HTML to PDF C# Conversion
As the developers of IronPDF, we understand that PDF documents made by IronPDF not only need to look perfect, but also need to look exactly how our customers expect them to. In this C# PDF tutorial will teach you how to build an HTML to PDF converter in your C# applications, projects, and websites. We will build a C# html-to-pdf converter. The output PDF documents from IronPDF are pixel identical to the PDF functionality in a Google Chrome web browser.
Using the IronPDF C# Library we will:
- Create a PDF document from HTML string or HTML file as the 'content' within a C# application.
- Apply editing and PDF generation functionality in C#
- Convert URLs to PDF without losing any format
Overview
How to Convert HTML to PDF in C#
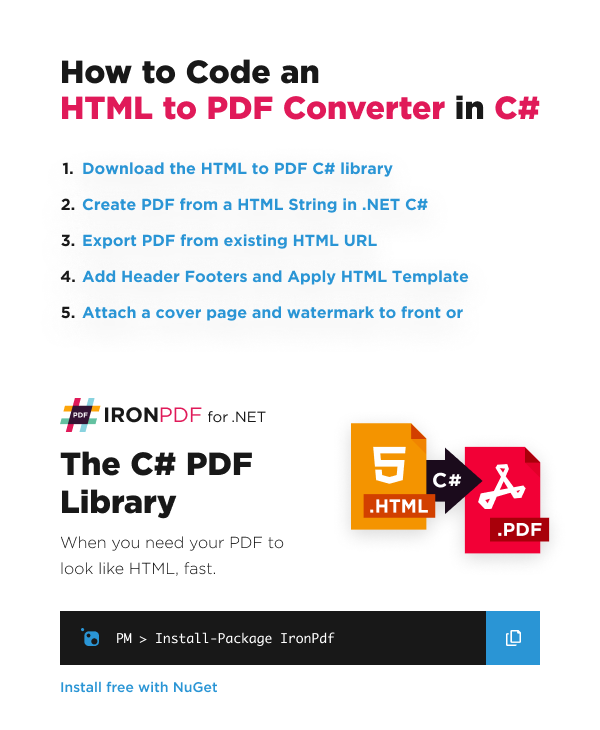
HTML to PDF Converter for C# & VB.NET
Creating PDF files programmatically in .NET can be a frustrating task. The PDF document file format was designed more for printers than for developers. And C# doesn't have many suitable libraries or features for PDF generation built-in, many of the libraries that are on the market do not work out-of-the-box, and cause further frustration when they require multiple lines of code to accomplish a simple task.
The C# HTML to PDF conversion tool we will be using in this tutorial is IronPDF by Iron Software, a highly popular C# PDF generation and editing library. This library has comprehensive PDF editing and generation functionality, works completely out-of-the-box, does exactly what you need it to do in the least amount of lines, and has outstanding documentation of its 50+ features. IronPDF stands out in that it supports .NET 8, .NET 7, .NET 6, and .NET 5, .NET Core, Standard, and Framework on Windows, macOS, Linux, Docker, Azure, and AWS.
With C# and IronPDF, the logic to "generate a PDF document" or "HTML to PDF conversion" is straightforward. Becuase of IronPDF's advanced Chrome Renderer, most or all of the PDF document design and layout will use existing HTML assets.
This method of dynamic PDF generation in .NET with HTML5 works equally well in console applications, windows forms applications, WPF, as well as websites and MVC.
IronPDF also supports debugging of your HTML with Chrome for Pixel Perfect PDFs. A tutorial for setting this up can be found here.
VB.NET : Convert HTML to PDF
IronPDF is a C# Library that allows developers to create PDF documents easily in C#, F#, and VB.NET for .NET Core and .NET Framework. This ensures that we, as .NET coders, do not need to learn proprietary file formats or new APIs. We can easily output dynamic PDF files from our programs and web applications.
To learn about using IronPDF on VB.NET view our guide.
To learn about using IronPDF on F# view our guide.
