How to use JavaScript with HTML to PDF
JavaScript is a high-level, versatile programming language commonly used in web development. It allows developers to add interactive and dynamic features to websites. jQuery, on the other hand, is not a separate programming language but rather a JavaScript library. It is designed to simplify common tasks in JavaScript, such as DOM manipulation, event handling, and AJAX requests.
IronPDF supports JavaScript efficiently via the Chromium rendering engine. In this article, we'll demonstrate how to use JavaScript and jQuery in HTML to PDF conversion in .NET C# projects. IronPDF is free for development.
How to Use JavaScript with HTML to PDF
Install with NuGet
Install-Package IronPdf
Render JavaScript Example
JavaScript functionalities in HTML are supported when converting from HTML to PDF. As rendering happens without delay, JavaScript might not be executed properly. In most cases, it is advised to configure the Waitfor class in the RenderingOptions to allow JavaScript time to execute. In the following code, I have used WaitFor.JavaScript
and specified a maximum wait time of 500 ms.
:path=/static-assets/pdf/content-code-examples/how-to/javascript-to-pdf-render-javascript.cs
using IronPdf;
string htmlWithJavaScript = @"<h1>This is HTML</h1>
<script>
document.write('<h1>This is JavaScript</h1>');
window.ironpdf.notifyRender();
</script>";
// Instantiate Renderer
var renderer = new ChromePdfRenderer();
// Enable JavaScript
renderer.RenderingOptions.EnableJavaScript = true;
// Set waitFor for JavaScript
renderer.RenderingOptions.WaitFor.JavaScript(500);
// Render HTML contains JavaScript
var pdfJavaScript = renderer.RenderHtmlAsPdf(htmlWithJavaScript);
// Export PDF
pdfJavaScript.SaveAs("javascriptHtml.pdf");
Imports IronPdf
Private htmlWithJavaScript As String = "<h1>This is HTML</h1>
<script>
document.write('<h1>This is JavaScript</h1>');
window.ironpdf.notifyRender();
</script>"
' Instantiate Renderer
Private renderer = New ChromePdfRenderer()
' Enable JavaScript
renderer.RenderingOptions.EnableJavaScript = True
' Set waitFor for JavaScript
renderer.RenderingOptions.WaitFor.JavaScript(500)
' Render HTML contains JavaScript
Dim pdfJavaScript = renderer.RenderHtmlAsPdf(htmlWithJavaScript)
' Export PDF
pdfJavaScript.SaveAs("javascriptHtml.pdf")
Some very complex JavaScript frameworks might not always work 100% with IronPDF and .NET. This is mostly due to limited allocation of memory for JavaScript execution.
Execute Custom JavaScript Example
The JavaScript property in rendering options is used to execute custom JavaScript before rendering HTML to PDF. This is very useful when rendering from a URL where it is not possible to inject JavaScript code. Simply assign a JavaScript code to the JavaScript property of the rendering options.
:path=/static-assets/pdf/content-code-examples/how-to/javascript-to-pdf-execute-javascript.cs
using IronPdf;
ChromePdfRenderer renderer = new ChromePdfRenderer();
// JavaScript code
renderer.RenderingOptions.Javascript = @"
document.querySelectorAll('h1').forEach(function(el){
el.style.color='red';
})";
// Render HTML to PDF
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Happy New Year!</h1>");
pdf.SaveAs("executed_js.pdf");
Imports IronPdf
Private renderer As New ChromePdfRenderer()
' JavaScript code
renderer.RenderingOptions.Javascript = "
document.querySelectorAll('h1').forEach(function(el){
el.style.color='red';
})"
' Render HTML to PDF
Dim pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Happy New Year!</h1>")
pdf.SaveAs("executed_js.pdf")
JavaScript Message Listener Example
IronPDF also allows you to listen to the JavaScript messages that have been logged to the console. Both error logs and custom log messages will be captured by the library. Utilize the JavascriptMessageListener property to it. The code example below demonstrates both the logging of custom text and errors.
