How to Render HTML String to PDF
IronPDF allows developers to create PDF documents easily in C#, F#, and VB.NET for .NET Core and .NET Framework. IronPdf supports rendering any HTML string into a PDF, and the rendering process is undertaken by a fully functional version of the Google Chromium engine.
Get started with IronPDF
Start using IronPDF in your project today with a free trial.
How to Render HTML String to PDF
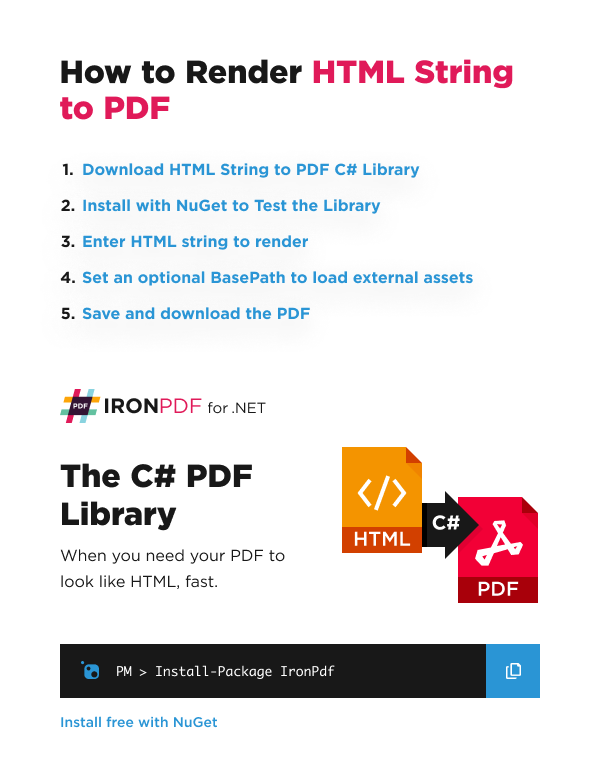
- Download IronPDF C# Library from NuGet
- Instantiate the PDF Renderer and Pass the HTML String
- Configure BasePath for External Assets in PDF
- Configure the RenderingOptions to fine-tune the output PDF
- Save and Download the Generated PDF
HTML String to PDF Example
Here we have an example of IronPDF rendering an HTML string into a PDF by using the RenderHtmlAsPdf
method. The parameter is an HTML string to be rendered as a PDF.
:path=/static-assets/pdf/content-code-examples/how-to/html-string-to-pdf.cs
using IronPdf;
// Instantiate Renderer
var renderer = new ChromePdfRenderer();
// Create a PDF from a HTML string using C#
var pdf = renderer.RenderHtmlAsPdf("<h1>Hello World</h1>");
// Export to a file or Stream
pdf.SaveAs("output.pdf");
Imports IronPdf
' Instantiate Renderer
Private renderer = New ChromePdfRenderer()
' Create a PDF from a HTML string using C#
Private pdf = renderer.RenderHtmlAsPdf("<h1>Hello World</h1>")
' Export to a file or Stream
pdf.SaveAs("output.pdf")
The RenderHtmlAsPdf
method returns a PdfDocument object, which is a class used to hold PDF information.
In cases where an HTML string is obtained from an external source, and disabling local disk access or cross-origin requests is desired, the Installation.EnableWebSecurity property can be set to true to achieve that.
Result
This is the file that the code produced:
Advanced HTML to PDF Example
Here we have an example of IronPDF loading an external image asset from an optional BasePath. Setting the BaseUrlOrPath property gives the relative file path or URL context for hyperlinks, images, CSS, and JavaScript files.
:path=/static-assets/pdf/content-code-examples/how-to/html-string-to-pdf-2.cs
using IronPdf;
// Instantiate Renderer
var renderer = new ChromePdfRenderer();
// Advanced Example with HTML Assets
// Load external html assets: Images, CSS and JavaScript.
// An optional BasePath 'C:\site\assets\' is set as the file location to load assets from
var myAdvancedPdf = renderer.RenderHtmlAsPdf("<img src='icons/iron.png'>", @"C:\site\assets\");
myAdvancedPdf.SaveAs("html-with-assets.pdf");
Imports IronPdf
' Instantiate Renderer
Private renderer = New ChromePdfRenderer()
' Advanced Example with HTML Assets
' Load external html assets: Images, CSS and JavaScript.
' An optional BasePath 'C:\site\assets\' is set as the file location to load assets from
Private myAdvancedPdf = renderer.RenderHtmlAsPdf("<img src='icons/iron.png'>", "C:\site\assets\")
myAdvancedPdf.SaveAs("html-with-assets.pdf")
This is the file that the code produced: