如何將 Razor 頁面轉換為 PDF,在 ASP.NET Core Web 應用程式中
Razor 頁面是一個帶有 .cshtml 延伸名的文件,它結合了 C# 與 HTML 來生成網頁內容。 在ASP.NET Core中,Razor Pages是一種組織網頁應用程式代碼的更簡單方式,非常適合用於只讀或進行簡單數據輸入的簡單頁面。
ASP.NET Core Web 應用程式是使用 ASP.NET Core 開發的網頁應用程式,ASP.NET Core 是一個跨平台框架,用於開發現代網頁應用程式。
IronPDF 簡化了在 ASP.NET Core Web 應用程式專案中從 Razor 頁面創建 PDF 文件的過程。 這使得在ASP.NET Core Web應用程序中的PDF生成變得簡單直接。
如何將 Razor 頁面轉換為 PDF,在 ASP.NET Core Web 應用程式中
IronPDF 擴充套件包
IronPdf.Extensions.Razor 套件是主要IronPdf套件的擴充功能。 在 ASP.NET Core 網頁應用程式中,將 Razor 頁面渲染為 PDF 文件需要同時使用 IronPdf.Extensions.Razor 和 IronPdf 套件。
:InstallCmd Install-Package IronPdf.Extensions.Razor
:InstallCmd Install-Package IronPdf.Extensions.Razor
使用 NuGet 安裝
Install-Package IronPdf.Extensions.Razor
將 Razor 頁面渲染成 PDF 文件
您需要一個ASP.NET Core Web App專案來將Razor頁面轉換成PDF文件。
創建模型類别
- 在專案中建立一個新資料夾,並將其命名為「Models」。
- 在資料夾中新增一個標準的C#類別,並將其命名為「Person」。這個類別將作為個人數據的模型。 使用以下代碼片段:
:path=/static-assets/pdf/content-code-examples/how-to/cshtml-to-pdf-razor-model.cs
namespace RazorPageSample.Models
{
public class Person
{
public int Id { get; set; }
public string Name { get; set; }
public string Title { get; set; }
public string Description { get; set; }
}
}
Namespace RazorPageSample.Models
Public Class Person
Public Property Id() As Integer
Public Property Name() As String
Public Property Title() As String
Public Property Description() As String
End Class
End Namespace
加入 Razor 頁面
將一個空的 Razor 頁面添加到“Pages”文件夾並將其命名為“persons.cshtml”。
-
修改新建的 "Persons.cshtml" 文件,使用下面提供的代碼示例。
下面的代碼用於在瀏覽器中顯示信息。
@page
@using RazorPageSample.Models;
@model RazorPageSample.Pages.PersonsModel
@{
}
<table class="table">
<tr>
<th>Name</th>
<th>Title</th>
<th>Description</th>
</tr>
@foreach (var person in ViewData ["personList"] as List<Person>)
{
<tr>
<td>@person.Name</td>
<td>@person.Title</td>
<td>@person.Description</td>
</tr>
}
</table>
<form method="post">
<button type="submit">print</button>
</form>
@page
@using RazorPageSample.Models;
@model RazorPageSample.Pages.PersonsModel
@{
}
<table class="table">
<tr>
<th>Name</th>
<th>Title</th>
<th>Description</th>
</tr>
@foreach (var person in ViewData ["personList"] as List<Person>)
{
<tr>
<td>@person.Name</td>
<td>@person.Title</td>
<td>@person.Description</td>
</tr>
}
</table>
<form method="post">
<button type="submit">print</button>
</form>
接下來,下面的代碼首先實例化ChromePdfRenderer類。 將this 傳遞給 RenderRazorToPdf
方法即可將此 Razor 頁面轉換為 PDF 文件。
用戶可以完全訪問RenderingOptions中的功能。 這些功能包括在生成的 PDF 中應用頁碼的能力,設置自定義邊距,以及添加自定義文本和 HTML 頁眉和頁腳。
- 打開 "Persons.cshtml" 檔案的下拉選單以查看 "Persons.cshtml.cs" 檔案。
-
