IronPDF Razor 擴展
IronPDF 是一個適用於 .NET 和 .NET Core 的 PDF 程式庫。 這主要是一個免費的 PDF 庫,因為 IronPDF 是一個商業的 C# PDF 庫。 開發時免費,但商業部署必須授權。 這種更清晰的授權模型不需要開發者去學習GNU / AGPL授權模型的各種細節,而可以專注於他們的項目。
IronPDF 讓 .NET 和 .NET Core 開發人員能夠在 C#、F# 和 VB.NET 上輕鬆生成、合併、拆分、編輯和擷取 PDF 內容,適用於 .NET Core 和 .NET Framework,還可以從 HTML、ASPX、CSS、JS 和圖像文件中創建 PDF。
IronPDF具有通過HTML到PDF的全面PDF編輯和生成功能。 它是如何運作的? 大多數的文件設計和佈局可以使用現有的HTML和HTML5資源。
您可以從下載 C# Razor-to-PDF 示例專案IronPDF Razor View 轉 PDF 下載.
如何從Razor View Web生成PDF
IronPDF 功能適用於 .NET 與 .NET Core 應用程式
一些出色的IronPDF PDF庫功能包括:
- .NET PDF 庫可以 生成PDF 從 HTML、圖像和 ASPX 文件生成文件
- 在 .NET 和 .NET Core 應用程式中讀取 PDF 文本
- 從PDF中提取數據和圖像
- 合併PDF文件
- 拆分PDFs
- 操作 PDF
IronPDF 優勢
- IronPDF PDF 庫易於安裝
- IronPDF .NET 庫提供快速且簡便的授權選項
- IronPDF 優於大多數 .NET PDF 庫,並超越大多數 .NET Core PDF 庫。
IronPDF 是您一直在尋找的 PDF 解決方案。
安裝 IronPDF PDF 庫
在 .NET 或 .NET Core 中安裝 IronPDF PDF 庫相當簡單。 您可以通过以下方式安装:
使用 NuGet 包管理器並在命令提示符中輸入以下內容:
Install-Package IronPdf
在 Visual Studio 中使用 NuGet 套件管理器,開啟專案功能表中的「選擇管理 NuGet 套件」,然後搜尋 IronPDF,如下所示:
圖 1 - IronPDF NuGet 套件
這會安裝PDF擴展。
使用 IronPDF,您可以使用 ASP.NET MVC 返回 PDF 檔案。以下是一些程式碼範例:
以下顯示的是一個可能由您的控制器提供服務的方法範例。
public FileResult Generate_PDF_FromHTML_Or_MVC(long id) {
using var objPDF = Renderer.RenderHtmlAsPdf("<h1>IronPDF and MVC Example</h1>"); //Create a PDF Document
var objLength = objPDF.BinaryData.Length; //return a PDF document from a view
Response.AppendHeader("Content-Length", objLength.ToString());
Response.AppendHeader("Content-Disposition", "inline; filename=PDFDocument_" + id + ".pdf");
return File(objPDF.BinaryData, "application/pdf;");
}
public FileResult Generate_PDF_FromHTML_Or_MVC(long id) {
using var objPDF = Renderer.RenderHtmlAsPdf("<h1>IronPDF and MVC Example</h1>"); //Create a PDF Document
var objLength = objPDF.BinaryData.Length; //return a PDF document from a view
Response.AppendHeader("Content-Length", objLength.ToString());
Response.AppendHeader("Content-Disposition", "inline; filename=PDFDocument_" + id + ".pdf");
return File(objPDF.BinaryData, "application/pdf;");
}
Public Function Generate_PDF_FromHTML_Or_MVC(ByVal id As Long) As FileResult
Dim objPDF = Renderer.RenderHtmlAsPdf("<h1>IronPDF and MVC Example</h1>") 'Create a PDF Document
Dim objLength = objPDF.BinaryData.Length 'return a PDF document from a view
Response.AppendHeader("Content-Length", objLength.ToString())
Response.AppendHeader("Content-Disposition", "inline; filename=PDFDocument_" & id & ".pdf")
Return File(objPDF.BinaryData, "application/pdf;")
End Function
以下是如何在 ASP.NET 中呈現已存在的 PDF 的範例。
Response.Clear();
Response.ContentType = "application/pdf";
