Published February 24, 2023
How to Password Protect PDF in Java
Portable Document Format known as PDF, is the most popular digital data transferring document format used across the globe. Since the introduction of PDF files, it has been the key format for saving and retrieving sensitive data without any loss in formatting. It transfers data securely and efficiently.
One of the important features of PDF is that it can be password protected using encryption key. This means only authorized users can open the file and view its contents. This enhances security and protect the rights of owners as well.
Java is a popular programming language which is platform independent. It is used to build applications which can be executed on different platforms without any problem. This feature of Java is pretty similar to PDF as it is also platform independent. With the need to store data in secure and formatted form, programs developed in Java also require to save data and PDF is a great option.
Here, we will use IronPDF to work with PDF documents and also protect new files with user password.
IronPDF - Java PDF Library
IronPDF is a Java library for working with PDF documents. It provides a wide range of features for generating and manipulating PDFs, including the ability to add text, images, and other types of content, and control the layout and formatting of the document. It also provides a number of important features for securing PDF content, such as password protection.
Steps to Protect PDF using Password in Java Applications
Prerequisites for Project Setup
To use IronPDF to work with PDFs in a Java Maven project, you will need to make sure that you have the following prerequisites:
- Java Development Kit (JDK): A running current version of Java must be installed on your computer. If you don't have JAR files, then download the latest JDK from the Oracle website.
- Maven: Maven is an important build automation tool for Java projects which is required to manage the project and its dependencies. Download Maven or JAR file from the Apache Maven website if you don't have it installed.
IronPDF Java Library: You will also require the IronPDF Java library which will be added to your Maven project as a dependency. This can be done by adding the following dependency to your project's pom.xml file. Maven will automatically download and install it in the project.
<dependency>
<groupId>com.ironsoftware</groupId>
<artifactId>com.ironsoftware</artifactId>
<version>2024.3.1</version>
</dependency>XMLAnother dependency required is Slf4j. Add Slf4j dependency in the pom.xml file.
<dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-simple</artifactId> <version>2.0.3</version> </dependency>
XML
Once you have downloaded and installed your password protect PDF in Java program, you are ready to use IronPDF to protect PDF file with password protection.
Important Steps Before Writing Code
First of all, we need to import the IronPDF required classes in Java code. Add the following code at the top of "Main.java" file:
import com.ironsoftware.ironpdf.PdfDocument;
import com.ironsoftware.ironpdf.metadata.MetadataManager;
import com.ironsoftware.ironpdf.security.PdfPrintSecurity;
import com.ironsoftware.ironpdf.security.SecurityManager;
import com.ironsoftware.ironpdf.security.SecurityOptions;
import java.io.IOException;
import java.nio.file.Paths;
import java.util.Date;
Now, in the main method, enter your license key using the IronPDF setLicenseKey
method.
License.setLicenseKey("Your license key");
Open an Encrypted PDF Document
The following code snippet will open an document that was encrypted with the password "password":
PdfDocument pdf = PdfDocument.fromFile(Paths.get("encrypted.pdf", "secretPassword"));
In the above code snippet, I opened an encrypted file and PDF file password was "password".
