
.HtmlFragment = "<center><i>{page} of {total-pages}<i></center>",
.DrawDividerLine = True
}
' Build a header using an image asset
' Note the use of BaseUrl to set a relative path to the assets
renderer.RenderingOptions.HtmlHeader = New HtmlHeaderFooter() With {
.MaxHeight = 20,
.HtmlFragment = "<img src='logo.png'>",
.BaseUrl = (New System.Uri("C:\assets\images")).AbsoluteUri
}
For a full in-depth example with multiple use-cases see this code tutorial: here.
Text Header and Footer
The basic header and footer is the Text HeaderFooter, please see the example below.
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-13.cs
var renderer = new ChromePdfRenderer
{
RenderingOptions =
{
FirstPageNumber = 1, // use 2 if a cover-page will be appended
// Add a header to every page easily:
TextHeader =
{
DrawDividerLine = true,
CenterText = "{url}",
Font = IronSoftware.Drawing.FontTypes.Helvetica,
FontSize = 12
},
// Add a footer too:
TextFooter =
{
DrawDividerLine = true,
Font = IronSoftware.Drawing.FontTypes.Arial,
FontSize = 10,
LeftText = "{date} {time}",
RightText = "{page} of {total-pages}"
}
}
};
Dim renderer = New ChromePdfRenderer With {
.RenderingOptions = {
FirstPageNumber = 1, TextHeader = {
DrawDividerLine = True,
CenterText = "{url}",
Font = IronSoftware.Drawing.FontTypes.Helvetica,
FontSize = 12
},
TextFooter = {
DrawDividerLine = True,
Font = IronSoftware.Drawing.FontTypes.Arial,
FontSize = 10,
LeftText = "{date} {time}",
RightText = "{page} of {total-pages}"
}
}
}
We also have the following merge fields that will be replaced with values when rendered: {page}
, {total-pages}
, {url}
, {date}
, {time}
, {html-title}
, {pdf-title}
Find and Replace Text in a PDF
Make placeholders in your PDFs and replace them programmatically, or simply replace all instances of a text phrase using our ReplaceTextOnPage
method.
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-14.cs
// Using an existing PDF
var pdf = PdfDocument.FromFile("sample.pdf");
// Parameters
int pageIndex = 1;
string oldText = ".NET 6"; // Old text to remove
string newText = ".NET 7"; // New text to add
// Replace Text on Page
pdf.ReplaceTextOnPage(pageIndex, oldText, newText);
// Placeholder Template Example
pdf.ReplaceTextOnPage(pageIndex, "[DATE]", "01/01/2000");
// Save your new PDF
pdf.SaveAs("new_sample.pdf");
' Using an existing PDF
Dim pdf = PdfDocument.FromFile("sample.pdf")
' Parameters
Dim pageIndex As Integer = 1
Dim oldText As String = ".NET 6" ' Old text to remove
Dim newText As String = ".NET 7" ' New text to add
' Replace Text on Page
pdf.ReplaceTextOnPage(pageIndex, oldText, newText)
' Placeholder Template Example
pdf.ReplaceTextOnPage(pageIndex, "[DATE]", "01/01/2000")
' Save your new PDF
pdf.SaveAs("new_sample.pdf")
To see the Text Find and Replace example on our Code Examples page, please visit here
Outlines and Bookmarks
An outline or "bookmark" adds a way to navigate to key pages of a PDF. In Adobe Acrobat Reader these bookmarks (which may be nested) are shown in the application's left sidebar. IronPDF will automatically import existing bookmarks from PDF documents and allow more to be added, edited and nested.
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-15.cs
// Create a new PDF or edit an existing document.
PdfDocument pdf = PdfDocument.FromFile("existing.pdf");
// Add bookmark
pdf.Bookmarks.AddBookMarkAtEnd("Author's Note", 2);
pdf.Bookmarks.AddBookMarkAtEnd("Table of Contents", 3);
// Store new bookmark in a variable to add nested bookmarks to
var summaryBookmark = pdf.Bookmarks.AddBookMarkAtEnd("Summary", 17);
// Add a sub-bookmark within the summary
var conclusionBookmark = summaryBookmark.Children.AddBookMarkAtStart("Conclusion", 18);
// Add another bookmark to end of highest-level bookmark list
pdf.Bookmarks.AddBookMarkAtEnd("References", 20);
pdf.SaveAs("existing.pdf");
' Create a new PDF or edit an existing document.
