How to Set Password and Permissions on a PDF
Password protection involves encrypting the document to restrict unauthorized access. It typically includes two types of passwords: the user password (or open password), required to open the document, and the owner password (or permissions password), which controls permissions for editing, printing, and other actions.
IronPDF supports everything you need for Password and Permissions for your existing and new PDF files. Granular meta-data and security settings can be applied, this includes the ability to limit PDF documents to be unprintable, read only, and encrypted, 128 bit encryption, decryption, and password protection are all supported.
How to Protect PDF with Password and Permissions in C#
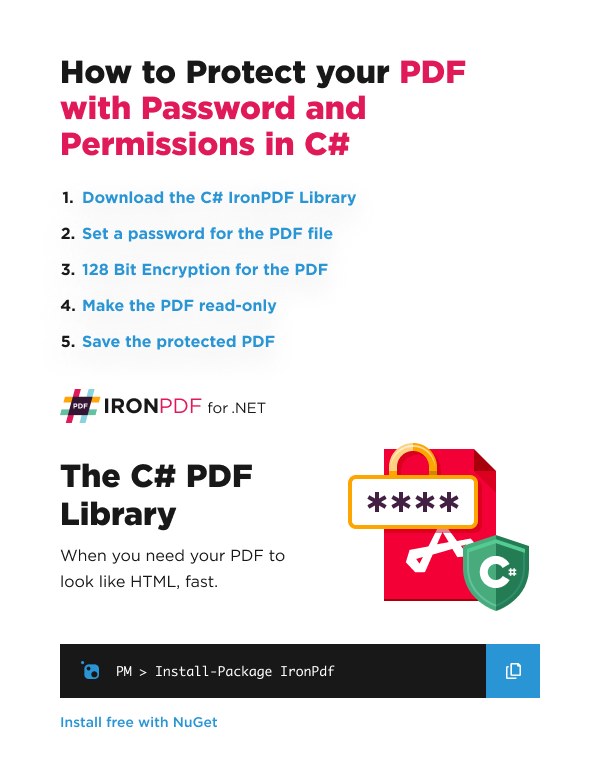
- Download C# library to protect PDF with password
- Set OwnerPassword property to prevent PDF file from being edited
- Set UserPassword property to prevent PDF file from being opened
- Encrypt PDF file with 128 Bit Encryption
- Supply the password to
FromFile
method to open PDF document
Install with NuGet
Install-Package IronPdf
Install with NuGet
Install-Package IronPdf
Start using IronPDF in your project today with a free trial.
Check out IronPDF on Nuget for quick installation and deployment. With over 8 million downloads, it's transforming PDF with C#.
Consider installing the IronPDF DLL directly. Download and manually install it for your project or GAC form: IronPdf.zip
Set a Password for a PDF
Here we have an example PDF that we want to protect using IronPDF. Let's execute the following code to add a password to the PDF. In this example, we will use the password password123.
:path=/static-assets/pdf/content-code-examples/how-to/pdf-permissions-passwords-add-password.cs
using IronPdf;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Secret Information:</h1> Hello World");
// Password to edit the pdf
pdf.SecuritySettings.OwnerPassword = "123password";
// Password to open the pdf
pdf.SecuritySettings.UserPassword = "password123";
pdf.SaveAs("protected.pdf");
Imports IronPdf
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Secret Information:</h1> Hello World")
' Password to edit the pdf
pdf.SecuritySettings.OwnerPassword = "123password"
' Password to open the pdf
pdf.SecuritySettings.UserPassword = "password123"
pdf.SaveAs("protected.pdf")
The result is the following PDF which you can view by typing the password password123.
Open a PDF that has a Password
This is how we can open a PDF that has a password. The PdfDocument.FromFile
method has a second optional parameter which is the password. Provide the correct password to this parameter to open the PDF.
:path=/static-assets/pdf/content-code-examples/how-to/pdf-permissions-passwords-open-password.cs
using IronPdf;
var pdf = PdfDocument.FromFile("protected.pdf", "password123");
//... perform PDF-tasks
pdf.SaveAs("protected_2.pdf"); // Saved as another file
Imports IronPdf
Private pdf = PdfDocument.FromFile("protected.pdf", "password123")
'... perform PDF-tasks
pdf.SaveAs("protected_2.pdf") ' Saved as another file
Advanced Security and Permissions Settings
The PdfDocument object also has MetaData fields you may set such as Author and ModifiedDate. You can also disable User Annotations, User Printing, and many more as shown below:
:path=/static-assets/pdf/content-code-examples/how-to/pdf-permissions-passwords-advanced.cs
using IronPdf;
// Open an Encrypted File, alternatively create a new PDF from HTML
var pdf = PdfDocument.FromFile("protected.pdf", "password123");
// Edit file security settings
// The following code makes a PDF read only and will disallow copy & paste and printing
pdf.SecuritySettings.RemovePasswordsAndEncryption();
pdf.SecuritySettings.MakePdfDocumentReadOnly("secret-key");
pdf.SecuritySettings.AllowUserAnnotations = false;
pdf.SecuritySettings.AllowUserCopyPasteContent = false;
pdf.SecuritySettings.AllowUserFormData = false;
pdf.SecuritySettings.AllowUserPrinting = IronPdf.Security.PdfPrintSecurity.FullPrintRights;
// Save the secure PDF
pdf.SaveAs("secured.pdf");
Imports IronPdf
' Open an Encrypted File, alternatively create a new PDF from HTML
Private pdf = PdfDocument.FromFile("protected.pdf", "password123")
' Edit file security settings
' The following code makes a PDF read only and will disallow copy & paste and printing
pdf.SecuritySettings.RemovePasswordsAndEncryption()
pdf.SecuritySettings.MakePdfDocumentReadOnly("secret-key")
pdf.SecuritySettings.AllowUserAnnotations = False
pdf.SecuritySettings.AllowUserCopyPasteContent = False
pdf.SecuritySettings.AllowUserFormData = False
pdf.SecuritySettings.AllowUserPrinting = IronPdf.Security.PdfPrintSecurity.FullPrintRights
' Save the secure PDF
pdf.SaveAs("secured.pdf")
The permissions setting is related to the document password and behaves as follows. For example, setting the AllowUserCopyPasteContent property to false is intended to prevent copy/paste of content:
- No password set: Without a password, copy/paste of content remains blocked.
