How to Stamp Text & Image on PDFs
Stamping text and images on a PDF involves overlaying additional content onto an existing PDF document. This content, often referred to as a "stamp," can be text, images, or a combination of both. Stamps are typically used to add information, labels, watermarks, or annotations to a PDF.
There are a total of 5 stampers that can be used in IronPdf. This article is going to talk about TextStamper, ImageStamper, and HTMLStamper. HTMLStamper is particularly powerful as it can utilize all HTML features along with CSS styling.
How to Stamp Text & Image on PDFs
- Download the C# library to stamp text and image
- Create and configure the desired stamper class
- Use the
ApplyStamp
method to apply the stamp to the PDF - Apply multiple stamps using the
ApplyMultipleStamps
method - Specify particular pages to apply the stamps to
Install with NuGet
Install-Package IronPdf
Stamp Text Example
First, create an object from the TextStamper class. This object will contain all the configurations to specify how we want our text stamper to display. Pass the TextStamper object to the ApplyStamp
method. The Text property will be the displayed text. Furthermore, we can specify font family, font styling, as well as the location of the stamp.
:path=/static-assets/pdf/content-code-examples/how-to/stamp-text-image-stamp-text.cs
using IronPdf;
using IronPdf.Editing;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>");
// Create text stamper
TextStamper textStamper = new TextStamper()
{
Text = "Text Stamper!",
FontFamily = "Bungee Spice",
UseGoogleFont = true,
FontSize = 30,
IsBold = true,
IsItalic = true,
VerticalAlignment = VerticalAlignment.Top,
};
// Stamp the text stamper
pdf.ApplyStamp(textStamper);
pdf.SaveAs("stampText.pdf");
Imports IronPdf
Imports IronPdf.Editing
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>")
' Create text stamper
Private textStamper As New TextStamper() With {
.Text = "Text Stamper!",
.FontFamily = "Bungee Spice",
.UseGoogleFont = True,
.FontSize = 30,
.IsBold = True,
.IsItalic = True,
.VerticalAlignment = VerticalAlignment.Top
}
' Stamp the text stamper
pdf.ApplyStamp(textStamper)
pdf.SaveAs("stampText.pdf")
Output PDF
Stamp Image Example
Similar to the text stamper, we first create an object from the ImageStamper class and then use the ApplyStamp
method to apply the image to the document. The second parameter of this method also accepts a page index, which can be used to apply the stamp to a single or multiple pages. In the example below, we specify that the image should be stamped on page 1 of the PDF.
Tips
:path=/static-assets/pdf/content-code-examples/how-to/stamp-text-image-stamp-image.cs
using IronPdf;
using IronPdf.Editing;
using System;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>");
// Create image stamper
ImageStamper imageStamper = new ImageStamper(new Uri("https://ironpdf.com/img/svgs/iron-pdf-logo.svg"))
{
VerticalAlignment = VerticalAlignment.Top,
};
// Stamp the image stamper
pdf.ApplyStamp(imageStamper, 0);
pdf.SaveAs("stampImage.pdf");
Imports IronPdf
Imports IronPdf.Editing
Imports System
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>")
' Create image stamper
Private imageStamper As New ImageStamper(New Uri("https://ironpdf.com/img/svgs/iron-pdf-logo.svg")) With {.VerticalAlignment = VerticalAlignment.Top}
' Stamp the image stamper
pdf.ApplyStamp(imageStamper, 0)
pdf.SaveAs("stampImage.pdf")
Output PDF
Apply Multiple Stamps
Use the ApplyMultipleStamps
method to apply multiple stamps onto the document by passing an array of stampers to it.
:path=/static-assets/pdf/content-code-examples/how-to/stamp-text-image-multiple-stamps.cs
using IronPdf;
using IronPdf.Editing;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>");
// Create two text stampers
TextStamper stamper1 = new TextStamper()
{
Text = "Text stamp 1",
VerticalAlignment = VerticalAlignment.Top,
HorizontalAlignment = HorizontalAlignment.Left,
};
TextStamper stamper2 = new TextStamper()
{
Text = "Text stamp 2",
VerticalAlignment = VerticalAlignment.Top,
HorizontalAlignment = HorizontalAlignment.Right,
};
Stamper[] stampersToApply = { stamper1, stamper2 };
// Apply multiple stamps
pdf.ApplyMultipleStamps(stampersToApply);
pdf.SaveAs("multipleStamps.pdf");
Imports IronPdf
Imports IronPdf.Editing
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>")
' Create two text stampers
Private stamper1 As New TextStamper() With {
.Text = "Text stamp 1",
.VerticalAlignment = VerticalAlignment.Top,
.HorizontalAlignment = HorizontalAlignment.Left
}
Private stamper2 As New TextStamper() With {
.Text = "Text stamp 2",
.VerticalAlignment = VerticalAlignment.Top,
.HorizontalAlignment = HorizontalAlignment.Right
}
Private stampersToApply() As Stamper = { stamper1, stamper2 }
' Apply multiple stamps
pdf.ApplyMultipleStamps(stampersToApply)
pdf.SaveAs("multipleStamps.pdf")
Output PDF
Stamp location
To define the placement of the stamp, we utilize a 3x3 grid with three horizontal columns and three vertical rows. You have choices for horizontal alignment: left, center, and right, as well as vertical alignment: top, middle, and bottom. For added precision, you can adjust both horizontal and vertical offsets for each position. Please refer to the image below for a visual representation of this concept.
