How to Add Table of Contents
A table of contents (TOC) is like a roadmap that helps readers navigate through the PDF document's contents. It typically appears at the beginning and lists the main sections or chapters of the PDF, along with the page numbers where each section begins. This allows readers to quickly find and jump to specific parts of the document, making it easier to access the information they need.
IronPDF provides a feature to create a table of contents with hyperlinks to the 'h1', 'h2', 'h3', 'h4', 'h5', and 'h6' elements. The default styling of this table of contents will not conflict with other styles in the HTML content.
How to Add a Table of Contents
- Download the C# library for adding a table of contents
- Prepare the HTML to be converted to PDF
- Set the TableOfContents property to enable the table of contents
- Customize the table of contents by choosing whether to display page numbers or not
- Optimize the placement of the table of contents on the output PDF
Install with NuGet
Install-Package IronPdf
Add Table of Contents Example
Use the TableOfContents property to enable the creation of a table of contents in the output PDF document. This property can be assigned to one of three TableOfContentsTypes, which are described as follows:
- None: Do not create a table of contents
- Basic: Create a table of contents without page numbers
- WithPageNumbers: Create a table of contents WITH page numbers
To understand this feature better, you can download the sample HTML file below:
Code
:path=/static-assets/pdf/content-code-examples/how-to/table-of-contents.cs
using IronPdf;
using System.IO;
// Instantiate Renderer
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Configure render options
renderer.RenderingOptions = new ChromePdfRenderOptions
{
// Enable table of content feature
TableOfContents = TableOfContentsTypes.WithPageNumbers,
};
PdfDocument pdf = renderer.RenderHtmlFileAsPdf("tableOfContent.html");
pdf.SaveAs("tableOfContents.pdf");
Imports IronPdf
Imports System.IO
' Instantiate Renderer
Private renderer As New ChromePdfRenderer()
' Configure render options
renderer.RenderingOptions = New ChromePdfRenderOptions With {.TableOfContents = TableOfContentsTypes.WithPageNumbers}
Dim pdf As PdfDocument = renderer.RenderHtmlFileAsPdf("tableOfContent.html")
pdf.SaveAs("tableOfContents.pdf")
Output PDF
The table of contents will be created with hyperlinks to each of the 'h1', 'h2', 'h3', 'h4', 'h5', and 'h6'.
Table of Contents Placement on the PDF
- Ensure an HTML document has proper header tags (h1 up to h6).
- Optionally insert a div for where you want the Table of Contents to appear. If the below div is not provided, IronPDF will insert the Table of Contents at the start.
<div id="ironpdf-toc"></div>
<div id="ironpdf-toc"></div>
HTML - In the render options choose to render table of contents either with or without page numbers.
Styling the Table of Contents
The Table of Contents can be styled using CSS, by targeting the various CSS selectors that define the style of the Table of Contents.
In addition, styling modification is done using the CustomCssUrl property. Let's begin by downloading a CSS file that contains the original styling for the table of contents below.
Before proceeding
:path=/static-assets/pdf/content-code-examples/how-to/table-of-contents-overwrite-styling.cs
using IronPdf;
using System.IO;
// Instantiate Renderer
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Configure render options
renderer.RenderingOptions = new ChromePdfRenderOptions
{
// Enable table of content feature
TableOfContents = TableOfContentsTypes.WithPageNumbers,
CustomCssUrl = "./custom.css"
};
// Read HTML text from file
string html = File.ReadAllText("tableOfContent.html");
PdfDocument pdf = renderer.RenderHtmlAsPdf(html);
pdf.SaveAs("tableOfContents.pdf");
Imports IronPdf
Imports System.IO
' Instantiate Renderer
Private renderer As New ChromePdfRenderer()
' Configure render options
renderer.RenderingOptions = New ChromePdfRenderOptions With {
.TableOfContents = TableOfContentsTypes.WithPageNumbers,
.CustomCssUrl = "./custom.css"
}
' Read HTML text from file
Dim html As String = File.ReadAllText("tableOfContent.html")
Dim pdf As PdfDocument = renderer.RenderHtmlAsPdf(html)
pdf.SaveAs("tableOfContents.pdf")
Font Family
With both the '#ironpdf-toc li .title' and '#ironpdf-toc li .page' selectors, it is possible to overwrite the font family of the table of contents. Let's use the 'cursive' font for the title and utilize the @font-face attribute to use the custom 'Lemon' font designed by Eduardo Tunni.