:path=/static-assets/pdf/content-code-examples/how-to/javascript-to-pdf-message-listener.cs
using IronPdf;
using System;
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Method callback to be invoked whenever a browser console message becomes available:
renderer.RenderingOptions.JavascriptMessageListener = message => Console.WriteLine($"JS: {message}");
// Log 'foo' to the console
renderer.RenderingOptions.Javascript = "console.log('foo');";
// Render HTML to PDF
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1> Hello World </h1>");
//--------------------------------------------------//
// Error will be logged
// message => Uncaught TypeError: Cannot read properties of null (reading 'style')
renderer.RenderingOptions.Javascript = "document.querySelector('non-existent').style.color='foo';";
// Render HTML to PDF
PdfDocument pdf2 = renderer.RenderHtmlAsPdf("<h1> Hello World </h1>");
Imports IronPdf
Imports System
Private renderer As New ChromePdfRenderer()
' Method callback to be invoked whenever a browser console message becomes available:
renderer.RenderingOptions.JavascriptMessageListener = Sub(message) Console.WriteLine($"JS: {message}")
' Log 'foo' to the console
renderer.RenderingOptions.Javascript = "console.log('foo');"
' Render HTML to PDF
Dim pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1> Hello World </h1>")
'--------------------------------------------------//
' Error will be logged
' message => Uncaught TypeError: Cannot read properties of null (reading 'style')
renderer.RenderingOptions.Javascript = "document.querySelector('non-existent').style.color='foo';"
' Render HTML to PDF
Dim pdf2 As PdfDocument = renderer.RenderHtmlAsPdf("<h1> Hello World </h1>")
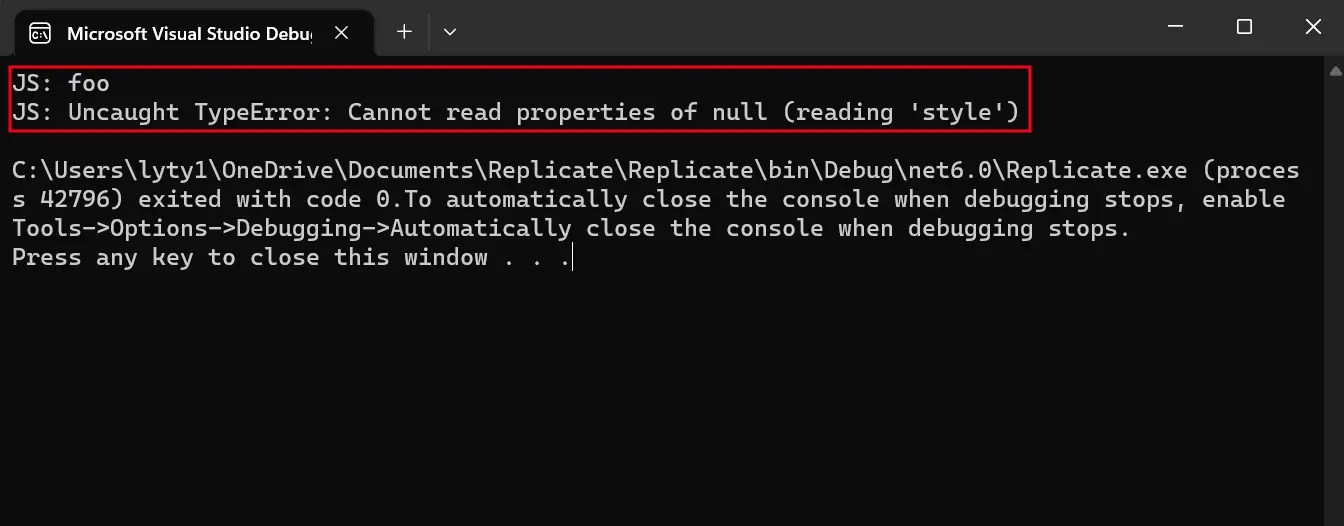
Charting with JavaScript & IronPDF
D3.js is a perfect fit for IronPDF when you want to create charts or images.
D3.js or, simply D3, stands for Data-Driven Documents. It is a JavaScript library that assists in creating dynamic, interactive data visualizations in web browsers. D3 uses Scalable Vector Graphics SVG in HTML5 and Cascading Style Sheets (CSS3).
To create a chart using D3.js and then convert it into a PDF document, use code similar to the following:
- Create a renderer object.
- Set the JavaScript and CSS properties.
