How to Convert Razor Views to PDFs Headlessly
The term 'headless rendering' refers to the process of rendering web content without a graphical user interface (GUI) or browser window. While the IronPdf.Extensions.Razor package is very useful, it does not offer headless rendering capabilities. Headless rendering can address the use case gap that the IronPdf.Extensions.Razor package cannot fulfill.
We will utilize the Razor.Templating.Core package to convert from cshtml (Razor Views) to html, and then utilize IronPDF to generate PDF documents from it.
This article is inspired by the following YouTube video:
How to Convert Razor Views to PDFs Headlessly
- Download the C# library for converting Razor Views to PDFs in ASP.NET Core Web App
- Create a new Razor View and edit the file to display the data
- Use the
RenderAsync
method to convert from Razor View to HTML - Conert HTML to PDF using the
RenderHtmlAsPdf
method - Download the sample project for a quick start
Install with NuGet
Install-Package IronPdf
Install the Razor.Templating.Core package to convert Razor Views to html documents in a ASP.NET Core Web App.
Install-Package Razor.Templating.Core
Render Razor Views to PDFs
You'll need an ASP.NET Core Web App (Model-View-Controller) project to convert Views into PDF files.
Add a View
- Right-click on the "Home" folder. Choose "add" and "Add View."
- Create empty Razor View and name it "Data.cshtml".
Edit Data.cshtml File
Add the HTML string that you would like to render to PDF:
<table class="table">
<tr>
<th>Name</th>
<th>Title</th>
<th>Description</th>
</tr>
<tr>
<td>John Doe</td>
<td>Software Engineer</td>
<td>Experienced software engineer specializing in web development.</td>
</tr>
<tr>
<td>Alice Smith</td>
<td>Project Manager</td>
<td>Seasoned project manager with expertise in agile methodologies.</td>
</tr>
<tr>
<td>Michael Johnson</td>
<td>Data Analyst</td>
<td>Skilled data analyst proficient in statistical analysis and data visualization.</td>
</tr>
</table>
<table class="table">
<tr>
<th>Name</th>
<th>Title</th>
<th>Description</th>
</tr>
<tr>
<td>John Doe</td>
<td>Software Engineer</td>
<td>Experienced software engineer specializing in web development.</td>
</tr>
<tr>
<td>Alice Smith</td>
<td>Project Manager</td>
<td>Seasoned project manager with expertise in agile methodologies.</td>
</tr>
<tr>
<td>Michael Johnson</td>
<td>Data Analyst</td>
<td>Skilled data analyst proficient in statistical analysis and data visualization.</td>
</tr>
</table>
Edit Program.cs File
Inside the "Program.cs" file. add the following code. The code below uses the RenderAsync
method from the Razor.Templating.Core library to convert Razor Views to HTML. Secondly, it instantiates the ChromePdfRenderer class and passes the returned HTML string to the RenderHtmlAsPdf
method. Users can utilize RenderingOptions to access a range of features, such as adding custom text, including HTML headers and footers in the resulting PDF, defining custom margins, and applying page numbers.
app.MapGet("/PrintPdf", async () =>
{
IronPdf.License.LicenseKey = "IRONPDF-MYLICENSE-KEY-1EF01";
IronPdf.Logging.Logger.LoggingMode = IronPdf.Logging.Logger.LoggingModes.All;
string html = await RazorTemplateEngine.RenderAsync("Views/Home/Data.cshtml");
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf(html, "./wwwroot");
return Results.File(pdf.BinaryData, "application/pdf", "razorViewToPdf.pdf");
});
app.MapGet("/PrintPdf", async () =>
{
IronPdf.License.LicenseKey = "IRONPDF-MYLICENSE-KEY-1EF01";
IronPdf.Logging.Logger.LoggingMode = IronPdf.Logging.Logger.LoggingModes.All;
string html = await RazorTemplateEngine.RenderAsync("Views/Home/Data.cshtml");
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf(html, "./wwwroot");
return Results.File(pdf.BinaryData, "application/pdf", "razorViewToPdf.pdf");
});
app.MapGet("/PrintPdf", Async Function()
IronPdf.License.LicenseKey = "IRONPDF-MYLICENSE-KEY-1EF01"
IronPdf.Logging.Logger.LoggingMode = IronPdf.Logging.Logger.LoggingModes.All
Dim html As String = Await RazorTemplateEngine.RenderAsync("Views/Home/Data.cshtml")
Dim renderer As New ChromePdfRenderer()
Dim pdf As PdfDocument = renderer.RenderHtmlAsPdf(html, "./wwwroot")
Return Results.File(pdf.BinaryData, "application/pdf", "razorViewToPdf.pdf")
End Function)
Change The Asset Links
Navigate to the "Views" folder -> "Shared" folder -> "_Layout.cshtml" file. In the link tags, change the "~/" to "./".
