在实际环境中测试
在生产中测试无水印。
随时随地为您服务。
C# 中用于构建基本独立 Web 服务器最有用的工具之一是 HttpListener 类。它包含在 System.Net 命名空间中,并提供了一种接收和回复方法。 超文本传输协议 来自客户的请求。这对于管理桌面程序中的基于网页的通信或构建轻量级的在线服务特别有用。
一个名为 IronPDF 用于从PDF文件中生成、修改和提取内容。它提供了创建PDF(从HTML),将现有的PDF转换为不同格式,以及使用编程修改PDF的全面功能。
开发人员可以结合HttpListener和IronPDF设计网络服务,以动态生成并响应HTTP请求提供PDF文档。需要根据用户输入或其他动态数据实时生成PDF的应用程序可能会发现这非常有用。
HttpListener ```Chinese 是一個簡單但靈活的類,屬於.NET框架的System.Net命名空間,允許開發者使用C#設計簡單的HTTP服務器。其目的是接收客戶的HTTP請求,處理它們並返回正確的信息。由於該類不需要完整功能的網絡服務器(如IIS),因此它是輕量級獨立網絡服務或將網絡通信功能集成到桌面程序中的一個很好的選擇。
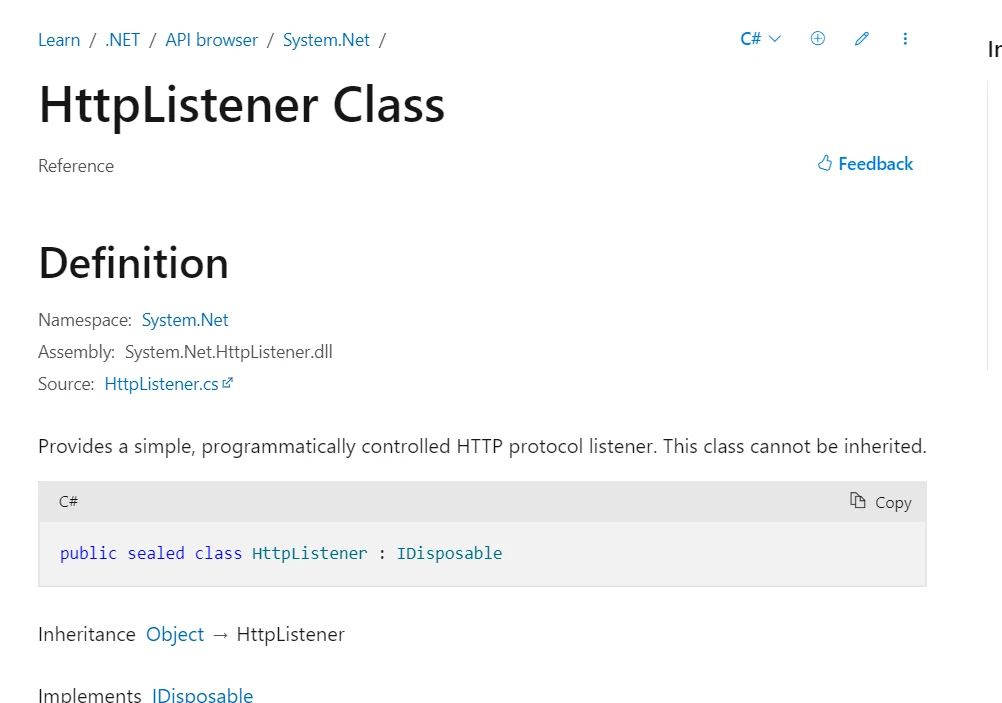
开发人员可以使用HttpListener设置URI前缀,以确定服务器应监听哪些地址。一旦监听器启动,它会响应所有传入的请求并使用HttpListenerContext来访问请求和响应对象。此配置使得可以创建特定于应用程序需求的HTTP请求处理逻辑。HttpListener的易用性和适应性使其在需要快速、有效和可配置的HTTP服务器的情况下特别有用。HttpListener提供了一个稳定的解决方案,没有额外开销,用于开发本地服务器进行测试、原型化在线服务,或将通信协议集成到桌面应用程序中。
### HttpListener C# 的功能
C# 的 HttpListener 具有许多功能,使其成为构建 HTTP 服务器的有效工具。其主要元素包括:
**易用性**: HttpListener 是一个易于使用的库,程序员可以编写更少的代码来建立基本的 HTTP 服务器。
**URI 前缀**: 可以指定多个 URI 前缀进行监听,为处理各种端点提供灵活性,并确保服务器仅对相关查询做出反应。
**异步操作**: HttpListener 支持异步方法,通过有效地同时处理多个请求而不中断主线程,从而增强了服务器的可扩展性和响应能力。
**身份验证**: HttpListener 支持多种身份验证技术,如 Basic、Digest、NTLM 和集成 Windows 身份验证,使您可以根据需要保护您的端点。
**HTTPS 支持**: 可以设置 HttpListener 以响应 HTTPS 请求,例如,启用安全的客户端-服务器数据通信。
**请求和响应处理**: HttpListener 让您完全控制请求和响应过程,可以通过添加新头、状态码和内容类型,读取请求数据、头和参数来修改响应。
**监听器配置**: HttpListener 提供特定于监听器的配置选项来调整服务器行为,例如证书管理。 (用于HTTPS), 超时和其他参数。
**日志记录和诊断:** 启用日志记录和诊断,提供全面的请求和响应信息,有助于监控和故障排除。
**兼容性:** 允许与当前 .NET 服务和应用程序无缝集成,因为它很好地与其他 .NET 组件和库配合使用。
**跨平台:** HttpListener 兼容 Windows、Linux 和 macOS,并且可与 .NET Core 和 .NET 5+ 一起使用,提供跨平台开发的灵活性。
## 创建和配置 HttpListener C#
创建和配置 HttpListener 在 C# 中涉及多个步骤。以下是一个关于配置 HttpListener 来处理 HTTP 请求的详细教程。
**创建一个新的 .NET 项目**
打开您的命令提示符、控制台或终端。
通过键入启动新创建的 .NET 控制台应用程序
```cs
dotnet new console -n HttplistenerExample
cd HttplistenerExample
创建 HttpListener 实例
首先,创建 HttpListener 类的实例。
配置 URI 前缀
添加 URI 前缀以指定监听器应处理的地址。
启动监听器
启动 HttpListener 以开始侦听传入的 HTTP 请求。
处理传入请求
创建一个循环来处理传入请求,处理它们并发送响应。
停止监听器
当不再需要时,优雅地停止 HttpListener。
以下是这些阶段中一个请求的操作示例:
using System;
using System.Net;
using System.Text;
class Program
{
public static string url="http://localhost:8080/";
public static HttpListener listener;
public static void Main(string[] args)
{
// Step 1: Create an HttpListener instance
listener = new HttpListener();
// Step 2: Configure URI prefixes
listener.Prefixes.Add(url);
// Step 3: Start the listener
listener.Start();
Console.WriteLine("Listening for requests on "+url);
// Step 4: Handle incoming requests
// Wait for an incoming request
while (true)
{
