How to Convert a PDF to an Image File
To convert PDF files to images, use the rasterizeToImageFiles
method provided by IronPDF's NodeJS module. You can configure this method to support a variety of PDF-to-Image conversion operations. Convert PDFs to JPG, PNG, and other image formats. Convert every PDF page to a JPEG or PNG image, or convert only a few pages. IronPDF gives you full control.
Continue reading to learn how to do PDF to image tasks with IronPDF for Node.js!
Install IronPDF using NPM
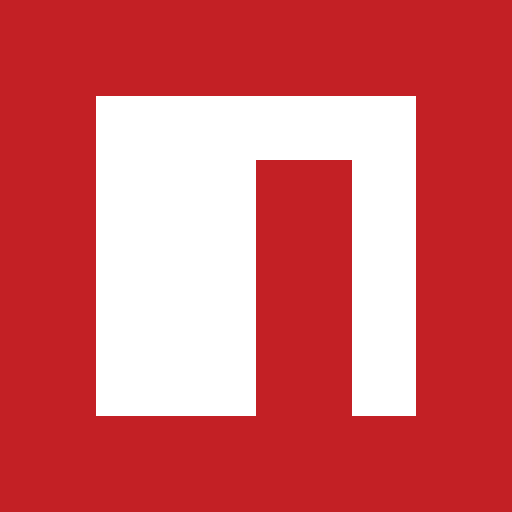
Install with npm
npm i @ironsoftware/ironpdf
Install IronPDF's Nodejs module from NPM to convert PDFs to PNG, JPG (or JPEG), GIF, and other image types.
Converting PDF to Image Format
Let's assume that we are working with a one-page sample PDF document containing placeholder text.
An image depicting our sample PDF file opened in a PDF viewer application. Download this PDF file and others for testing purposes from the Learning Container: https://www.learningcontainer.com/
The source code below converts the PDF file to a PNG file.
import {PdfDocument} from "@ironsoftware/ironpdf";
// Convert PDF File to a PNG File
await PdfDocument.fromFile("./sample-pdf-file.pdf").then((resolve) => {
resolve.rasterizeToImageFiles("./images/sample-pdf-file.png");
return resolve;
});
We use the PdfDocument.fromFile
method to load our sample document into the Node library. This function gives a PdfDocument
representing our sample file. Since the object we need is contained in a Promise, we attach a callback function to run when the promise resolves the PdfDocument
.
Inside the callback, we call the rasterizeToImageFiles
method on the resolved object to convert the single-page document into an image. As shown above, we specify the destination path (which includes the filename and file extension) for our new image as an argument.
The image was generated from the source code above. IronPDF converted our sample PDF into a PNG file in as little as three lines of code!
Learning Container provides sample PDF files that you can use in your projects for testing purposes. You can download the sample PDF file used in this example for free (along with similar sample files) from the website. Feel free to try the example above on other PDFs with different sizes and complexity.
The next section provides additional PDF-to-image conversion details worth considering.
Advanced Image Conversion Options
Convert PDF to JPEG
By default, rasterizeToImageFiles
converts documents according to the file type specified in the destination path.
As such, to convert our sample PDF used in the previous example to a JPG file (instead of converting PDF to a PNG), we can simply change the file name extension used in the destination file path:
// Convert PDF to JPG (not to PNG)
pdf.rasterizeToImageFiles("./images/pdf-to-jpeg.jpg");
Another way to do the same thing is to specify a(n) ImageType
. An ImageType
value supersedes the image file type declared in the destination path. This forces rasterizeToImageFiles
to not consider the filename when performing image-to-PDF tasks.
You can see this in action in the next example. Here, we include a JSON options argument with our call to rasterizeToImageFiles
that declares an ImageType
.
import {PdfDocument, ImageType} from "@ironsoftware/ironpdf";
// Convert PDF to JPEG Format using ImageType.JPG
const options = {
type: ImageType.JPG
};
await PdfDocument.fromFile("./sample-pdf-file.pdf").then((resolve) => {
pdf.rasterizeToImageFiles("./images/pdf-to-jpeg.png", options);
return resolve;
});
Running the program above also creates a JPG image as in our previous example. Notice, however, that the destination filename still uses the PNG file extension. rasterizeToImageFiles
ignored the .PNG file name extension used in the path, following instead the ImageType.JPG type value.
You can adapt this example to convert PDF into other image types, including GIF format and Bitmap format.
Tip: Using this approach can be particularly useful in situations in which changing filenames to specific types is not feasible or desired.
Converting PDF Files with Multiple Pages
To convert documents containing more than one page into a desired image type (PNG, JPG, Bitmap, etc.), we can also use the rasterizeToImageFiles
method in the same manner as before. When invoked, the method will each page as a separate image in the specified type.
A two-page sample PDF document.
The next block of sample code generates two PNG files from the two-page document shown above.
import {PdfDocument} from "@ironsoftware/ironpdf";
// Convert PDF with two pages to a set of images.
await PdfDocument.fromFile("./multipage-pdf.pdf").then((pdf) => {
pdf.rasterizeToImageFiles("./images/multipage-pdf/multipage-pdf-page.png");
});
The result of using the
rasterizeToImageFiles
method on a two-page PDF file. The method creates an image for each page of the original file.
Convert Specific PDF Pages to Images
Declaring a JSON object with the fromPages
property set allows us to rasterize one or more pages from a multipage document (rather than all pages).
The following code example only converts the first, fourth, sixth, and ninth page of this large sample file into bitmaps.
import {PdfDocument, ImageType} from "@ironsoftware/ironpdf";
// Convert PDF containing many pages to BMP images.
const options = {
type: ImageType.BMP,
fromPages: [0, 3, 5, 8] // Select only the pages with an image on it.
}
await PdfDocument.fromFile("./sample-pdf-with-images.pdf").then((pdf) => {
pdf.rasterizeToImageFiles("./images/multipage-selective-pdf/multipage-pdf-page.bmp", options);
});
IronPDF performed the PDF-to-Image operation on only the pages that we specified in the
options
argument.
Further Reading
API Reference
Read the API documentation on the PdfDocument
class and its rasterizeToImageFiles
methods for more insights on how to adapt the method to suit your needs.
Code Examples
- Convert a PDF to Images: See
rasterizeToImageFiles
used in a slightly different way. - Images to PDF: Learn how to convert one or more images into a single PDF file.