How to Apply Custom PDF Watermarks
A custom watermark is a personalized background image or text overlay added to a PDF page. It serves various purposes, including branding with logos or names, enhancing security with labels like 'Confidential,' ensuring copyright protection, and indicating document status. Custom watermarks can include text, images, or both, be applied selectively or universally, and their opacity can be adjusted for versatility in personalizing, securing, and contextualizing PDFs.
IronPdf offers a one-liner to add watermark to PDF format documents. The watermark feature accepts a HTML string to generate the watermark, which is capable of using all HTML features as well as CSS styling.
How to Apply Custom Watermarks
- Download the C# library to apply custom watermarks
- Render a new or import an existing PDF document
- Configure the HTML string to be used as a watermark
- Use the
ApplyWatermark
method to apply watermark - Specify rotation, opacity, and location on the document as required
Install with NuGet
Install-Package IronPdf
Apply Watermark Example
Utilize the ApplyWatermark
method to apply a watermark to a newly rendered PDF or an existing one. This method accepts a HTML string as the watermark, enabling it to have all the features that HTML offers, including CSS styling. Let's use both an image and text as our watermark in the example below. Please note that the watermark will be applied to all the pages; it's not possible to apply the watermark to specific pages.
Code
:path=/static-assets/pdf/content-code-examples/how-to/custom-watermark-apply-watermark.cs
using IronPdf;
string watermarkHtml = @"
<img src='https://ironsoftware.com/img/products/ironpdf-logo-text-dotnet.svg'>
<h1>Iron Software</h1>";
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Watermark</h1>");
// Apply watermark
pdf.ApplyWatermark(watermarkHtml);
pdf.SaveAs("watermark.pdf");
Imports IronPdf
Private watermarkHtml As String = "
<img src='https://ironsoftware.com/img/products/ironpdf-logo-text-dotnet.svg'>
<h1>Iron Software</h1>"
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Watermark</h1>")
' Apply watermark
pdf.ApplyWatermark(watermarkHtml)
pdf.SaveAs("watermark.pdf")
Output PDF
This is a very easy way to add image watermark text from a variety of image formats, such as PNG, and text watermark with a custom font.
Watermark Opacity and Rotation
Add watermark with the default opacity of 50%. This level can be further configured according to the user's requirements. As for rotation, there is an overload of the ApplyWatermark
method that also takes rotation as a parameter. By specifying the 'rotation:' and 'opacity:', we can adjust these two parameters.
Code
:path=/static-assets/pdf/content-code-examples/how-to/custom-watermark-apply-rotation-opacity.cs
using IronPdf;
using IronPdf.Editing;
string watermarkHtml = @"
<img style='width: 200px;' src='https://ironsoftware.com/img/products/ironpdf-logo-text-dotnet.svg'>
<h1>Iron Software</h1>";
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Watermark</h1>");
// Apply watermark with 45 degrees rotation and 70% opacity
pdf.ApplyWatermark(watermarkHtml, rotation: 45, opacity: 70);
pdf.SaveAs("watermarkOpacity&Rotation.pdf");
Imports IronPdf
Imports IronPdf.Editing
Private watermarkHtml As String = "
<img style='width: 200px;' src='https://ironsoftware.com/img/products/ironpdf-logo-text-dotnet.svg'>
<h1>Iron Software</h1>"
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Watermark</h1>")
' Apply watermark with 45 degrees rotation and 70% opacity
pdf.ApplyWatermark(watermarkHtml, rotation:= 45, opacity:= 70)
pdf.SaveAs("watermarkOpacity&Rotation.pdf")
Output PDF
Watermark Location on PDF file
To specify the watermark location, we use a 3x3 grid divided into 3 columns horizontally and 3 rows vertically. The horizontal options are left, center, and right, while the vertical options are top, middle, and bottom. With this configuration, we can set 9 different locations on each page of the document. Please refer to the image below for a visual representation of this concept.
