How to Merge or Split PDFs
Merging multiple PDF files into one can be highly useful in various scenarios. For instance, you can consolidate similar documents such as resumes into a single file instead of sharing multiple files. This article guides you through the process of merging multiple PDF files using C#. IronPDF simplifies PDF splitting and merging with intuitive method calls within your C# application. Below, we'll walk you through all the page manipulation functionalities.
How to Merge and Split PDF Pages in C#
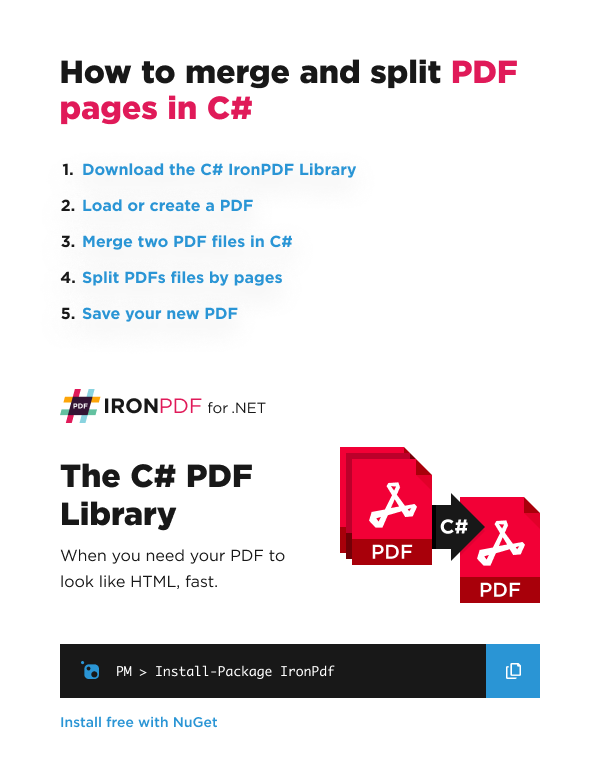
- Download C# library to merge and split PDF document
- Load existing or create a PDF from HTML string, file or URL
- Merge two PDF files in C# with
Merge
method - Split a PDF file by pages utilizing the
CopyPage
andCopyPages
methods - Save the PDF document to desired location
Install with NuGet
Install-Package IronPdf
Merge PDFs Example
In the following demonstration, we will initialize two two-paged HTML strings, render them as separate PDFs IronPDF, and then merge them:
:path=/static-assets/pdf/content-code-examples/how-to/merge-or-split-pdfs-merge.cs
using IronPdf;
// Two paged PDF
const string html_a =
@"<p> [PDF_A] </p>
<p> [PDF_A] 1st Page </p>
<div style = 'page-break-after: always;' ></div>
<p> [PDF_A] 2nd Page</p>";
// Two paged PDF
const string html_b =
@"<p> [PDF_B] </p>
<p> [PDF_B] 1st Page </p>
<div style = 'page-break-after: always;' ></div>
<p> [PDF_B] 2nd Page</p>";
var renderer = new ChromePdfRenderer();
var pdfdoc_a = renderer.RenderHtmlAsPdf(html_a);
var pdfdoc_b = renderer.RenderHtmlAsPdf(html_b);
// Four paged PDF
var merged = PdfDocument.Merge(pdfdoc_a, pdfdoc_b);
merged.SaveAs("Merged.pdf");
Imports IronPdf
' Two paged PDF
Private Const html_a As String = "<p> [PDF_A] </p>
<p> [PDF_A] 1st Page </p>
<div style = 'page-break-after: always;' ></div>
<p> [PDF_A] 2nd Page</p>"
' Two paged PDF
Private Const html_b As String = "<p> [PDF_B] </p>
<p> [PDF_B] 1st Page </p>
<div style = 'page-break-after: always;' ></div>
<p> [PDF_B] 2nd Page</p>"
Private renderer = New ChromePdfRenderer()
Private pdfdoc_a = renderer.RenderHtmlAsPdf(html_a)
Private pdfdoc_b = renderer.RenderHtmlAsPdf(html_b)
' Four paged PDF
Private merged = PdfDocument.Merge(pdfdoc_a, pdfdoc_b)
merged.SaveAs("Merged.pdf")
Result
This is the file that the code produced:
Split PDF Example
In the following demonstration, we will split the multi-pages PDF document from the previous example.
:path=/static-assets/pdf/content-code-examples/how-to/merge-or-split-pdfs-split.cs
using IronPdf;
// We will use the 4-page PDF from the Merge example above:
var pdf = PdfDocument.FromFile("Merged.pdf");
// Takes only the first page into a new PDF
var page1doc = pdf.CopyPage(0);
page1doc.SaveAs("Page1Only.pdf");
// Take the pages 2 & 3 (Note: index starts at 0)
var page23doc = pdf.CopyPages(1, 2);
page23doc.SaveAs("Pages2to3.pdf");
Imports IronPdf
' We will use the 4-page PDF from the Merge example above:
Private pdf = PdfDocument.FromFile("Merged.pdf")
' Takes only the first page into a new PDF
Private page1doc = pdf.CopyPage(0)
page1doc.SaveAs("Page1Only.pdf")
' Take the pages 2 & 3 (Note: index starts at 0)
Dim page23doc = pdf.CopyPages(1, 2)
page23doc.SaveAs("Pages2to3.pdf")
So this code saves two files:
- Page1Only.pdf (Only the first page)
- Pages2to3.pdf (Second to Third page)
Results
These are the two files produced: