Published January 20, 2023
Convert Excel to PDF in C# (Step-by-Step) Tutorial
Excel files can be difficult to share because they can be easily altered and are not compatible with all devices and programs. PDFs (Portable Document Format), on the other hand, are universally compatible and cannot be edited without specialized software. For this reason, many businesses require that developers convert Excel files (.xls, .xlsx) to ISO standardized version PDF format programmatically.
There are several reasons why this is important. First, it ensures that the data contained in the Excel workbook cannot be altered. Second, it allows the file to be viewed on any device or program. And finally, it makes it possible to password-protect the file, preventing unauthorized access. Overall, converting Excel files to PDF format is an important way to protect data and ensure its compatibility across all platforms.
Converting Excel to PDF document formats can be a simple task if you have the right tools and knowledge. Let's see how we can convert using the IronPDF C# library in .NET Core.
How to Convert Excel to PDF in C#
- Install C# library to convert Excel files to PDFs
- Export the Excel files into HTML files
- Generate PDF from HTML file with
RenderHtmlFileAsPdf
method - Add password optionally to the new PDF
- Save PDF that have identical styling of Excel file
IronPDF: .NET PDF Library
IronPDF is a .NET library that makes it easy to generate, edit, and merge PDF files in C# and VB.NET. With IronPDF, you can create PDF in C# from scratch or convert existing HTML, CSS, and JavaScript files into PDFs. You can also add headers, footers, watermarks, and page numbers to your PDFs. And if you need to collaborate with others on a project, IronPDF makes it easy to merge multiple PDF files into one.
Throughout this article, IronPDF will be used to show how to convert an Excel document into a PDF document using C# and .NET Core.
Prerequisites
There are some prerequisites to convert an xlsx file to a PDF document.
- Visual Studio 2022 (Recommended)
- A running .NET application with the latest .NET Framework (Recommended)
- Microsoft Office installed
- A stable internet connection to install the IronPDF library for Excel to PDF conversion process
Let's move to the main steps to convert an Excel file to PDF.
Step 1: Export your Excel Workbooks as HTML
To export your Excel file to HTML format, do the following steps:
Launch Microsoft Excel and load the Excel spreadsheets there.
Load Excel Spreadsheets
Select "Save As" from the "File" menu.
SaveAs Option
Click on the 'Browse' button and navigate to your desired location. From the File type dropdown, select the 'HTML Page'.
