Published March 29, 2023
How to Generate PDF Files From Java Applications Dynamically
In this tutorial, we will learn to create PDF files dynamically in Java applications. We will see the code examples for creating PDF pages from text, URL, and HTML pages. We will also learn to create a password-protected PDF file from new document instance.
Java is a popular programming language for large-scale and enterprise applications. The PDF format, which stands for Portable Document Format, gives people an easy, dependable way to present and exchange documents - regardless of the software, hardware, or operating systems that anyone viewing the document is using. As a result, PDF is a popular format for generating documents/PDF forms in software applications. Therefore, it is necessary for a Java Application Developer to know about generating document dynamically.
We need a third-party Java library to create a PDF file. There are numerous libraries available, each with its own set of advantages and disadvantages such as iText open source library, IronPDF, PDFBox library and so on. Some are paid, some do not provide sufficient functionality, some do not provide accuracy, and some have performance issues.
Tthe IronPDF library is ideal for this purpose because it is free for development, more secure, provides all functionalities in a single library with 100% accuracy, and performs exceptionally well.
Before moving forward, let's have a brief introduction about IronPDF.
How to Generate PDF Files From Java Applications Dynamically
IronPDF
IronPDF is the most popular Java PDF library developed by IronSoftware for creating PDF, editing new file, and manipulating the existing PDF. It is designed to be compatible with a wide range of JVM languages, including Java, Scala, and Kotlin, and it can run on a wide range of platforms, including Windows, Linux, Docker, Azure, and AWS. IronPDF works with popular IDEs such as JetBrains, IntelliJ, IDEA and Eclipse.
The main features include the ability to create a PDF file from HTML, HTTP, JavaScript, CSS, XML documents and various image formats. In addition, we can include headers and footers, create tables, digital signatures, attachments, passwords, and security. It supports complete multithreading and much more!
Now, let us begin with the code examples for creating dynamic documents.
First of all, we need to create a new Maven repository project.
Create a New Java Project
I will use IntelliJ IDE for this tutorial. You can use any of your choices. Steps for creating a new Java project may differ from IDE to IDE. Use the following steps:
- Launch the IntelliJ IDE.
- Select File > New > Project.
- Enter Project Title
- Choose a location, a language, a build system, and a JDK.
Click the Create Button.
Create a Project
Name your project, choose a location, a language, a build system, and a JDK. Select the Create button option. A new project will be created.
Now, we need to install IronPDF in our Java application.
Install IronPDF Java library
We need to add a dependency in the pom.xml file for installing IronPDF. Add the following XML source code in the pom.xml file as shown below.
<dependency>
<groupId>com.ironsoftware</groupId>
<artifactId>com.ironsoftware</artifactId>
<version>2024.3.1</version>
</dependency>
Build the project now. Our application will automatically install the library from the Maven repository.
Let's begin with the straightforward example of converting an HTML string into a PDF file.
Create PDF Documents
Consider the following example:
public static void main(String[] args) throws IOException, PrinterException {
String htmlString = "<h1>My First PDF File<h1/><p> This is sample PDF file</p>";
//document class
PdfDocument myPdf = PdfDocument.renderHtmlAsPdf(htmlString);
// Save the PdfDocument to a file
try {
myPdf.saveAs(Paths.get("myPDF.pdf") );
} catch (IOException e) {
throw new RuntimeException(e);
}
}
In the above function, we have assigned the HTML content to a string variable. The renderHtmlAsPdf
method takes a string as an argument and converts HTML content into a PDF document instance. The saveAs
method accepts the location path as an input and saves the instance of the PDF file in the directory we've specified.
The PDF produced by the aforementioned code is shown below.
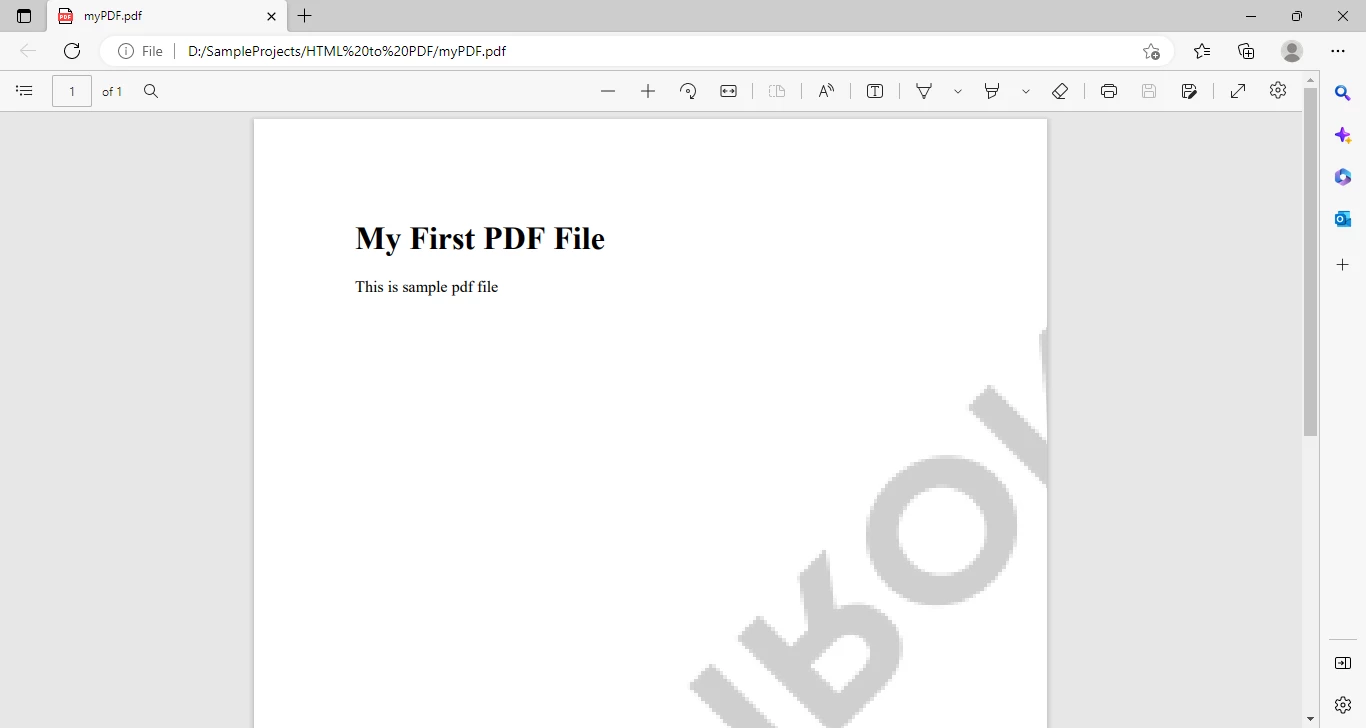
Output
Generate PDF File from HTML File
IronPDF also provides the amazing functionality of generating PDF files from HTML files.
The sample HTML file that will be used in the example is shown below.
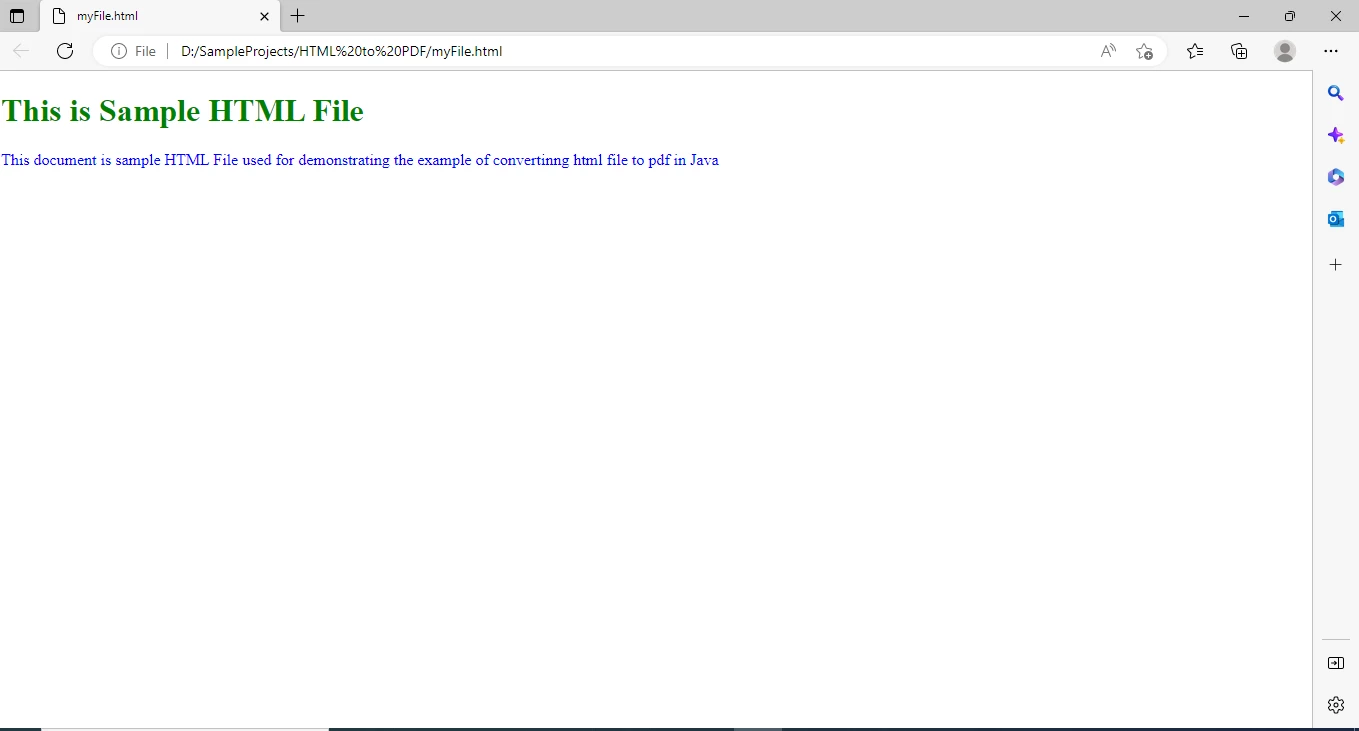
Rendered HTML with new paragraph
The following is the sample code snippet for generating PDFs:
PdfDocument myPdf = PdfDocument.renderHtmlFileAsPdf("myFile.html");
// Save the PdfDocument to a file
