How to Create PDF Forms
IronPDF offers a comprehensive set of form creation capabilities. It provides support for various types of form elements, including input fields, text areas, checkboxes, comboboxes, radio buttons, and image form. With IronPDF, you can easily generate dynamic PDF forms that allow users to interact with the document by filling out form fields, making selections, and saving changes. This enables you to create interactive and user-friendly PDF forms for a wide range of applications and scenarios.
How to Create PDF Forms
Install with NuGet
Install-Package IronPdf
Create Forms
IronPDF effortlessly create PDF documents with embedded form fields of various types. By adding dynamic form elements to an otherwise static PDF document, you can enhance its flexibility and interactivity.
Text Area and Input Forms
Render From HTML
You can easily create text area and input forms to capture user input within your PDF documents. Text area forms provide ample space for displaying and capturing larger amounts of text, while input forms allow users to enter specific values or responses.
:path=/static-assets/pdf/content-code-examples/how-to/create-forms-input-textarea.cs
using IronPdf;
// Input and Text Area forms HTML
string FormHtml = @"
<html>
<body>
<h2>Editable PDF Form</h2>
<form>
First name: <br> <input type='text' name='firstname' value=''> <br>
Last name: <br> <input type='text' name='lastname' value=''> <br>
Address: <br> <textarea name='address' rows='4' cols='50'></textarea>
</form>
</body>
</html>
";
// Instantiate Renderer
ChromePdfRenderer Renderer = new ChromePdfRenderer();
Renderer.RenderingOptions.CreatePdfFormsFromHtml = true;
Renderer.RenderHtmlAsPdf(FormHtml).SaveAs("textAreaAndInputForm.pdf");
Imports IronPdf
' Input and Text Area forms HTML
Private FormHtml As String = "
<html>
<body>
<h2>Editable PDF Form</h2>
<form>
First name: <br> <input type='text' name='firstname' value=''> <br>
Last name: <br> <input type='text' name='lastname' value=''> <br>
Address: <br> <textarea name='address' rows='4' cols='50'></textarea>
</form>
</body>
</html>
"
' Instantiate Renderer
Private Renderer As New ChromePdfRenderer()
Renderer.RenderingOptions.CreatePdfFormsFromHtml = True
Renderer.RenderHtmlAsPdf(FormHtml).SaveAs("textAreaAndInputForm.pdf")
Output PDF document
Add Text Form via Code
The above code example talks about rendering HTML that contains text areas and input forms. However, it is also possible to add a text form field through code. First, instantiate a TextFormField object with the required parameters. Next, use the Add
method of the Form property to add the created form.
:path=/static-assets/pdf/content-code-examples/how-to/create-forms-add-input-textarea.cs
using IronPdf;
using IronSoftware.Forms;
// Instantiate ChromePdfRenderer
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h2>Editable PDF Form</h2>");
// Configure required parameters
string name = "firstname";
string value = "first name";
int pageIndex = 0;
double x = 100;
double y = 700;
double width = 50;
double height = 15;
// Create text form field
var textForm = new TextFormField(name, value, pageIndex, x, y, width, height);
// Add form
pdf.Form.Add(textForm);
pdf.SaveAs("addTextForm.pdf");
Imports IronPdf
Imports IronSoftware.Forms
' Instantiate ChromePdfRenderer
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h2>Editable PDF Form</h2>")
' Configure required parameters
Private name As String = "firstname"
Private value As String = "first name"
Private pageIndex As Integer = 0
Private x As Double = 100
Private y As Double = 700
Private width As Double = 50
Private height As Double = 15
' Create text form field
Private textForm = New TextFormField(name, value, pageIndex, x, y, width, height)
' Add form
pdf.Form.Add(textForm)
pdf.SaveAs("addTextForm.pdf")
Output PDF document
Having a text form field in a PDF without a label might not be very meaningful. To enhance its usefulness, you should add text to the PDF as a label for the form field. IronPdf has you covered. You can achieve this by using the DrawText
method. Learn more about How to Draw Text and Bitmap on PDFs.
Checkbox and Combobox Forms
Render From HTML
Similarly, checkbox and combobox forms can be created by rendering from an HTML string, file, or web URL that contains checkboxes and comboboxes. Set the CreatePdfFormsFromHtml property to true to enable the creation of these forms.
Combobox forms provide users with a dropdown selection of options. Users can choose from the available options, offering valuable input within the PDF documents.