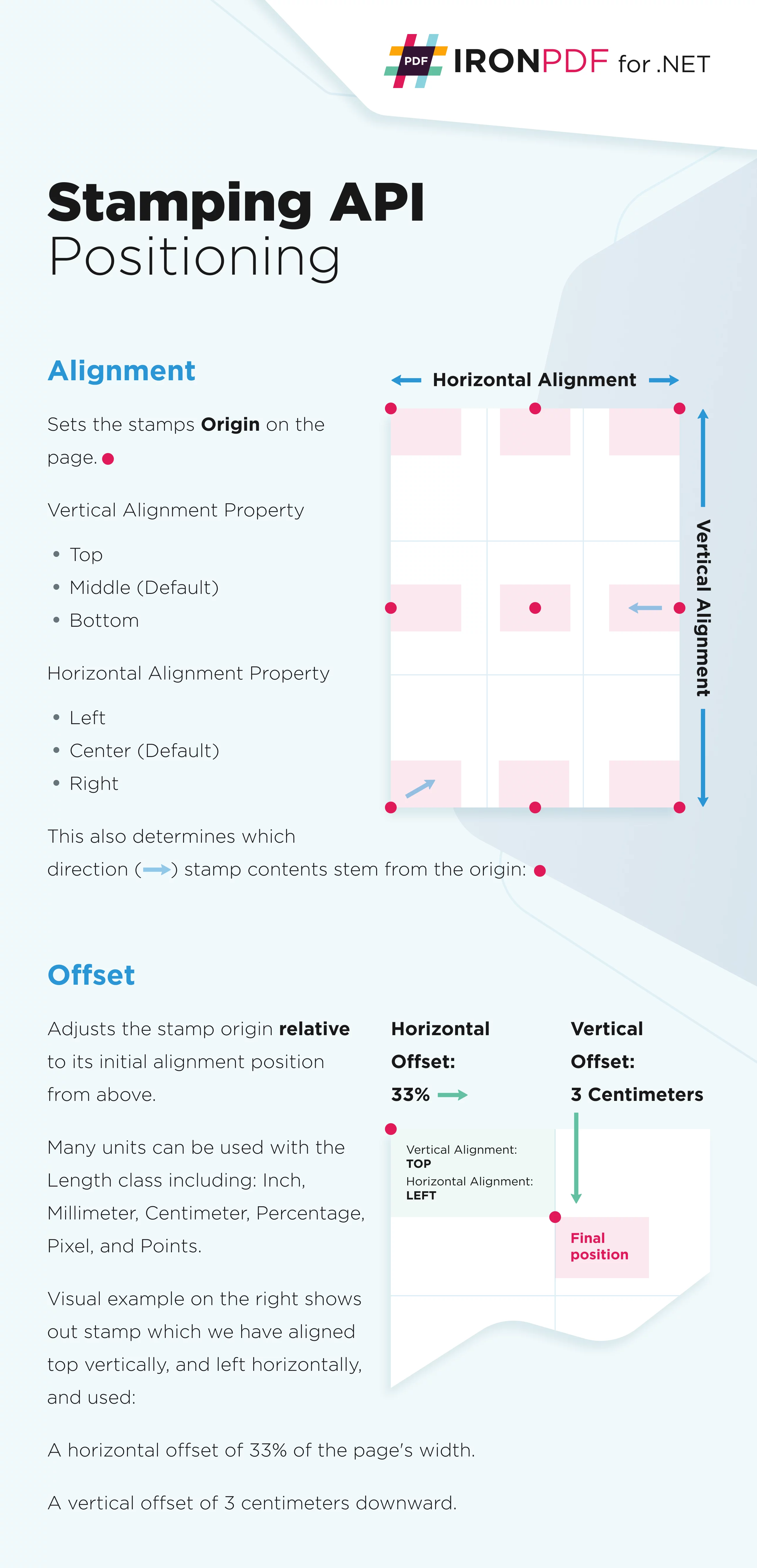
- HorizontalAlignment: The horizontal alignment of the stamp relative to the page.