Response.AddHeader("Content-Disposition", "attachment;filename=\"FileName.pdf\"");
Response.BinaryWrite(System.IO.File.ReadAllBytes("PdfName.pdf"));
Response.Flush();
Response.End();
Response.Clear();
Response.ContentType = "application/pdf";
Response.AddHeader("Content-Disposition", "attachment;filename=\"FileName.pdf\"");
Response.BinaryWrite(System.IO.File.ReadAllBytes("PdfName.pdf"));
Response.Flush();
Response.End();
Response.Clear()
Response.ContentType = "application/pdf"
Response.AddHeader("Content-Disposition", "attachment;filename=""FileName.pdf""")
Response.BinaryWrite(System.IO.File.ReadAllBytes("PdfName.pdf"))
Response.Flush()
Response.End()
讓我們使用MVC和.NET Core在ASP.NET中快速示範一個例子。 打開 Visual Studio 並創建一個新的 ASP.NET Core 網頁應用程式。
1. 在 Visual Studio 中創建一個新的 ASP.NET Core 網絡專案
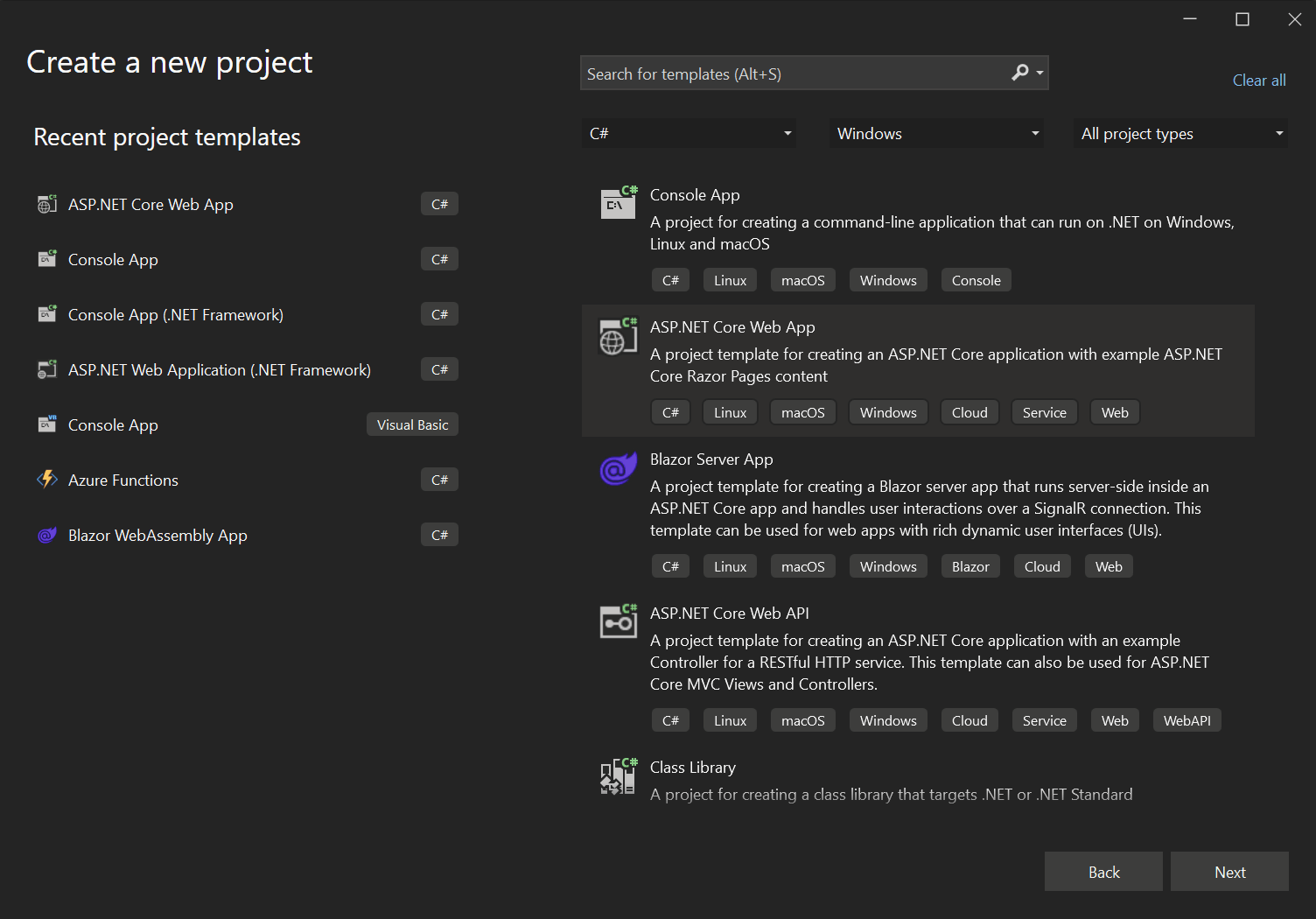
2. 建立 MVC 模型
- 創建一個新資料夾並將其命名為「Models」。
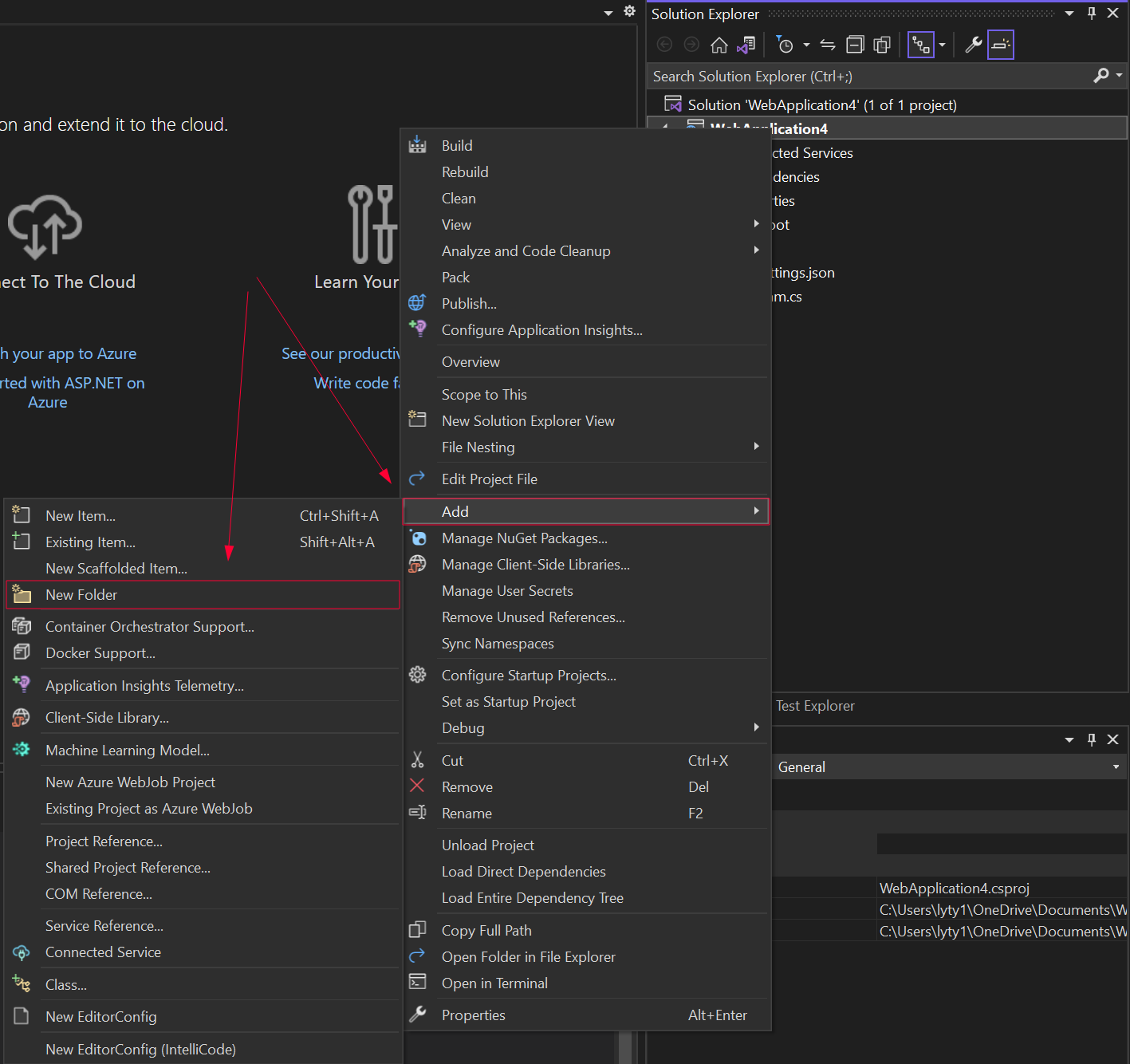
- 在模型資料夾上點擊右鍵,並新增一個類別。
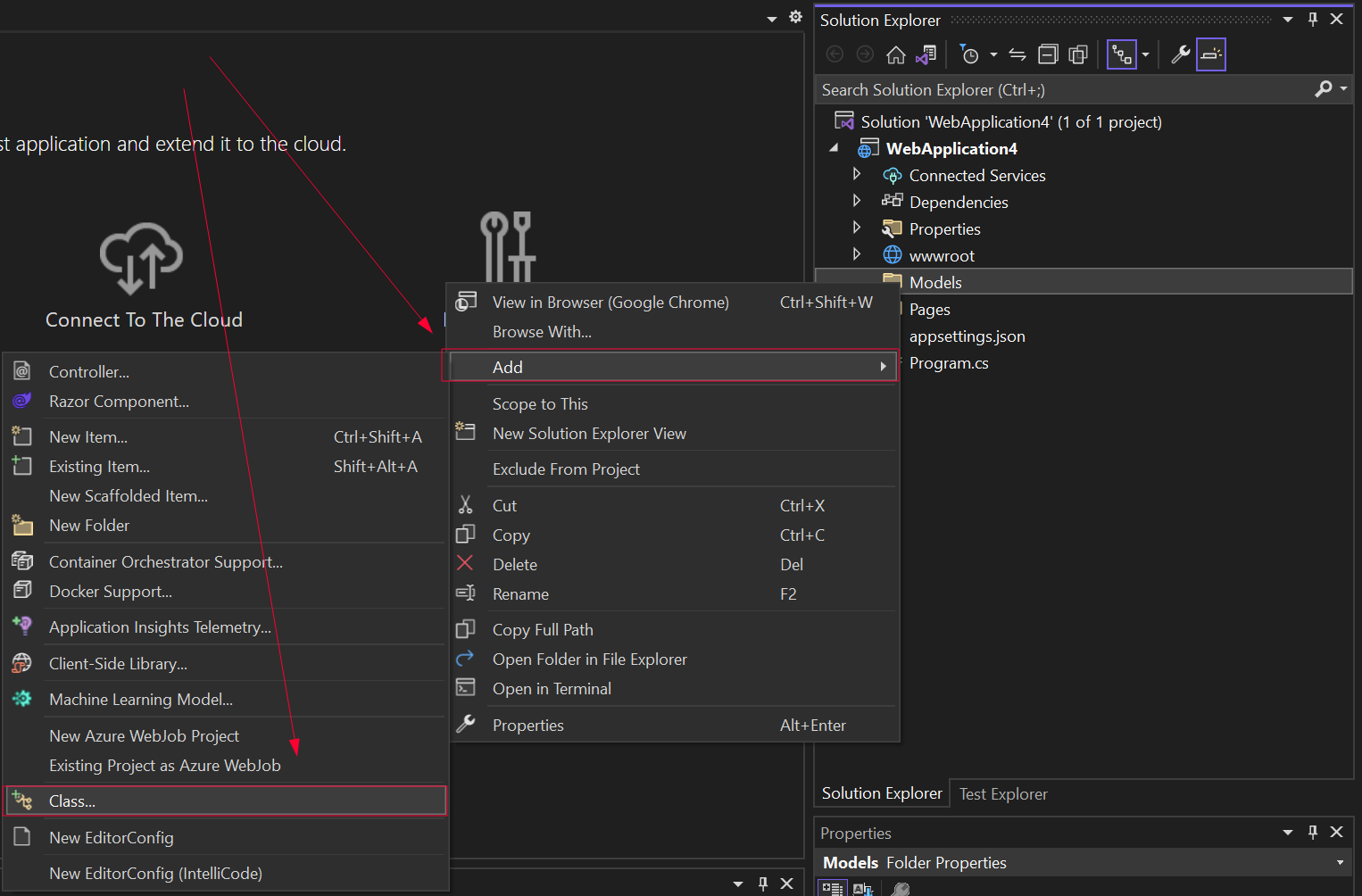
- 將類名更改為“ExampleModel”。 將內容添加到模型,例如:
namespace WebApplication4.Models
{
public class ExampleModel
{
public string Name { get; set; }
public string Surname { get; set; }
public int Age { get; set; }
}
}
namespace WebApplication4.Models
{
public class ExampleModel
{
public string Name { get; set; }
public string Surname { get; set; }
public int Age { get; set; }
}
}
Namespace WebApplication4.Models
Public Class ExampleModel
Public Property Name() As String
Public Property Surname() As String
Public Property Age() As Integer
End Class
End Namespace
3. 新增 MVC 控制器
- 創建一個新文件夾並將其命名為“Controllers”。
- 在 Controllers 資料夾上右鍵點擊,然後新增一個「MVC 控制器 - 空白」
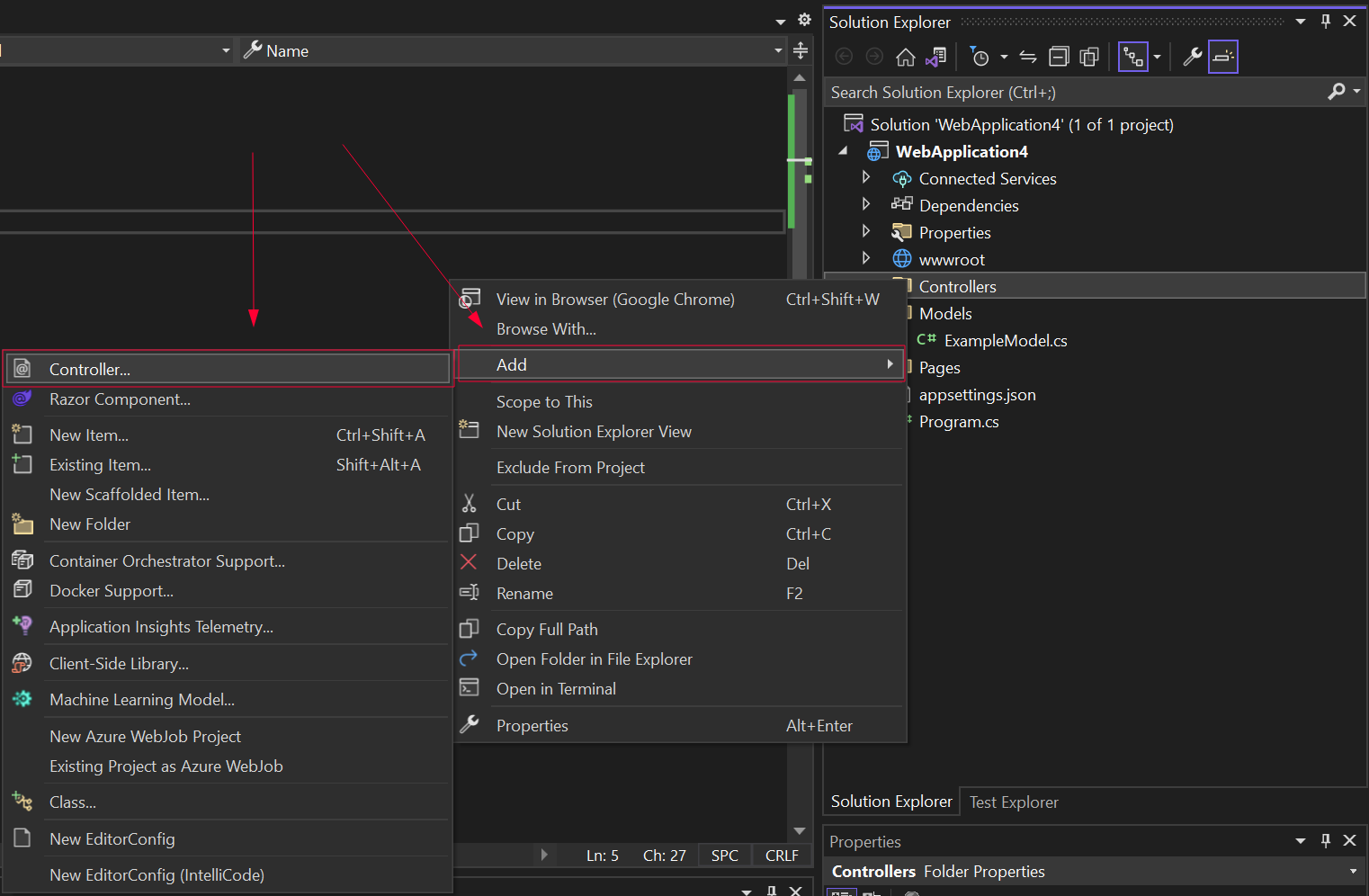
將內容新增到控制器:
namespace WebApplication4.Models
{
public class HomeController : Controller
{
[HttpPost]
public IActionResult ExampleView(ExampleModel model)
{
var html = this.RenderViewAsync("_Example", model);
var ironPdfRender = new IronPdf.ChromePdfRenderer();
using var pdfDoc = ironPdfRender.RenderHtmlAsPdf(html.Result);
return File(pdfDoc.Stream.ToArray(), "application/pdf");
}
}
}
namespace WebApplication4.Models
{
public class HomeController : Controller
{
[HttpPost]
public IActionResult ExampleView(ExampleModel model)
{
var html = this.RenderViewAsync("_Example", model);
var ironPdfRender = new IronPdf.ChromePdfRenderer();
using var pdfDoc = ironPdfRender.RenderHtmlAsPdf(html.Result);
return File(pdfDoc.Stream.ToArray(), "application/pdf");
}
}
}
Namespace WebApplication4.Models
Public Class HomeController
Inherits Controller
<HttpPost>
Public Function ExampleView(ByVal model As ExampleModel) As IActionResult
Dim html = Me.RenderViewAsync("_Example", model)