:path=/static-assets/pdf/content-code-examples/how-to/create-forms-checkbox-combobox.cs
using IronPdf;
// Input and Text Area forms HTML
string FormHtml = @"
<html>
<body>
<h2>Editable PDF Form</h2>
<h2>Task Completed</h2>
<label>
<input type='checkbox' id='taskCompleted' name='taskCompleted'> Mark task as completed
</label>
<h2>Select Priority</h2>
<label for='priority'>Choose priority level:</label>
<select id='priority' name='priority'>
<option value='high'>High</option>
<option value='medium'>Medium</option>
<option value='low'>Low</option>
</select>
</body>
</html>
";
// Instantiate Renderer
ChromePdfRenderer Renderer = new ChromePdfRenderer();
Renderer.RenderingOptions.CreatePdfFormsFromHtml = true;
Renderer.RenderHtmlAsPdf(FormHtml).SaveAs("checkboxAndComboboxForm.pdf");
Imports IronPdf
' Input and Text Area forms HTML
Private FormHtml As String = "
<html>
<body>
<h2>Editable PDF Form</h2>
<h2>Task Completed</h2>
<label>
<input type='checkbox' id='taskCompleted' name='taskCompleted'> Mark task as completed
</label>
<h2>Select Priority</h2>
<label for='priority'>Choose priority level:</label>
<select id='priority' name='priority'>
<option value='high'>High</option>
<option value='medium'>Medium</option>
<option value='low'>Low</option>
</select>
</body>
</html>
"
' Instantiate Renderer
Private Renderer As New ChromePdfRenderer()
Renderer.RenderingOptions.CreatePdfFormsFromHtml = True
Renderer.RenderHtmlAsPdf(FormHtml).SaveAs("checkboxAndComboboxForm.pdf")
Output PDF document
Add Form via Code
Checkbox
To add a checkbox form field, first instantiate a CheckboxFormField object with the required parameters. The value parameter of the checkbox will determine whether the form should be checked or not, with "no" value for not checked and "yes" value for checked. Finally, use the Add
method of the Form property to add the created form.
:path=/static-assets/pdf/content-code-examples/how-to/create-forms-add-checkbox.cs
using IronPdf;
using IronSoftware.Forms;
// Instantiate ChromePdfRenderer
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h2>Checkbox Form Field</h2>");
// Configure required parameters
string name = "checkbox";
string value = "no";
int pageIndex = 0;
double x = 100;
double y = 700;
double width = 15;
double height = 15;
// Create checkbox form field
var checkboxForm = new CheckboxFormField(name, value, pageIndex, x, y, width, height);
// Add form
pdf.Form.Add(checkboxForm);
pdf.SaveAs("addCheckboxForm.pdf");
Imports IronPdf
Imports IronSoftware.Forms
' Instantiate ChromePdfRenderer
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h2>Checkbox Form Field</h2>")
' Configure required parameters
Private name As String = "checkbox"
Private value As String = "no"
Private pageIndex As Integer = 0
Private x As Double = 100
Private y As Double = 700
Private width As Double = 15
Private height As Double = 15
' Create checkbox form field
Private checkboxForm = New CheckboxFormField(name, value, pageIndex, x, y, width, height)
' Add form
pdf.Form.Add(checkboxForm)
pdf.SaveAs("addCheckboxForm.pdf")
Output PDF document
Combobox
To add a combobox form field, first instantiate a ComboboxFormField object with the required parameters. Similar to the checkbox form, the value parameter of the combobox determines which choice will be selected. Finally, use the Add
method of the Form property to add the created form.
:path=/static-assets/pdf/content-code-examples/how-to/create-forms-add-combobox.cs
using IronPdf;
using IronSoftware.Forms;
using System.Collections.Generic;
// Instantiate ChromePdfRenderer
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h2>Combobox Form Field</h2>");
// Configure required parameters
string name = "combobox";
string value = "Car";
int pageIndex = 0;
double x = 100;
double y = 700;
double width = 15;
double height = 15;
var choices = new List<string>() { "Car", "Bike", "Airplane" };
// Create combobox form field
var comboboxForm = new ComboboxFormField(name, value, pageIndex, x, y, width, height, choices);
// Add form
pdf.Form.Add(comboboxForm);
pdf.SaveAs("addComboboxForm.pdf");
Imports IronPdf
Imports IronSoftware.Forms
Imports System.Collections.Generic
' Instantiate ChromePdfRenderer
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h2>Combobox Form Field</h2>")
' Configure required parameters
Private name As String = "combobox"
Private value As String = "Car"
Private pageIndex As Integer = 0
Private x As Double = 100
Private y As Double = 700
Private width As Double = 15
Private height As Double = 15
Private choices = New List(Of String)() From {"Car", "Bike", "Airplane"}
' Create combobox form field
Private comboboxForm = New ComboboxFormField(name, value, pageIndex, x, y, width, height, choices)
' Add form
pdf.Form.Add(comboboxForm)
pdf.SaveAs("addComboboxForm.pdf")
Radio buttons Forms
Render From HTML
When working with radio button forms in IronPDF, radio buttons of the same group are contained within one form object. You can retrieve that form by inputting its name into the FindFormField
method. If any of the radio choices is selected, the Value property of that form will have that value; otherwise, it will have a value of 'None'.
