How to Convert Razor to PDF in Blazor Server
A Razor component is a user interface element, such as a page, dialog, or data entry form, built using C# and Razor syntax. It serves as a reusable piece of UI.
Blazor Server is a web framework that allows you to build interactive web UIs using C# instead of JavaScript. In this framework, the logic for components runs on the server.
IronPDF enables you to generate PDF documents from Razor components in a Blazor Server project or application. This makes the creation of PDF files/pages straightforward in Blazor Server.
How to Convert Razor Components to PDF
IronPDF Extension Package
The IronPdf.Extensions.Blazor package is an extension of the main IronPdf package. Both the IronPdf.Extensions.Blazor and IronPdf packages are needed to render Razor components to PDF documents in a Blazor Server App.
PM > Install-Package IronPdf.Extensions.Blazor
Install with NuGet
Install-Package IronPdf.Extensions.Blazor
Render Razor Components to PDFs
A Blazor Server App project is required to convert Razor components to PDFs.
Add a Model Class
Add a standard C# class and name it PersonInfo. This class will serve as the model for storing person information. Insert the following code:
:path=/static-assets/pdf/content-code-examples/how-to/razor-to-pdf-blazor-server-model.cs
namespace BlazorSample.Data
{
public class PersonInfo
{
public int Id { get; set; }
public string Name { get; set; }
public string Title { get; set; }
public string Description { get; set; }
}
}
Namespace BlazorSample.Data
Public Class PersonInfo
Public Property Id() As Integer
Public Property Name() As String
Public Property Title() As String
Public Property Description() As String
End Class
End Namespace
Add a Razor Component
Use the RenderRazorComponentToPdf
method to convert Razor components into PDFs. Access this method by instantiating the ChromePdfRenderer class. The method returns a PdfDocument object, which allows you to either export the PDF document or modify it further.
The returned PdfDocument can undergo additional modifications, such as conversion to PDF/A or PDF/UA formats. You can also merge or split the PDF document, rotate its pages, and add annotations or bookmarks. Custom watermarks can also be stamped onto your PDF.
Add a Razor component and name it Person. Input the following code:
@page "/Person"
@using BlazorSample.Data;
@using IronPdf;
@using IronPdf.Extensions.Blazor;
<h3>Person</h3>
@code {
[Parameter]
public IEnumerable<PersonInfo> persons { get; set; }
public Dictionary<string, object> Parameters { get; set; } = new Dictionary<string, object>();
protected override async Task OnInitializedAsync()
{
persons = new List<PersonInfo>
{
new PersonInfo { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new PersonInfo { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new PersonInfo { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
}
private async void PrintToPdf()
{
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Apply text footer
renderer.RenderingOptions.TextFooter = new TextHeaderFooter()
{
LeftText = "{date} - {time}",
DrawDividerLine = true,
RightText = "Page {page} of {total-pages}",
Font = IronSoftware.Drawing.FontTypes.Arial,
FontSize = 11
};
Parameters.Add("persons", persons);
// Render razor component to PDF
PdfDocument pdf = renderer.RenderRazorComponentToPdf<Person>(Parameters);
File.WriteAllBytes("razorComponentToPdf.pdf", pdf.BinaryData);
}
}
<table class="table">
<tr>
<th>Name</th>
<th>Title</th>
<th>Description</th>
</tr>
@foreach (var person in persons)
{
<tr>
<td>@person.Name</td>
<td>@person.Title</td>
<td>@person.Description</td>
</tr>
}
</table>
<button class="btn btn-primary" @onclick="PrintToPdf">Print to Pdf</button>
@page "/Person"
@using BlazorSample.Data;
@using IronPdf;
@using IronPdf.Extensions.Blazor;
<h3>Person</h3>
@code {
[Parameter]
public IEnumerable<PersonInfo> persons { get; set; }
public Dictionary<string, object> Parameters { get; set; } = new Dictionary<string, object>();
protected override async Task OnInitializedAsync()
{
persons = new List<PersonInfo>
{
new PersonInfo { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new PersonInfo { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new PersonInfo { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
}
private async void PrintToPdf()
{
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Apply text footer
renderer.RenderingOptions.TextFooter = new TextHeaderFooter()
{
LeftText = "{date} - {time}",
DrawDividerLine = true,
RightText = "Page {page} of {total-pages}",
Font = IronSoftware.Drawing.FontTypes.Arial,
FontSize = 11
};
Parameters.Add("persons", persons);
// Render razor component to PDF
PdfDocument pdf = renderer.RenderRazorComponentToPdf<Person>(Parameters);
File.WriteAllBytes("razorComponentToPdf.pdf", pdf.BinaryData);
}
}
<table class="table">
<tr>
<th>Name</th>
<th>Title</th>
<th>Description</th>
</tr>
@foreach (var person in persons)
{
<tr>
<td>@person.Name</td>
<td>@person.Title</td>
<td>@person.Description</td>
</tr>
}
</table>
<button class="btn btn-primary" @onclick="PrintToPdf">Print to Pdf</button>
Private page "/Person" [using] BlazorSample.Data
Private IronPdf As [using]
Private IronPdf As [using]
'INSTANT VB TODO TASK: The following line could not be converted:
(Of h3) Person</h3> code
If True Then
<Parameter>
public IEnumerable(Of PersonInfo) persons {get;set;}
public Dictionary(Of String, Object) Parameters {get;set;} = New Dictionary(Of String, Object)()
'INSTANT VB TODO TASK: Local functions are not converted by Instant VB:
' protected override async Task OnInitializedAsync()
' {
' persons = New List<PersonInfo> { New PersonInfo { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" }, New PersonInfo { Name = "Bob", Title = "Mr.", Description = "Software Engineer" }, New PersonInfo { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" } };
' }
'INSTANT VB TODO TASK: Local functions are not converted by Instant VB:
' private async void PrintToPdf()
' {
' ChromePdfRenderer renderer = New ChromePdfRenderer();
'
' ' Apply text footer
' renderer.RenderingOptions.TextFooter = New TextHeaderFooter() { LeftText = "{date} - {time}", DrawDividerLine = True, RightText = "Page {page} of {total-pages}", Font = IronSoftware.Drawing.FontTypes.Arial, FontSize = 11 };
'
' Parameters.Add("persons", persons);
'
' ' Render razor component to PDF
' PdfDocument pdf = renderer.RenderRazorComponentToPdf<Person>(Parameters);
'
' File.WriteAllBytes("razorComponentToPdf.pdf", pdf.BinaryData);
' }
End If
<table class="table"> (Of tr) (Of th) Name</th> (Of th) Title</th> (Of th) Description</th> </tr> foreach(var person in persons)
If True Then
(Of tr) (Of td) person.Name</td> (Of td) person.Title</td> (Of td) person.Description</td> </tr>
End If
'INSTANT VB TODO TASK: The following line uses invalid syntax:
'</table> <button class="btn btn-primary" @onclick="PrintToPdf"> Print @to Pdf</button>
Moreover, using this method to generate a PDF grants you complete access to all feature in RenderingOptions. This includes the ability to insert text, as well as HTML headers and footers. Additionally, you can add page numbers and adjust the page dimensions and layout to your liking.
Add a Section to the Left Menu
- Navigate to the "Shared folder" and open NavMenu.razor.
- Add the section that will open our Razor component, Person. Our Person component will be the second option.
<div class="@NavMenuCssClass" @onclick="ToggleNavMenu">
<nav class="flex-column">
<div class="nav-item px-3">
<NavLink class="nav-link" href="" Match="NavLinkMatch.All">
<span class="oi oi-home" aria-hidden="true"></span> Home
</NavLink>
</div>
<div class="nav-item px-3">
<NavLink class="nav-link" href="Person">
<span class="oi oi-list-rich" aria-hidden="true"></span> Person
</NavLink>
</div>
<div class="nav-item px-3">
<NavLink class="nav-link" href="counter">
<span class="oi oi-plus" aria-hidden="true"></span> Counter
</NavLink>
</div>
<div class="nav-item px-3">
<NavLink class="nav-link" href="fetchdata">
<span class="oi oi-list-rich" aria-hidden="true"></span> Fetch data
</NavLink>
</div>
</nav>
</div>
<div class="@NavMenuCssClass" @onclick="ToggleNavMenu">
<nav class="flex-column">
<div class="nav-item px-3">
<NavLink class="nav-link" href="" Match="NavLinkMatch.All">
<span class="oi oi-home" aria-hidden="true"></span> Home
</NavLink>
</div>
<div class="nav-item px-3">
<NavLink class="nav-link" href="Person">
<span class="oi oi-list-rich" aria-hidden="true"></span> Person
</NavLink>
</div>
<div class="nav-item px-3">
<NavLink class="nav-link" href="counter">
<span class="oi oi-plus" aria-hidden="true"></span> Counter
</NavLink>
</div>
<div class="nav-item px-3">
<NavLink class="nav-link" href="fetchdata">
<span class="oi oi-list-rich" aria-hidden="true"></span> Fetch data
</NavLink>
</div>
</nav>
</div>
Run the Project
This will show you how to run the project and generate a PDF document.