Dim ironPdfRender = New IronPdf.ChromePdfRenderer()
Dim pdfDoc = ironPdfRender.RenderHtmlAsPdf(html.Result)
Return File(pdfDoc.Stream.ToArray(), "application/pdf")
End Function
End Class
End Namespace
4. 修改 Index.cshtml
在 Pages 資料夾內,修改 Index.cshtml 檔案為:
@page
@model WebApplication4.Models.ExampleModel
@{
ViewBag.Title = "Example Index View";
}
<h2>Index</h2>
<form asp-action="ExampleView" enctype="multipart/form-data">
@using (Html.BeginForm())
{
<div class="form-horizontal">
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
<div class="form-group">
@Html.LabelFor(model => model.Name, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Name, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Name, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Surname, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Surname, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Surname, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Age, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Age, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Age, "", new { @class = "text-danger" })
</div>
</div>
<button type="submit">Save</button>
</div>
}
</form>
@page
@model WebApplication4.Models.ExampleModel
@{
ViewBag.Title = "Example Index View";
}
<h2>Index</h2>
<form asp-action="ExampleView" enctype="multipart/form-data">
@using (Html.BeginForm())
{
<div class="form-horizontal">
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
<div class="form-group">
@Html.LabelFor(model => model.Name, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Name, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Name, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Surname, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Surname, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Surname, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Age, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Age, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Age, "", new { @class = "text-danger" })
</div>
</div>
<button type="submit">Save</button>
</div>
}
</form>
5. 添加 Razor 頁面
在 Pages 的 Shared 資料夾內,新增一個 Razor 頁面並命名為 "_Example.cshtml"。
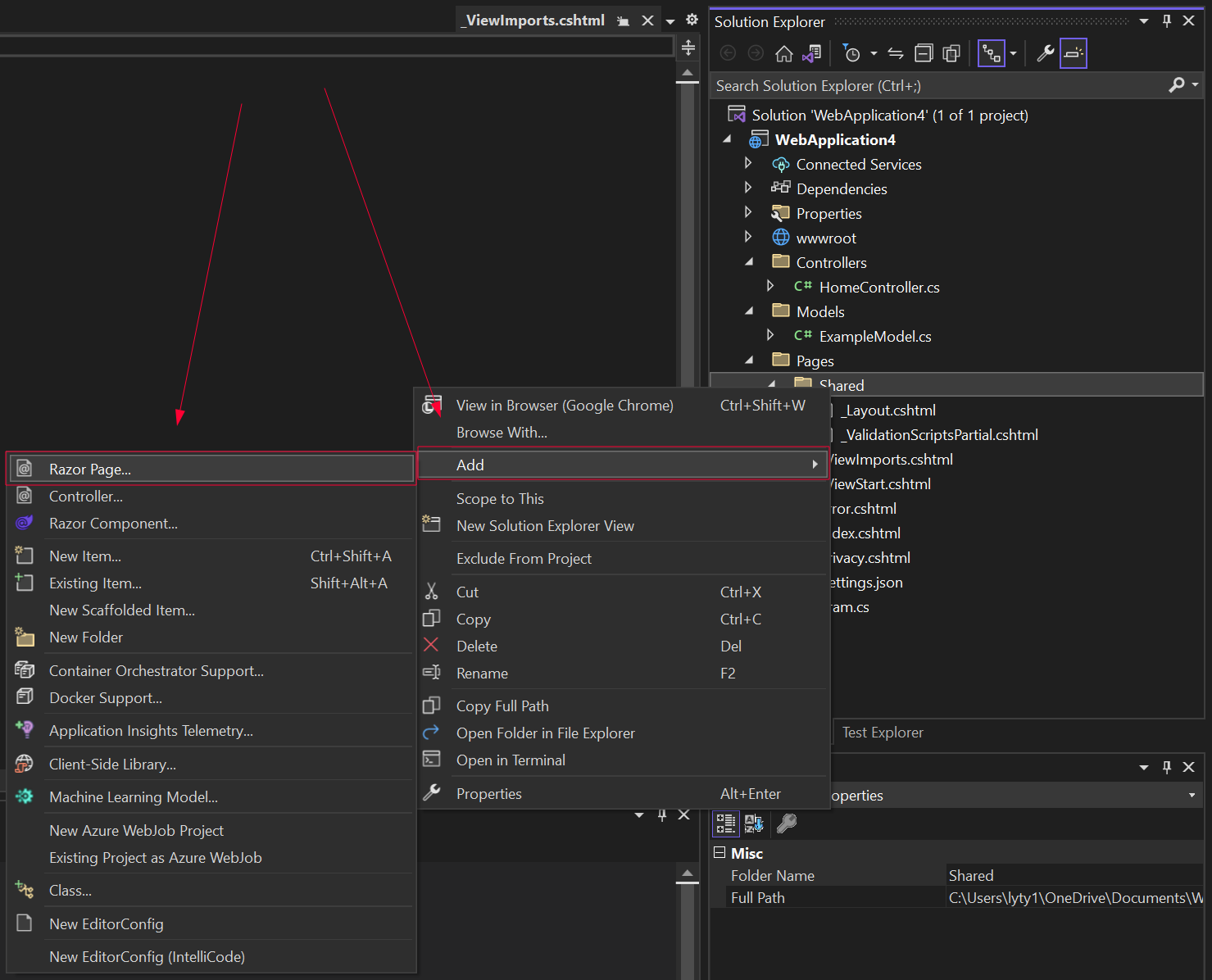
將以下代碼新增至 _Example.cshtml:
@Html.Partial("../Index.cshtml")
@Html.Partial("../Index.cshtml")
6. 添加新類別
-
添加一個名為「ControllerPDF」的新類別
此類別將使用 _Layout.cshtml 包裝 _Example.cshtml 的 HTML 並將其返回到 HomeController.cs。
- 添加以下代碼:
namespace WebApplication4
{
public static class ControllerPDF
{
public static async Task<string> RenderViewAsync<TModel>(this Controller controller, string viewName, TModel model, bool partial = false)
