透かしなしで本番環境でテストしてください。
必要な場所で動作します。
30日間、完全に機能する製品をご利用いただけます。
数分で稼働させることができます。
製品トライアル期間中にサポートエンジニアリングチームへの完全アクセス
プロジェクトでOctokit.NETの使用を始めるには、まずパッケージをインストールする必要があります。 それは、最も簡単な方法であるNuGetを使用して追加できます。 Visual Studioでは、NuGetパッケージマネージャーを使用できます。 Octokit
を検索して、プロジェクトにインストールしてください。
以下は、Octokit.NET を使用して GitHub ユーザーに関する情報を取得する方法の簡単な例です。 この例では、すでにOctokit.NETでプロジェクトを設定していることを前提としています。
using Octokit;
using System;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
// Create a new instance of the GitHubClient class
var client = new GitHubClient(new ProductHeaderValue("YourAppName"));
// Retrieve user information
var user = await client.User.Get("octocat");
// Output the user's name
Console.WriteLine("User Name: " + user.Name);
}
}
using Octokit;
using System;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
// Create a new instance of the GitHubClient class
var client = new GitHubClient(new ProductHeaderValue("YourAppName"));
// Retrieve user information
var user = await client.User.Get("octocat");
// Output the user's name
Console.WriteLine("User Name: " + user.Name);
}
}
このコードスニペットは新しいGitHubクライアントを作成し、特定のユーザー「octocat」のリポジトリ名によって情報を取得します。 その後、コンソールにユーザー名を表示します。 これは、ユーザーのユーザー名を使用してGitHubのAPIに認証されたアクセスを示し、認証なしで公開リポジトリにもアクセスできることを示しています。
Octokit.NETを使用して、条件でGitHubリポジトリを検索できます。 検索を実行する方法は次のとおりです:
using Octokit;
using System;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
var client = new GitHubClient(new ProductHeaderValue("YourAppName"));
var searchRepositoriesRequest = new SearchRepositoriesRequest("machine learning")
{
Language = Language.CSharp
};
var result = await client.Search.SearchRepo(searchRepositoriesRequest);
foreach (var repo in result.Items)
{
Console.WriteLine(repo.FullName);
}
}
}
using Octokit;
using System;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
var client = new GitHubClient(new ProductHeaderValue("YourAppName"));
var searchRepositoriesRequest = new SearchRepositoriesRequest("machine learning")
{
Language = Language.CSharp
};
var result = await client.Search.SearchRepo(searchRepositoriesRequest);
foreach (var repo in result.Items)
{
Console.WriteLine(repo.FullName);
}
}
}
このコードは、C#で書かれた「機械学習」に関連するリポジトリを検索します。 リポジトリのフルネームを出力します。
フォークされたリポジトリを管理するには、リストおよび作成することができます。 リポジトリのフォークをリストアップする方法は次のとおりです:
using Octokit;
using System;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
var client = new GitHubClient(new ProductHeaderValue("YourAppName"));
var forks = await client.Repository.Forks.GetAll("octocat", "Hello-World");
foreach (var fork in forks)
{
Console.WriteLine("Fork ID: " + fork.Id + " - Owner: " + fork.Owner.Login);
}
}
}
using Octokit;
using System;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
var client = new GitHubClient(new ProductHeaderValue("YourAppName"));