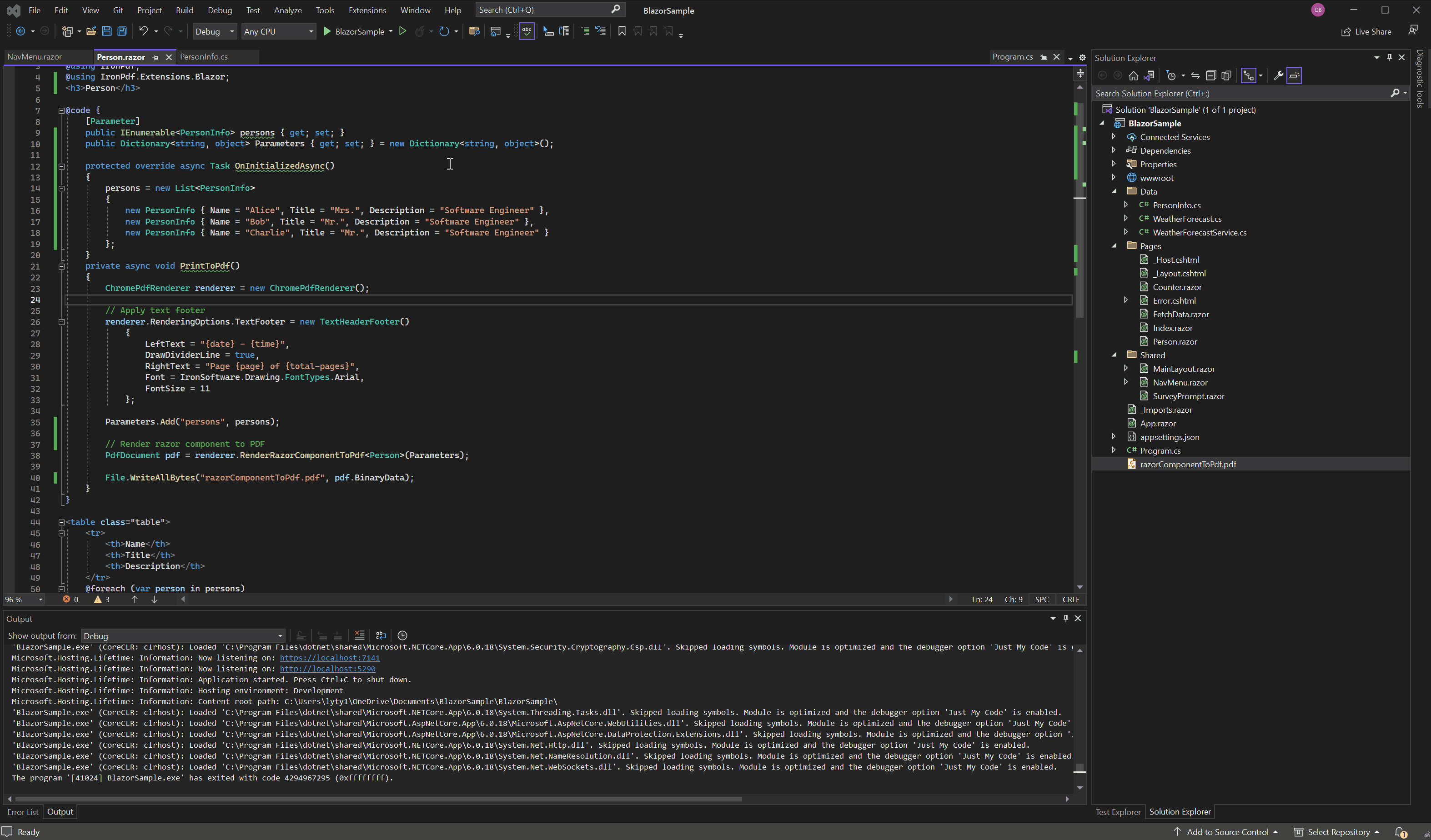
Download Blazor Server App Project
You can download the complete code for this guide. It comes as a zipped file that you can open in Visual Studio as a Blazor Server App project.