Dim pdf As PdfDocument = PdfDocument.FromFile("existing.pdf")
' Add bookmark
pdf.Bookmarks.AddBookMarkAtEnd("Author's Note", 2)
pdf.Bookmarks.AddBookMarkAtEnd("Table of Contents", 3)
' Store new bookmark in a variable to add nested bookmarks to
Dim summaryBookmark = pdf.Bookmarks.AddBookMarkAtEnd("Summary", 17)
' Add a sub-bookmark within the summary
Dim conclusionBookmark = summaryBookmark.Children.AddBookMarkAtStart("Conclusion", 18)
' Add another bookmark to end of highest-level bookmark list
pdf.Bookmarks.AddBookMarkAtEnd("References", 20)
pdf.SaveAs("existing.pdf")
To see the Outlines and Bookmarks example on our Code Examples page, please visit here.
Add and Edit Annotations
IronPDF has a plethora of customization on annotations for PDFs. Please refer to the following example showcasing it's capabilities:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-16.cs
// create a new PDF or load and edit an existing document.
var pdf = PdfDocument.FromFile("existing.pdf");
// Create a PDF annotation object
var textAnnotation = new IronPdf.Annotations.TextAnnotation(PageIndex: 0)
{
Title = "This is the major title",
Contents = "This is the long 'sticky note' comment content...",
Subject = "This is a subtitle",
Icon = IronPdf.Annotations.TextAnnotation.AnnotationIcon.Help,
Opacity = 0.9,
Printable = false,
Hidden = false,
OpenByDefault = true,
ReadOnly = false,
Rotatable = true,
};
// Add the annotation "sticky note" to a specific page and location within any new or existing PDF.
pdf.Annotations.Add(textAnnotation);
pdf.SaveAs("existing.pdf");
IRON VB CONVERTER ERROR developers@ironsoftware.com
PDF annotations allow "sticky note" like comments to be added to PDF pages. The AddTextAnnotation
method and TextAnnotation
class allows annotations to be added programmatically. Advanced text annotation features supported include coloring, sizing, opacity, icons, and editing.
Add Backgrounds and Foregrounds