try {
myPdf.saveAs("myPDF.pdf");
} catch (IOException e) {
throw new RuntimeException(e);
}
The renderHtmlFileAsPdf
method accepts the path to the HTML file as an argument and produces a PDF document from the HTML file. This PDF file is afterwards saved using the saveAs
method to a local drive.
The document that our program generated in PDF format is shown below.
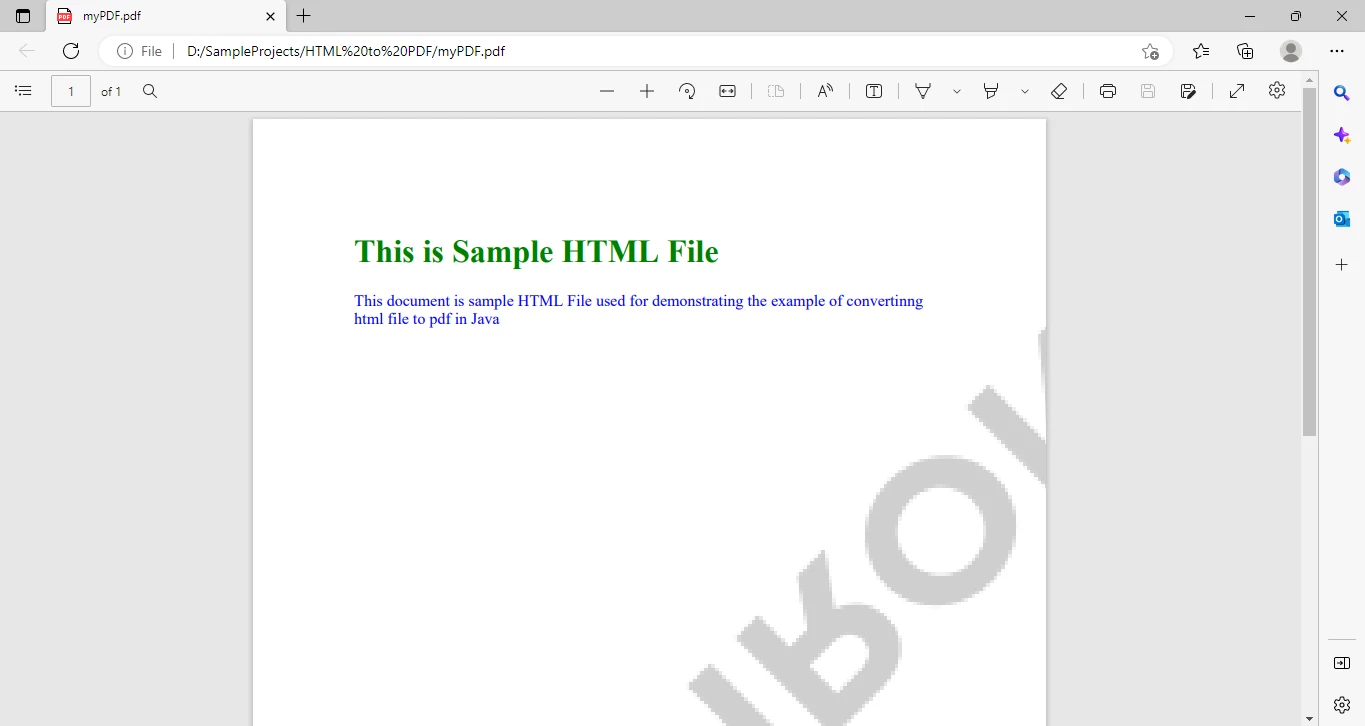
PDF Output