var forks = await client.Repository.Forks.GetAll("octocat", "Hello-World");
foreach (var fork in forks)
{
Console.WriteLine("Fork ID: " + fork.Id + " - Owner: " + fork.Owner.Login);
}
}
}
この例では、octocat
によって所有されている「Hello-World」リポジトリのすべてのフォークを一覧表示します。
GitHub APIを操作する際に、レート制限を理解し対処することは非常に重要です。 Octokit.NET は、レート制限を確認するためのツールを提供します:
using Octokit;
using System;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
var client = new GitHubClient(new ProductHeaderValue("YourAppName"));
var rateLimit = await client.Miscellaneous.GetRateLimits();
Console.WriteLine("Core Limit: " + rateLimit.Resources.Core.Limit);
}
}
using Octokit;
using System;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
var client = new GitHubClient(new ProductHeaderValue("YourAppName"));
var rateLimit = await client.Miscellaneous.GetRateLimits();
Console.WriteLine("Core Limit: " + rateLimit.Resources.Core.Limit);
}
}
このスニペットは GitHub API 使用のコア制限をチェックし表示します。これにより、レート制限を超えないようリクエストを管理するのに役立ちます。
Octokit.NETはリアクティブ拡張機能に対応しています。(Rx)リアクティブプログラミング用。 以下は基本的な例です:
using Octokit.Reactive;
using System;
var client = new ObservableGitHubClient(new ProductHeaderValue("YourAppName"));
var subscription = client.User.Get("octocat").Subscribe(
user => Console.WriteLine("User Name: " + user.Name),
error => Console.WriteLine("Error: " + error.Message)
);
// Unsubscribe when done
subscription.Dispose();
using Octokit.Reactive;
using System;
var client = new ObservableGitHubClient(new ProductHeaderValue("YourAppName"));
var subscription = client.User.Get("octocat").Subscribe(
user => Console.WriteLine("User Name: " + user.Name),
error => Console.WriteLine("Error: " + error.Message)
);
// Unsubscribe when done
subscription.Dispose();
この例では、非同期的にユーザー情報を取得し、それをリアクティブに処理する方法を示します。
Octokit.NETを使用してGitタグを操作するには、リポジトリからタグを取得できます:
using Octokit;
using System;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
var client = new GitHubClient(new ProductHeaderValue("YourAppName"));
var tags = await client.Repository.GetAllTags("octocat", "Hello-World");
foreach (var tag in tags)
{
Console.WriteLine("Tag Name: " + tag.Name);
}
}
}
using Octokit;
using System;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
var client = new GitHubClient(new ProductHeaderValue("YourAppName"));
var tags = await client.Repository.GetAllTags("octocat", "Hello-World");
foreach (var tag in tags)
{
Console.WriteLine("Tag Name: " + tag.Name);
}
}
}
このコードは、octocat
が所有する"Hello-World"リポジトリのすべてのタグを一覧表示します。
IronPDFは、開発者がC#および.NETアプリケーション内で直接PDFの作成、操作、およびレンダリングを行うことができる人気のある.NETライブラリです。 HTMLや請求書、または固定レイアウト形式が必要な任意のドキュメントからPDFレポートを生成するための強力なツールです。 Octokit.NETと組み合わせると、GitHubのAPIと連携して、特にコードリポジトリに関わるドキュメントプロセスの自動化の可能性が大幅に向上します。
IronPDFとその機能についてもっと知りたい方はIronPDF 公式ウェブサイト. 同社のサイトは、開発プロセスをサポートする包括的なリソースとドキュメントを提供しています。