With IronPDF, we can easily merge 2 PDF files, using one as a background or foreground:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-17.cs
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf");
pdf.AddBackgroundPdf(@"MyBackground.pdf");
pdf.AddForegroundOverlayPdfToPage(0, @"MyForeground.pdf", 0);
pdf.SaveAs(@"C:\Path\To\Complete.pdf");
Dim renderer = New ChromePdfRenderer()
Dim pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf")
pdf.AddBackgroundPdf("MyBackground.pdf")
pdf.AddForegroundOverlayPdfToPage(0, "MyForeground.pdf", 0)
pdf.SaveAs("C:\Path\To\Complete.pdf")
Stamping and Watermarking
The ability to do stamping and watermarking are fundamental features of any PDF editor. IronPDF has an amazing API for creating a wide range of stamps such as Image Stamps and HTML Stamps. These all have highly customizable positioning using alignment and offsets which can be seen here:
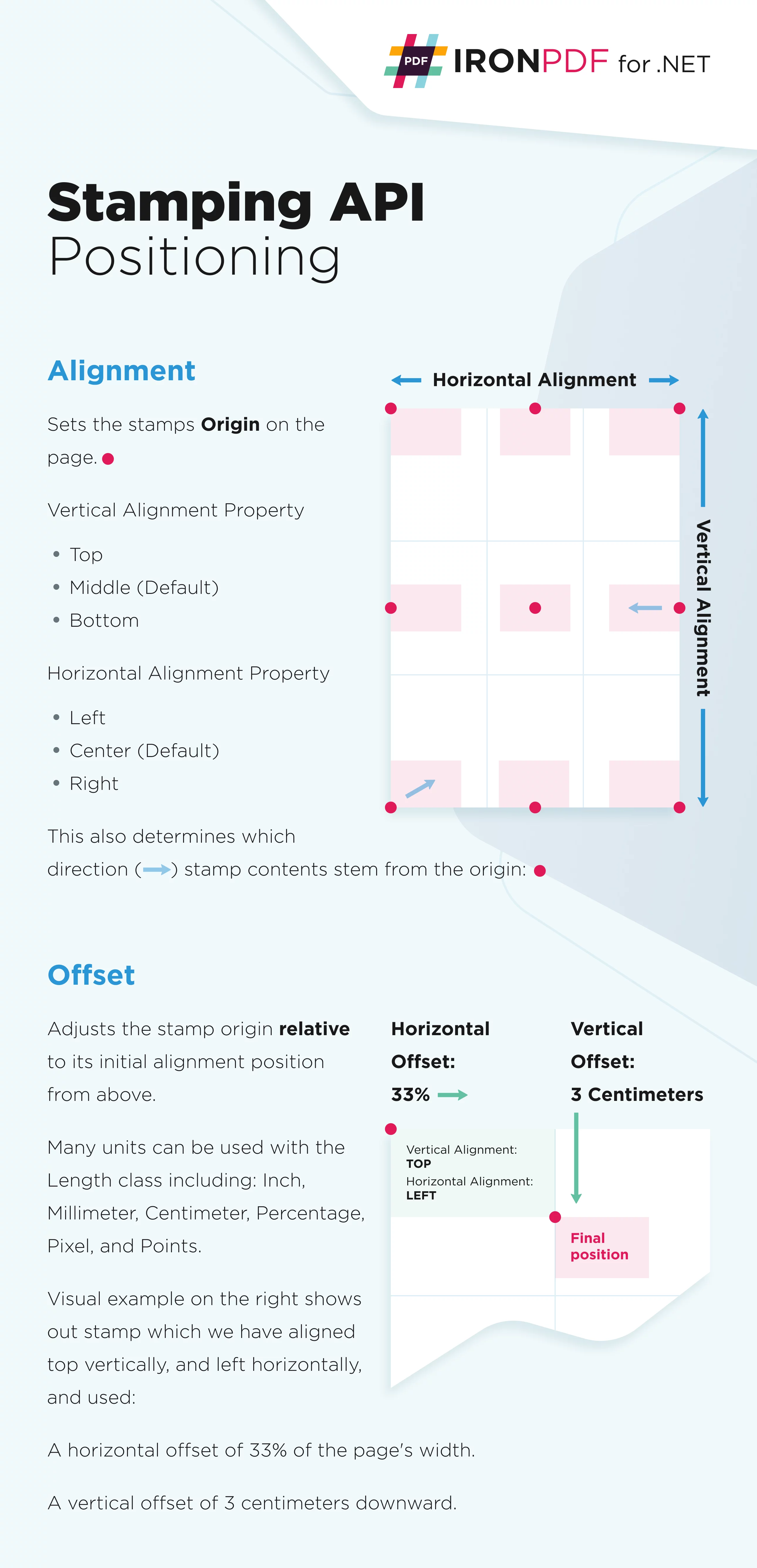
Stamper Abstract Class
The Stamper
abstract class is used as a parameter for all of the IronPDF's stamping methods.
There are many classes for each use-case:
TextStamper
to stamp Text - Text ExampleImageStamper
to stamp Images - Image ExampleHtmlStamper
to stamp HTML - HTML ExampleBarcodeStamper
to stamp Barcodes - Barcode ExampleBarcodeStamper
to stamp QR Codes - QR Code Example- For Watermarking see here.
To apply any of these, use one of our ApplyStamp()
methods. See: the Apply Stamp section of this tutorial.
abstract class Stamper
|
└─── int : Opacity
└─── int : Rotation
|
└─── double : Scale
|
└─── Length : HorizontalOffset
└─── Length : VerticalOffset
|
└─── Length : MinWidth
└─── Length : MaxWidth
|
└─── Length : MinHeight
└─── Length : MaxHeight
|
└─── string : Hyperlink
|
└─── bool : IsStampBehindContent (default : false)
|
└─── HorizontalAlignment : HorizontalAlignment
│ │ Left
│ │ Center (default)
│ │ Right
│
└─── VerticalAlignment : VerticalAlignment
│ Top
│ Middle (default)
│ Bottom
Stamping Examples
Below we showcase every one of Stamper's subclasses with a code example.