IronPDF Features:
- Generating PDFs from: HTML, URL, JavaScript, CSS and many image formats
- Adding headers/footers, signatures, attachments, and passwords and security
- Performance optimization: Full Multithreading and Async support
Step 1
Download and Install the HTML to PDF C# Library
Install with NuGet
Install-Package IronPdf
Visual Studio - NuGet Package Manager
In Visual Studio, right click on your project solution explorer and select Manage NuGet Packages...
, From there simply search for IronPDF and install the latest version to your solution... click OK to any dialog boxes that come up. This will also work just as well in VB.NET projects.
Install-Package IronPdf
IronPDF on NuGet Website
For a comprehensive rundown of IronPDF's features, compatibility and downloads, please check out IronPDF on NuGet's official website: https://www.nuget.org/packages/IronPdf
Install via DLL
Another option is to install the IronPDF DLL directly. IronPDF can be downloaded and manually installed to the project or GAC from https://ironpdf.com/packages/IronPdf.zip
How to Tutorials
Create a PDF with an HTML String in C# .NET
How to: Convert HTML String to PDF? It is a very efficient and rewarding skill to create a new PDF file in C#.
We can simply use the ChromePdfRenderer.RenderHtmlAsPdf method to turn any HTML (HTML5) string into a PDF. C# HTML to PDF rendering is undertaken by a fully functional version of the Google Chromium engine, embedded within IronPDF DLL.
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-1.cs
using IronPdf;
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderHtmlAsPdf("<h1> Hello IronPdf </h1>");
pdf.SaveAs("pixel-perfect.pdf");
Imports IronPdf
Private renderer = New ChromePdfRenderer()
Private pdf = renderer.RenderHtmlAsPdf("<h1> Hello IronPdf </h1>")
pdf.SaveAs("pixel-perfect.pdf")
RenderHtmlAsPdf
fully supports HTML5, CSS3, JavaScript, and Images. If these assets are on a hard disk, we may wish to set the second parameter of RenderHtmlAsPdf
to the directory of the assets.
IronPDF will render your HTML exactly as it appears in Chrome
We have a full tutorial dedicated to allowing you to set up Chrome for full HTML debugging to make sure the changes you see there when editing your HTML, CSS, and JavaScript are pixel-perfect the same as the output PDF from IronPDF when you choose to render. Please find the tutorial here: How to Debug HTML in Chrome to Create Pixel Perfect PDFs.
BaseUrlPath:
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-2.cs
using IronPdf;
// this will render C:\MyProject\Assets\image1.png
var pdf = renderer.RenderHtmlAsPdf("<img src='image1.png'/>", @"C:\MyProject\Assets\");
Imports IronPdf
' this will render C:\MyProject\Assets\image1.png
Private pdf = renderer.RenderHtmlAsPdf("<img src='image1.png'/>", "C:\MyProject\Assets\")
All referenced CSS stylesheets, images and JavaScript files will be relative to the BaseUrlPath
and can be kept in a neat and logical structure. You may also, of course opt to reference images, stylesheets and assets online, including web-fonts such as Google Fonts and even jQuery.
Export a PDF Using Existing URL
(URL to PDF)
Rendering existing URLs as PDFs with C# is very efficient and intuitive. This also allows teams to split PDF design and back-end PDF rendering work across multiple teams.
Lets render a page from Wikipedia.com in the following example:
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-3.cs
using IronPdf;
// Create a PDF from any existing web page
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderUrlAsPdf("https://en.wikipedia.org/wiki/PDF");
pdf.SaveAs("wikipedia.pdf");
Imports IronPdf
' Create a PDF from any existing web page
Private renderer = New ChromePdfRenderer()
Private pdf = renderer.RenderUrlAsPdf("https://en.wikipedia.org/wiki/PDF")
pdf.SaveAs("wikipedia.pdf")
You will notice that hyperlinks and even HTML forms are preserved within the PDF generated by our C# code.
