Test in a live environment
Test in production without watermarks.
Works wherever you need it to.
Portable Document Format (PDF) is a widely used file format for sharing and presenting documents. Unlike paper documents, data can be saved exactly the same way it was created. However, not all PDFs are created equal when it comes to long-term preservation and accessibility.
To ensure that your PDF documents are archived and accessed reliably over time, you can convert them to the PDF/A (PDF/A-1a or PDF/A-1b) format. PDF/A is an ISO-standardized version of PDF designed for the digital preservation of electronic documents.
In this article, we will explore how to convert PDF files to PDF/A format in Java using IronPDF.
PdfDocument.renderHtmlAsPdf
PdfDocument.fromFile
convertToPdfA
methodsaveAsPdfA
IronPDF is a powerful and versatile library for working with PDFs in Java. It allows you to create, manipulate, and convert PDF documents with ease. One of its key features is the ability to convert regular PDFs to the PDF/A format, ensuring long-term document preservation. IronPDF provides a simple and efficient way to perform this conversion within your Java applications.
Before we get started, make sure you have the following prerequisites in place:
Click on "New Project" or from "File" > "New" > "Project".
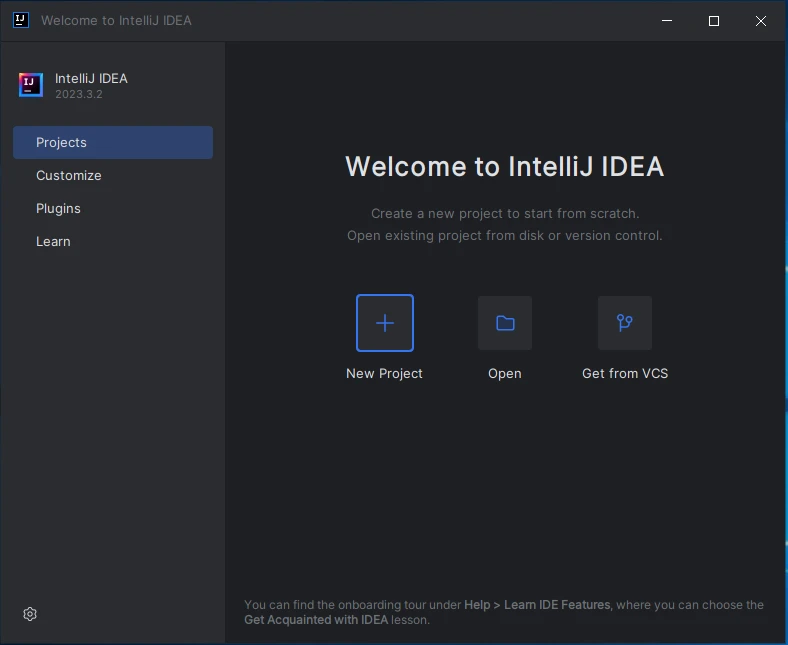
Choose your project's JDK (Java version).
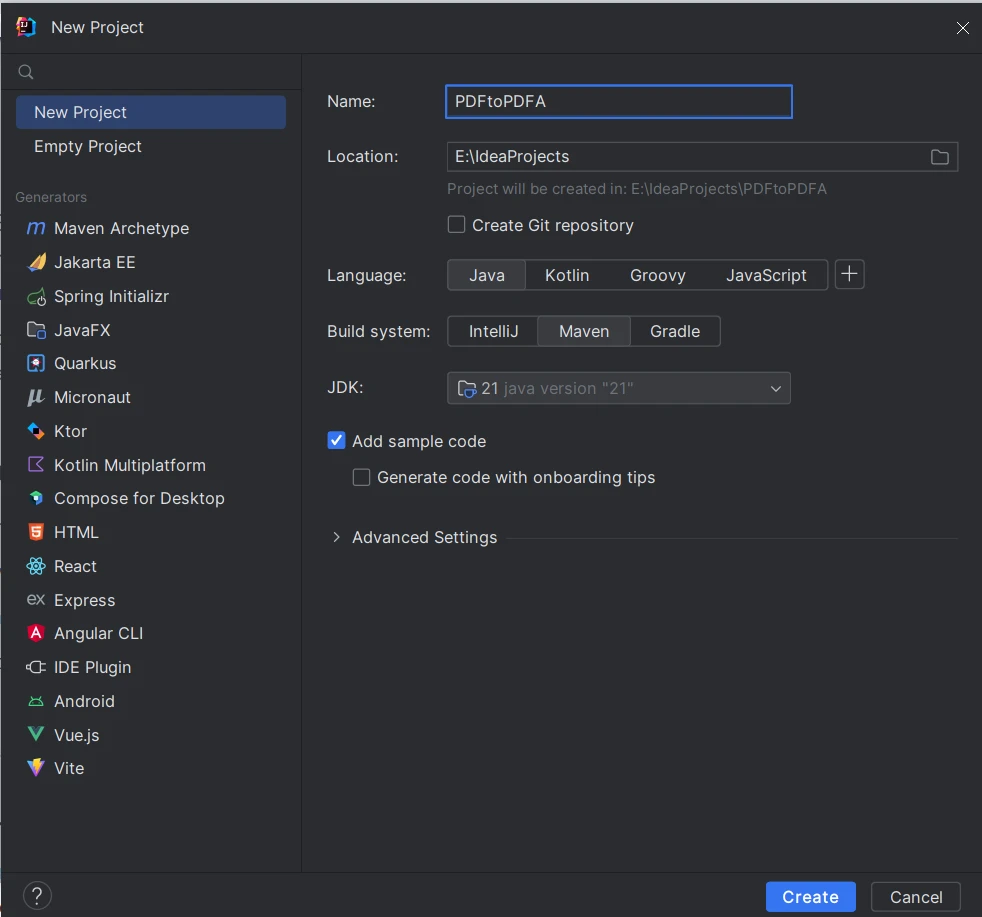
To include IronPDF in your project, you need to import jar dependency in your project's pom.xml file. Open the pom.xml file and add the following dependency:
```shell
:ProductInstall
```
Save the POM XML file, and Maven will automatically download and include the IronPDF library for Java in your project.
There is also another jar dependency called Slf4j-simple, that needs to be added to work correctly with IronPDF.
```xml
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-simple</artifactId>
<version>2.0.9</version>
</dependency>
```
Reload the Maven project to update the dependencies added to the POM file. Once installed, we are ready to use IronPDF to convert PDF files to PDF/A.
Now that we have set up your project and added the IronPDF dependency, we can start converting PDFs to PDF/A within our Java application. Here are the steps that demonstrate how to convert PDF to PDF/A:
IronPDF provides the facility to create a PDF file from an HTML String, file, or URL. It provides RenderHtmlAsPdf, RenderHtmlFileAsPdf, and RenderUrlAsPdf methods respectively to create a PDF format designed using HTML.
```java
PdfDocument pdfDocument = PdfDocument.renderHtmlAsPdf("<h1> ~Hello World~ </h1> Made with IronPDF!");
```
Alternatively, we can load the existing PDF document that we want to convert to PDF/A using IronPDF.
```java
PdfDocument pdfDocument = PdfDocument.fromFile(Paths.get("input.pdf"));
```
The input file looks like this:
Use IronPDF convertToPdfA
method to convert the loaded PDF document to PDF/A format.
```java
PdfDocument pdfa = pdfDocument.convertToPdfA();
```
Save the converted PDF/A document to a new file.
```java
pdfaDocument.saveAsPdfA("input_pdfa.pdf");
```
That's it! We have successfully converted a regular PDF to the PDF/A format using IronPDF in a Java application.
Here is the complete source code:
```java
package org.example;
import com.ironsoftware.ironpdf.*;
import java.io.IOException;
import java.nio.file.Paths;
public class Main {
public static void main(String [] args) throws IOException {
License.setLicenseKey("YOUR-LICENSE-KEY-HERE");
// Convert From HTML String
PdfDocument pdfDocument = PdfDocument.renderHtmlAsPdf("<h1> ~Hello World~ </h1> Made with IronPDF!");
PdfDocument pdfa = pdfDocument.convertToPdfA();
pdfa.saveAsPdfA("html_saved.pdf");
// Convert From File
pdfDocument = PdfDocument.fromFile(Paths.get("input.pdf"));
pdfa = pdfDocument.convertToPdfA();
pdfa.saveAsPdfA("input_pdfa.pdf");
}
}
```
The above code when executed results in two output files. One from HTML to PDF/A and the other from the original PDF file to PDF/A.
To explore more features of IronPDF and its PDF-related functionalities, please visit code examples and documentation pages.
Converting PDF documents to PDF/A is essential for long-term document preservation and accessibility. IronPDF is a reliable Java library that simplifies this conversion process. In this article, we discussed the prerequisites, setting up a Java project using IntelliJ IDEA, and adding IronPDF as a Maven dependency.
We also outlined the steps to convert a regular PDF to the PDF/A format. With IronPDF, you can ensure that your documents remain accessible and reliable over time, complying with ISO standards for archiving electronic documents.
IronPDF offers a free trial for commercial use. Download the IronPDF JAR file from here.
9 .NET API products for your office documents