{
if (string.IsNullOrEmpty(viewName))
{
viewName = controller.ControllerContext.ActionDescriptor.ActionName;
}
controller.ViewData.Model = model;
using (var writer = new StringWriter())
{
IViewEngine viewEngine = controller.HttpContext.RequestServices.GetService(typeof(ICompositeViewEngine)) as ICompositeViewEngine;
ViewEngineResult viewResult = viewEngine.FindView(controller.ControllerContext, viewName, !partial);
if (viewResult.Success == false)
{
return $"A view with the name {viewName} could not be found";
}
ViewContext viewContext = new ViewContext(controller.ControllerContext, viewResult.View, controller.ViewData, controller.TempData, writer, new HtmlHelperOptions());
await viewResult.View.RenderAsync(viewContext);
return writer.GetStringBuilder().ToString();
}
}
}
}
namespace WebApplication4
{
public static class ControllerPDF
{
public static async Task<string> RenderViewAsync<TModel>(this Controller controller, string viewName, TModel model, bool partial = false)
{
if (string.IsNullOrEmpty(viewName))
{
viewName = controller.ControllerContext.ActionDescriptor.ActionName;
}
controller.ViewData.Model = model;
using (var writer = new StringWriter())
{
IViewEngine viewEngine = controller.HttpContext.RequestServices.GetService(typeof(ICompositeViewEngine)) as ICompositeViewEngine;