When rendering existing web pages we have some tricks we may wish to apply:
Print and Screen CSS
In modern CSS3 we have css directives for both print and screen. We can instruct IronPDF to render "Print" CSSs which are often simplified or overlooked. By default "Screen" CSS styles will be rendered, which IronPDF users have found most intuitive.
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-4.cs
using IronPdf;
using IronPdf.Rendering;
renderer.RenderingOptions.CssMediaType = PdfCssMediaType.Screen;
// or
renderer.RenderingOptions.CssMediaType = PdfCssMediaType.Print;
Imports IronPdf
Imports IronPdf.Rendering
renderer.RenderingOptions.CssMediaType = PdfCssMediaType.Screen
' or
renderer.RenderingOptions.CssMediaType = PdfCssMediaType.Print
Main Page: A full comparison with images of Screen and Print can be found here.
JavaScript
IronPDF supports JavaScript, jQuery and even AJAX. We may need to instruct IronPDF to wait for JS or ajax to finish running before rendering a snapshot of our web-page.
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-5.cs
renderer.RenderingOptions.EnableJavaScript = true;
renderer.RenderingOptions.WaitFor.RenderDelay(500); // milliseconds
IRON VB CONVERTER ERROR developers@ironsoftware.com
We can demonstrate compliance with the JavaScript standard by rendering an advanced d3.js JavaScript chord chart from a CSV dataset like this:
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-6.cs
using IronPdf;
// Create a PDF Chart a live rendered dataset using d3.js and javascript
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderUrlAsPdf("https://bl.ocks.org/mbostock/4062006");
pdf.SaveAs("chart.pdf");
Imports IronPdf
' Create a PDF Chart a live rendered dataset using d3.js and javascript
Private renderer = New ChromePdfRenderer()
Private pdf = renderer.RenderUrlAsPdf("https://bl.ocks.org/mbostock/4062006")
pdf.SaveAs("chart.pdf")
Responsive CSS
HTML to PDF using response CSS in .NET! Responsive web pages are designed to be viewed in a browser. IronPDF does not open a real browser window within your server's OS. This can lead to responsive elements rendering at their smallest size.
We recommend using Print css media types to navigate this issue. Print CSS should not normally be responsive.
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-7.cs
renderer.RenderingOptions.CssMediaType = IronPdf.Rendering.PdfCssMediaType.Print;
renderer.RenderingOptions.CssMediaType = IronPdf.Rendering.PdfCssMediaType.Print
Generate a PDF from a HTML Page
We can also render any HTML page to PDF on our hard disk. All relative assets such as CSS, images and js will be rendered as if the file had been opened using the file:// protocol.
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-8.cs
using IronPdf;
// Create a PDF from an existing HTML using C#
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderHtmlFileAsPdf("Assets/TestInvoice1.html");
pdf.SaveAs("Invoice.pdf");
Imports IronPdf
' Create a PDF from an existing HTML using C#
Private renderer = New ChromePdfRenderer()
Private pdf = renderer.RenderHtmlFileAsPdf("Assets/TestInvoice1.html")
pdf.SaveAs("Invoice.pdf")
This method has the advantage of allowing the developer the opportunity to test the HTML content in a browser during development. We recommend Chrome as it is the web browser on which IronPDF's rendering engine is based.
To convert XML to PDF you can use XSLT templating to print your XML content to PDF.
Add Custom Headers and Footers
Headers and footers can be added to PDFs when they are rendered, or to existing PDF files using IronPDF.
With IronPDF, Headers and footers can contain simple text based content using the TextHeaderFooter
class - or with images and rich HTML content using the HtmlHeaderFooter
class.