We'll now use a sizable HTML document that contains JavaScript and CSS. We'll check the accuracy and consistency of the design as it converts HTML to PDF.
Generate PDF files from HTML files
We will use the following sample HTML page, which has images, animation, styling, jQuery, and Bootstrap.
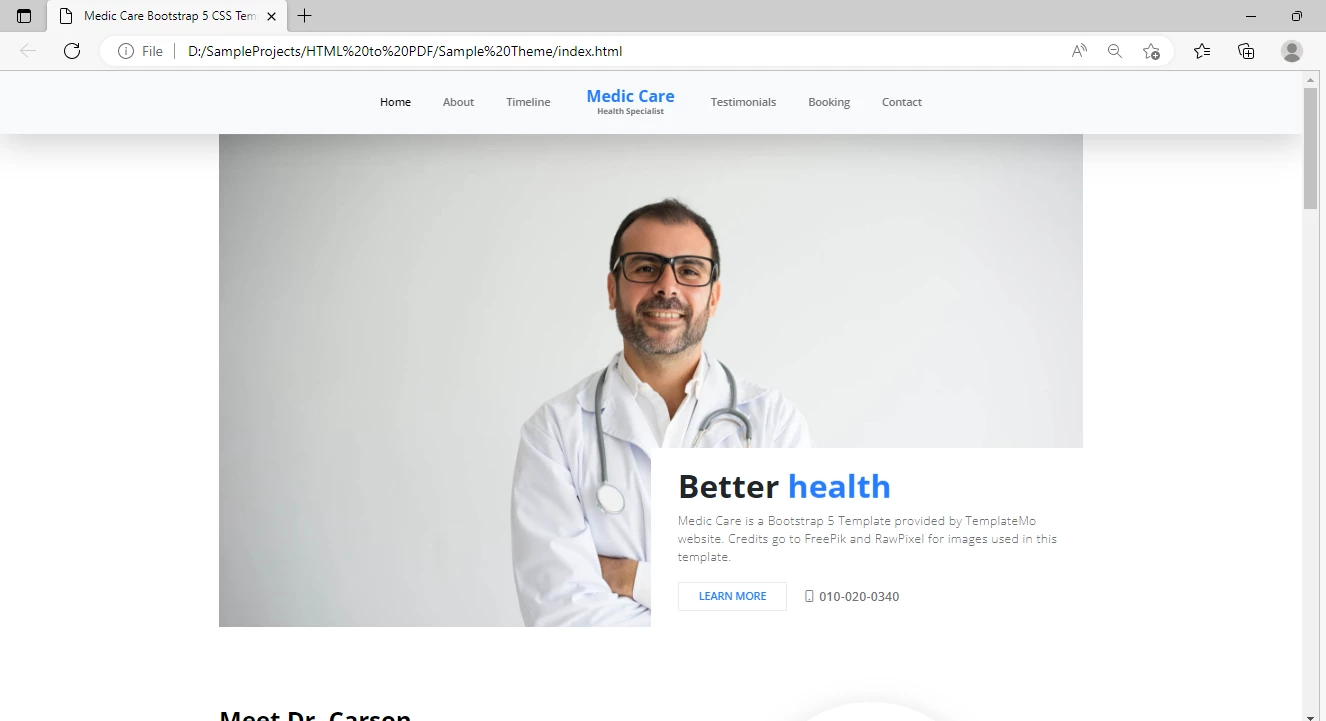
Sample HTML Page
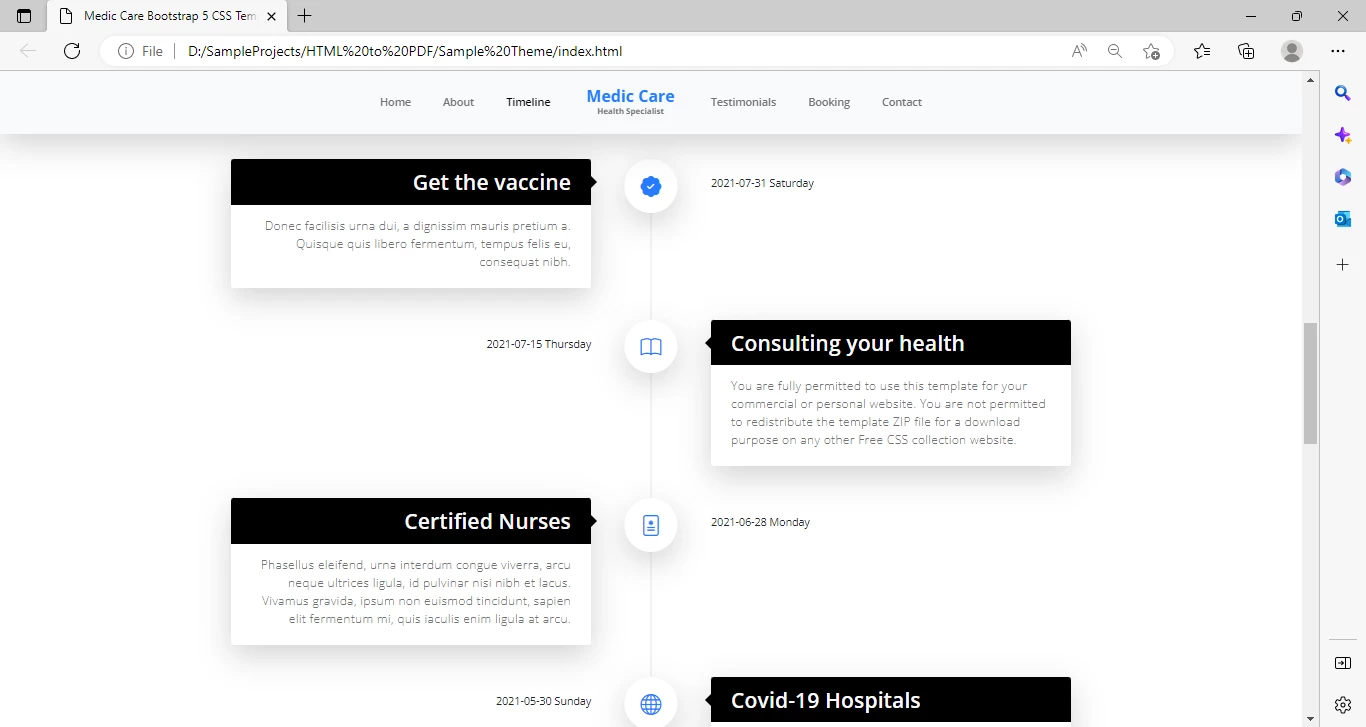
Sample HTML
We can observe that the sample HTML document has extensive styling and includes graphics. This HTML file will be converted into a PDF document, and the accuracy of the content and styling will be evaluated.
The same line of code from the example above will be used.
PdfDocument myPdf = PdfDocument.renderHtmlFileAsPdf("index.html");
// Save the PdfDocument to a file
try {
myPdf.saveAs("myPDF.pdf");
} catch (IOException e) {
throw new RuntimeException(e);
}
The previous example already includes a code explanation. The rest is unchanged; we have only modified the path.
This is our output PDF file:
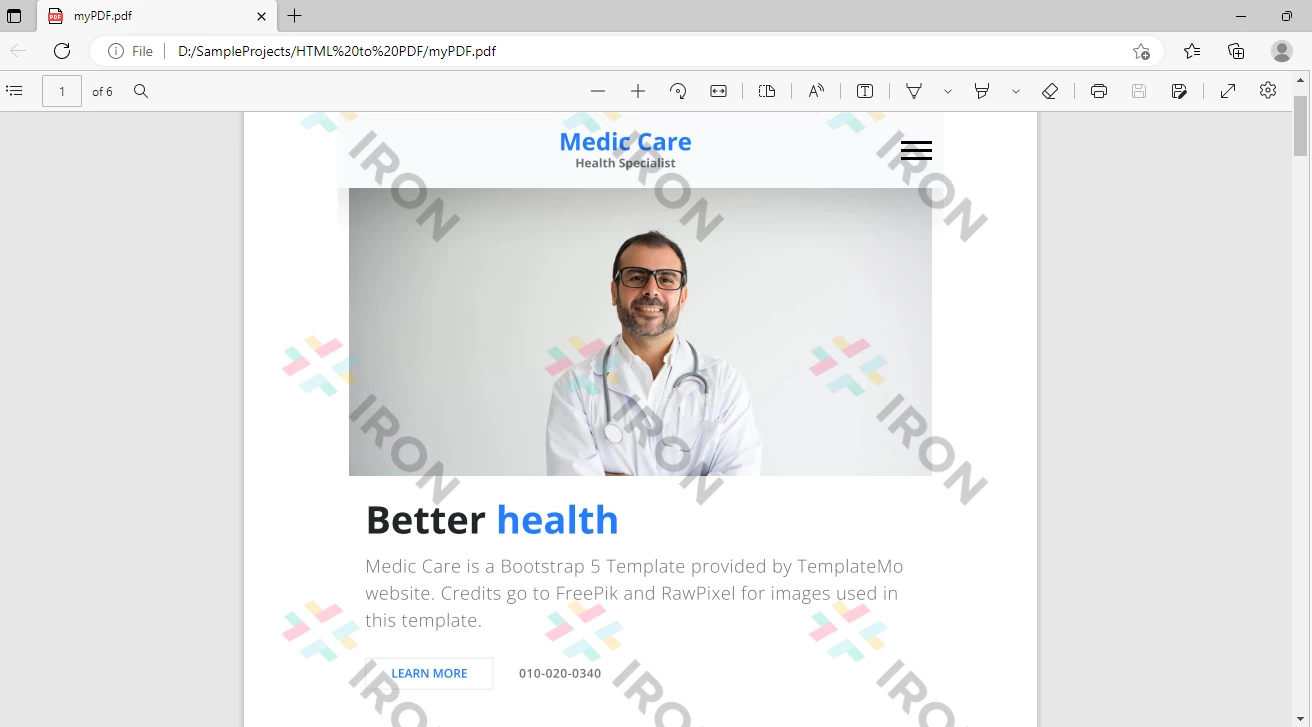
HTML to PDF
We can see that using IronPDF to create PDF files is quite simple. The source document's format and content are both consistent.
A URL can also be used to create a PDF file.
Convert URL to PDF document
The following code sample will generate a PDF file from a URL.
PdfDocument myPdf = PdfDocument.renderUrlAsPdf("https://en.wikipedia.org/wiki/PDF");
// Save the PdfDocument to a file
try {
myPdf.saveAs("myPDF.pdf");
} catch (IOException e) {
throw new RuntimeException(e);
}
The renderUrlAsPdf
function accepts a URL as an argument and converts it to a PDF document. This PDF document is later saved to a local drive using the saveAs
function.