File Type Selection
Your Excel file will be converted to an HTML file if you complete the procedures above. We'll now use the exported HTML file to convert to PDF.
Step 2: Add IronPDF to your solution
We can install IronPDF in our project using the NuGet Package Manager Console. Click on the Tools from the top menu and select the NuGet Package Manager followed by "Package Manager Console".
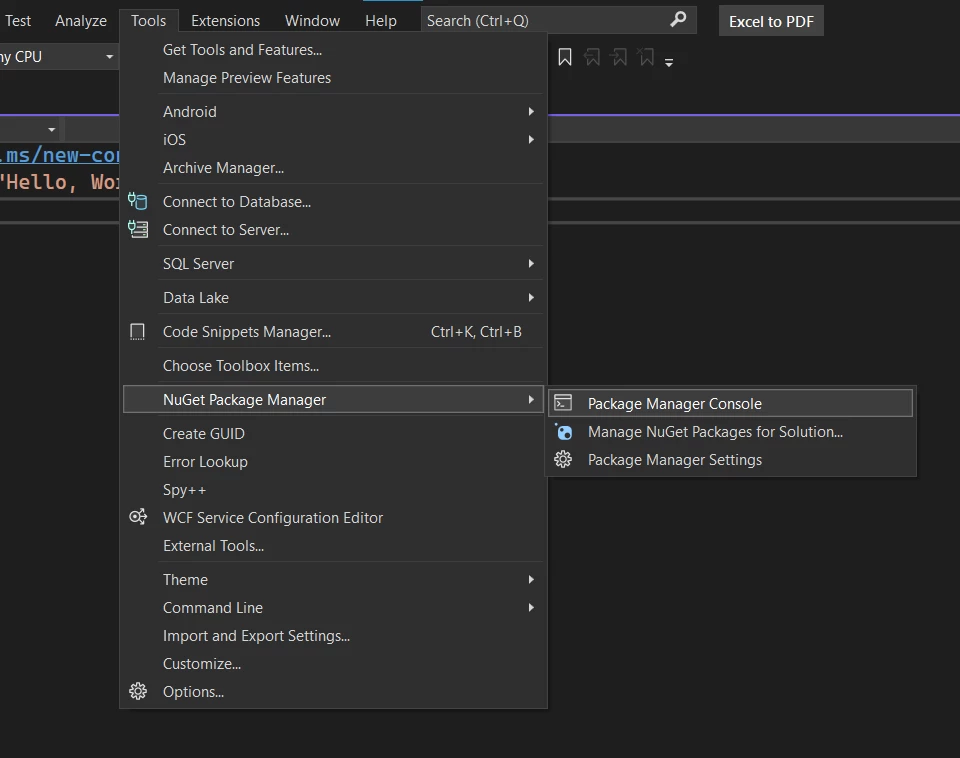
Package Manager
In the Console, write the following command to install IronPDF.
Install-Package IronPdf
This command will install the latest version of IronPDF in the project.
Step 3: Code Examples to Convert Excel File to PDF
Open the "Program.cs" file and write the following code fragments to convert the Excel file to PDF using the IronPDF C# library.
Step 3.1: Include IronPDF
Import the IronPDF in the code file to use it in our program.
using IronPdf;
using IronPdf;
Imports IronPdf
The above code enables IronPDF to take part in the code.
Step 3.2: Instantiate a ChromePDFRenderer Object
Instantiate a ChromePDFRenderer
object in the program. It'll enable to use IronPDF to convert the HTML file to PDF.
var renderer= new ChromePdfRenderer();
var renderer= new ChromePdfRenderer();
Dim renderer= New ChromePdfRenderer()
Step 3.3: Convert the HTML (Exported from Excel file) to PDF
Use the RenderHtmlFileAsPdf
function to convert HTML files to PDFs. In the parameter list, pass the HTML file path. IronPDF will read the contents of the HTML file and convert it into a PDF document.
var document = renderer.RenderHtmlFileAsPdf(@"C:\Users\Administrator\Desktop\Inventory.html");
var document = renderer.RenderHtmlFileAsPdf(@"C:\Users\Administrator\Desktop\Inventory.html");
Dim document = renderer.RenderHtmlFileAsPdf("C:\Users\Administrator\Desktop\Inventory.html")
IronPDF also supports adding passwords to the PDF file. We can prevent the PDF file from editing, opening, printing, and other operations. Let's have a look at how we can add password using IronPDF.
Add Password to PDF file
Use the SecuritySettings
property on the PDF document created previously in the previous step to set a password. It helps to secure the PDF file from data breaches.
document.SecuritySettings.UserPassword = "editable";
document.SecuritySettings.UserPassword = "editable";
document.SecuritySettings.UserPassword = "editable"
Step 3.4: Save the PDF File
Now, it's time to save the generated PDF file on the local machine by using the following code.
document.SaveAs("C:\\excelToPdf.pdf");
document.SaveAs("C:\\excelToPdf.pdf");
document.SaveAs("C:\excelToPdf.pdf")
Output PDF File
When you try to open the file, it'll require a password.
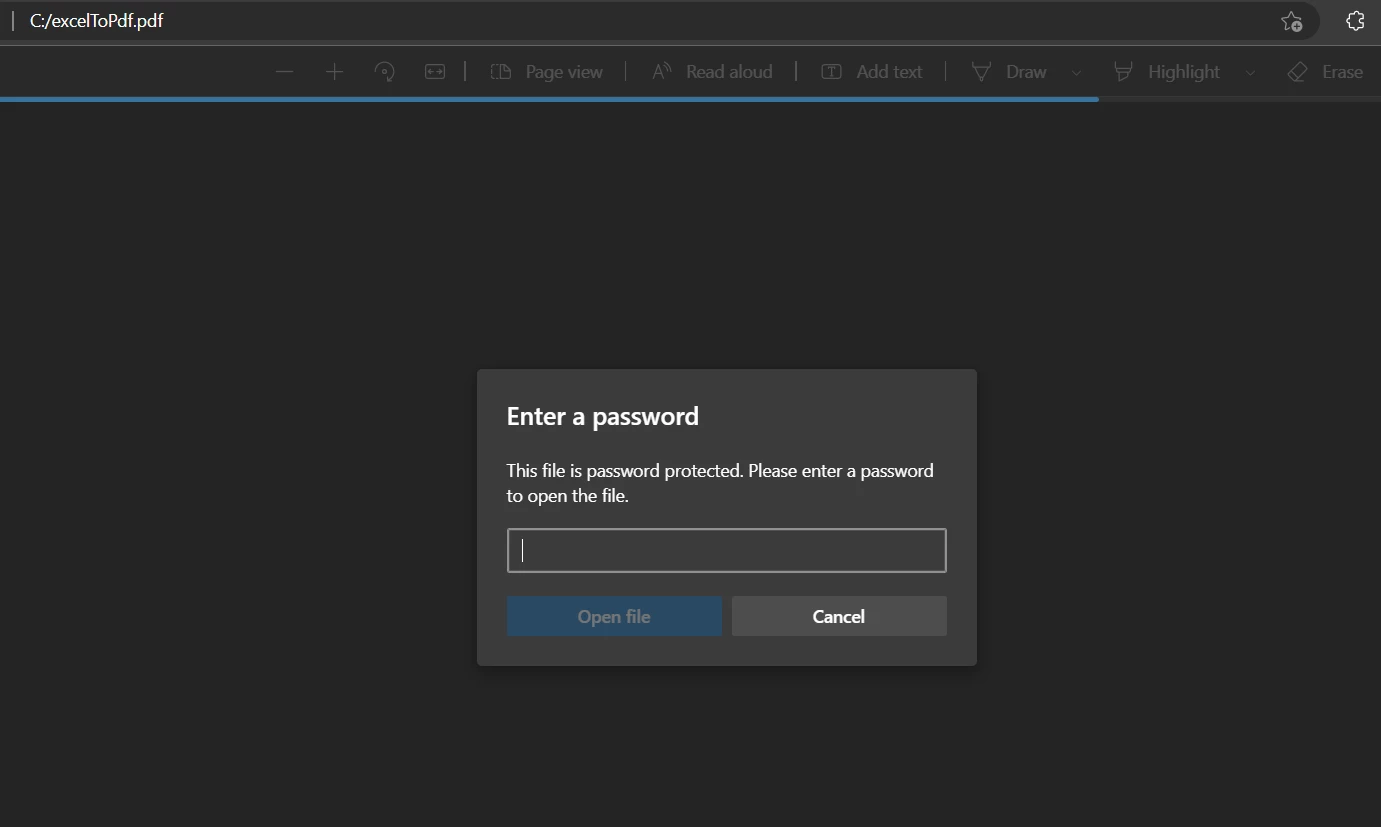
Enter Password for File
After entering the password, you can see the converted PDF file from an Excel file. IronPDF beautifully converts Excel documents to PDF while retaining the original formatting.