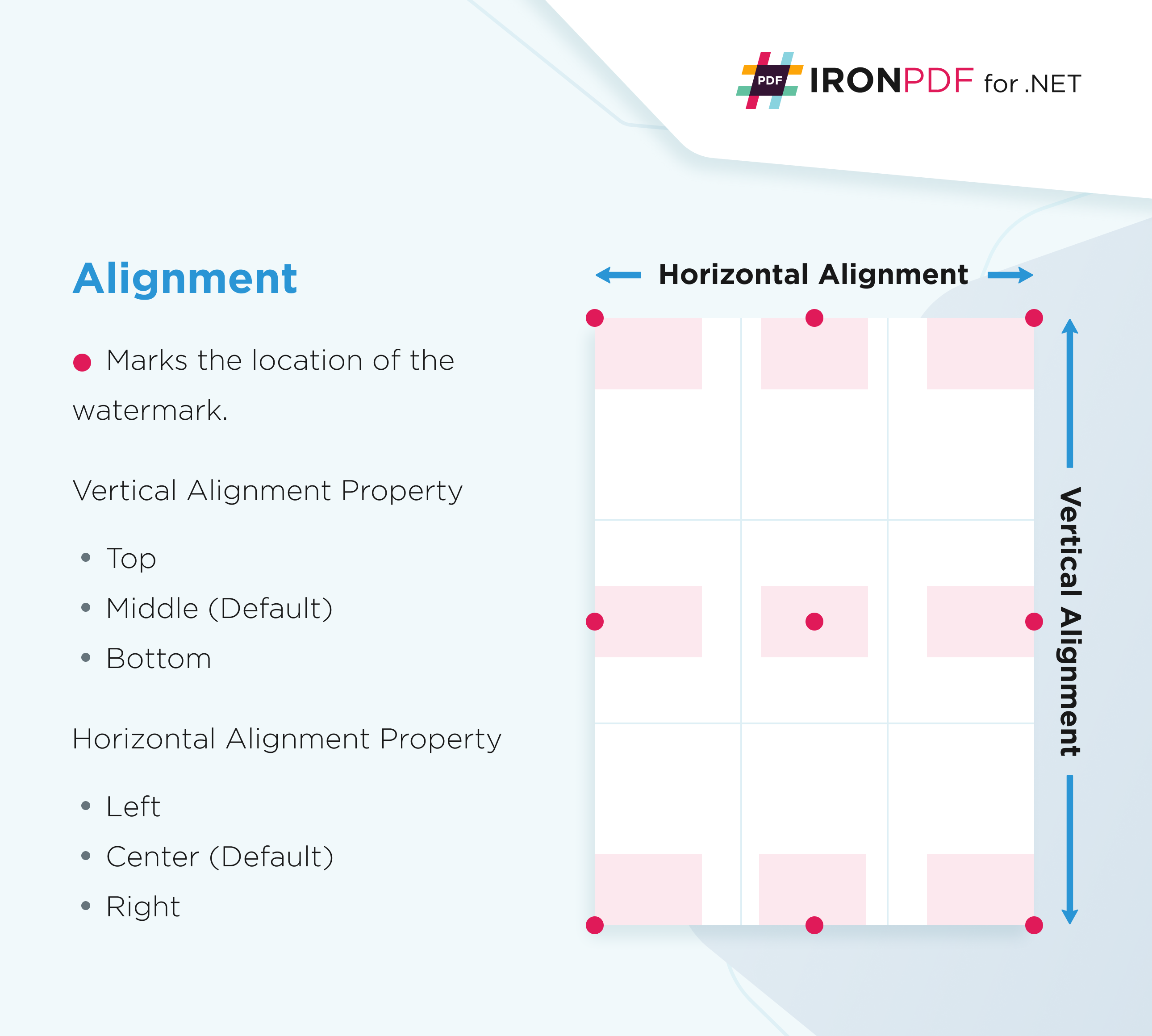
Add watermark to a specific location using the VerticalAlignment and HorizontalAlignment enums in the IronPdf.Editing namespace.
Code
:path=/static-assets/pdf/content-code-examples/how-to/custom-watermark-apply-watermark-top-right.cs
using IronPdf;
using IronPdf.Editing;
string watermarkHtml = @"
<img style='width: 200px;' src='https://ironsoftware.com/img/products/ironpdf-logo-text-dotnet.svg'>
<h1>Iron Software</h1>";
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Watermark</h1>");
// Apply watermark on the top-right of the document
pdf.ApplyWatermark(watermarkHtml, 50, VerticalAlignment.Top, HorizontalAlignment.Right);
pdf.SaveAs("watermarkLocation.pdf");
Imports IronPdf
Imports IronPdf.Editing
Private watermarkHtml As String = "
<img style='width: 200px;' src='https://ironsoftware.com/img/products/ironpdf-logo-text-dotnet.svg'>
<h1>Iron Software</h1>"
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Watermark</h1>")
' Apply watermark on the top-right of the document
pdf.ApplyWatermark(watermarkHtml, 50, VerticalAlignment.Top, HorizontalAlignment.Right)
pdf.SaveAs("watermarkLocation.pdf")