修改以下代碼的 "Persons.cshtml.cs"。
[{i:(可以使用以下程式碼在瀏覽器中查看 PDF 文件:
File(pdf.BinaryData, "application/pdf")
。 然而,在瀏覽器中查看後下載 PDF 會導致 PDF 文件損壞。
using IronPdf.Razor.Pages;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using RazorPageSample.Models;
namespace RazorPageSample.Pages
{
public class PersonsModel : PageModel
{
[BindProperty(SupportsGet = true)]
public List<Person> persons { get; set; }
public void OnGet()
{
persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
ViewData ["personList"] = persons;
}
public IActionResult OnPostAsync()
{
persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
ViewData ["personList"] = persons;
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render Razor Page to PDF document
PdfDocument pdf = renderer.RenderRazorToPdf(this);
Response.Headers.Add("Content-Disposition", "inline");
return File(pdf.BinaryData, "application/pdf", "razorPageToPdf.pdf");
// View output PDF on browser
return File(pdf.BinaryData, "application/pdf");
}
}
}
using IronPdf.Razor.Pages;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using RazorPageSample.Models;
namespace RazorPageSample.Pages
{
public class PersonsModel : PageModel
{
[BindProperty(SupportsGet = true)]
public List<Person> persons { get; set; }
public void OnGet()
{
persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
ViewData ["personList"] = persons;
}
public IActionResult OnPostAsync()
{
persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
ViewData ["personList"] = persons;
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render Razor Page to PDF document
PdfDocument pdf = renderer.RenderRazorToPdf(this);
Response.Headers.Add("Content-Disposition", "inline");
return File(pdf.BinaryData, "application/pdf", "razorPageToPdf.pdf");
// View output PDF on browser
return File(pdf.BinaryData, "application/pdf");
}
}
}
Imports IronPdf.Razor.Pages
Imports Microsoft.AspNetCore.Mvc
Imports Microsoft.AspNetCore.Mvc.RazorPages
Imports RazorPageSample.Models
Namespace RazorPageSample.Pages
Public Class PersonsModel
Inherits PageModel
<BindProperty(SupportsGet := True)>
Public Property persons() As List(Of Person)
Public Sub OnGet()
persons = New List(Of Person) From {
New Person With {
.Name = "Alice",
.Title = "Mrs.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Bob",
.Title = "Mr.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Charlie",
.Title = "Mr.",
.Description = "Software Engineer"
}
}
ViewData ("personList") = persons
End Sub
Public Function OnPostAsync() As IActionResult