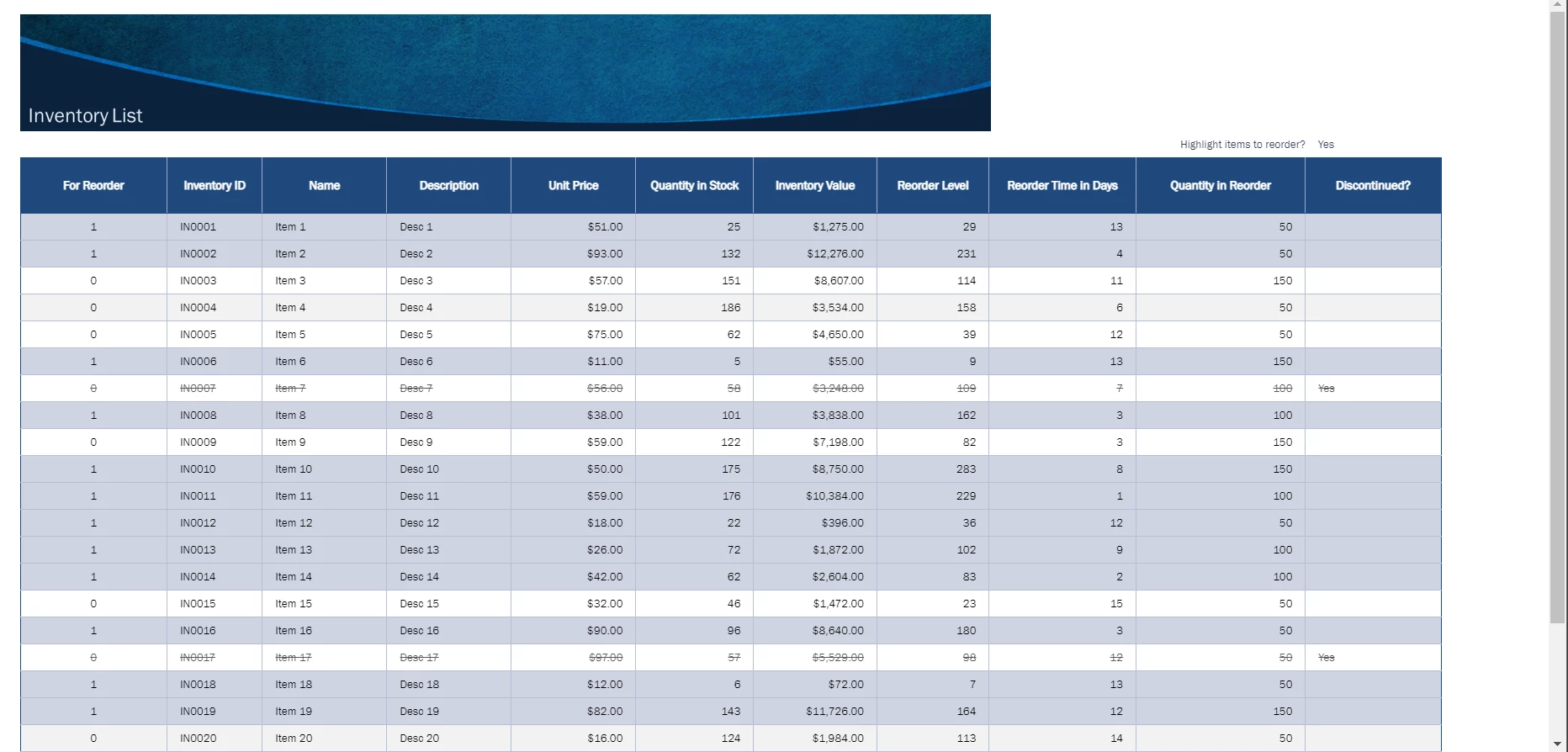
Output PDF File
You can see all worksheets there. You can open any worksheet by clicking on any worksheet name.