Encrypted PDF document looks like the following:
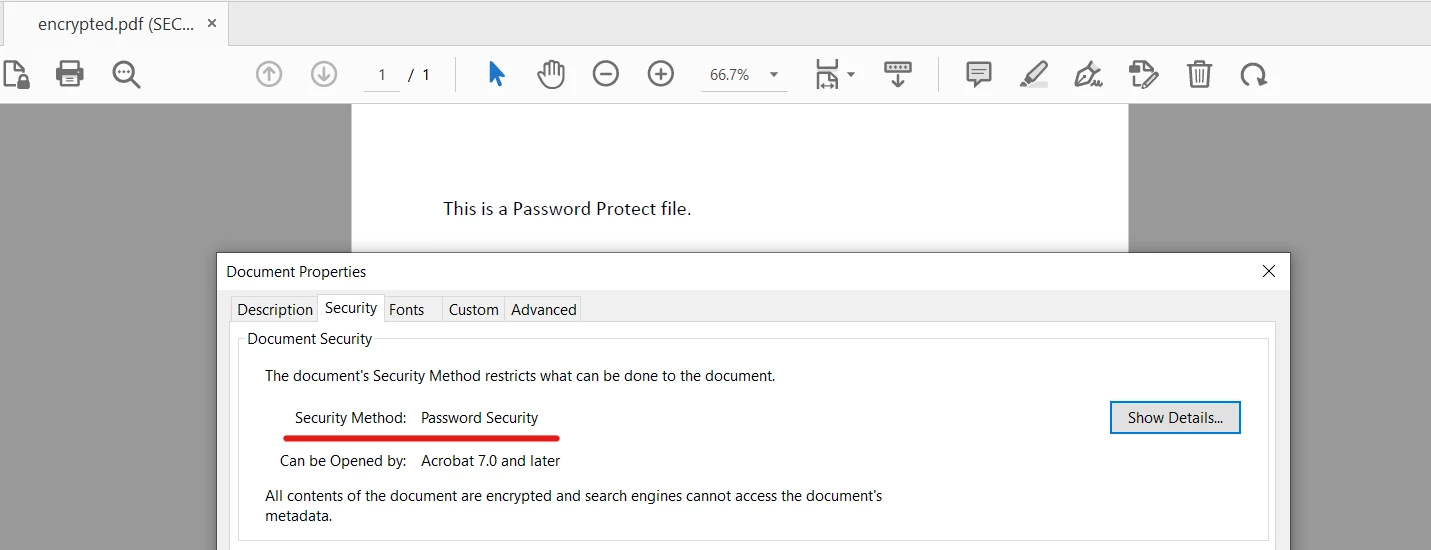
Opening an PDF Document that has been encrypted with a password
Encrypt PDF Document using Password Protection
Let's change the owner password of "encrypted.pdf" file which we opened in previous step. The following code helps to achieve this task:
// Change or set the document owner password
SecurityManager securityManager = pdf.getSecurity();
securityManager.removePasswordsAndEncryption();
securityManager.setPassword("secret-key");
We first remove the password using removePasswordsAndEncryption
method, and then we set a new password using the setPassword