// getcontext method blocks
HttpListenerContext context = listener.GetContext();
HttpListenerRequest request = context.Request;
// Process the request (e.g., log the request URL with or without query string)
Console.WriteLine($"Received request for {request.Url}");
// Create a response
HttpListenerResponse response = context.Response;
// add response info
string responseString = "<html><body>Hello, world!</body></html>";
byte[] buffer = Encoding.UTF8.GetBytes(responseString);
// Set the content length and type
response.ContentLength64 = buffer.Length;
response.ContentType = "text/html";
// Write the response to the output stream
using (System.IO.Stream output = response.OutputStream)
{
output.Write(buffer, 0, buffer.Length);
}
// Close the response
response.Close();
}
// Step 5: Stop the listener (this code is unreachable in the current loop structure)
// listener.Stop();
}
}
using System;
using System.Net;
using System.Text;
class Program
{
public static string url="http://localhost:8080/";
public static HttpListener listener;
public static void Main(string[] args)
{
// Step 1: Create an HttpListener instance
listener = new HttpListener();
// Step 2: Configure URI prefixes
listener.Prefixes.Add(url);
// Step 3: Start the listener
listener.Start();
Console.WriteLine("Listening for requests on "+url);
// Step 4: Handle incoming requests
// Wait for an incoming request
while (true)
{
// getcontext method blocks
HttpListenerContext context = listener.GetContext();
HttpListenerRequest request = context.Request;
// Process the request (e.g., log the request URL with or without query string)
Console.WriteLine($"Received request for {request.Url}");
// Create a response
HttpListenerResponse response = context.Response;
// add response info
string responseString = "<html><body>Hello, world!</body></html>";
byte[] buffer = Encoding.UTF8.GetBytes(responseString);
// Set the content length and type
response.ContentLength64 = buffer.Length;
response.ContentType = "text/html";
// Write the response to the output stream
using (System.IO.Stream output = response.OutputStream)
{
output.Write(buffer, 0, buffer.Length);
}
// Close the response
response.Close();
}
// Step 5: Stop the listener (this code is unreachable in the current loop structure)
// listener.Stop();
}
}
IRON VB CONVERTER ERROR developers@ironsoftware.com
包含的 C# 代码演示了创建和配置 HttpListener 的过程,该过程的功能是作为一个基础的 HTTP 服务器。要定义它将处理请求的地址,首先要实例化一个 HttpListener 对象并附加一个 URI 前缀。 (http://localhost:8080/)接下来,使用 Start 方法启动监听器。我们使用一个无限循环来持续监听新的 HTTP 请求。在循环中,GetContext 等待请求并返回一个包含请求和响应对象的 HttpListenerContext 对象。
