How to use OpenAI for PDF
OpenAI is an artificial intelligence research laboratory, consisting of the for-profit OpenAI LP and its non-profit parent company, OpenAI Inc. It was founded with the goal of advancing digital intelligence in a way that benefits humanity as a whole. OpenAI conducts research in various areas of artificial intelligence (AI) and aims to develop AI technologies that are safe, beneficial, and accessible.
The IronPdf.Extensions.AI
NuGet package now enables OpenAI for PDF enhancement: summarization, querying, and memorization. The package utilizes Microsoft Semantic Kernel.
How to use OpenAI for PDF
- Download the C# library to utilize OpenAI for PDF
- Prepare the Azure Endpoint and API Key for OpenAI
- Import the target PDF document
- Use the
Summarize
method to generate a summary of the PDF - Use the
Query
method for continuous querying
Install with NuGet
Install-Package IronPdf
Summarize PDF Example
To use the OpenAI feature, an Azure Endpoint, and an API Key are needed. Configure the Semantic Kernel according to the code example below. Import the PDF document and utilize the Summarize
method to generate a summary of the PDF document. You can download the sample PDF file here.
:path=/static-assets/pdf/content-code-examples/how-to/openai-summarize.cs
using IronPdf;
using IronPdf.AI;
using Microsoft.SemanticKernel;
using Microsoft.SemanticKernel.Memory;
using System;
using System.Threading.Tasks;
// Setup OpenAI
string azureEndpoint = "AzureEndPoint";
string apiKey = "APIKEY";
var volatileMemoryStore = new VolatileMemoryStore();
var builder = new KernelBuilder()
.WithAzureTextEmbeddingGenerationService("oaiembed", azureEndpoint, apiKey)
.WithAzureChatCompletionService("oaichat", azureEndpoint, apiKey)
.WithMemoryStorage(volatileMemoryStore);
var kernel = builder.Build();
// Initialize IronAI
IronAI.Initialize(kernel);
// Import PDF document
PdfDocument pdf = PdfDocument.FromFile("wikipedia.pdf");
// Summarize the document
string summary = await pdf.Summarize(); // optionally pass AI instance or use AI instance directly
Console.WriteLine($"Document summary: {summary}");
Imports IronPdf
Imports IronPdf.AI
Imports Microsoft.SemanticKernel
Imports Microsoft.SemanticKernel.Memory
Imports System
Imports System.Threading.Tasks
' Setup OpenAI
Private azureEndpoint As String = "AzureEndPoint"
Private apiKey As String = "APIKEY"
Private volatileMemoryStore = New VolatileMemoryStore()
Private builder = (New KernelBuilder()).WithAzureTextEmbeddingGenerationService("oaiembed", azureEndpoint, apiKey).WithAzureChatCompletionService("oaichat", azureEndpoint, apiKey).WithMemoryStorage(volatileMemoryStore)
Private kernel = builder.Build()
' Initialize IronAI
IronAI.Initialize(kernel)
' Import PDF document
Dim pdf As PdfDocument = PdfDocument.FromFile("wikipedia.pdf")
' Summarize the document
Dim summary As String = Await pdf.Summarize() ' optionally pass AI instance or use AI instance directly
Console.WriteLine($"Document summary: {summary}")
Output Summary
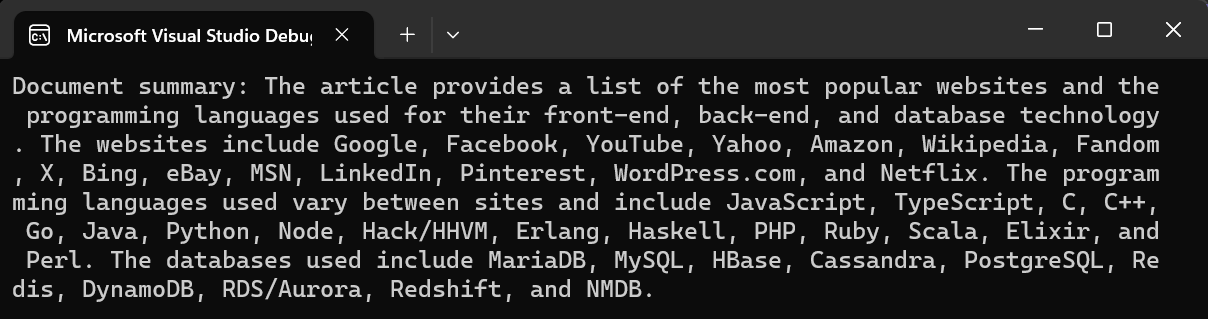
Continuous Query Example
A single query may not be suitable for all scenarios. The IronPdf.Extensions.AI
package also offers a Query method that allows users to perform continuous queries.
:path=/static-assets/pdf/content-code-examples/how-to/openai-query.cs
using IronPdf;
using IronPdf.AI;
using Microsoft.SemanticKernel;
using Microsoft.SemanticKernel.Memory;
using System;
using System.Threading.Tasks;
// Setup OpenAI
string azureEndpoint = "AzureEndPoint";
string apiKey = "APIKEY";
var volatileMemoryStore = new VolatileMemoryStore();
var builder = new KernelBuilder()
.WithAzureTextEmbeddingGenerationService("oaiembed", azureEndpoint, apiKey)
.WithAzureChatCompletionService("oaichat", azureEndpoint, apiKey)
.WithMemoryStorage(volatileMemoryStore);
var kernel = builder.Build();
// Initialize IronAI
IronAI.Initialize(kernel);
// Import PDF document
PdfDocument pdf = PdfDocument.FromFile("wikipedia.pdf");
// Continuous query
while (true)
{
Console.Write("User Input: ");
var response = await pdf.Query(Console.ReadLine());
Console.WriteLine($"\n{response}");
}
Imports Microsoft.VisualBasic
Imports IronPdf
Imports IronPdf.AI
Imports Microsoft.SemanticKernel
Imports Microsoft.SemanticKernel.Memory
Imports System
Imports System.Threading.Tasks
' Setup OpenAI
Private azureEndpoint As String = "AzureEndPoint"
Private apiKey As String = "APIKEY"
Private volatileMemoryStore = New VolatileMemoryStore()
Private builder = (New KernelBuilder()).WithAzureTextEmbeddingGenerationService("oaiembed", azureEndpoint, apiKey).WithAzureChatCompletionService("oaichat", azureEndpoint, apiKey).WithMemoryStorage(volatileMemoryStore)
Private kernel = builder.Build()
' Initialize IronAI
IronAI.Initialize(kernel)
' Import PDF document
Dim pdf As PdfDocument = PdfDocument.FromFile("wikipedia.pdf")
' Continuous query
Do
Console.Write("User Input: ")
Dim response = Await pdf.Query(Console.ReadLine())
Console.WriteLine($vbLf & "{response}")
Loop