如何在 Azure Function 上運行和部署 IronPDF .NET

是的。 IronPDF 可用於在 Azure 上生成、操作和讀取 PDF 文件。 IronPDF 已在包括 MVC 網站、Azure Functions 等多個 Azure 平台上進行了全面測試。
如何在 Azure Function 中製作 PDF 生成器
- 在 Azure 中安裝 C# 庫來生成 PDF
- 選擇 Azure Basic B1 託管層或以上
- 取消勾選
從套件檔案執行
發布時的選項 - 請遵循建議的配置說明
- 使用範例程式碼在 Azure 上建立 PDF 生成器
如何操作教程
安裝 IronPdf 套件
Azure Function Apps 有三種不同的環境:Linux、Windows 和 Container。 本文說明如何在所有三种環境中設置IronPdf。 在這些選項中,建議使用 Azure Function App 容器,因為它提供一個獨立的環境。 首先,讓我們選擇適當的套件來安裝。
Azure Function App 容器
Azure Function App Container 涉及最少的麻煩,是部署 IronPdf 的推薦方式。
Install-Package IronPdf.Linux
配置 Docker 檔案
根據您使用的 Linux 發行版配置 Docker 文件。 請參考這篇文章詳細說明。
Azure Function App(Windows)
若要使用標準IronPDF包,確保未勾選從封裝檔案執行選項。 啟用此選項將專案部署為 ZIP 文件,這會干擾 IronPdf 的文件配置。 如果您更喜歡啟用從套件檔案執行選項,請安裝IronPdf.Slim請改用 package。
-IronPDF包裹
Install-Package IronPdf
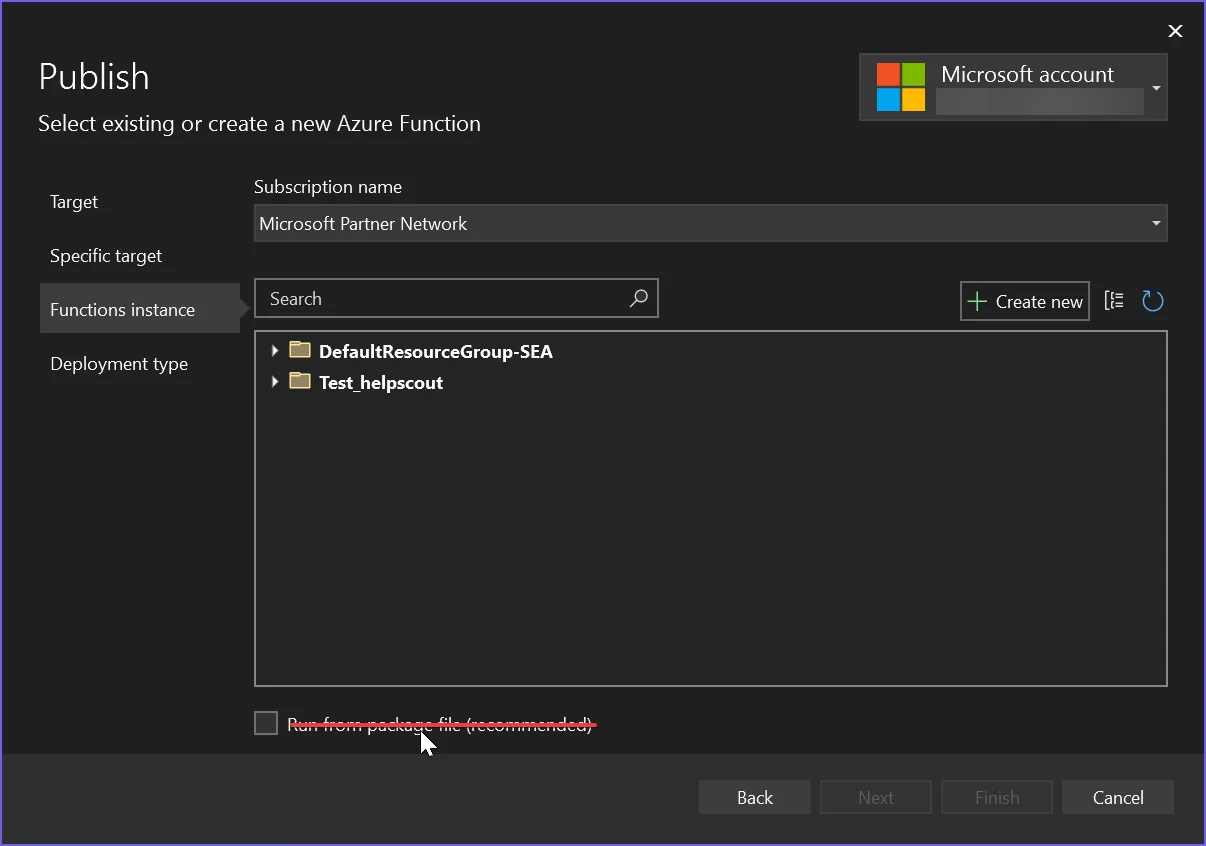
Azure Function App(Linux)
適用於 Azure Function App(Linux),專案預設會部署為 ZIP 檔,且此行為無法停用。 這類似於在 Azure Function App 上啟用 從包檔案運行 選項。(Windows).
-IronPdf.Slim包裹
安裝套件 IronPdf.Slim
選擇正確的Azure選項
選擇正確的託管層級
Azure Basic B1 是滿足我們終端用戶渲染需求的最低主機等級。 如果您正在創建一個高吞吐量系統,可能需要對其進行升級。
在繼續之前
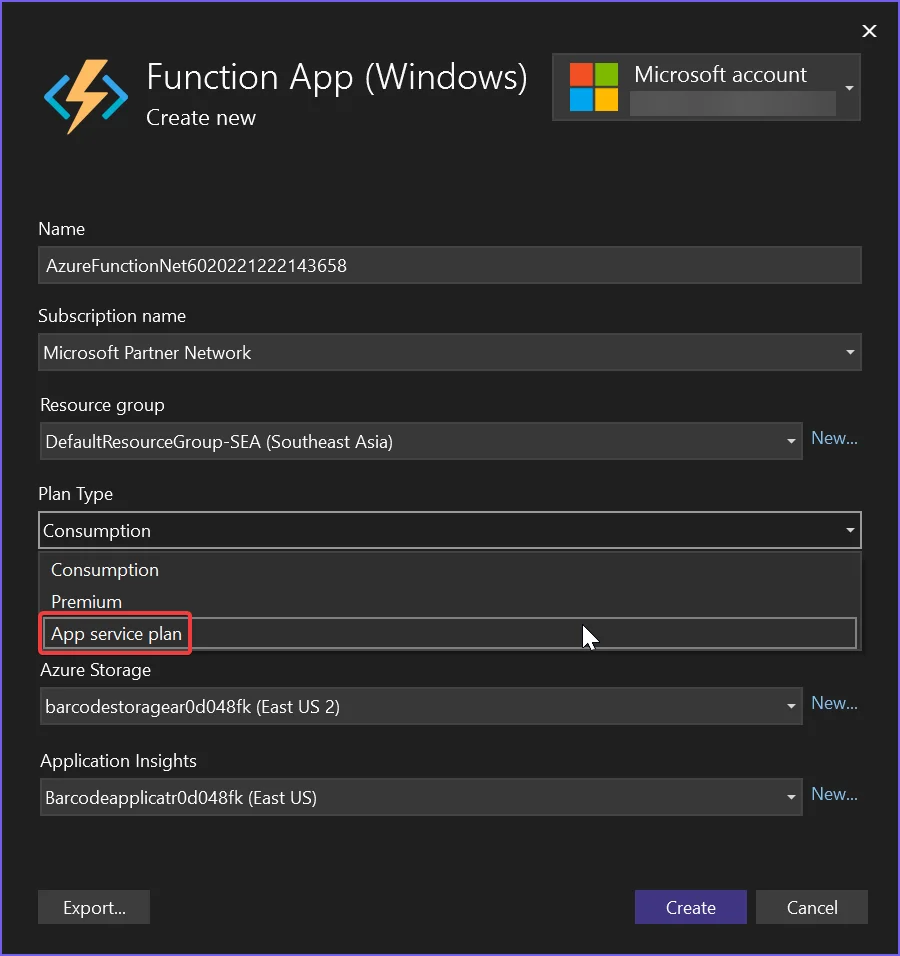
配置 .NET 6
Microsoft 最近從 .NET 6+ 中移除了成像庫,導致許多舊版 API 失效。因此,有必要配置您的項目以便仍能使用這些舊版 API。
-
在 Linux 上,設定
Installation.LinuxAndDockerDependenciesAutoConfig=true;
以確保機器上安裝了libgdiplus
。- 將以下內容添加到您的 .NET 6 項目的 .csproj 檔案中:
真 ```
- 將以下內容添加到您的 .NET 6 項目的 .csproj 檔案中:
- 在您的專案中創建一個名為
runtimeconfig.template.json
的文件,並用以下內容填充它:
{
"configProperties": {
