HTML to PDF in Java
This tutorial instructs Java developers on how to use the IronPDF library to convert HTML content into pixel-perfect PDF (portable document format) documents.
IronPDF is a fully-featured PDF converter and PDF processing library. IronPDF is available for both .NET and Java programming languages. This tutorial covers the library's use for converting HTML content (files, markup, etc) in Java applications. The tutorial for converting HTML to PDF in .NET applications is available here.
Overview
How to Convert HTML to PDF in Java
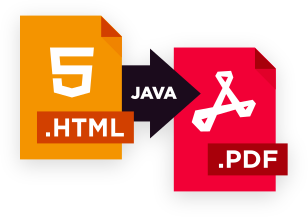
- Install Java library to convert HTML to PDF
- Convert HTML String to PDF document using
renderHtmlAsPdf
method - Generate PDF files from website URL in Java
- Convert HTML files to PDF files with
renderHtmlFileAsPdf
method - Save the generated PDF as a new file
Getting Started
1. Installing the IronPDF PDF Library for Java
Install with Maven
There are two ways to incorporate the IronPDF Library in a Java Project:
- Add IronPDF as a dependency in a Maven-configured Java Project
- Download the IronPDF JAR File and add it to the project classpath manually.
The following section will briefly cover both installation methods.
Option 1: Install IronPDF as a Maven Dependency
To install IronPDF in a Java project using Maven, add the following artifacts to the dependency section of the Java project's pom.xml file.
<dependency>
<groupId>com.ironsoftware</groupId>
<artifactId>com.ironsoftware</artifactId>
<version>2024.9.1</version>
</dependency>
The first artifact references the latest version of the IronPDF library. The second artifact references an SL4J implementation. This dependency is required to enable IronPDF's rendering engine to generate logging messages during execution. Software engineers can substitute this dependency for other logging providers (such as Logback and Log4J); or omit it entirely if they do not need or desire logging.
Run the mvn install
command inside a terminal at the Java project's root directory to download the previously mentioned libraries.
Option 2: Install the IronPDF JAR Manually
Developers who prefer not to use Maven or any other dependency management system will need to download the IronPDF library JAR file (and the optional SL4J implementation) and manually add it to their project's class path.
Download the IronPDF JAR file directly from here (or from the Maven Repository).
How-To Guide and Code Examples
2. Converting HTML to PDF
This section showcases IronPDF's flagship HTML-to-PDF rendering abilities.
The PdfDocument
class is the entry point for all of IronPDF's PDF document rendering and manipulation features. The class includes a set of robust methods for converting HTML to PDF documents in support of the following three use cases: converting from HTML string/markup, converting from an HTML file, and converting from a URL. This section will briefly cover each of these use cases, with links to additional content for gathering additional information.
2.1 Import the IronPDF Package
All of IronPDF conversion and processing components are contained in the com.ironsoftware.ironpdf package.
Include the following import statement at the top of the Java source files (where IronPDF will be used) to make these components accessible to the application's source code.
// Import statement for IronPDF for Java
import com.ironsoftware.ironpdf.*;
2.2. Set the License Key (optional)
IronPDF for Java is free to use. However, for free users, it will brand PDF documents with a tiled background watermark (as shown in the image below).
To use IronPDF to generate PDFs without the watermarks, the library must use a valid license key. The line of code below configures the library with a license key.
// Apply your license key
License.setLicenseKey("YOUR-LICENSE-KEY");
The license key should be set before generating PDF files or customizing file content. We recommend that you call the setLicenseKey
method before all other lines of code.
Purchase a license key from our licensing page, or contact us to obtain a free trial license key.
2.3 Set the Log File Location (optional)
By default (and assuming that there is an SLF4J provider installed), IronPDF generates log messages to a text file called IronPdfEngine.log located in the Java application's root directory.
To specify a different name and location for the log file, use the Settings.setLogPath
method:
// Set a log path
Settings.setLogPath(Paths.get("IronPdfEngine.log"));
Please Note: Settings.setLogPath
must be called before using any PDF conversion and manipulation methods.
2.4. Creating a PDF from HTML String
PdfDocument.renderHtmlAsPdf
converts a string of HTML content into a PDF document.
The following code sample uses a single headline element to generate a new file.