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-9.cs
using IronPdf;
// Create a PDF from an existing HTML
var renderer = new ChromePdfRenderer
{
RenderingOptions =
{
MarginTop = 50, //millimeters
MarginBottom = 50,
CssMediaType = IronPdf.Rendering.PdfCssMediaType.Print,
TextHeader = new TextHeaderFooter
{
CenterText = "{pdf-title}",
DrawDividerLine = true,
FontSize = 16
},
TextFooter = new TextHeaderFooter
{
LeftText = "{date} {time}",
RightText = "Page {page} of {total-pages}",
DrawDividerLine = true,
FontSize = 14
}
}
};
var pdf = renderer.RenderHtmlFileAsPdf("assets/TestInvoice1.html");
pdf.SaveAs("Invoice.pdf");
// This neat trick opens our PDF file so we can see the result
System.Diagnostics.Process.Start("Invoice.pdf");
Imports IronPdf
' Create a PDF from an existing HTML
Private renderer = New ChromePdfRenderer With {
.RenderingOptions = {
MarginTop = 50, MarginBottom = 50, CssMediaType = IronPdf.Rendering.PdfCssMediaType.Print, TextHeader = New TextHeaderFooter With {
.CenterText = "{pdf-title}",
.DrawDividerLine = True,
.FontSize = 16
},
TextFooter = New TextHeaderFooter With {
.LeftText = "{date} {time}",
.RightText = "Page {page} of {total-pages}",
.DrawDividerLine = True,
.FontSize = 14
}
}
}
Private pdf = renderer.RenderHtmlFileAsPdf("assets/TestInvoice1.html")
pdf.SaveAs("Invoice.pdf")
' This neat trick opens our PDF file so we can see the result
System.Diagnostics.Process.Start("Invoice.pdf")
HTML Headers and Footers
The HtmlHeaderFooter
class allows for rich headers and footers to be generated using HTML5 content which may even include images, stylesheets and hyperlinks.
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-10.cs
using IronPdf;
renderer.RenderingOptions.HtmlFooter = new HtmlHeaderFooter
{
HtmlFragment = "<div style='text-align:right'><em style='color:pink'>page {page} of {total-pages}</em></div>"
};
Imports IronPdf
renderer.RenderingOptions.HtmlFooter = New HtmlHeaderFooter With {.HtmlFragment = "<div style='text-align:right'><em style='color:pink'>page {page} of {total-pages}</em></div>"}
Dynamic Data in PDF Headers and Footers
We may "mail-merge" content into the text and even HTML of headers and footers using placeholders such as:
- {page} for the current page number
- {total-pages} for the total number of pages in the PDF
- {url} for the URL of the rendered PDF if rendered from a web page
- {date} for today's date
- {time} for the current time
- {html-title} for the title attribute of the rendered HTML document
- {pdf-title} for the document title, which may be set via ChromePdfRenderOptions
C# HTML to PDF Conversion Settings
There are many nuances to how our users and clients may expect PDF content to be rendered.
The ChromePdfRenderer
class contains a RenderingOptions property which can be used to set these options.
For example we may wish to choose to only accept "print
" style CSS3 directives:
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-11.cs
using IronPdf;
renderer.RenderingOptions.CssMediaType = IronPdf.Rendering.PdfCssMediaType.Print;
Imports IronPdf
renderer.RenderingOptions.CssMediaType = IronPdf.Rendering.PdfCssMediaType.Print
We may also wish to change the size of our print margins to create more whitespace on the page, to make room for large headers or footers, or even set zero margins for commercial printing of brochures or posters:
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-12.cs
using IronPdf;
renderer.RenderingOptions.MarginTop = 50; // millimeters
renderer.RenderingOptions.MarginBottom = 50; // millimeters
Imports IronPdf
renderer.RenderingOptions.MarginTop = 50 ' millimeters
renderer.RenderingOptions.MarginBottom = 50 ' millimeters
We may wish to turn on or off background images from HTML elements:
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-13.cs
using IronPdf;
renderer.RenderingOptions.PrintHtmlBackgrounds = true;
Imports IronPdf
renderer.RenderingOptions.PrintHtmlBackgrounds = True
It is also possible to set our output PDFs to be rendered on any virtual paper size - including portrait and landscape sizes and even custom sizes which may be set in millimeters or inches.
