Flatten PDF in C#
PDF documents often include forms featuring interactive fillable widgets, such as radio buttons, checkboxes, text boxes, lists, etc. In order to make it non-editable for different application purposes, we need to flatten the PDF file. IronPDF provides the function to flatten your PDF in C# with just one line of code.
How to Flatten PDF Files in C#
- Install C# library to flatten PDF files
- Load existing or create new PDF from HTML
- Use
Flatten
method to flatten the PDF - Save the flatten PDF as a new document
- Flatten PDF document in C# with 1 line of code
How to Flatten a C# PDF
Step 1
1. Install the IronPDF Software
First things first, let's install IronPDF, available free for development projects. Get it from:
Direct Download ZIP or through the NuGet format here.
Install-Package IronPdf
How to Tutorial
2. Flatten C# PDF Document
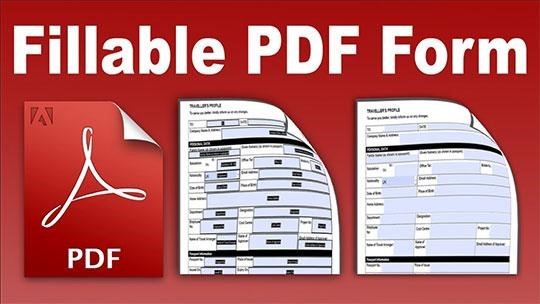
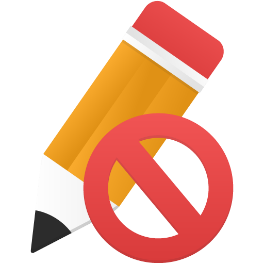
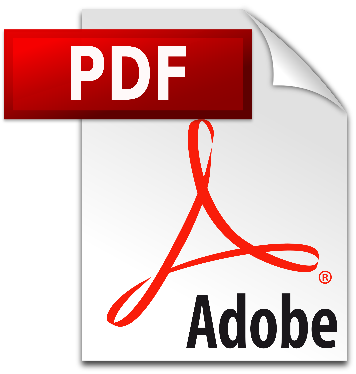
Once the IronPDF package is loaded in your Visual Studio project, you can use it to flatten your PDF file with just one line of code.
In the below code example, we have selected our own PDF using the “PDFDocument”
class. If your project calls for it, you can also create a PDF using “ChromePdfRenderer()”
method.
Then, to flatten a PDF file, the method is “Flatten()”
. This will make it uneditable using the interactive widgets that may have accompanied the file generation, such as radio buttons and checkboxes.
Let's see it in action in the flatten PDF C# code example below.
/**
Flatten PDF in C#
anchor-flatten-c-num-pdf-document
**/
using IronPdf;
using System.Windows.Forms;
namespace flattenpdf
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
//Select the Desired PDF File
using PdfDocument PDF = PdfDocument.FromFile("before.pdf");
//Flatten method compress the file & reduce the size of a pdf with same quality
PDF.Flatten();
//SaveAs the new Flattened.pdf
PDF.SaveAs("after_flatten.pdf");
}
}
}
/**
Flatten PDF in C#
anchor-flatten-c-num-pdf-document
**/
using IronPdf;
using System.Windows.Forms;
namespace flattenpdf
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
//Select the Desired PDF File
using PdfDocument PDF = PdfDocument.FromFile("before.pdf");
//Flatten method compress the file & reduce the size of a pdf with same quality
PDF.Flatten();
//SaveAs the new Flattened.pdf
PDF.SaveAs("after_flatten.pdf");
}
}
}
'''
'''Flatten PDF in C#
'''anchor-flatten-c-num-pdf-document
'''*
Imports IronPdf
Imports System.Windows.Forms
Namespace flattenpdf
Partial Public Class Form1
Inherits Form
Public Sub New()
InitializeComponent()
'Select the Desired PDF File
Using PDF As PdfDocument = PdfDocument.FromFile("before.pdf")
'Flatten method compress the file & reduce the size of a pdf with same quality
PDF.Flatten()
'SaveAs the new Flattened.pdf
PDF.SaveAs("after_flatten.pdf")
End Using
End Sub
End Class
End Namespace
3. Check the Flattened Document
In the below output, the first PDF is editable, our original file. Using IronPDF and the code above, we have made it flat or non-editable. You can use this code for any of your .NET PDF project needs.
Library Quick Access
Read More Documentation
Read the Documentation for more on how to flatten PDFs, edit and manipulate them, and more.
Read More Documentation