How to Convert Images to a PDF
Converting images to PDF is a useful process that combines multiple image files (such as JPG, PNG, or TIFF) into a single PDF document. This is often done to create digital portfolios, presentations, or reports, making it easier to share and store a collection of images in a more organized and universally readable format.
IronPdf allows you to convert a single or multiple images into a PDF with unique image placements and behaviors. These behaviors include fitting to the page, centering on the page, and cropping the page. Additionally, you can add text and HTML headers and footers, apply watermarks, set custom page sizes, and include background and foreground overlays.
How to Convert Images to a PDF
Install with NuGet
Install-Package IronPdf
Install with NuGet
Install-Package IronPdf
Start using IronPDF in your project today with a free trial.
Check out IronPDF on Nuget for quick installation and deployment. With over 8 million downloads, it's transforming PDF with C#.
Consider installing the IronPDF DLL directly. Download and manually install it for your project or GAC form: IronPdf.zip
Convert Image to PDF Example
Use the ImageToPdf
static method within the ImageToPdfConverter class to convert an image to a PDF document. This method requires only the file path to the image, and it will convert it to a PDF document with default image placement and behavior. Supported image formats include .bmp, .jpeg, .jpg, .gif, .png, .svg, .tif, .tiff, .webp, .apng, .avif, .cur, .dib, .ico, .jfif, .jif, .jpe, .pjp, and .pjpeg.
Sample Image
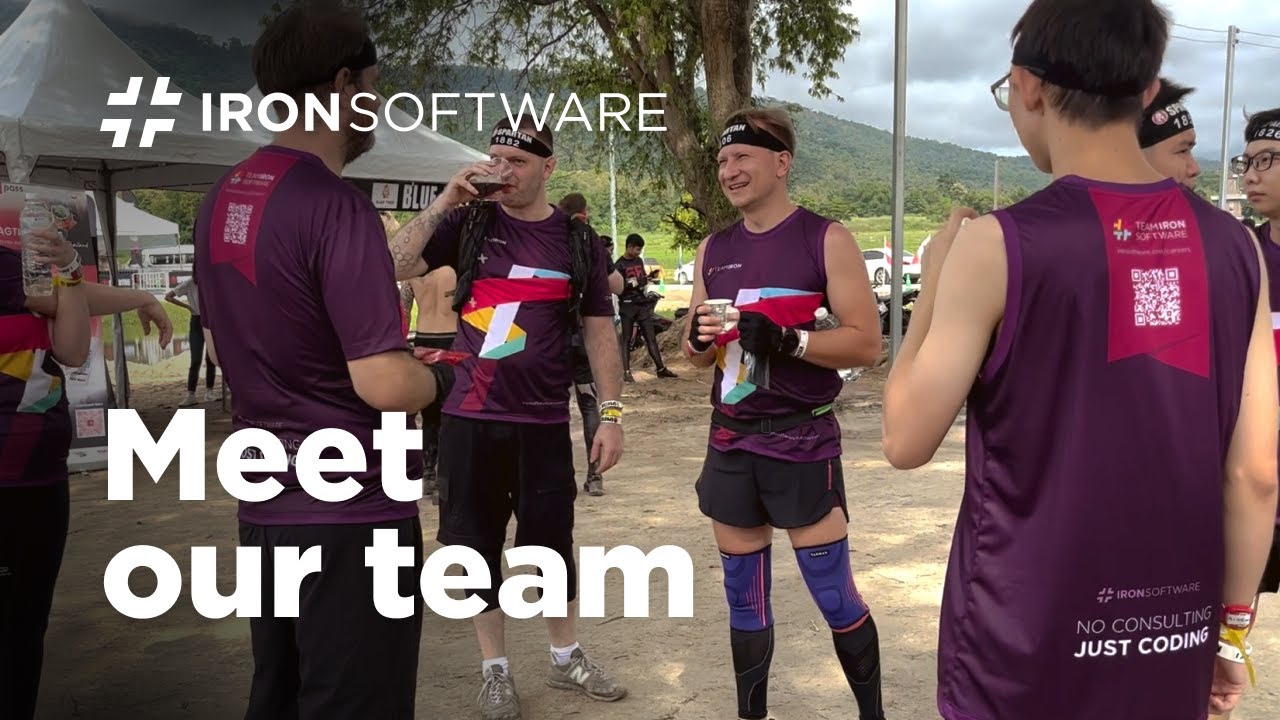
Code
:path=/static-assets/pdf/content-code-examples/how-to/image-to-pdf-convert-one-image.cs
using IronPdf;
string imagePath = "meetOurTeam.jpg";
// Convert an image to a PDF
PdfDocument pdf = ImageToPdfConverter.ImageToPdf(imagePath);
// Export the PDF
pdf.SaveAs("imageToPdf.pdf");
Imports IronPdf
Private imagePath As String = "meetOurTeam.jpg"
' Convert an image to a PDF
Private pdf As PdfDocument = ImageToPdfConverter.ImageToPdf(imagePath)
' Export the PDF
pdf.SaveAs("imageToPdf.pdf")
Output PDF
Convert Images to PDF Example
To convert multiple images into a PDF document, you should provide an IEnumerable object that contains file paths instead of a single file path, as shown in our previous example. This will once again generate a PDF document with default image placement and behavior.