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-14.cs
using IronPdf;
using IronPdf.Rendering;
renderer.RenderingOptions.PaperSize = PdfPaperSize.A4;
renderer.RenderingOptions.PaperOrientation = PdfPaperOrientation.Landscape;
Imports IronPdf
Imports IronPdf.Rendering
renderer.RenderingOptions.PaperSize = PdfPaperSize.A4
renderer.RenderingOptions.PaperOrientation = PdfPaperOrientation.Landscape
Full documentation of the HTML C# PDF Creator Settings may be found at https://ironpdf.com/object-reference/api/IronPdf.ChromePdfRenderer.html
The full set of PDF Print Options includes:
- CreatePdfFormsFromHtml: Turns all HTML forms elements into editable PDF forms.
- CssMediaType:
Screen
orPrint
CSS Styles and StyleSheets. See our full in-depth tutorial with comparison images. - CustomCssUrl: Allows a custom CSS style-sheet to be applied to HTML before rendering. May be a local file path, or a remote URL.
- EnableMathematicalLaTex: Enables or disables the rendering of mathematical LaTeX elements.
- EnableJavaScript: Enables JavaScript and JSON to be executed before the page is rendered. Ideal for printing from Ajax / Angular Applications. Also see RenderDelay.
- Javascript: Specifies a custom JavaScript string to be executed after all HTML has loaded but before PDF rendering.
- JavascriptMessageListener: A method callback to be invoked whenever a browser JavaScript console message becomes available.
- FirstPageNumber: First page number to be used in PDF headers and footers.
- TableOfContents: Generates a table of contents at the location in the HTML document where an element is found with id "ironpdf-toc".
- TextHeader: Sets the header content for every PDF page as a String. Supports 'mail-merge'
- TextFooter: Sets the footer content for every PDF page as a String. Supports 'mail-merge'
- HtmlHeader: Sets the header content for every PDF page as HTML
- HtmlFooter: Sets the footer content for every PDF page as HTML
- MarginBottom: Paper margin in millimeters. Set to zero for border-less and commercial printing applications
- MarginLeft: Paper margin in millimeters
- MarginRight: Paper margin in millimeters
- MarginTop: Paper margin in millimeters. Set to zero for border-less and commercial printing applications
- UseMarginsOnHeaderAndFooter: Specifies whether to use margin values from the main document when rendering headers and footers.
- PaperFit: A manager for setting up virtual paper layouts, controlling how content will be laid out on PDF "paper" pages. Includes options for Default Chrome Behavior, Zoomed, Responsive CSS3 Layouts, Scale-To-Page & Continuous Feed style PDF page setups.
- PaperOrientation: Paper orientation for new document. Full explanation and accompanying code example.
- PageRotation: Page rotation from existing document. Full explanation and accompanying code example.
- PaperSize: Set an output paper size for PDF pages.
System.Drawing.Printing.PaperKind
. - SetCustomPaperSizeinCentimeters: Sets the paper size in centimeters.
- SetCustomPaperSizeInInches: Sets the paper size in inches.
- SetCustomPaperSizeinMilimeters: Sets the paper size in millimeters.
- SetCustomPaperSizeinPixelsOrPoints: Sets the paper size in screen pixels or printer points.
- ForcePaperSize: Specifies whether to force page sizes to be exactly what is specified via PaperSize by resizing the page after generating a PDF from HTML.
- PrintHtmlBackgrounds: Prints background-colors and images from HTML.
- GrayScale: Specifies whether to output a black-and-white PDF.
- Timeout: Sets the render timeout in seconds.
- WaitFor: A wrapper object that holds configuration for wait-for mechanism. This can be useful when considering the rendering of JavaScript, Ajax or animations.
- PageLoad: Default render with no waiting.
- RenderDelay: Setting an arbitrary waiting time.
- Fonts: Waits until all the fonts have loaded.
- JavaScript: Triggering the render with a JavaScript function.
- HTML elements: Waits for specific HTML elements, such as element IDs, names, tag names, and query selectors to target elements.
- NetworkIdle: Waiting for network idle (0, 2, or a custom amount).
- Title: PDF Document Name and Title meta-data. Not required.