Creating two different Text Stampers in different ways and applying them both:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-18.cs
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>");
TextStamper stamper1 = new TextStamper
{
Text = "Hello World! Stamp One Here!",
FontFamily = "Bungee Spice",
UseGoogleFont = true,
FontSize = 100,
IsBold = true,
IsItalic = true,
VerticalAlignment = VerticalAlignment.Top
};
TextStamper stamper2 = new TextStamper()
{
Text = "Hello World! Stamp Two Here!",
FontFamily = "Bungee Spice",
UseGoogleFont = true,
FontSize = 30,
VerticalAlignment = VerticalAlignment.Bottom
};
Stamper[] stampersToApply = { stamper1, stamper2 };
pdf.ApplyMultipleStamps(stampersToApply);
pdf.ApplyStamp(stamper2);
Dim renderer = New ChromePdfRenderer()
Dim pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>")
Dim stamper1 As New TextStamper With {
.Text = "Hello World! Stamp One Here!",
.FontFamily = "Bungee Spice",
.UseGoogleFont = True,
.FontSize = 100,
.IsBold = True,
.IsItalic = True,
.VerticalAlignment = VerticalAlignment.Top
}
Dim stamper2 As New TextStamper() With {
.Text = "Hello World! Stamp Two Here!",
.FontFamily = "Bungee Spice",
.UseGoogleFont = True,
.FontSize = 30,
.VerticalAlignment = VerticalAlignment.Bottom
}
Dim stampersToApply() As Stamper = { stamper1, stamper2 }
pdf.ApplyMultipleStamps(stampersToApply)
pdf.ApplyStamp(stamper2)
Applying an Image Stamp to an existing PDF document on various page combinations:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-19.cs
var pdf = new PdfDocument("/attachments/2022_Q1_sales.pdf");
ImageStamper logoImageStamper = new ImageStamper("/assets/logo.png");
// Apply to every page, one page, or some pages
pdf.ApplyStamp(logoImageStamper);
pdf.ApplyStamp(logoImageStamper, 0);
pdf.ApplyStamp(logoImageStamper, new[] { 0, 3, 11 });
Dim pdf = New PdfDocument("/attachments/2022_Q1_sales.pdf")
Dim logoImageStamper As New ImageStamper("/assets/logo.png")
' Apply to every page, one page, or some pages
pdf.ApplyStamp(logoImageStamper)
pdf.ApplyStamp(logoImageStamper, 0)
pdf.ApplyStamp(logoImageStamper, { 0, 3, 11 })
Write your own HTML to be used as a stamp:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-20.cs
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderHtmlAsPdf("<p>Hello World, example HTML body.</p>");
HtmlStamper stamper = new HtmlStamper($"<p>Example HTML Stamped</p><div style='width:250pt;height:250pt;background-color:red;'></div>")
{
HorizontalOffset = new Length(-3, MeasurementUnit.Inch),
VerticalAlignment = VerticalAlignment.Bottom
};
pdf.ApplyStamp(stamper);
Dim renderer = New ChromePdfRenderer()
Dim pdf = renderer.RenderHtmlAsPdf("<p>Hello World, example HTML body.</p>")
Dim stamper As New HtmlStamper($"<p>Example HTML Stamped</p><div style='width:250pt;height:250pt;background-color:red;'></div>")
If True Then
'INSTANT VB WARNING: An assignment within expression was extracted from the following statement:
'ORIGINAL LINE: HorizontalOffset = new Length(-3, MeasurementUnit.Inch), VerticalAlignment = VerticalAlignment.Bottom
New Length(-3, MeasurementUnit.Inch), VerticalAlignment = VerticalAlignment.Bottom
HorizontalOffset = New Length(-3, MeasurementUnit.Inch), VerticalAlignment
End If
pdf.ApplyStamp(stamper)
Stamp a Barcode onto a PDF
Example of creating and stamping a Barcode:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-21.cs
BarcodeStamper bcStamp = new BarcodeStamper("IronPDF", BarcodeEncoding.Code39);
bcStamp.HorizontalAlignment = HorizontalAlignment.Left;
bcStamp.VerticalAlignment = VerticalAlignment.Bottom;
var pdf = new PdfDocument("example.pdf");
pdf.ApplyStamp(bcStamp);
Dim bcStamp As New BarcodeStamper("IronPDF", BarcodeEncoding.Code39)
bcStamp.HorizontalAlignment = HorizontalAlignment.Left
bcStamp.VerticalAlignment = VerticalAlignment.Bottom
Dim pdf = New PdfDocument("example.pdf")
pdf.ApplyStamp(bcStamp)
Stamp a QR Code onto a PDF
Example of creating and stamping a QR Code:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-22.cs
BarcodeStamper qrStamp = new BarcodeStamper("IronPDF", BarcodeEncoding.QRCode);
qrStamp.Height = 50; // pixels
qrStamp.Width = 50; // pixels
qrStamp.HorizontalAlignment = HorizontalAlignment.Left;
qrStamp.VerticalAlignment = VerticalAlignment.Bottom;