:path=/static-assets/pdf/content-code-examples/how-to/image-to-pdf-convert-multiple-images.cs
using IronPdf;
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
// Retrieve all JPG and JPEG image paths in the 'images' folder.
IEnumerable<String> imagePaths = Directory.EnumerateFiles("images").Where(f => f.EndsWith(".jpg") || f.EndsWith(".jpeg"));
// Convert images to a PDF
PdfDocument pdf = ImageToPdfConverter.ImageToPdf(imagePaths);
// Export the PDF
pdf.SaveAs("imagesToPdf.pdf");
Imports IronPdf
Imports System
Imports System.Collections.Generic
Imports System.IO
Imports System.Linq
' Retrieve all JPG and JPEG image paths in the 'images' folder.
Private imagePaths As IEnumerable(Of String) = Directory.EnumerateFiles("images").Where(Function(f) f.EndsWith(".jpg") OrElse f.EndsWith(".jpeg"))
' Convert images to a PDF
Private pdf As PdfDocument = ImageToPdfConverter.ImageToPdf(imagePaths)
' Export the PDF
pdf.SaveAs("imagesToPdf.pdf")
Output PDF
Image Placements and Behaviors
For ease of use, we offer a range of helpful image placement and behavior options. For instance, you can center the image on the page or fit it to the page size while maintaining its aspect ratio. All available image placements and behaviors are as follows:
- TopLeftCornerOfPage: Image is placed at the top-left corner of the page.
- TopRightCornerOfPage: Image is placed at the top-right corner of the page.
- CenteredOnPage: Image is centered on the page.
- FitToPageAndMaintainAspectRatio: Image fits the page while keeping its original aspect ratio.
- BottomLeftCornerOfPage: Image is placed at the bottom-left corner of the page.
- BottomRightCornerOfPage: Image is placed at the bottom-right corner of the page.
- FitToPage: Image fits the page.
- CropPage: Page is adjusted to fit the image.
:path=/static-assets/pdf/content-code-examples/how-to/image-to-pdf-convert-one-image-image-behavior.cs
using IronPdf;
using IronPdf.Imaging;
string imagePath = "meetOurTeam.jpg";
// Convert an image to a PDF with image behavior of centered on page
PdfDocument pdf = ImageToPdfConverter.ImageToPdf(imagePath, ImageBehavior.CenteredOnPage);
// Export the PDF
pdf.SaveAs("imageToPdf.pdf");
Imports IronPdf
Imports IronPdf.Imaging
Private imagePath As String = "meetOurTeam.jpg"
' Convert an image to a PDF with image behavior of centered on page
Private pdf As PdfDocument = ImageToPdfConverter.ImageToPdf(imagePath, ImageBehavior.CenteredOnPage)
' Export the PDF
pdf.SaveAs("imageToPdf.pdf")
Image Behaviors Comparison
![]() TopLeftCornerOfPage | ![]() TopRightCornerOfPage |
![]() CenteredOnPage | ![]() FitToPageAndMaintainAspectRatio |
![]() BottomLeftCornerOfPage | ![]() BottomRightCornerOfPage |
![]() FitToPage | ![]() CropPage |
Apply Rendering Options
The key to converting various types of images into a PDF document under the hood of the ImageToPdf
static method is to import the image as an HTML img tag and then convert the HTML to PDF. This is also the reason we can pass the ChromePdfRenderOptions object as a third parameter of the ImageToPdf
method to customize the rendering process directly.
:path=/static-assets/pdf/content-code-examples/how-to/image-to-pdf-convert-one-image-rendering-options.cs
using IronPdf;
string imagePath = "meetOurTeam.jpg";
ChromePdfRenderOptions options = new ChromePdfRenderOptions()
{
HtmlHeader = new HtmlHeaderFooter()
{
HtmlFragment = "<h1 style='color: #2a95d5;'>Content Header</h1>",
DrawDividerLine = true,
},
};
// Convert an image to a PDF with custom header
PdfDocument pdf = ImageToPdfConverter.ImageToPdf(imagePath, options: options);
// Export the PDF
pdf.SaveAs("imageToPdfWithHeader.pdf");
Imports IronPdf
Private imagePath As String = "meetOurTeam.jpg"
Private options As New ChromePdfRenderOptions() With {
.HtmlHeader = New HtmlHeaderFooter() With {
.HtmlFragment = "<h1 style='color: #2a95d5;'>Content Header</h1>",
.DrawDividerLine = True
}
}
' Convert an image to a PDF with custom header
Private pdf As PdfDocument = ImageToPdfConverter.ImageToPdf(imagePath, options:= options)
' Export the PDF
pdf.SaveAs("imageToPdfWithHeader.pdf")
Output PDF
If you'd like to convert or rasterize a PDF document into images, please refer to our How to Rasterize a PDF to Image article.