IronPDFをOctokit.NETと統合する実用的な使用例の一つとして、GitHubリポジトリに保管されているプロジェクトのドキュメントのPDFレポートを自動生成することが挙げられます。 たとえば、特定のリポジトリからすべてのMarkdownファイルを取得し、それらをPDFドキュメントに変換して、コンパイルされた版のドキュメントやリリースノートを好む利害関係者や顧客に配布することができます。
この統合を示す簡単なアプリケーションを作ってみましょう。 アプリケーションは次のタスクを実行します:
Octokit.NETを使用してGitHubに認証および接続します。
特定のリポジトリからファイルを取得します。
これらのファイルをIronPDFを使用してMarkdownからPDFに変換します。
PDFをローカルマシンに保存します。
以下は、C#でこのように記述する方法です:
using Octokit;
using IronPdf;
using System;
using System.Threading.Tasks;
using System.Linq;
class Program
{
static async Task Main(string[] args)
{
// GitHub client setup
var client = new GitHubClient(new ProductHeaderValue("YourAppName"));
var tokenAuth = new Credentials("your_github_token"); // Replace with your GitHub token
client.Credentials = tokenAuth;
// Repository details
var owner = "repository_owner";
var repo = "repository_name";
// Fetch repository content
var contents = await client.Repository.Content.GetAllContents(owner, repo);
// Initialize the PDF builder
var pdf = new ChromePdfRenderer();
// Convert each markdown file to PDF
foreach (var content in contents.Where(c => c.Name.EndsWith(".md")))
{
pdf.RenderHtmlAsPdf(content.Content).SaveAs($"{content.Name}.pdf");
Console.WriteLine($"Created PDF for: {content.Name}");
}
}
}
using Octokit;
using IronPdf;
using System;
using System.Threading.Tasks;
using System.Linq;
class Program
{
static async Task Main(string[] args)
{
// GitHub client setup
var client = new GitHubClient(new ProductHeaderValue("YourAppName"));
var tokenAuth = new Credentials("your_github_token"); // Replace with your GitHub token
client.Credentials = tokenAuth;
// Repository details
var owner = "repository_owner";
var repo = "repository_name";
// Fetch repository content
var contents = await client.Repository.Content.GetAllContents(owner, repo);
// Initialize the PDF builder
var pdf = new ChromePdfRenderer();
// Convert each markdown file to PDF
foreach (var content in contents.Where(c => c.Name.EndsWith(".md")))
{
pdf.RenderHtmlAsPdf(content.Content).SaveAs($"{content.Name}.pdf");
Console.WriteLine($"Created PDF for: {content.Name}");
}
}
}
次の例では、GitHubクライアントを設定し、認証情報を指定した後、リポジトリからコンテンツを取得します。 リポジトリ内の各Markdownファイルに対して、IronPDFはその内容をPDFファイルに変換し、ローカルに保存します。 このシンプルながら効果的なワークフローは、より複雑なフィルタリング、フォーマット、あるいは大規模なリポジトリ向けのファイルのバッチ処理を含むように拡張することができます。
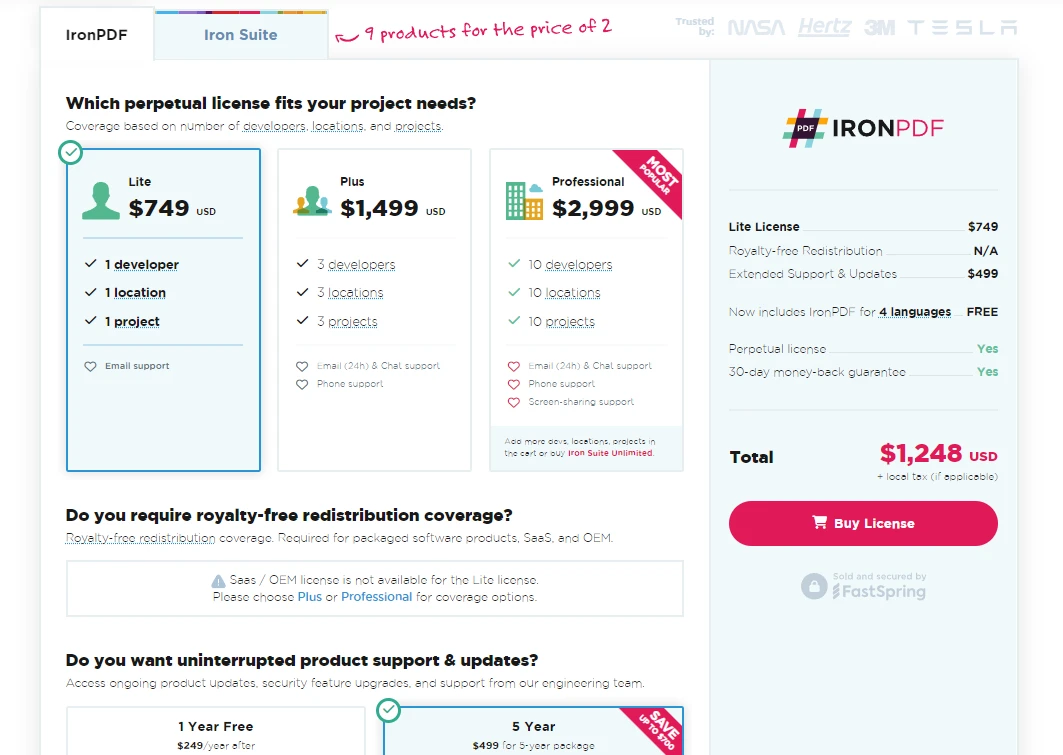
Octokit.NETとIronPDFを統合することで、GitHubプロジェクト内でのドキュメントワークフローを自動化および効率化するためのシームレスなアプローチが提供されます。 これらのツールを活用することで、文書処理の効率を向上させ、様々な専門的ニーズに対応するフォーマットで簡単にアクセス可能にすることができます。 特にIronPDFはPDF操作のための堅牢なプラットフォームを提供し、無料トライアルを提供していることは注目に値します。 プロジェクトに実装することを決定された場合、ライセンスは $749 から始まります。
IronPDFやIronBarcode、IronOCR、IronWebScraperなどのライブラリを含むIron Softwareの製品についての詳細は、こちらをご覧ください。Ironソフトウェア製品ライブラリ.