- Use the
RenderHTMLFileAsPdf
method of the IronPDF library to read an HTML file (web page). - Simply save it as a PDF.
:path=/static-assets/pdf/content-code-examples/how-to/javascript-to-pdf-render-chart.cs
using IronPdf;
string html = @"
<!DOCTYPE html>
<html>
<head>
<meta charset=""utf-8"" />
<title>C3 Bar Chart</title>
</head>
<body>
<div id=""chart"" style=""width: 950px;""></div>
<script src=""https://d3js.org/d3.v4.js""></script>
<!-- Load c3.css -->
<link href=""https://cdnjs.cloudflare.com/ajax/libs/c3/0.5.4/c3.css"" rel=""stylesheet"">
<!-- Load d3.js and c3.js -->
<script src=""https://cdnjs.cloudflare.com/ajax/libs/c3/0.5.4/c3.js""></script>
<script>
Function.prototype.bind = Function.prototype.bind || function (thisp) {
var fn = this;
return function () {
return fn.apply(thisp, arguments);
};
};
var chart = c3.generate({
bindto: '#chart',
data: {
columns: [['data1', 30, 200, 100, 400, 150, 250],
['data2', 50, 20, 10, 40, 15, 25]]
}
});
</script>
</body>
</html>
";
// Instantiate Renderer
var renderer = new ChromePdfRenderer();
// Enable JavaScript
renderer.RenderingOptions.EnableJavaScript = true;
// Set waitFor for JavaScript
renderer.RenderingOptions.WaitFor.JavaScript(500);
// Render HTML contains JavaScript
var pdfJavaScript = renderer.RenderHtmlAsPdf(html);
// Export PDF
pdfJavaScript.SaveAs("renderChart.pdf");
Imports IronPdf
Private html As String = "
<!DOCTYPE html>
<html>
<head>
<meta charset=""utf-8"" />
<title>C3 Bar Chart</title>
</head>
<body>
<div id=""chart"" style=""width: 950px;""></div>
<script src=""https://d3js.org/d3.v4.js""></script>
<!-- Load c3.css -->
<link href=""https://cdnjs.cloudflare.com/ajax/libs/c3/0.5.4/c3.css"" rel=""stylesheet"">
<!-- Load d3.js and c3.js -->
<script src=""https://cdnjs.cloudflare.com/ajax/libs/c3/0.5.4/c3.js""></script>
<script>
Function.prototype.bind = Function.prototype.bind || function (thisp) {
var fn = this;
return function () {
return fn.apply(thisp, arguments);
};
};
var chart = c3.generate({
bindto: '#chart',
data: {
columns: [['data1', 30, 200, 100, 400, 150, 250],
['data2', 50, 20, 10, 40, 15, 25]]
}
});
</script>
</body>
</html>
"
' Instantiate Renderer
Private renderer = New ChromePdfRenderer()
' Enable JavaScript
renderer.RenderingOptions.EnableJavaScript = True
' Set waitFor for JavaScript
renderer.RenderingOptions.WaitFor.JavaScript(500)
' Render HTML contains JavaScript
Dim pdfJavaScript = renderer.RenderHtmlAsPdf(html)
' Export PDF
pdfJavaScript.SaveAs("renderChart.pdf")
Output PDF
Explore more WaitFor options, such as those for fonts JavaScript, HTML elemnts, and network idle at 'How to use the Waitfor Method to Delay C# PDF Renders.'
Angular JS HTML to PDF
According to Wikipedia:
AngularJS is a JavaScript-based open-source front-end web framework mainly maintained by Google and by a community of individuals and corporations to address many of the challenges encountered in developing single-page applications. It aims to simplify both the development and the testing of such applications by providing a framework for client-side model–view–controller (MVC) and model–view–view model (MVVM) architectures, along with components commonly used in rich Internet applications.
Angular should, however, be used with care. It is better to rely on Server-side rendering (SSR) with Angular Universal for full compatibility.
Server-side rendering (SSR) with Angular Universal
Angular vs. Angular Universal
A typical Angular application runs in the browser (client-side). It renders pages in the DOM (Document Object Model) based on user actions.
Angular Universal runs on the server (server-side). It generates static pages that are later bootstrapped on the client. This results in faster rendering of the Angular application(s) and allows users to view the application layout before it becomes fully interactive.