This is important because the "~/" does not work well with IronPDF.
Run the Project
This will show you how to run the project and generate a PDF document.
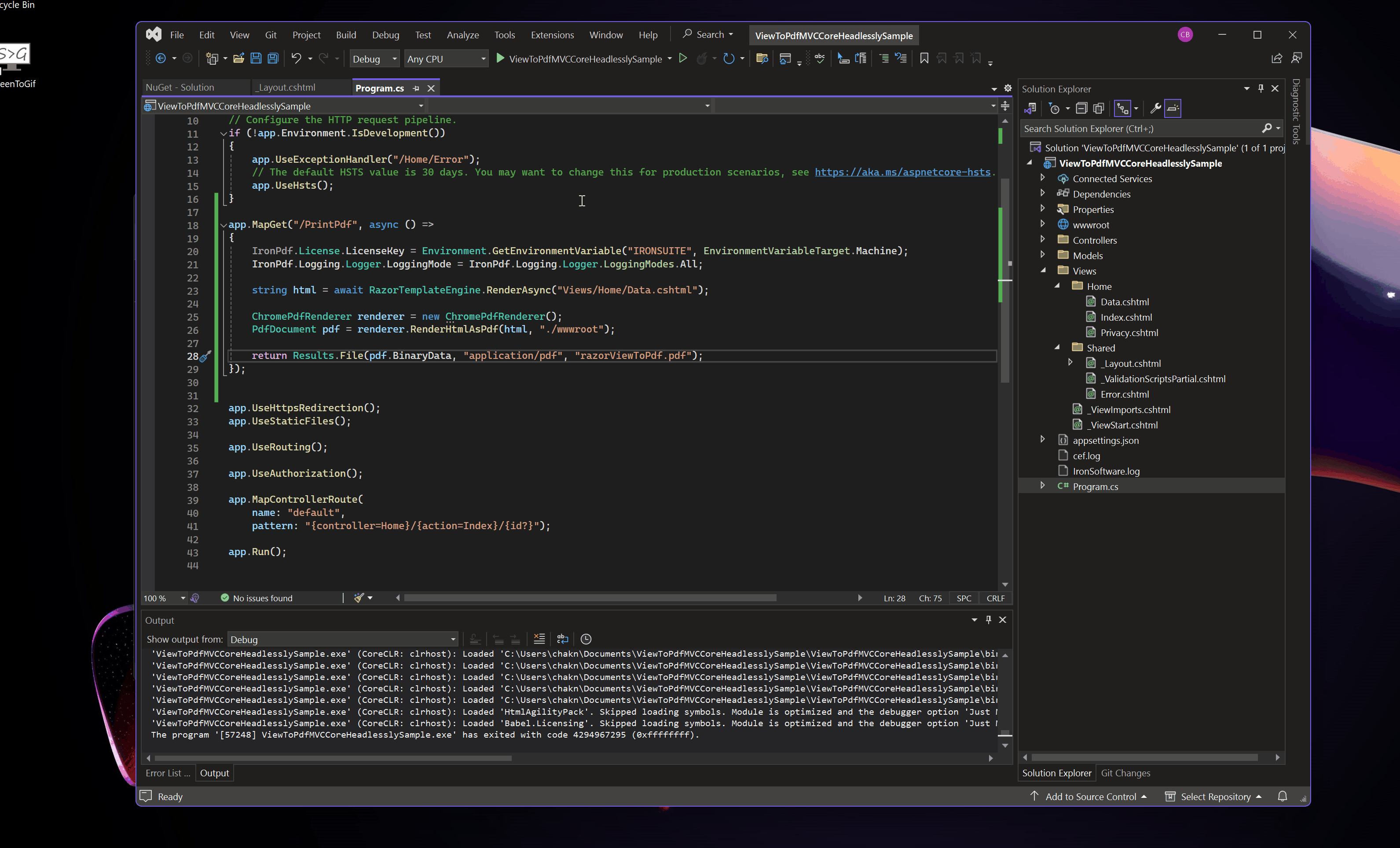
Output PDF
Download ASP.NET Core MVC Project
You can download the complete code for this guide. It comes as a zipped file that you can open in Visual Studio as a ASP.NET Core Web App (Model-View-Controller) project.