The following is the output PDF:
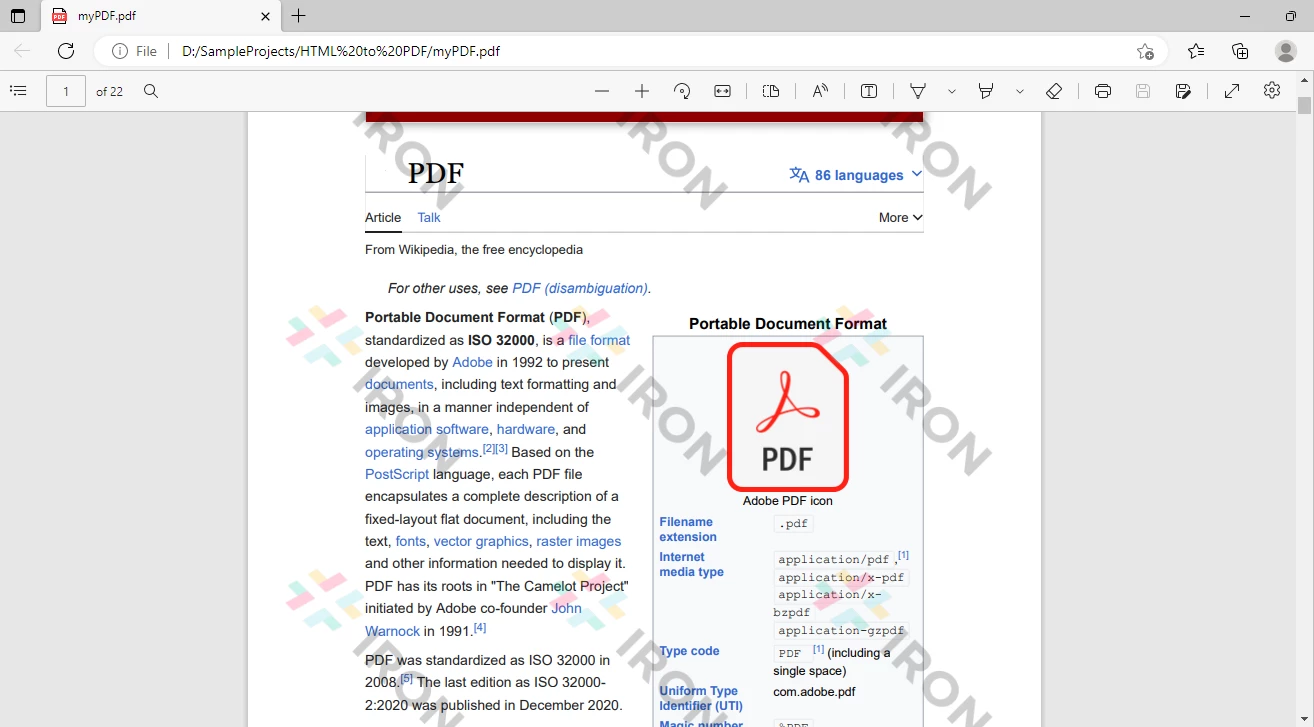
Output PDF
We can also add watermark, header, footer, digital signature, convert XML files/JSP page and many more.
We can also generate password protected PDFs.
Generate Password-protected PDF File
The following sample code demonstrates the example of adding security to our generated PDF file.
PdfDocument myPdf = PdfDocument.fromFile(Paths.get("myPDf.pdf"));
SecurityOptions securityOptions = new SecurityOptions();
securityOptions.setAllowUserEdits(PdfEditSecurity.NO_EDIT);
securityOptions.setAllowUserAnnotations(false);
securityOptions.setAllowUserPrinting(PdfPrintSecurity.NO_PRINT);
securityOptions.setAllowUserFormData(false);
securityOptions.setOwnerPassword("123456");
securityOptions.setUserPassword("123412");
try {
myPdf.saveAs(Paths.get("myNewPDF.pdf"));
} catch (IOException e) {
throw new RuntimeException(e);
}
The above code will make the PDF file read-only, and will not allow any edits or paragraph alignment. We also restrict this document from printing such that this document cannot be printed. We have also set the password. Now, this file is very much secure. In this way, IronPDF allows us to define different file permissions and generate dynamic output.
Summary
In this tutorial, we have learned to generate PDF files. We created a PDF file from an HTML string, an HTML file, and a URL. We used examples ranging from simple to complex. There are many more useful features available such as adding a watermark, footer, header, foreground color, merge and split pages, etc. We cannot cover all of them here. You may visit the official documentation for further exploration.
IronPDF made HTML to PDF conversion a breeze. We converted HTML to PDF with just one line of code. We have also added some security measures to our PDF file. It's faster, more accurate, and safer. Each generated PDF includes the IronPDF watermark. This is due to the fact that we are using a free development version with limited permissions, not the commercial license. We can get rid of it by purchasing a free trial version or a license as needed.