#ironpdf-toc li .title {
order: 1;
font-family: cursive;
}
@font-face {
font-family: 'lemon';
src: url('Lemon-Regular.ttf')
}
#ironpdf-toc li .page {
order: 3;
font-family: 'lemon', sans-serif;
}
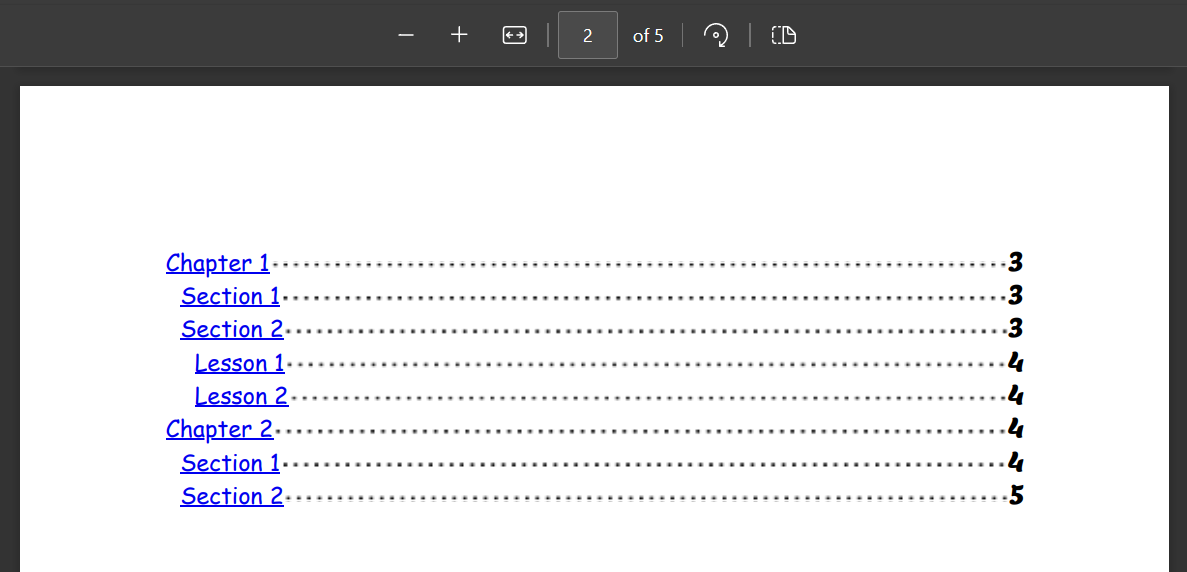
Indentation
Indentation can be controlled using the ':root' selector. This value determines the amount of indent for each header level (h1, h2, ...) in the table of contents. It can be increased as needed, or there can be no indentation with a value of 0.