- VerticalAlignment: The vertical alignment of the stamp relative to the page.
- HorizontalOffset: The horizontal offset. Default value is 0, and the default unit is IronPdf.Editing.MeasurementUnit.Percentage. Positive values indicate an offset to the right direction, while negative values indicate an offset to the left direction.
- VerticalOffset: The vertical offset. Default value is 0, and the default unit is IronPdf.Editing.MeasurementUnit.Percentage. Positive values indicate an offset in the downward direction, while negative values indicate an offset in the upward direction.
To specify the HorizontalOffset and VerticalOffset properties, we instantiate the Length class. The default measurement unit for Length is a percentage, but it is also capable of using measurement units such as inches, millimeters, centimeters, pixels, and points.
Code
:path=/static-assets/pdf/content-code-examples/how-to/stamp-text-image-stamp-location.cs
using IronPdf.Editing;
using System;
// Create text stamper
ImageStamper imageStamper = new ImageStamper(new Uri("https://ironpdf.com/img/svgs/iron-pdf-logo.svg"))
{
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Top,
// Specify offsets
HorizontalOffset = new Length(10),
VerticalOffset = new Length(10),
};
Imports IronPdf.Editing
Imports System
' Create text stamper
Private imageStamper As New ImageStamper(New Uri("https://ironpdf.com/img/svgs/iron-pdf-logo.svg")) With {
.HorizontalAlignment = HorizontalAlignment.Center,
.VerticalAlignment = VerticalAlignment.Top,
.HorizontalOffset = New Length(10),
.VerticalOffset = New Length(10)
}
Stamp HTML Example
There is another stamper class that we can use to stamp both text and images. The HtmlStamper class can be used to render HTML designs with CSS styling and then stamp them onto the PDF document. The InnerHtmlBaseUrl property is used to specify the base URL for the HTML string assets, such as CSS and image files.
Code
:path=/static-assets/pdf/content-code-examples/how-to/stamp-text-image-stamp-html.cs
using IronPdf;
using IronPdf.Editing;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>");
// Create HTML stamper
HtmlStamper htmlStamper = new HtmlStamper()
{
Html = @"<img src='https://ironpdf.com/img/svgs/iron-pdf-logo.svg'>
<h1>Iron Software</h1>",
VerticalAlignment = VerticalAlignment.Top,
};
// Stamp the HTML stamper
pdf.ApplyStamp(htmlStamper);
pdf.SaveAs("stampHtml.pdf");
Imports IronPdf
Imports IronPdf.Editing
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>")
' Create HTML stamper
Private htmlStamper As New HtmlStamper() With {
.Html = "<img src='https://ironpdf.com/img/svgs/iron-pdf-logo.svg'>
<h1>Iron Software</h1>",
.VerticalAlignment = VerticalAlignment.Top
}
' Stamp the HTML stamper
pdf.ApplyStamp(htmlStamper)
pdf.SaveAs("stampHtml.pdf")
- Html: The HTML fragment to be stamped onto your PDF. All external references to JavaScript, CSS, and image files will be relative to IronPdf.Editing.Stamper.InnerHtmlBaseUrl.
- InnerHtmlBaseUrl: The HTML base URL for which references to external CSS, Javascript, and Image files will be relative.
Explore Stamper Options
In addition to the options mentioned and explained above, below are more options available to the stamper classes.
- Opacity: Allows the stamp to be transparent. 0 is fully invisible, 100 is fully opaque.
- Rotation: Rotates the stamp clockwise from 0 to 360 degrees as specified.
- MaxWidth: The maximum width of the output stamp.
- MaxHeight: The maximum height of the output stamp.
- MinWidth: The minimum width of the output stamp.
- MinHeight: The minimum height of the output stamp.
- Hyperlink: Makes stamped elements of this Stamper have an on-click hyperlink. Note: HTML links created by link(a) tags are not reserved by stamping.
- Scale: Applies a percentage scale to the stamps to make them larger or smaller. Default is 100 (Percent), which has no effect.
- IsStampBehindContent: Set to true to apply the stamp behind the content. If the content is opaque, the stamp may be invisible.
- WaitFor: A convenient wrapper to wait for various events or just wait for an amount of time.
- Timeout: Render timeout in seconds. The default value is 60.