记录请求 URL 后,创建一个简洁的 HTML 和响应对象,将其转换为字节数组并发送到响应输出流。在将响应返回给客户端之前,响应的类型和持续时间会被正确指定。循环表示服务器不断处理请求,一个接一个。需要调用 halt 方法来停止请求控制台监听器,但在这种情况下,无限循环阻止它被触及。
IronPDF 帮助您在 .NET 中创建和修改高质量的 PDF 文件,您需要这些文件来创建文档和报告。HttpListener 内置的 HTTP 服务器功能允许您管理小型应用程序或服务中的 Web 请求。两种工具在各自领域提高了 .NET 应用程序的实用性和速度。要开始使用 C# 的 HttpListener 并将其与 IronPDF 集成以创建 PDF,请执行以下操作:
这款功能丰富的 .NET 库 IronPDF 允许C#程序生成、读取和编辑PDF文档。借助这一工具,开发人员可以快速将HTML、CSS和JavaScript材料转化为高质量、可打印的PDF。最关键的任务包括添加页眉和页脚、分割和合并PDF、为文档添加水印以及将HTML转换为PDF。IronPDF对各种应用程序都很有帮助,因为它支持.NET Framework和.NET Core。
由于PDF易于使用且包含大量信息,开发人员可以轻松将它们集成到他们的产品中。由于IronPDF能够处理复杂的数据布局和格式化,它生成的PDF与客户端或原始HTML文本看起来非常相似。
从HTML生成PDF
将JavaScript、HTML和CSS转换为PDF。IronPDF支持媒体查询和响应式设计,这两种现代网页标准。它对现代网页标准的支持对于使用HTML和CSS动态装饰PDF报告、发票和文档非常有用。
PDF编辑
可以在现有的PDF中添加文本、图片和其他内容。使用IronPDF,开发者可以从PDF文件中提取文本和图像,将多个PDF合并成一个文件,将PDF文件拆分为多个单独的文档,并在PDF页面上添加水印、注释、页眉和页脚。
PDF转换
将包括Word、Excel和图片文件在内的多种文件格式转换为PDF。IronPDF还支持PDF到图像的转换。 (PNG、JPEG 等。)性能和可靠性
在工业环境中,高性能和可靠性是理想的设计品质。开发者可以轻松管理大量文档集。
要在 .NET 项目中使用 PDF 工具,请安装 IronPDF 包。
dotnet add package IronPdf
dotnet add package IronPdf
'INSTANT VB TODO TASK: The following line uses invalid syntax:
'dotnet add package IronPdf
这是一个全面的示例,向您展示如何使用 IronPDF 创建和提供 PDF 文档并设置 HttpListener:
using System;
using System.Net;
using System.Text;
using IronPdf;
class Program
{
static void Main(string[] args)
{
// Step 1: Create an HttpListener instance
HttpListener listener = new HttpListener();
// Step 2: Configure URI prefixes
listener.Prefixes.Add("http://localhost:8080/");
// Step 3: Start the listener
listener.Start();
Console.WriteLine("Listening for requests on http://localhost:8080/");
// Step 4: Handle incoming requests
while (true)
{
// Wait for an incoming request
HttpListenerContext context = listener.GetContext();
HttpListenerRequest request = context.Request;
// Process the request (e.g., log the request URL)
Console.WriteLine($"Received request for {request.Url}");
// Generate PDF using IronPDF
var htmlContent = "<h1>PDF generated by IronPDF</h1><p>This is a sample PDF document.</p>";
var pdf = IronPdf.HtmlToPdf.StaticRenderHtmlAsPdf(htmlContent);
// Get the PDF as a byte array
byte[] pdfBytes = pdf.BinaryData;
// Create a response
HttpListenerResponse response = context.Response;
// Set the content length and type
response.ContentLength64 = pdfBytes.Length;
response.ContentType = "application/pdf";
// Write the PDF to the response output stream
using (System.IO.Stream output = response.OutputStream)
{
output.Write(pdfBytes, 0, pdfBytes.Length);
}
// Close the response
response.Close();
}
// Step 5: Stop the listener (this code is unreachable in the current loop structure)
// listener.Stop();
}
}
using System;
using System.Net;
using System.Text;
using IronPdf;
class Program
{
static void Main(string[] args)
{
// Step 1: Create an HttpListener instance
HttpListener listener = new HttpListener();
// Step 2: Configure URI prefixes
listener.Prefixes.Add("http://localhost:8080/");
// Step 3: Start the listener
listener.Start();
Console.WriteLine("Listening for requests on http://localhost:8080/");
// Step 4: Handle incoming requests
while (true)
{
// Wait for an incoming request
HttpListenerContext context = listener.GetContext();
HttpListenerRequest request = context.Request;
// Process the request (e.g., log the request URL)
Console.WriteLine($"Received request for {request.Url}");
// Generate PDF using IronPDF
var htmlContent = "<h1>PDF generated by IronPDF</h1><p>This is a sample PDF document.</p>";
var pdf = IronPdf.HtmlToPdf.StaticRenderHtmlAsPdf(htmlContent);
// Get the PDF as a byte array
byte[] pdfBytes = pdf.BinaryData;
// Create a response
HttpListenerResponse response = context.Response;
// Set the content length and type
response.ContentLength64 = pdfBytes.Length;
response.ContentType = "application/pdf";
// Write the PDF to the response output stream
using (System.IO.Stream output = response.OutputStream)
{
output.Write(pdfBytes, 0, pdfBytes.Length);
}
// Close the response
response.Close();
}
// Step 5: Stop the listener (this code is unreachable in the current loop structure)
// listener.Stop();
}
}
IRON VB CONVERTER ERROR developers@ironsoftware.com
所包含的C#代码显示了如何连接 IronPDF 通过 HttpListener 动态生成和传递 PDF 文档,以及如何将其设置为基本的 HTTP 方法服务器。第一步是创建一个 HttpListener 实例并将其设置为监听 http://localhost:8080/ 上的 HTTP 请求。
启动监听器后,一个无限循环接管以处理传入的请求。代码记录每个请求的请求 URL,使用 IronPDF 从 HTML 文本创建 PDF 文档,然后将 PDF 转换为字节数组。接下来,用适当的 MIME 类型设置响应。 (应用程序/pdf) 和内容长度。
在将 PDF 字节数组写入响应输出流后,关闭第一个响应流将其发送回客户端。通过这种配置,服务器可以有效地响应 HTTP 请求并返回动态创建的 PDF 文档。
总而言之,使用IronPDF和C#的HttpListener可以可靠地动态创建和通过HTTP传递PDF文件。借助HttpListener,C#应用程序可以创建轻量级HTTP服务器,能够处理传入请求并提供灵活的响应生成。通过利用IronPDF的动态HTML到PDF转换功能,开发人员可以直接从服务器端逻辑中有效地产生自定义或数据驱动的PDF报告、发票或其他文档。
需要通过Web接口或API进行实时文档生成和传递的应用程序可能会发现这种组合特别有用。开发人员可以通过使用HttpListener和IronPDF实施可扩展且响应迅速的解决方案来满足特定的业务需求。这些工具通过促进文档的无缝生成和传递来增强用户体验。
通过使用OCR、处理条形码、创建PDF、连接Excel以及更多功能,您可以通过IronPDF改善您的.NET开发工具箱。 铁软件(Iron Software) 通过将其基本基础与高度适应的Iron Software套件和技术相结合,提供更高效的开发,为开发人员提供更卓越的网络应用和能力,起价为$749。
通过清晰地概述适合项目的许可选项,将简化开发人员选择最佳模型的过程。这些优势使开发人员能够以高效、及时和协调的方式应用解决方案来应对各种问题。