- InputEncoding: Specifies the input character encoding.
- RequestContext: Specifies the request context for the render.
Apply HTML Templating
To template or "batch create" PDFs is a common requirement for Internet and website developers.
Rather than templating a PDF document itself, with IronPDF we can template our HTML using existing, well tried technologies. When the HTML template is combined with data from a query-string or database we end up with a dynamically generated PDF document.
In the simplest instance, using the C# String.Format method is effective for basic sample.
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-15.cs
using System;
String.Format("<h1>Hello {0} !</h1>", "World");
Imports System
String.Format("<h1>Hello {0} !</h1>", "World")
If the HTML file is longer, often we can use arbitrary placeholders such as [[NAME]]
and replace them with real data later.
The following example will create 3 PDFs, each personalized to a user.
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-16.cs
var htmlTemplate = "<p>[[NAME]]</p>";
var names = new[] { "John", "James", "Jenny" };
foreach (var name in names)
{
var htmlInstance = htmlTemplate.Replace("[[NAME]]", name);
var pdf = renderer.RenderHtmlAsPdf(htmlInstance);
pdf.SaveAs(name + ".pdf");
}
Dim htmlTemplate = "<p>[[NAME]]</p>"
Dim names = { "John", "James", "Jenny" }
For Each name In names
Dim htmlInstance = htmlTemplate.Replace("[[NAME]]", name)
Dim pdf = renderer.RenderHtmlAsPdf(htmlInstance)
pdf.SaveAs(name & ".pdf")
Next name
Advanced Templating With Handlebars.NET
A sophisticated method to merge C# data with HTML for PDF generation is using the Handlebars Templating standard.
Handlebars makes it possible to create dynamic HTML from C# objects and class instances including database records. Handlebars is particularly effective where a query may return an unknown number of rows such as in the generation of an invoice.
We must first add an additional NuGet Package to our project: https://www.nuget.org/packages/Handlebars.NET/
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-17.cs
var source =
@"<div class=""entry"">
<h1>{{title}}</h1>
<div class=""body"">
{{body}}
</div>
</div>";
var template = Handlebars.Compile(source);
var data = (title: "My new post", body: "This is my first post!");
var result = template(data);
/* Would render:
<div class="entry">
<h1>My New Post</h1>
<div class="body">
This is my first post!
</div>
</div>
*/
Dim source = "<div class=""entry"">
<h1>{{title}}</h1>
<div class=""body"">
{{body}}
</div>
</div>"
Dim template = Handlebars.Compile(source)
Dim data = (title:= "My new post", body:= "This is my first post!")
Dim result = template(data)
' Would render:
'<div class="entry">
' <h1>My New Post</h1>
' <div class="body">
' This is my first post!
' </div>
'</div>
'
To render this HTML we can simply use the RenderHtmlAsPdf
method.
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-18.cs
using IronPdf;
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderHtmlAsPdf(htmlInstance);
pdf.SaveAs("Handlebars.pdf");
Imports IronPdf
Private renderer = New ChromePdfRenderer()
Private pdf = renderer.RenderHtmlAsPdf(htmlInstance)
pdf.SaveAs("Handlebars.pdf")
You can learn more about the handlebars html templating standard and its C# using from https://github.com/rexm/Handlebars.NET
Add Page Breaks using HTML5
A common requirement in a PDF document is for pagination. Developers need to control where PDF pages start and end for a clean, readable layout.
The easiest way to do this is with a less known CSS trick which will render a page break into any printed HTML document.
<div style='page-break-after: always;'> </div>
<div style='page-break-after: always;'> </div>
The provided HTML works, but is hardly best practice. We advise to adjust the media attribute like the following example. Such a neat and tidy way to lay out multipage HTML content.