var pdf = new PdfDocument("example.pdf");
pdf.ApplyStamp(qrStamp);
Dim qrStamp As New BarcodeStamper("IronPDF", BarcodeEncoding.QRCode)
qrStamp.Height = 50 ' pixels
qrStamp.Width = 50 ' pixels
qrStamp.HorizontalAlignment = HorizontalAlignment.Left
qrStamp.VerticalAlignment = VerticalAlignment.Bottom
Dim pdf = New PdfDocument("example.pdf")
pdf.ApplyStamp(qrStamp)
Add a Watermark to a PDF
Watermark is a type of stamp for every page that can easily be applied using the ApplyWatermark
method:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-23.cs
var pdf = new PdfDocument("/attachments/design.pdf");
string html = "<h1> Example Title <h1/>";
int rotation = 0;
int watermarkOpacity = 30;
pdf.ApplyWatermark(html, rotation, watermarkOpacity);
Dim pdf = New PdfDocument("/attachments/design.pdf")
Dim html As String = "<h1> Example Title <h1/>"
Dim rotation As Integer = 0
Dim watermarkOpacity As Integer = 30
pdf.ApplyWatermark(html, rotation, watermarkOpacity)
To see the Watermarking example on our Code Examples page, please visit here.
There are a few overloads to the ApplyStamp mehod that can be used to apply your Stamper to a PDF.
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-24.cs
var pdf = new PdfDocument("/assets/example.pdf");
// Apply one stamp to all pages
pdf.ApplyStamp(myStamper);
// Apply one stamp to a specific page
pdf.ApplyStamp(myStamper, 0);
// Apply one stamp to specific pages
pdf.ApplyStamp(myStamper, new[] { 0, 3, 5 });
// Apply a stamp array to all pages
pdf.ApplyMultipleStamps(stampArray);
// Apply a stamp array to a specific page
pdf.ApplyMultipleStamps(stampArray, 0);
// Apply a stamp array to specific pages
pdf.ApplyMultipleStamps(stampArray, new[] { 0, 3, 5 });
// And some Async versions of the above
await pdf.ApplyStampAsync(myStamper, 4);
await pdf.ApplyMultipleStampsAsync(stampArray);
// Additional Watermark apply method
string html = "<h1> Example Title <h1/>";
int rotation = 0;
int watermarkOpacity = 30;
pdf.ApplyWatermark(html, rotation, watermarkOpacity);
Dim pdf = New PdfDocument("/assets/example.pdf")
' Apply one stamp to all pages
pdf.ApplyStamp(myStamper)
' Apply one stamp to a specific page
pdf.ApplyStamp(myStamper, 0)
' Apply one stamp to specific pages
pdf.ApplyStamp(myStamper, { 0, 3, 5 })
' Apply a stamp array to all pages
pdf.ApplyMultipleStamps(stampArray)
' Apply a stamp array to a specific page
pdf.ApplyMultipleStamps(stampArray, 0)
' Apply a stamp array to specific pages
pdf.ApplyMultipleStamps(stampArray, { 0, 3, 5 })
' And some Async versions of the above
Await pdf.ApplyStampAsync(myStamper, 4)
Await pdf.ApplyMultipleStampsAsync(stampArray)
' Additional Watermark apply method
Dim html As String = "<h1> Example Title <h1/>"
Dim rotation As Integer = 0
Dim watermarkOpacity As Integer = 30
pdf.ApplyWatermark(html, rotation, watermarkOpacity)
Length Class
The Length class has two properties: Unit
and Value
. Once you've decided which Unit to use from the MeasurementUnit
enum (the default is Percentage
of the page), then select the Value
to decide the amount of length to use as a multiple of the base unit.
Length Class Properties
class Length
|
└─── double : Value (default : 0)
|
└─── MeasurementUnit : Unit
| Inch
| Millimeter
| Centimeter
| Percentage (default)
| Pixel
| Points
Length Examples
Creating a Length
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-25.cs
new Length(value: 5, unit: MeasurementUnit.Inch); // 5 inches
new Length(value: 25, unit: MeasurementUnit.Pixel);// 25px
new Length(); // 0% of the page dimension because value is defaulted to zero and unit is defaulted to percentage
new Length(value: 20); // 20% of the page dimension
Dim tempVar As New Length(value:= 5, unit:= MeasurementUnit.Inch) ' 5 inches
Dim tempVar2 As New Length(value:= 25, unit:= MeasurementUnit.Pixel) ' 25px
Dim tempVar3 As New Length() ' 0% of the page dimension because value is defaulted to zero and unit is defaulted to percentage
Dim tempVar4 As New Length(value:= 20) ' 20% of the page dimension
Using Length as a parameter
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-26.cs
HtmlStamper logoStamper = new HtmlStamper
{
VerticalOffset = new Length(15, MeasurementUnit.Percentage),
HorizontalOffset = new Length(1, MeasurementUnit.Inch)
// set other properties...