:root {
--indent-length: 25px;
}
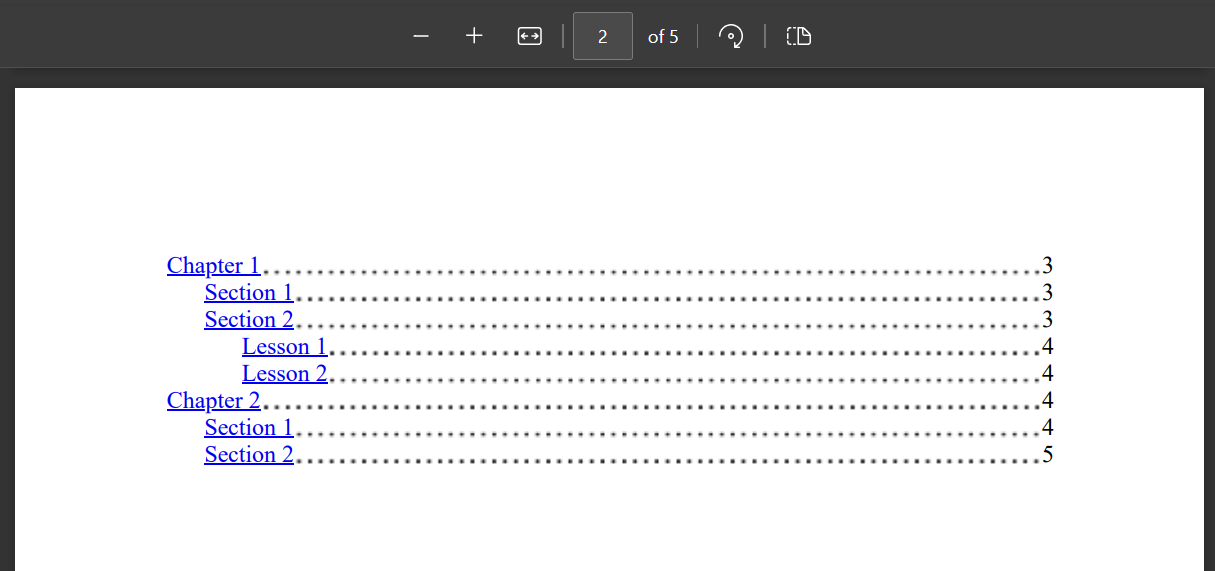
Dot Line
Remove the dotted lines between header title and page number. In the original styling the second parameter is "currentcolor 1px". Change it to "transparent 1px" to remove the dots. It is important to specify other attributes as well because, in this selector, the new styling will completely override the old styling rather than just adding to it.
#ironpdf-toc li::after {
background-image: radial-gradient(circle, transparent 1px, transparent 1.5px);
background-position: bottom;
background-size: 1ex 4.5px;
background-repeat: space no-repeat;
content: "";
flex-grow: 1;
height: 1em;
order: 2;
}
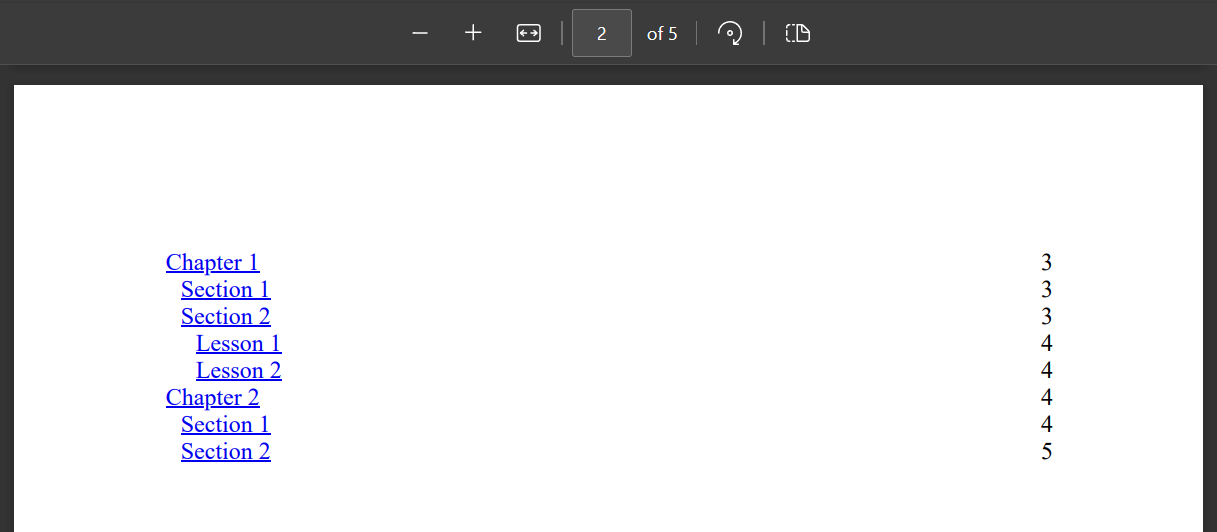