"System.Drawing.EnableUnixSupport": true
}
}
- 最後,在您的程序開頭添加以下行:
System.AppContext.SetSwitch("System.Drawing.EnableUnixSupport", true);
Azure 函數代碼示例
此範例會自動輸出日誌條目至內建的Azure記錄器。(請參閱 ILogger log
).
[FunctionName("PrintPdf")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Anonymous, "get", "post", Route = null)] HttpRequest req,
ILogger log, ExecutionContext context)
{
log.LogInformation("Entered PrintPdf API function...");
// Apply license key
IronPdf.License.LicenseKey = "IRONPDF-MYLICENSE-KEY-1EF01";
// Enable log
IronPdf.Logging.Logger.LoggingMode = IronPdf.Logging.Logger.LoggingModes.Custom;
IronPdf.Logging.Logger.CustomLogger = log;
// Configure IronPdf
IronPdf.Installation.LinuxAndDockerDependenciesAutoConfig = true;
IronPdf.Installation.AutomaticallyDownloadNativeBinaries = true;
IronPdf.Installation.ChromeGpuMode = IronPdf.Engines.Chrome.ChromeGpuModes.Disabled;
IronPdf.Installation.CustomDeploymentDirectory = "/tmp";
try
{
log.LogInformation("About to render pdf...");
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render PDF
var pdf = renderer.RenderUrlAsPdf("https://www.google.com/");
log.LogInformation("finished rendering pdf...");
return new FileContentResult(pdf.BinaryData, "application/pdf") { FileDownloadName = "google.pdf" };
}
catch (Exception e)
{
log.LogError(e, "Error while rendering pdf", e);
return new OkObjectResult($"Error while rendering pdf: {e}");
}
}
[FunctionName("PrintPdf")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Anonymous, "get", "post", Route = null)] HttpRequest req,
ILogger log, ExecutionContext context)
{
log.LogInformation("Entered PrintPdf API function...");
// Apply license key
IronPdf.License.LicenseKey = "IRONPDF-MYLICENSE-KEY-1EF01";
// Enable log
IronPdf.Logging.Logger.LoggingMode = IronPdf.Logging.Logger.LoggingModes.Custom;
IronPdf.Logging.Logger.CustomLogger = log;
// Configure IronPdf
IronPdf.Installation.LinuxAndDockerDependenciesAutoConfig = true;
IronPdf.Installation.AutomaticallyDownloadNativeBinaries = true;
IronPdf.Installation.ChromeGpuMode = IronPdf.Engines.Chrome.ChromeGpuModes.Disabled;
IronPdf.Installation.CustomDeploymentDirectory = "/tmp";
try
{
log.LogInformation("About to render pdf...");
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render PDF
var pdf = renderer.RenderUrlAsPdf("https://www.google.com/");
log.LogInformation("finished rendering pdf...");
return new FileContentResult(pdf.BinaryData, "application/pdf") { FileDownloadName = "google.pdf" };
}
catch (Exception e)
{
log.LogError(e, "Error while rendering pdf", e);
return new OkObjectResult($"Error while rendering pdf: {e}");
}
}
<FunctionName("PrintPdf")>
Public Shared Async Function Run(<HttpTrigger(AuthorizationLevel.Anonymous, "get", "post", Route := Nothing)> ByVal req As HttpRequest, ByVal log As ILogger, ByVal context As ExecutionContext) As Task(Of IActionResult)
log.LogInformation("Entered PrintPdf API function...")
' Apply license key
IronPdf.License.LicenseKey = "IRONPDF-MYLICENSE-KEY-1EF01"
' Enable log
IronPdf.Logging.Logger.LoggingMode = IronPdf.Logging.Logger.LoggingModes.Custom
IronPdf.Logging.Logger.CustomLogger = log
' Configure IronPdf
IronPdf.Installation.LinuxAndDockerDependenciesAutoConfig = True
IronPdf.Installation.AutomaticallyDownloadNativeBinaries = True
IronPdf.Installation.ChromeGpuMode = IronPdf.Engines.Chrome.ChromeGpuModes.Disabled
IronPdf.Installation.CustomDeploymentDirectory = "/tmp"
Try
log.LogInformation("About to render pdf...")
Dim renderer As New ChromePdfRenderer()
' Render PDF
Dim pdf = renderer.RenderUrlAsPdf("https://www.google.com/")
log.LogInformation("finished rendering pdf...")
Return New FileContentResult(pdf.BinaryData, "application/pdf") With {.FileDownloadName = "google.pdf"}
Catch e As Exception
log.LogError(e, "Error while rendering pdf", e)
Return New OkObjectResult($"Error while rendering pdf: {e}")
End Try
End Function
在 Visual Studio 中使用 Azure 函數範本建立專案可能會產生略有不同的程式碼。 由於這些差異,即使安裝了相同的套件,一個專案可能運行正常,而另一個則無法運行。 如果發生此情況,請將 CustomDeploymentDirectory 屬性設置為 "/tmp"。
了解每個安裝配置
- LinuxAndDockerDependenciesAutoConfig:此設定會檢查並嘗試下載 Chrome 引擎所需的所有依賴項。在使用非 GUI 系統時(例如 Linux)是必要的。 在容器系統中,依賴項通常列在 Dockerfile 中;因此,您可以將此設置為 false。
- AutomaticallyDownloadNativeBinaries:此選項會在運行時下載本機 Chrome 二進位文件。使用 IronPdf.Slim 套件時需要它。
- CustomDeploymentDirectory:這個設定對於寫入權限有限的系統是必需的。
已知問題
SVG 字體渲染在共享主機計劃上無法使用。
我們發現的一個限制是Azure代管平台不支持在其較便宜的共享 Web 應用層中加載 SVG 字體(例如 Google Fonts)的服務器。 這是因為出於安全考量,這些共享主機平台不允許訪問 Windows GDI+ 圖形對象。
我们建议使用Windows 或 Linux Docker 容器或者在 Azure 上使用 VPS 來解決需要最佳字體渲染的問題。
Azure 免費層主機速度慢
Azure 的免費和共享層級,以及消費計劃,不適合用於 PDF 渲染。 我們推薦使用 Azure B1 主機/高級方案,這也是我們自己使用的。 將 HTML 轉換為 PDF
的過程對任何電腦來說都是重要的「工作」-類似於在自己的機器上開啟和渲染網頁。使用了真正的瀏覽器引擎,因此我們需要相應地提供設備並期望與具有相似功率的桌面機器有類似的渲染時間。
創建工程支援請求票证
為了建立請求票,請參考「如何提出 IronPDF 工程支持請求指南