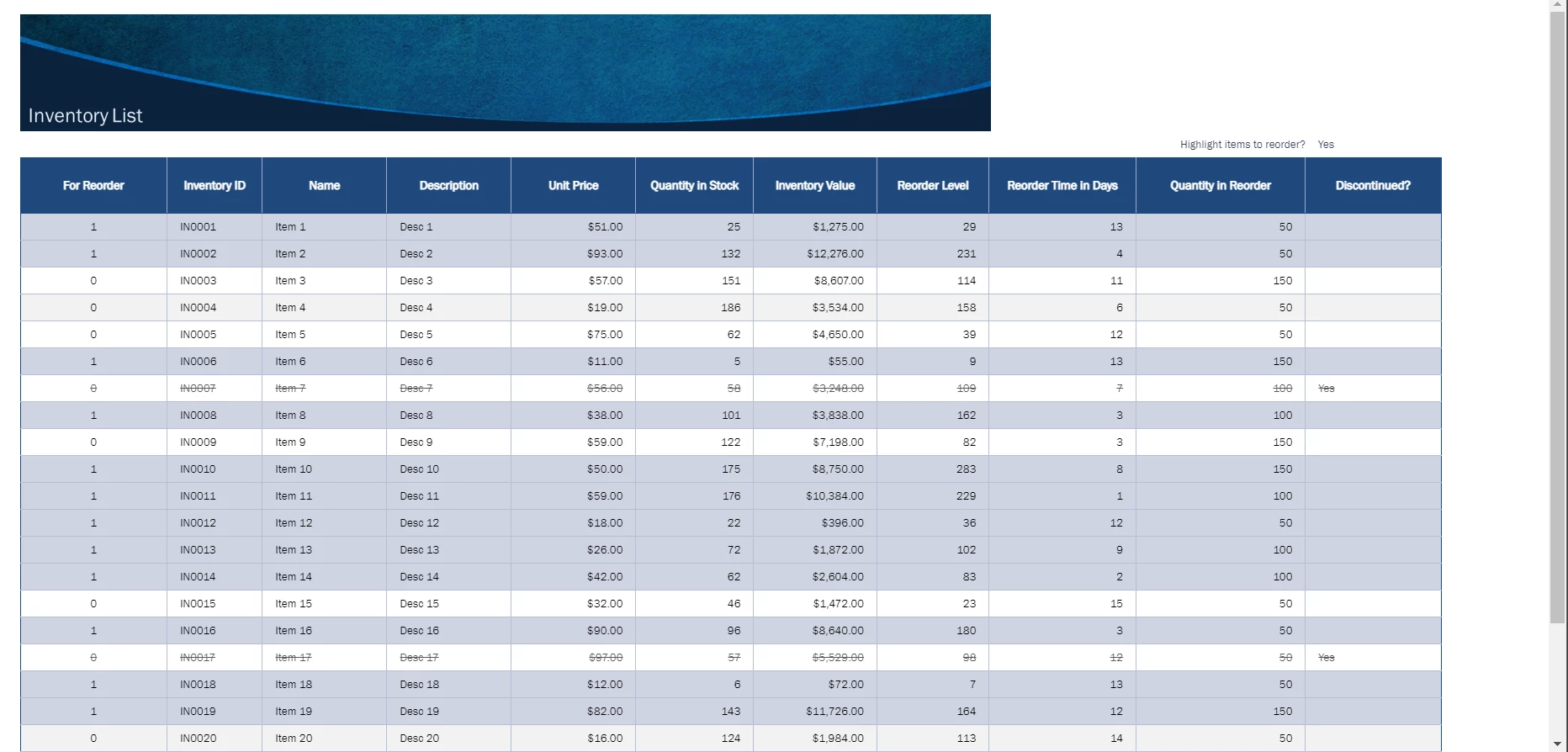
PDF Worksheets
Summary
IronPDF is an easy-to-use PDF converter that can be used in C#.NET. In this blog post, you were shown how to convert Excel to PDF using IronPDF. If you want to learn more about the capabilities of IronPDF or see some examples of how it can be used, check out some of our other articles.
You can start with a free trial, and then purchase IronPDF for as little as $749. Or, if you need more than one Iron Software product, you can buy the Iron Suite bundle of five software programs for the price of two.