C# 打印 PDF 文檔
您可以在 .NET 應用程式中輕鬆地使用 Visual Basic 或 C# 程式碼來列印 PDF。 本教程將指導您如何使用 C# 打印 PDF 功能來進行程式化列印。
如何在C#中列印PDF檔案
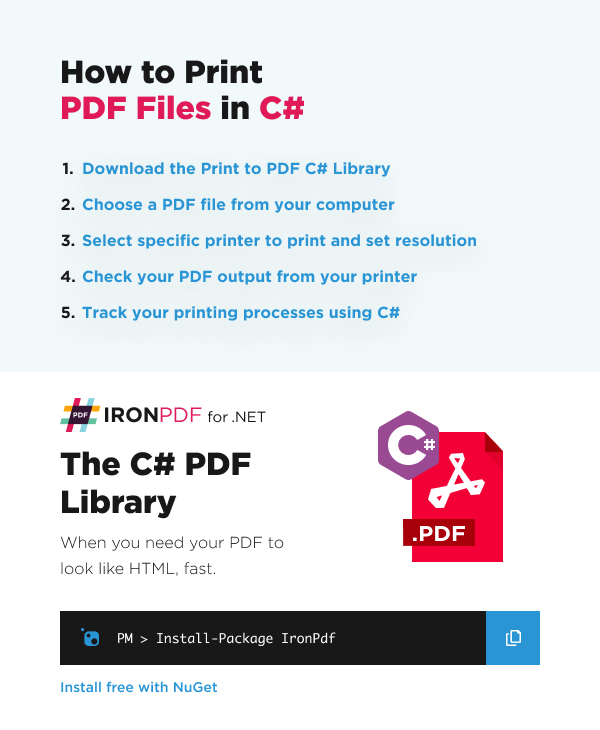
- 下載 Print to PDF C# 庫
- 從您的電腦選擇PDF文件
- 選擇特定的印表機進行列印並設置解析度
- 檢查您的印表機輸出的 PDF 文件
- 使用C#追踪您的打印流程
立即在您的專案中使用IronPDF,並享受免費試用。
建立 PDF 並列印
您可以將PDF文件直接靜默發送到打印機,或者創建一個System.Drawing.Printing.PrintDocument對象,可以進行操作並發送到 GUI 打印對話框。
以下程式碼可用於兩種選項:
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-create-and-print-pdf.cs
using IronPdf;
using System.Threading.Tasks;
// Create a new PDF and print it
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf");
// Print PDF from default printer
await pdf.Print();
// For advanced silent real-world printing options, use PdfDocument.GetPrintDocument
// Remember to add an assembly reference to System.Drawing.dll
System.Drawing.Printing.PrintDocument PrintDocYouCanWorkWith = pdf.GetPrintDocument();
Imports IronPdf
Imports System.Threading.Tasks
' Create a new PDF and print it
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf")
' Print PDF from default printer
Await pdf.Print()
' For advanced silent real-world printing options, use PdfDocument.GetPrintDocument
' Remember to add an assembly reference to System.Drawing.dll
Dim PrintDocYouCanWorkWith As System.Drawing.Printing.PrintDocument = pdf.GetPrintDocument()
進階列印
IronPDF 非常擅長處理高級打印功能,例如查找打印機名稱或設置打印機以及設置打印機解析度。
指定打印機名稱
要指定打印機名稱,您只需要獲取當前的打印文件對象。(使用用於 PDF 文件的 GetPrintDocument 方法PDF文件的)然後使用 PrinterSettings.PrinterName 屬性,如下所示:
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-specify-printer-name.cs
using IronPdf;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
// Get PrintDocument object
var printDocument = pdf.GetPrintDocument();
// Assign the printer name
printDocument.PrinterSettings.PrinterName = "Microsoft Print to PDF";
// Print document
printDocument.Print();
Imports IronPdf
Private pdf As PdfDocument = PdfDocument.FromFile("sample.pdf")
' Get PrintDocument object
Private printDocument = pdf.GetPrintDocument()
' Assign the printer name
printDocument.PrinterSettings.PrinterName = "Microsoft Print to PDF"
' Print document
printDocument.Print()
設定打印機解析度
解析度是指列印或顯示的像素數量,具體取決於您的輸出。 您可以通過使用來設置打印的解析度DefaultPageSettings.PrinterResolution
屬性PDF 文件的 這是一個非常快速的示範:
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-specify-printer-resolution.cs
using IronPdf;
using System.Drawing.Printing;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
// Get PrintDocument object
var printDocument = pdf.GetPrintDocument();
// Set printer resolution
printDocument.DefaultPageSettings.PrinterResolution = new PrinterResolution
{
Kind = PrinterResolutionKind.Custom,
X = 1200,
Y = 1200
};
// Print document
printDocument.Print();
Imports IronPdf
Imports System.Drawing.Printing
Private pdf As PdfDocument = PdfDocument.FromFile("sample.pdf")
' Get PrintDocument object
Private printDocument = pdf.GetPrintDocument()
' Set printer resolution
printDocument.DefaultPageSettings.PrinterResolution = New PrinterResolution With {
.Kind = PrinterResolutionKind.Custom,
.X = 1200,
.Y = 1200
}
' Print document
printDocument.Print()
如您所見,我已將解析度設為自定義等級:垂直 1200 和水平 1200。
PrintToFile 方法
PdfDocument.PrintToFile
方法允許您將 PDF 打印到文件。您只需提供輸出文件路徑並指定是否希望預覽。 下面的程式碼將會直接列印到指定的檔案,而不包含預覽:
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-printtofile.cs
using IronPdf;
using System.Threading.Tasks;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
await pdf.PrintToFile("PathToFile", false);
Imports IronPdf
Imports System.Threading.Tasks
Private pdf As PdfDocument = PdfDocument.FromFile("sample.pdf")
Await pdf.PrintToFile("PathToFile", False)
使用 C# 追踪打印过程
C# 與 IronPDF 的結合之美在於,當涉及到跟蹤打印頁面或任何與打印相關的內容時,實際上相當簡單。 在下一個範例中,我將演示如何更改打印機名稱、解析度,以及如何獲取已打印頁面的數量。
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-trace-printing-process.cs
using IronPdf;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
// Get PrintDocument object
var printDocument = pdf.GetPrintDocument();
// Subscribe to the PrintPage event
var printedPages = 0;
printDocument.PrintPage += (sender, args) => printedPages++;
// Print document
printDocument.Print();
Imports IronPdf
Private pdf As PdfDocument = PdfDocument.FromFile("sample.pdf")
' Get PrintDocument object
Private printDocument = pdf.GetPrintDocument()
' Subscribe to the PrintPage event
Private printedPages = 0
Private printDocument.PrintPage += Sub(sender, args) printedPages++
' Print document
printDocument.Print()