How to Convert Microsoft Word to PDF
A DOCX file is a document created in Microsoft Word, a word processing program by Microsoft. It uses the Office Open XML (OOXML) standard, making it efficient and compatible with various software. It's the default format for Word documents since Word 2007, replacing the older DOC format.
IronPDF allows you to convert DOCX documents into PDFs and provides a Mail Merge feature for generating personalized batches of documents for individual recipients. Converting from DOCX to PDF ensures universal compatibility, preserves formatting, and adds a layer of security.
How to Convert DOCX to PDF
- Download the C# library for converting DOCX to PDF
- Prepare the DOCX file you wish to convert
- Instantiate the DocxToPdfRenderer class to render PDF from DOCX file
- Use the
RenderDocxAsPdf
method and provide the DOCX filepath - Utilize the Mail Merge feature to generate a batch of documents
Install with NuGet
Install-Package IronPdf
Convert DOCX file to PDF Example
To convert a Microsoft Word file to PDF, instantiate the DocxToPdfRenderer class. Utilize the RenderDocxAsPdf
method of the DocxToPdfRenderer object by providing the filepath of the DOCX file. This method returns a PdfDocument object, allowing you to customize the PDF further. I've used the "Modern Chronological Resume" template from Microsoft Word as an example. You can download the sample DOCX file here.
Microsoft Word Preview
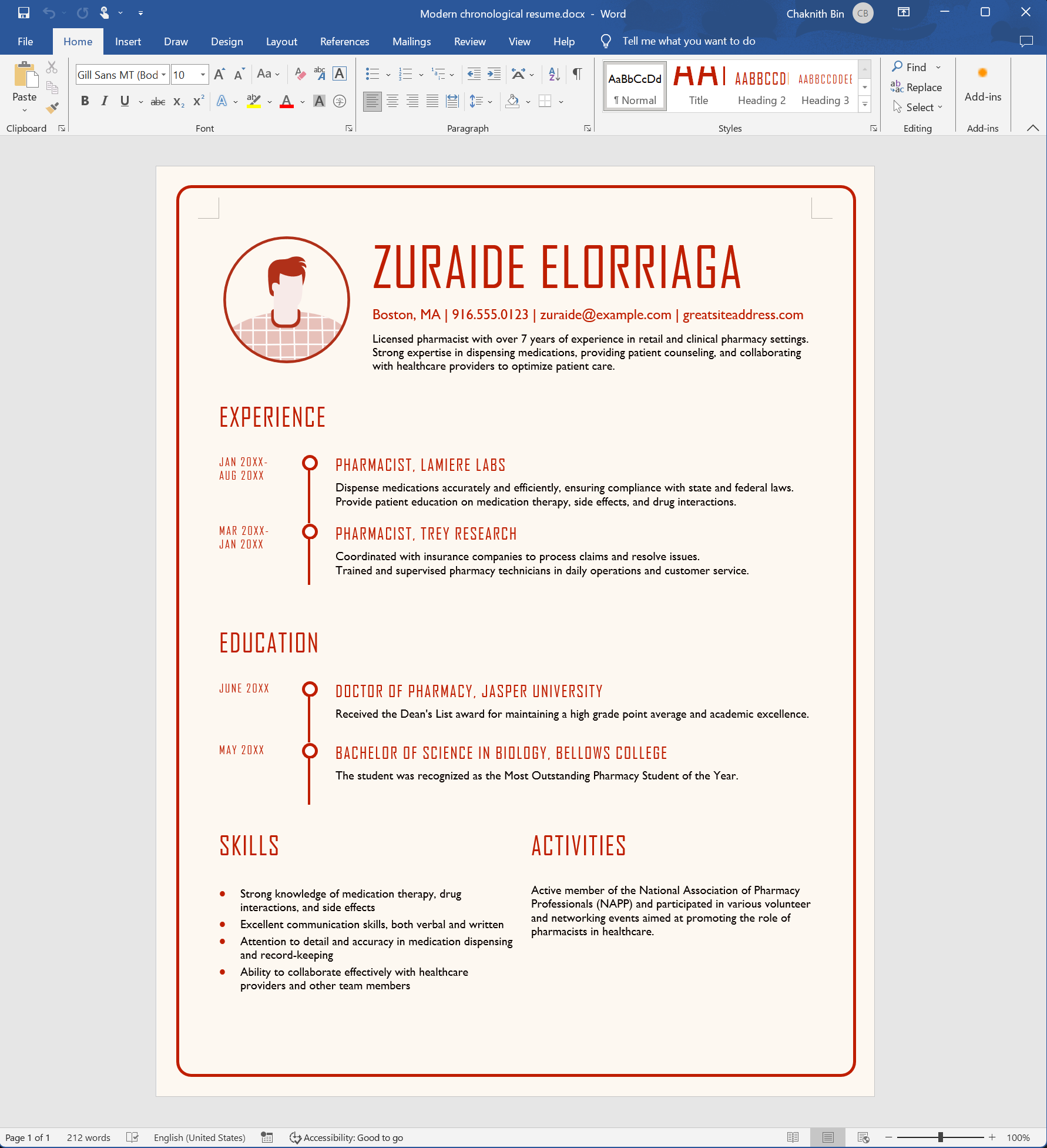
Code Sample
Additionally, the RenderDocxAsPdf
method also accepts DOCX data as bytes and streams.
:path=/static-assets/pdf/content-code-examples/how-to/docx-to-pdf-from-file.cs
using IronPdf;
// Instantiate Renderer
DocxToPdfRenderer renderer = new DocxToPdfRenderer();
// Render from DOCX file
PdfDocument pdf = renderer.RenderDocxAsPdf("Modern-chronological-resume.docx");
// Save the PDF
pdf.SaveAs("pdfFromDocx.pdf");
Imports IronPdf
' Instantiate Renderer
Private renderer As New DocxToPdfRenderer()
' Render from DOCX file
Private pdf As PdfDocument = renderer.RenderDocxAsPdf("Modern-chronological-resume.docx")
' Save the PDF
pdf.SaveAs("pdfFromDocx.pdf")
Output PDF
Mail Merge Example
Mail Merge, located on the "Mailings" tab in Microsoft Word, allows you to create a batch of documents with personalized information for each recipient or data entry. It's often used to generate personalized letters, envelopes, labels, or email messages, such as invitations, newsletters, or form letters, where much of the content is the same, but certain details vary for each recipient.
Model
First, let's create a model to store the information that will be mail-merged into its corresponding placeholder.
:path=/static-assets/pdf/content-code-examples/how-to/docx-to-pdf-mail-merge-model.cs
internal class RecipientsDataModel
{
public string Date { get; set; }
public string Location{ get; set; }
public string Recipients_Name { get; set; }
public string Contact_Us { get; set; }
}
Friend Class RecipientsDataModel
Public Property [Date]() As String
Public Property Location() As String
Public Property Recipients_Name() As String
Public Property Contact_Us() As String
End Class
I have modified a template provided by Microsoft Word for our purposes. Please download the sample DOTX file. For our use case, let's set the MailMergePrintAllInOnePdfDocument property to true, which combines the PDFs into a single PdfDocument object. The merge fields that we are going to use are Date, Location, Recipient's Name, and Contact Us.
Microsoft Word Preview
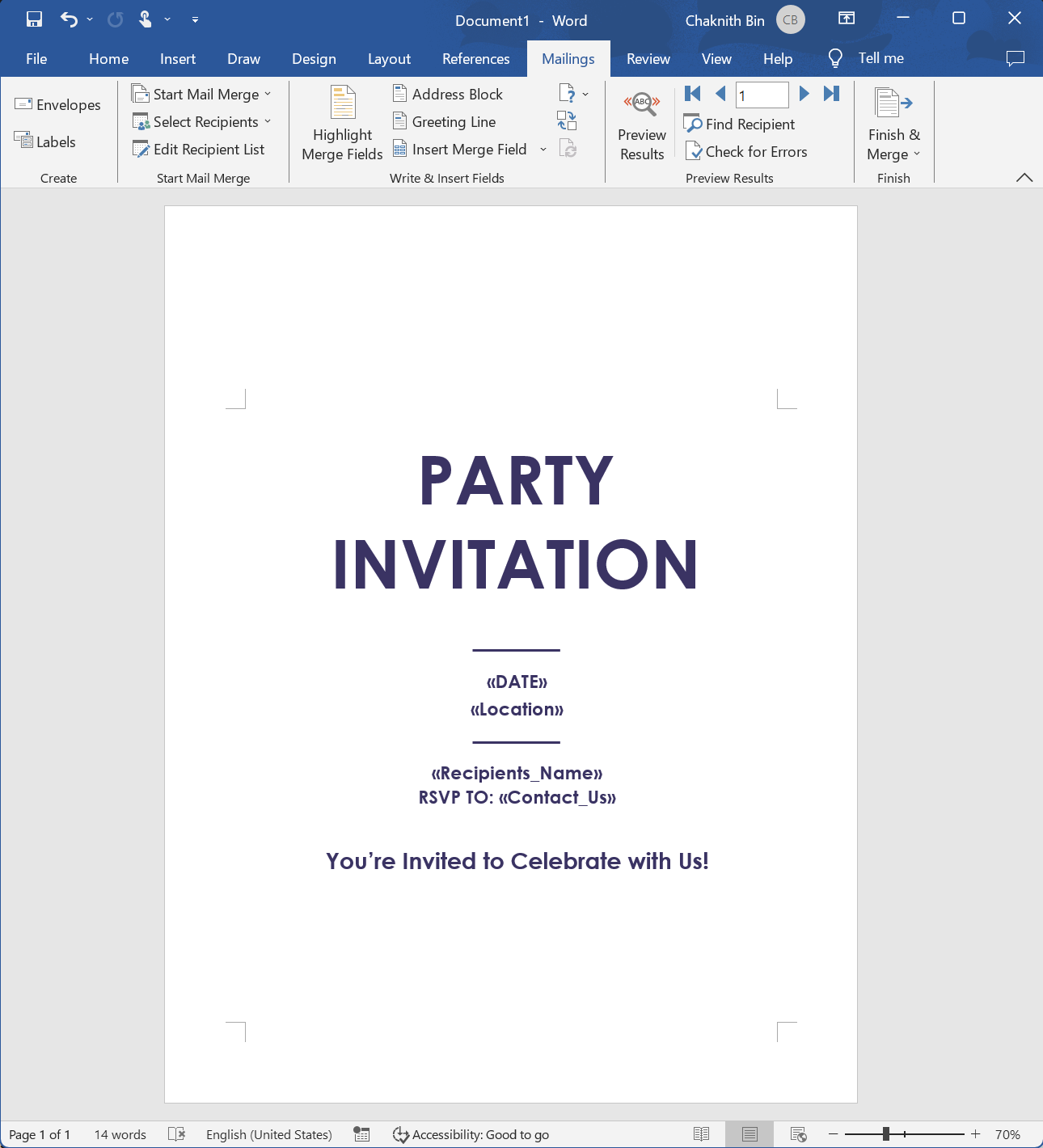
Code Sample
:path=/static-assets/pdf/content-code-examples/how-to/docx-to-pdf-mail-merge.cs
using IronPdf;
using System.Collections.Generic;
using System.Linq;