PdfDocument pdf = PdfDocument.renderHtmlAsPdf("<h1>Hello from IronPDF!</h1>");
pdf.saveAs("htmlstring_to_pdf.pdf");
renderHtmlAsPdf
processes all HTML, CSS, and JavaScript content the same way that modern, standards-compliant browsers can. This helps software engineers create PDF documents that look exactly as it appears in a web browser.
The renderHtmlAsPdf
method can source images, stylesheets and scripts located in folders on a computer or on a network drive. The next example produces a PDF document from HTML that references a CSS file and an image located in an assets
folder:
String html = "<html><head><title>Hello world!</title><link rel='stylesheet' href='assets/style.css'></head><body><h1>Hello from IronPDF!</h1><a href='https://ironpdf.com/java/'><img src='assets/logo.png' /></a></body></html>";
PdfDocument pdf = PdfDocument.renderHtmlAsPdf(html);
pdf.saveAs("output.pdf");
The result of the above code is shown in the image below.
A second (optional) argument to renderHtmlAsPdf
allows developers to specify a base path from which to reference web assets. This path can be to a directory on the local filesystem or even a URL path.
Learn more about the renderHtmlAsPdf
method from this code example, or read about it in the API Reference pages.
2.5. Creating a PDF from a URL
Developers can convert online web pages to PDF documents using IronPDF's PdfDocument.renderUrlAsPdf
method.
The next example renders the Wikipedia article into PDF content.
PdfDocument pdf = PdfDocument.renderUrlAsPdf("https://en.wikipedia.org/wiki/PDF");
pdf.saveAs("url_to_pdf.pdf");
The generated PDF file format is shown below.
Learn more about converting web pages into PDF content from the code example.
2.6. Creating a PDF from an HTML File
IronPDF can also render an HTML document stored on a local filesystem directly into its equivalent PDF format.
The next code example uses this invoice as a real-world demonstration of how well IronPDF can convert HTML files.
The HTML markup for the invoice is reproduced here for convenience:
<html>
<head>
<meta charset="utf-8">
<title>Invoice</title>
<link rel="stylesheet" href="style.css">
<link rel="license" href="https://www.opensource.org/licenses/mit-license/">
<script src="script.js"></script>
</head>
<body>
<header>
<h1>Invoice</h1>
<address contenteditable>
<p>Jonathan Neal</p>
<p>101 E. Chapman Ave<br>Orange, CA 92866</p>
<p>(800) 555-1234</p>
</address>
<span><img alt="" src="http://www.jonathantneal.com/examples/invoice/logo.png"><input type="file" accept="image/*"></span>
</header>
<article>
<h1>Recipient</h1>
<address contenteditable>
<p>Some Company<br>c/o Some Guy</p>
</address>
<table class="meta">
<tr>
<th><span contenteditable>Invoice #</span></th>
<td><span contenteditable>101138</span></td>
</tr>
<tr>
<th><span contenteditable>Date</span></th>
<td><span contenteditable>January 1, 2012</span></td>
</tr>
<tr>
<th><span contenteditable>Amount Due</span></th>
<td><span id="prefix" contenteditable>$</span><span>600.00</span></td>
</tr>
</table>
<table class="inventory">
<thead>
<tr>
<th><span contenteditable>Item</span></th>
<th><span contenteditable>Description</span></th>
<th><span contenteditable>Rate</span></th>
<th><span contenteditable>Quantity</span></th>
<th><span contenteditable>Price</span></th>
</tr>
</thead>
<tbody>
<tr>
<td><a class="cut">-</a><span contenteditable>Front End Consultation</span></td>
<td><span contenteditable>Experience Review</span></td>
<td><span data-prefix>$</span><span contenteditable>150.00</span></td>
<td><span contenteditable>4</span></td>
<td><span data-prefix>$</span><span>600.00</span></td>
</tr>
</tbody>
</table>
<a class="add">+</a>
<table class="balance">
<tr>
<th><span contenteditable>Total</span></th>
<td><span data-prefix>$</span><span>600.00</span></td>
</tr>
<tr>
<th><span contenteditable>Amount Paid</span></th>
<td><span data-prefix>$</span><span contenteditable>0.00</span></td>
</tr>
<tr>
<th><span contenteditable>Balance Due</span></th>
<td><span data-prefix>$</span><span>600.00</span></td>
</tr>
</table>
</article>
<aside>
<h1><span contenteditable>Additional Notes</span></h1>
<div contenteditable>
<p>A finance charge of 1.5% will be made on unpaid balances after 30 days.</p>
</div>
</aside>
</body>
</html>