persons = New List(Of Person) From {
New Person With {
.Name = "Alice",
.Title = "Mrs.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Bob",
.Title = "Mr.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Charlie",
.Title = "Mr.",
.Description = "Software Engineer"
}
}
ViewData ("personList") = persons
Dim renderer As New ChromePdfRenderer()
' Render Razor Page to PDF document
Dim pdf As PdfDocument = renderer.RenderRazorToPdf(Me)
Response.Headers.Add("Content-Disposition", "inline")
Return File(pdf.BinaryData, "application/pdf", "razorPageToPdf.pdf")
' View output PDF on browser
Return File(pdf.BinaryData, "application/pdf")
End Function
End Class
End Namespace
RenderRazorToPdf
方法返回一個 PdfDocument 對象,可進行額外的處理和編輯。 您可以將 PDF 匯出為 PDFA 或 PDFUA,對已呈現的 PDF 文件應用數位簽章,或是合併和拆分 PDF 文件。 該方法還允許您旋轉頁面、添加註釋或書籤,以及塗上一個自定義浮水印到您的PDF上。
將一個部分添加到頂部導航欄
-
導航到 Pages 資料夾 -> Shared 資料夾 -> _Layout.cshtml。 將「人員」導航項目放置在「首頁」之後。
請確保 asp-page 屬性的值與我們的檔案名稱完全匹配,在此例中為 "Persons"。
<header>
<nav class="navbar navbar-expand-sm navbar-toggleable-sm navbar-light bg-white border-bottom box-shadow mb-3">
<div class="container">
<a class="navbar-brand" asp-area="" asp-page="/Index">RazorPageSample</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target=".navbar-collapse" aria-controls="navbarSupportedContent"
aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="navbar-collapse collapse d-sm-inline-flex justify-content-between">
<ul class="navbar-nav flex-grow-1">
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Index">Home</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Persons">Person</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Privacy">Privacy</a>
</li>
</ul>
</div>
</div>
</nav>
</header>
<header>
<nav class="navbar navbar-expand-sm navbar-toggleable-sm navbar-light bg-white border-bottom box-shadow mb-3">
<div class="container">
<a class="navbar-brand" asp-area="" asp-page="/Index">RazorPageSample</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target=".navbar-collapse" aria-controls="navbarSupportedContent"
aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="navbar-collapse collapse d-sm-inline-flex justify-content-between">
<ul class="navbar-nav flex-grow-1">
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Index">Home</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Persons">Person</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Privacy">Privacy</a>
</li>
</ul>
</div>
</div>
</nav>
</header>
執行專案
這將向您展示如何運行項目並生成PDF文檔。
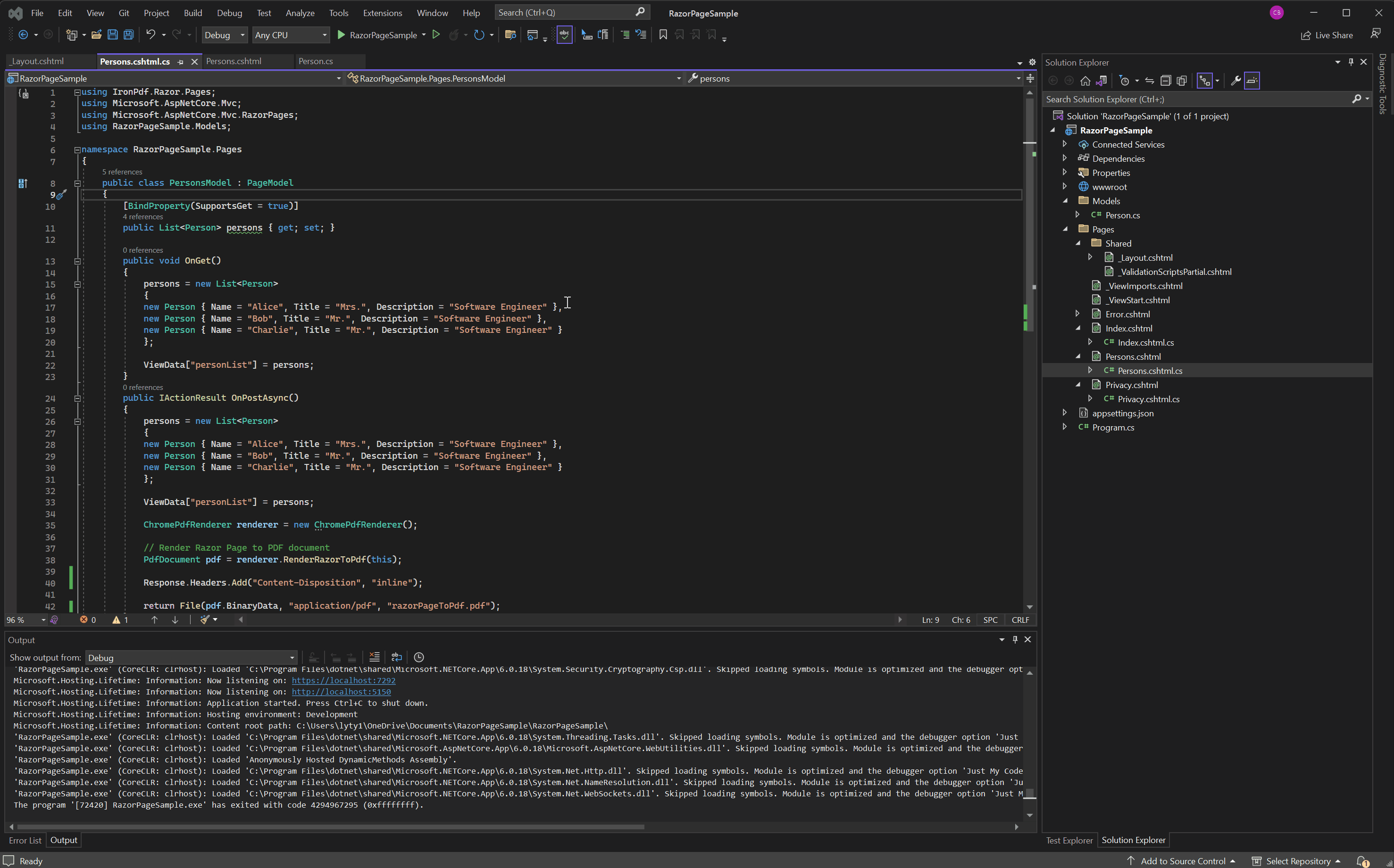