method.
Save Password Protected PDF Documents
Finally, save the PDF document with the following line of code:
pdf.saveAs(Paths.get("assets/secured.pdf"));
The output file is now opened with "secret-key" password.
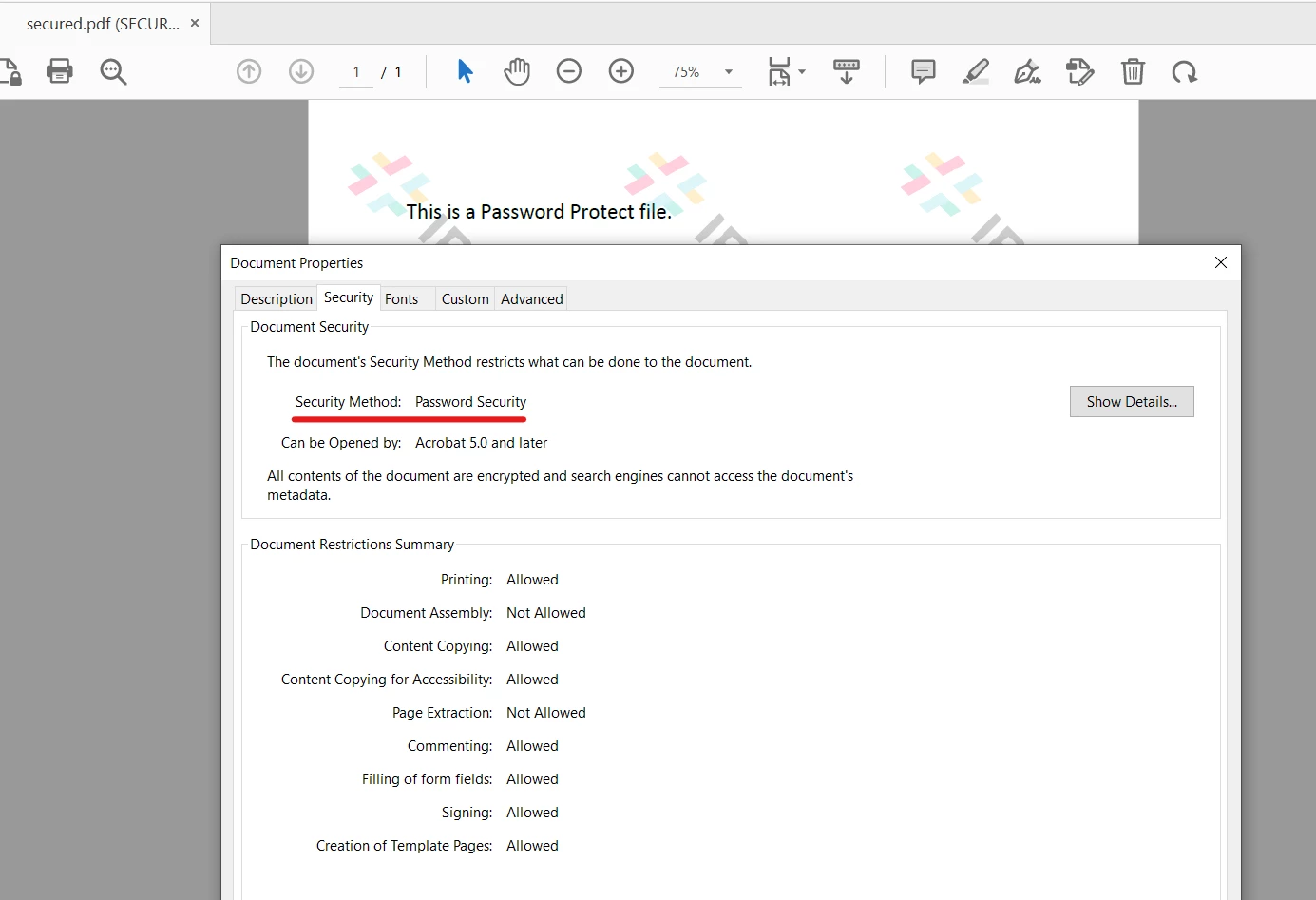
The PDF document from the previous step has been re-encrypted with a new password.
Edit File Security Settings
Important security options can be set easily with IronPDF in Java using the SecurityOptions
permission class. The code below makes the PDF read-only and disallows users to copy, paste, and print, and set passwords for owner and user.