- User password set: When a user password is set, entering the correct password will allow copy/paste of content.
- Owner password set: When an owner password is set, entering only the user password will not unlock the copy/paste feature. However, entering the correct owner password will allow copy/paste of content.
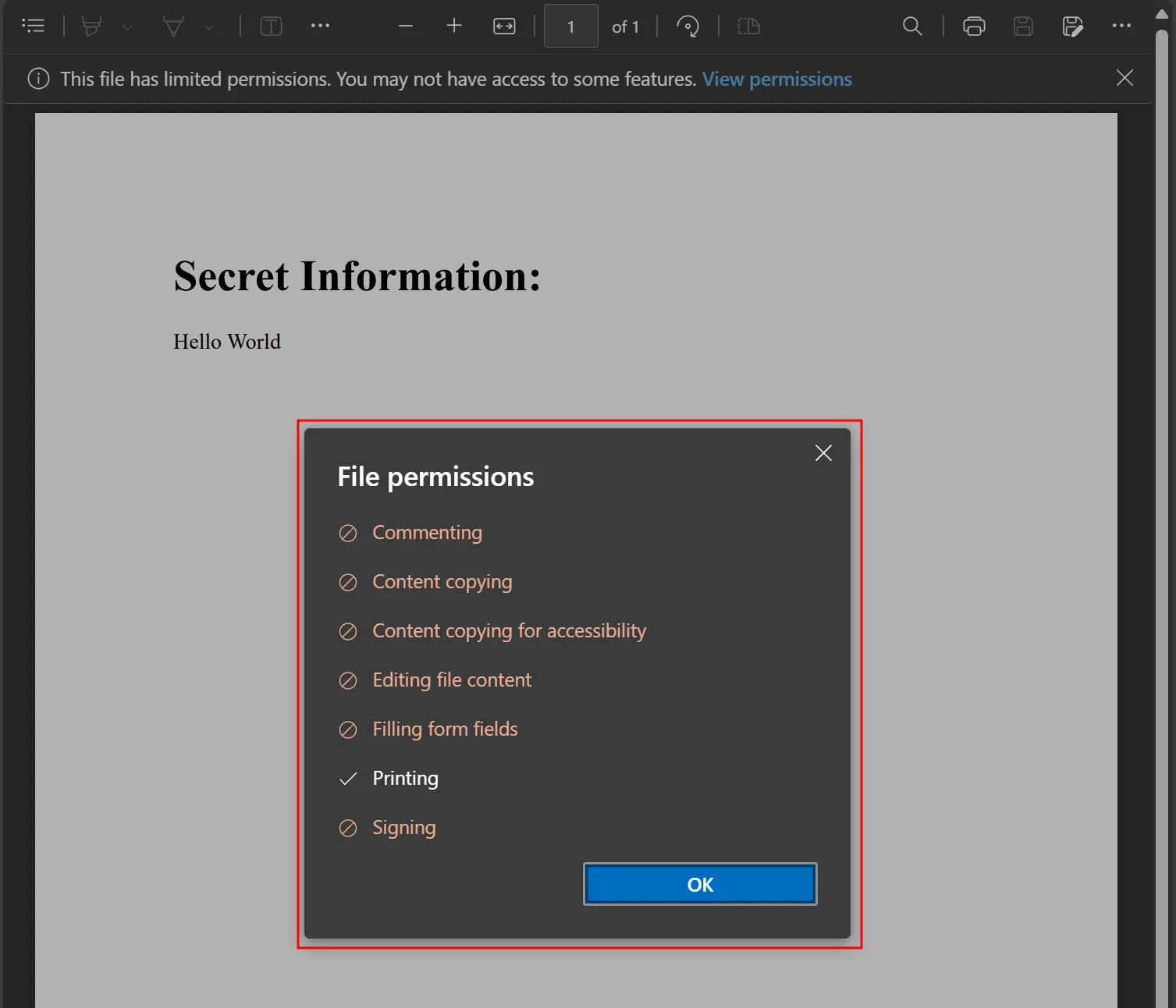
A closely related article discusses predefined and custom metadata. Learn more by following this link: "How to Set and Edit PDF Metadata."