:path=/static-assets/pdf/content-code-examples/how-to/create-forms-radiobutton.cs
using IronPdf;
// Radio buttons HTML
string FormHtml = @"
<html>
<body>
<h2>Editable PDF Form</h2>
Choose your preferred travel type: <br>
<input type='radio' name='traveltype' value='Bike'>
Bike <br>
<input type='radio' name='traveltype' value='Car'>
Car <br>
<input type='radio' name='traveltype' value='Airplane'>
Airplane
</body>
</html>
";
// Instantiate Renderer
ChromePdfRenderer Renderer = new ChromePdfRenderer();
Renderer.RenderingOptions.CreatePdfFormsFromHtml = true;
Renderer.RenderHtmlAsPdf(FormHtml).SaveAs("radioButtomForm.pdf");
Imports IronPdf
' Radio buttons HTML
Private FormHtml As String = "
<html>
<body>
<h2>Editable PDF Form</h2>
Choose your preferred travel type: <br>
<input type='radio' name='traveltype' value='Bike'>
Bike <br>
<input type='radio' name='traveltype' value='Car'>
Car <br>
<input type='radio' name='traveltype' value='Airplane'>
Airplane
</body>
</html>
"
' Instantiate Renderer
Private Renderer As New ChromePdfRenderer()
Renderer.RenderingOptions.CreatePdfFormsFromHtml = True
Renderer.RenderHtmlAsPdf(FormHtml).SaveAs("radioButtomForm.pdf")
Output PDF document
Add Radio Form via Code
Similarly radio button form field can also be added through code. First, instantiate a RadioFormField object with the required parameters. Next, use the Add
method of the Form property to add the created form.
:path=/static-assets/pdf/content-code-examples/how-to/create-forms-add-radiobutton.cs
using IronPdf;
using IronSoftware.Forms;
// Instantiate ChromePdfRenderer
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h2>Editable PDF Form</h2>");
// Configure required parameters
string name = "choice";
string value = "yes";
int pageIndex = 0;
double x = 100;
double y = 700;
double width = 15;
double height = 15;
// Create the first radio form
var yesRadioform = new RadioFormField(name, value, pageIndex, x, y, width, height);
value = "no";
x = 200;
// Create the second radio form
var noRadioform = new RadioFormField(name, value, pageIndex, x, y, width, height);
pdf.Form.Add(yesRadioform);
pdf.Form.Add(noRadioform);
pdf.SaveAs("addRadioForm.pdf");
Imports IronPdf
Imports IronSoftware.Forms
' Instantiate ChromePdfRenderer
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h2>Editable PDF Form</h2>")
' Configure required parameters
Private name As String = "choice"
Private value As String = "yes"
Private pageIndex As Integer = 0
Private x As Double = 100
Private y As Double = 700
Private width As Double = 15
Private height As Double = 15
' Create the first radio form
Private yesRadioform = New RadioFormField(name, value, pageIndex, x, y, width, height)
value = "no"
x = 200
' Create the second radio form
Dim noRadioform = New RadioFormField(name, value, pageIndex, x, y, width, height)
pdf.Form.Add(yesRadioform)
pdf.Form.Add(noRadioform)
pdf.SaveAs("addRadioForm.pdf")
Output PDF document
As a final touch, use the DrawText
method to add labels for the radio buttons. Learn more about How to Draw Text and Bitmap on PDFs.
Image Forms via Code
Image form field can only be added through code. First, instantiate a ImageFormField object with the required parameters. Next, use the Add
method of the Form property to add the created form.
:path=/static-assets/pdf/content-code-examples/how-to/create-forms-add-image.cs
using IronPdf;
using IronSoftware.Forms;
// Instantiate ChromePdfRenderer
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h2>Editable PDF Form</h2>");
// Configure required parameters
string name = "image1";
int pageIndex = 0;
double x = 100;
double y = 600;
double width = 200;
double height = 200;
// Create the image form
ImageFormField imageForm = new ImageFormField(name, pageIndex, x, y, width, height);
pdf.Form.Add(imageForm);
pdf.SaveAs("addImageForm.pdf");
Imports IronPdf
Imports IronSoftware.Forms
' Instantiate ChromePdfRenderer
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h2>Editable PDF Form</h2>")
' Configure required parameters
Private name As String = "image1"
Private pageIndex As Integer = 0
Private x As Double = 100
Private y As Double = 600
Private width As Double = 200
Private height As Double = 200
' Create the image form
Private imageForm As New ImageFormField(name, pageIndex, x, y, width, height)
pdf.Form.Add(imageForm)
pdf.SaveAs("addImageForm.pdf")
Output PDF document
Output PDF file: addImageForm.pdf. Browsers do not support image forms; please open in Adobe Acrobat to try the feature out.
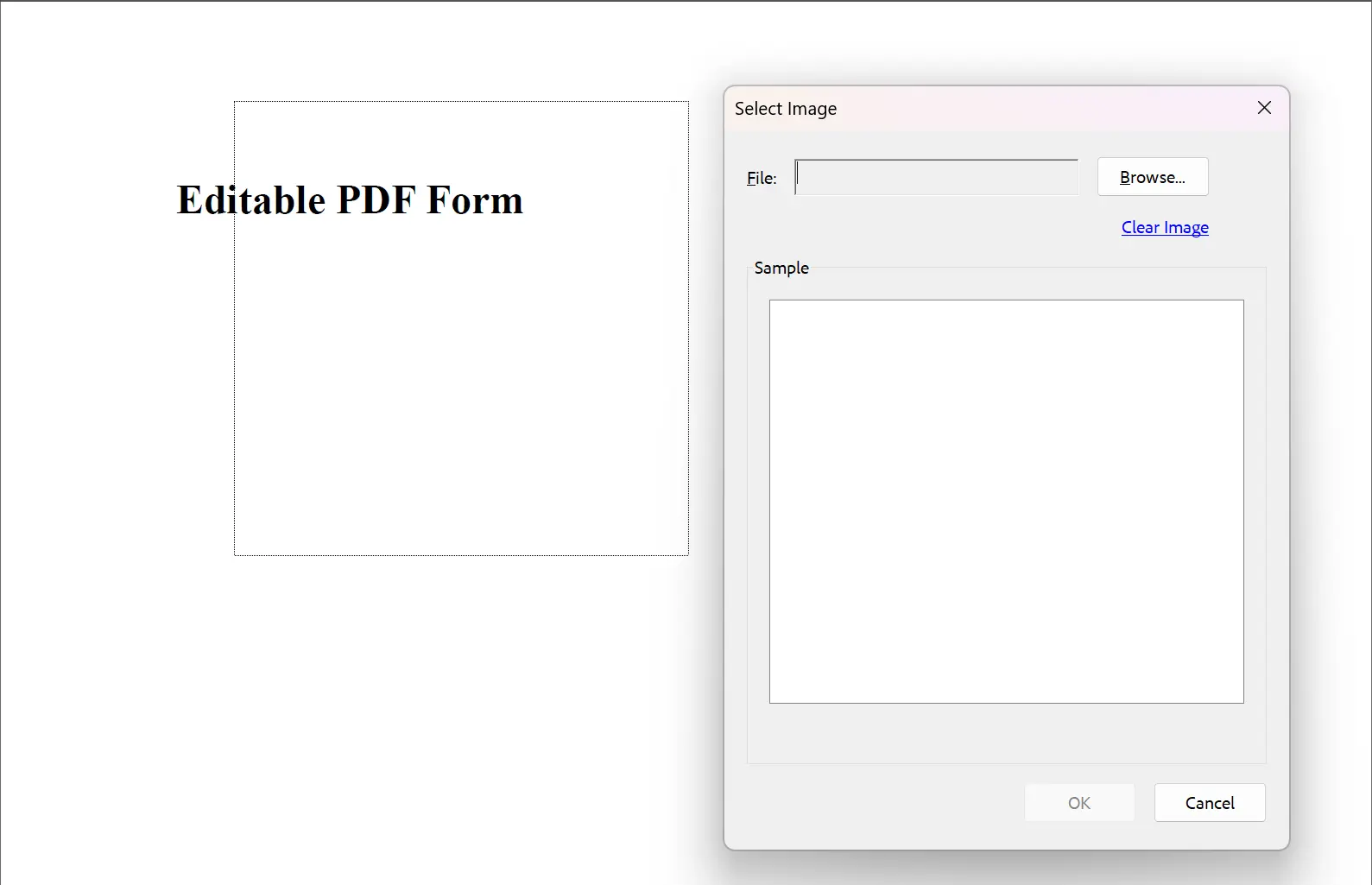
Learn how to fill and edit PDF forms programmatically in the following article: "How to Fill and Edit PDF Forms."