SecurityOptions securityOptions = new SecurityOptions();
securityOptions.setAllowUserCopyPasteContent(false);
securityOptions.setAllowUserAnnotations(false);
securityOptions.setAllowUserPrinting(PdfPrintSecurity.NO_PRINT);
securityOptions.setAllowUserFormData(false);
SecurityManager securityManager = pdf.getSecurity();
securityManager.setSecurityOptions(securityOptions);
This will set all the necessary security options of the PDF document. This can be seen in the output below:
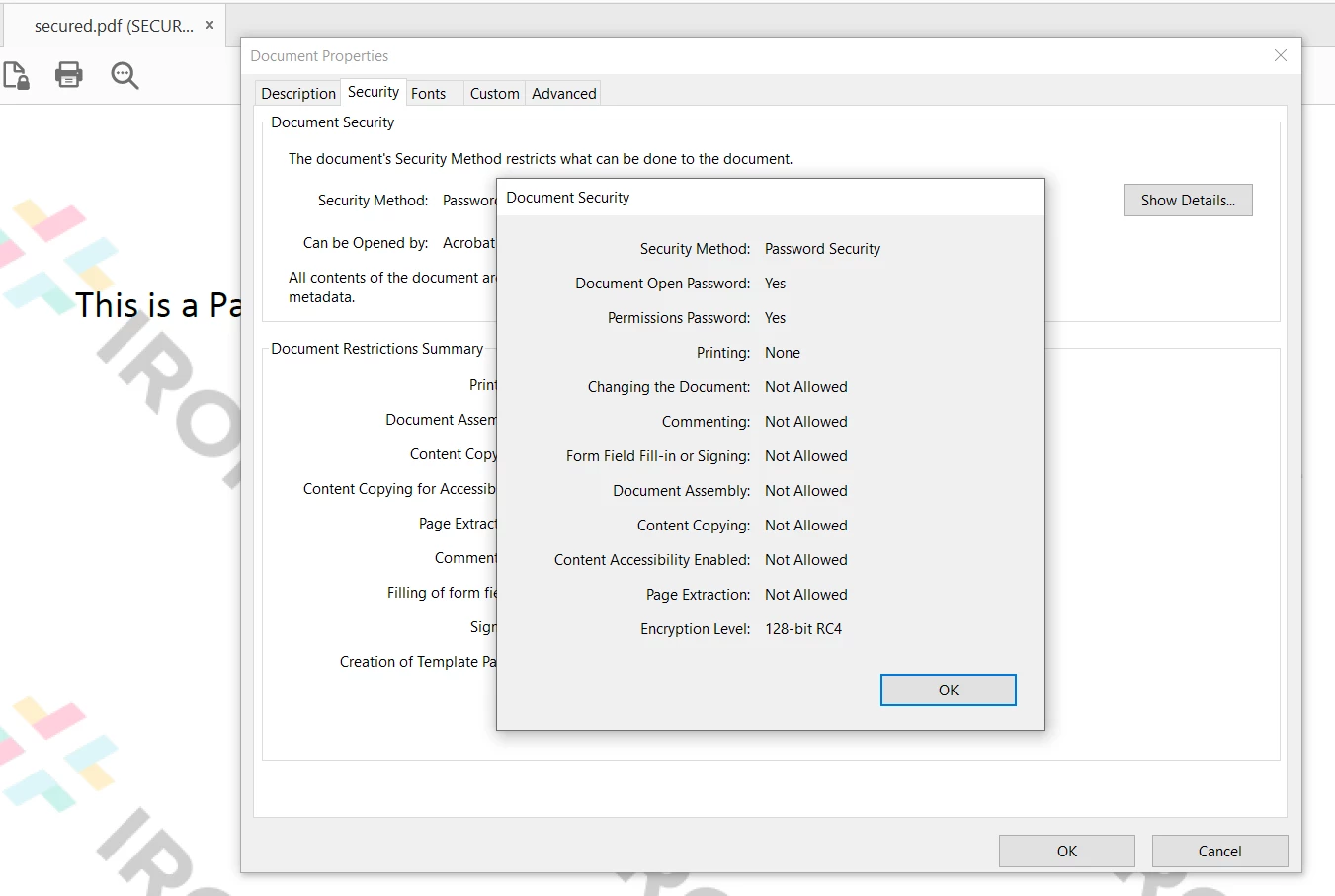
Here, we see that the options we specified using the SecurityOptions
class have been applied successfully to our PDF.
Summary
In this article, we looked at how Java opens an existing PDF document and add password protection using IronPDF Library. IronPDF makes a lot easier to work with PDF files in Java. Whether you want to create new document or make a PDF viewer, IronPDF helps to achieve this task with a single line of code. IronPDF's Engine is well suited for Java programming language, as it is fast and memory efficient. With IronPDF, you can set user password along with the owner password. It provides full protection options along with other features like converting to PDF from other formats and splitting and merging documents.
IronPDF can be used for free in a free trial and can be licensed for commercial use. Its lite package starts from $749. Download download IronPDFand give a try.