<!DOCTYPE html>
<html>
<head>
<style type="text/css" media="print">
.page{
page-break-after: always;
page-break-inside: avoid;
}
</style>
</head>
<body>
<div class="page">
<h1>This is Page 1</h1>
</div>
<div class="page">
<h1>This is Page 2</h1>
</div>
<div class="page">
<h1>This is Page 3</h1>
</div>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<style type="text/css" media="print">
.page{
page-break-after: always;
page-break-inside: avoid;
}
</style>
</head>
<body>
<div class="page">
<h1>This is Page 1</h1>
</div>
<div class="page">
<h1>This is Page 2</h1>
</div>
<div class="page">
<h1>This is Page 3</h1>
</div>
</body>
</html>
The How-To outline more tips and tricks with Page Breaks
Attach a Cover Page to a PDF
IronPDF makes it easy to Merge PDF documents. The most common usage of this technique is to add a cover page or back page to an existing rendered PDF document.
To do so, we first render a cover page, and then use the PdfDocument.Merge()
static method to combine the 2 documents.
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-19.cs
using IronPdf;
var pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf/");
var pdfMerged = PdfDocument.Merge(new PdfDocument("CoverPage.pdf"), pdf).SaveAs("Combined.Pdf");
Imports IronPdf
Private pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf/")
Private pdfMerged = PdfDocument.Merge(New PdfDocument("CoverPage.pdf"), pdf).SaveAs("Combined.Pdf")
A full code example can be found here: PDF Cover Page Code Example
Add a Watermark
A final C# PDF feature that IronPDF supports is to add a watermark to documents. This can be used to add a notice to each page that a document is "confidential" or a "sample".
:path=/static-assets/pdf/content-code-examples/tutorials/html-to-pdf-20.cs
using IronPdf;
using IronPdf.Editing;
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf");
// Watermarks all pages with red "SAMPLE" text at a custom location.
// Also adding a link to the watermark on-click
pdf.ApplyWatermark("<h2 style='color:red'>SAMPLE</h2>", 0, VerticalAlignment.Middle, HorizontalAlignment.Center);
pdf.SaveAs(@"C:\Path\To\Watermarked.pdf");
Imports IronPdf
Imports IronPdf.Editing
Private renderer = New ChromePdfRenderer()
Private pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf")
' Watermarks all pages with red "SAMPLE" text at a custom location.
' Also adding a link to the watermark on-click
pdf.ApplyWatermark("<h2 style='color:red'>SAMPLE</h2>", 0, VerticalAlignment.Middle, HorizontalAlignment.Center)
pdf.SaveAs("C:\Path\To\Watermarked.pdf")
A full code example can be found here: PDF Watermarking Code Example
Download C# Source Code
The full free HTML to PDF converter C# Source Code for this tutorial is available to download as a zipped Visual Studio 2022 project file. It will use its rendering engine to generate PDF document objects in C#.
Download this tutorial as a Visual Studio project
The free download contains everything you need to create a PDF from HTML - including working C# PDF code examples code for:
- Convert an HTML String to PDF using C#
- HTML File to PDF in C# (supporting CSS, JavaScript and images)
- C# HTML to PDF using a URL ("URL to PDF")
- C# PDF editing and settings examples
- Rendering JavaScript canvas charts such as d3.js to a PDF
- The PDF Library for C#
Class Reference
Developers may also be interested in the IronPdf.PdfDocument
Class reference:
https://ironpdf.com/object-reference/api/IronPdf.PdfDocument.html
This object model shows how PDF documents may be:
- Encrypted and password protected
- Edited or 'stamped' with new HTML content
- Enhanced with foreground and background images
- Merged, joined, truncated and spliced at a page or document level
- OCR processed to extract plain text and images
Blazor HTML to PDF
Adding HTML to PDF functionality to your Blazor server is easy, simply:
- Create a new Blazor server project or use an existing one
- Add the IronPDF library to your project using NuGet
- Add a new Razor Component or use an existing one
- Add a
InputTextArea
and link it to IronPDF - Let IronPDF take care of the rest and deploy
The full step-by-step guide with pictures and code examples can be found here.
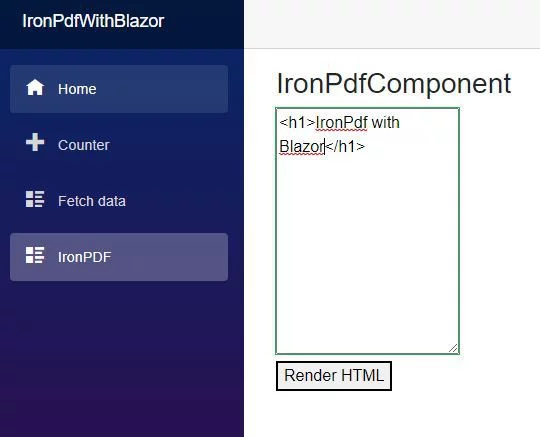
Compare with Other PDF Libraries
PDFSharp
PDFSharp is a free open source library which allows logical editing and creation of PDF documents in .NET.
A key difference between PDFSharp and IronPDF is that IronPDF has an embedded Web Browser which allows faithful creation of PDFs from HTML, CSS, JS and images.
The IronPDF API also differs from PDFSharp in that it is based around use cases rather than the technical structure of PDF documents. Many find this more logical and intuitive to use.
It can convert HTML to PDF, but HTML to PDF conversion is limited: including .html files to PDF files.
wkhtmltopdf
wkhtmltopdf is a free, open source library written in C++ which allows PDF documents to be rendered from HTML.
A key difference between wkhtmltopdf and IronPDF is that IronPDF is written in C# and is stable and thread safe for use in .NET applications and Websites.
IronPDF also fully supports CSS3 and HTML5, where as wkhtmltopdf is almost a decade out of date.
The IronPDF API also differs from wkhtmltopdf in that it has a large and advanced API allowing PDF documents to be edited, manipulated, compressed, imported, exported, signed, secured and watermarked.
HTML to PDF conversion with wkhtmltopdf is stable but utilizes a very outdated rendering engine.
iTextSharp
iTextSharp is an open source partial port of the iText java library for PDF generation and editing. Convert HTML to PDF- that is possible, but I notice its rendering was limited to what is available in Java or uses HTML to PDF conversion from wkhtmltopdf under the LGPL open sourced license.
A key difference with HTML to PDF between C# iTextSharp and IronPDF is that IronPDF has more advanced and accurate HTML-To-PDF rendering by using an embedded Chrome based web browser rather than the legacy wkhtmltopdf used in iText.
The IronPDF API also differs from iTextSharp in that IronPDF has explicit licenses for commercial or private usage, whereas iTextSharp's AGLP license is only suitable for applications where the full source code is presented for free to every user - even users across the internet.
A full breakdown of the differences is available in our iTextSharp C# documentation page.
Other Commercial Libraries
Aspose PDF, Spire PDF, EO PDF, and SelectPdf are competitor .NET commercial PDF libraries by other vendors. IronPDF has a comparatively strong feature set, excellent compatibility, well-written documentation, and a fair price point. You can see the comparison between IronPDF, competitors, and Chrome itself here.
Watch HTML to PDF Tutorial Video
Tutorial Quick Access
Download this Tutorial as C# Source Code
The full free HTML to PDF C# Source Code for this tutorial is available to download as a zipped Visual Studio project file.
DownloadExplore this Tutorial on GitHub
The source code for this project is available in C# and VB.NET on GitHub.
Use this code as an easy way to get up and running in just a few minutes. The project is saved as a Microsoft Visual Studio 2017 project, but is compatible with any .NET IDE.
C# HTML to PDF VB.NET HTML to PDFDownload the C# PDF Quickstart guide
To make developing PDFs in your .NET applications easier, we have compiled a quick-start guide as a PDF document. This "Cheat-Sheet" provide quick access to common functions and examples for generating and editing PDFs in C# and VB.NET - and may help save time in getting started using IronPDF in your .NET project.
DownloadView the API Reference
Explore the API Reference for IronPDF, outlining the details of all of IronPDF’s features, namespaces, classes, methods fields and enums.
View the API Reference