<html>
<head>
<meta charset="utf-8">
<title>Invoice</title>
<link rel="stylesheet" href="style.css">
<link rel="license" href="https://www.opensource.org/licenses/mit-license/">
<script src="script.js"></script>
</head>
<body>
<header>
<h1>Invoice</h1>
<address contenteditable>
<p>Jonathan Neal</p>
<p>101 E. Chapman Ave<br>Orange, CA 92866</p>
<p>(800) 555-1234</p>
</address>
<span><img alt="" src="http://www.jonathantneal.com/examples/invoice/logo.png"><input type="file" accept="image/*"></span>
</header>
<article>
<h1>Recipient</h1>
<address contenteditable>
<p>Some Company<br>c/o Some Guy</p>
</address>
<table class="meta">
<tr>
<th><span contenteditable>Invoice #</span></th>
<td><span contenteditable>101138</span></td>
</tr>
<tr>
<th><span contenteditable>Date</span></th>
<td><span contenteditable>January 1, 2012</span></td>
</tr>
<tr>
<th><span contenteditable>Amount Due</span></th>
<td><span id="prefix" contenteditable>$</span><span>600.00</span></td>
</tr>
</table>
<table class="inventory">
<thead>
<tr>
<th><span contenteditable>Item</span></th>
<th><span contenteditable>Description</span></th>
<th><span contenteditable>Rate</span></th>
<th><span contenteditable>Quantity</span></th>
<th><span contenteditable>Price</span></th>
</tr>
</thead>
<tbody>
<tr>
<td><a class="cut">-</a><span contenteditable>Front End Consultation</span></td>
<td><span contenteditable>Experience Review</span></td>
<td><span data-prefix>$</span><span contenteditable>150.00</span></td>
<td><span contenteditable>4</span></td>
<td><span data-prefix>$</span><span>600.00</span></td>
</tr>
</tbody>
</table>
<a class="add">+</a>
<table class="balance">
<tr>
<th><span contenteditable>Total</span></th>
<td><span data-prefix>$</span><span>600.00</span></td>
</tr>
<tr>
<th><span contenteditable>Amount Paid</span></th>
<td><span data-prefix>$</span><span contenteditable>0.00</span></td>
</tr>
<tr>
<th><span contenteditable>Balance Due</span></th>
<td><span data-prefix>$</span><span>600.00</span></td>
</tr>
</table>
</article>
<aside>
<h1><span contenteditable>Additional Notes</span></h1>
<div contenteditable>
<p>A finance charge of 1.5% will be made on unpaid balances after 30 days.</p>
</div>
</aside>
</body>
</html>
Assume that the HTML file has been saved to a folder called invoices along with its CSS file and JavaScript file. We can use IronPDF to convert the HTML file as follows:
PdfDocument pdf = PdfDocument.renderHtmlFileAsPdf("C:/invoices/TestInvoice1.html");
pdf.saveAs("htmlfile_to_pdf.pdf");
As with the HTML string to PDF conversion examples, IronPDF correctly resolves any relative URLs in an HTML document to their correct paths on the file system. As a result, the PDF File that this example produces is able to perfectly capture the visual influences that any referenced stylesheets and scripts would normally have on a web page.
3. Further Reading
We have only scratched the surface of IronPDF's HTML to PDF rendering abilities.
Further your understanding of how to use the HTML to PDF converter for Java development using the curated code samples featured in our Code Examples section.
- Read this code example to learn how to customize the appearance of a PDF document during the conversion process.
- Generate PDF files with custom headers and footers, margin sizes, page dimensions, watermarks, and much more.
- Extract PDF content (text and images) from documents, optimize file sizes, and print PDFs programmatically
Study the IronPDF Java API Reference page on the PdfDocument
class for even greater control over rendered HTML to PDF.
Watch the HTML to PDF Tutorial Video
Tutorial Quick Access
Download this Tutorial as Java Source Code
The full HTML to PDF Java Source Code for this tutorial is available to download for free as a zipped IntelliJ project.
DownloadExplore this Tutorial on GitHub
The source code for this project is available on GitHub.
Use this code as an easy way to get up and running in just a few minutes. The project is saved as an IntellJ IDEA project, but can be imported into other popular Java IDEs.
Java HTML to PDFView the API Reference
Explore the API Reference for IronPDF, outlining the details of all of IronPDF’s features, namespaces, classes, methods fields and enums.
View the API Reference