};
Dim logoStamper As New HtmlStamper With {
.VerticalOffset = New Length(15, MeasurementUnit.Percentage),
.HorizontalOffset = New Length(1, MeasurementUnit.Inch)
}
Using Forms in PDFs
Create and Edit Forms
Use IronPDF to create a PDF with embedded form fields:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-27.cs
// Step 1. Creating a PDF with editable forms from HTML using form and input tags
const string formHtml = @"
<html>
<body>
<h2>Editable PDF Form</h2>
<form>
First name: <br> <input type='text' name='firstname' value=''> <br>
Last name: <br> <input type='text' name='lastname' value=''>
</form>
</body>
</html>";
// Instantiate Renderer
var renderer = new ChromePdfRenderer();
renderer.RenderingOptions.CreatePdfFormsFromHtml = true;
renderer.RenderHtmlAsPdf(formHtml).SaveAs("BasicForm.pdf");
// Step 2. Reading and Writing PDF form values.
var formDocument = PdfDocument.FromFile("BasicForm.pdf");
// Read the value of the "firstname" field
var firstNameField = formDocument.Form.FindFormField("firstname");
// Read the value of the "lastname" field
var lastNameField = formDocument.Form.FindFormField("lastname");
' Step 1. Creating a PDF with editable forms from HTML using form and input tags
Const formHtml As String = "
<html>
<body>
<h2>Editable PDF Form</h2>
<form>
First name: <br> <input type='text' name='firstname' value=''> <br>
Last name: <br> <input type='text' name='lastname' value=''>
</form>
</body>
</html>"
' Instantiate Renderer
Dim renderer = New ChromePdfRenderer()
renderer.RenderingOptions.CreatePdfFormsFromHtml = True
renderer.RenderHtmlAsPdf(formHtml).SaveAs("BasicForm.pdf")
' Step 2. Reading and Writing PDF form values.
Dim formDocument = PdfDocument.FromFile("BasicForm.pdf")
' Read the value of the "firstname" field
Dim firstNameField = formDocument.Form.FindFormField("firstname")
' Read the value of the "lastname" field
Dim lastNameField = formDocument.Form.FindFormField("lastname")
To see the PDF Form example on our Code Examples page, please visit here.
Fill Existing Forms
Using IronPDF you can easily access all existing form fields in a PDF and fill them for a resave:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-28.cs
var formDocument = PdfDocument.FromFile("BasicForm.pdf");
// Set and Read the value of the "firstname" field
var firstNameField = formDocument.Form.FindFormField("firstname");
firstNameField.Value = "Minnie";
Console.WriteLine("FirstNameField value: {0}", firstNameField.Value);
// Set and Read the value of the "lastname" field
var lastNameField = formDocument.Form.FindFormField("lastname");
lastNameField.Value = "Mouse";
Console.WriteLine("LastNameField value: {0}", lastNameField.Value);
formDocument.SaveAs("FilledForm.pdf");
Dim formDocument = PdfDocument.FromFile("BasicForm.pdf")
' Set and Read the value of the "firstname" field
Dim firstNameField = formDocument.Form.FindFormField("firstname")
firstNameField.Value = "Minnie"
Console.WriteLine("FirstNameField value: {0}", firstNameField.Value)
' Set and Read the value of the "lastname" field
Dim lastNameField = formDocument.Form.FindFormField("lastname")
lastNameField.Value = "Mouse"
Console.WriteLine("LastNameField value: {0}", lastNameField.Value)
formDocument.SaveAs("FilledForm.pdf")
To see the PDF Form example on our Code Examples page, please visit here.
Conclusion
The list of examples above demonstrates that IronPDF has key features that work out-of-the-box when it comes to editing PDFs.
If you would like to make a feature request or have any general questions about IronPDF or licensing, please contact our support team. We will be more than happy to assist you.