var recipients = new List<RecipientsDataModel>()
{
new RecipientsDataModel()
{
Date ="Saturday, October 15th, 2023",
Location="Iron Software Cafe, Chiang Mai",
Recipients_Name="Olivia Smith",
Contact_Us = "support@ironsoftware.com"
},
new RecipientsDataModel()
{
Date ="Saturday, October 15th, 2023",
Location="Iron Software Cafe, Chiang Mai",
Recipients_Name="Ethan Davis",
Contact_Us = "support@ironsoftware.com"
},
};
DocxToPdfRenderer docxToPdfRenderer = new DocxToPdfRenderer();
// Apply render options
DocxPdfRenderOptions options = new DocxPdfRenderOptions();
// Configure the output PDF to be combined into a single PDF document
options.MailMergePrintAllInOnePdfDocument = true;
// Convert DOTX to PDF
var pdfs = docxToPdfRenderer.RenderDocxMailMergeAsPdf<RecipientsDataModel>(
recipients,
"Party-invitation.dotx",
options);
pdfs.First().SaveAs("mailMerge.pdf");
Imports IronPdf
Imports System.Collections.Generic
Imports System.Linq
Private recipients = New List(Of RecipientsDataModel)() From {
New RecipientsDataModel() With {
.Date ="Saturday, October 15th, 2023",
.Location="Iron Software Cafe, Chiang Mai",
.Recipients_Name="Olivia Smith",
.Contact_Us = "support@ironsoftware.com"
},
New RecipientsDataModel() With {
.Date ="Saturday, October 15th, 2023",
.Location="Iron Software Cafe, Chiang Mai",
.Recipients_Name="Ethan Davis",
.Contact_Us = "support@ironsoftware.com"
}
}
Private docxToPdfRenderer As New DocxToPdfRenderer()
' Apply render options
Private options As New DocxPdfRenderOptions()
' Configure the output PDF to be combined into a single PDF document
options.MailMergePrintAllInOnePdfDocument = True
' Convert DOTX to PDF
Dim pdfs = docxToPdfRenderer.RenderDocxMailMergeAsPdf(Of RecipientsDataModel)(recipients, "Party-invitation.dotx", options)
pdfs.First().SaveAs("mailMerge.pdf")
Output PDF
Once the PDF document is created, you have the flexibility to make additional changes. These include exporting it as PDF/A or PDF/UA, as well as adding a digital certificate. You can also manipulate individual pages by merging, splitting, and rotating them, and you have the option to apply annotations and bookmarks.