ViewEngineResult viewResult = viewEngine.FindView(controller.ControllerContext, viewName, !partial);
if (viewResult.Success == false)
{
return $"A view with the name {viewName} could not be found";
}
ViewContext viewContext = new ViewContext(controller.ControllerContext, viewResult.View, controller.ViewData, controller.TempData, writer, new HtmlHelperOptions());
await viewResult.View.RenderAsync(viewContext);
return writer.GetStringBuilder().ToString();
}
}
}
}
Namespace WebApplication4
Public Module ControllerPDF
<System.Runtime.CompilerServices.Extension> _
Public Async Function RenderViewAsync(Of TModel)(ByVal controller As Controller, ByVal viewName As String, ByVal model As TModel, Optional ByVal As Boolean = False) As Task(Of String)
If String.IsNullOrEmpty(viewName) Then
viewName = controller.ControllerContext.ActionDescriptor.ActionName
End If
controller.ViewData.Model = model
Using writer = New StringWriter()
Dim viewEngine As IViewEngine = TryCast(controller.HttpContext.RequestServices.GetService(GetType(ICompositeViewEngine)), ICompositeViewEngine)
Dim viewResult As ViewEngineResult = viewEngine.FindView(controller.ControllerContext, viewName, Not partial)
If viewResult.Success = False Then
Return $"A view with the name {viewName} could not be found"
End If
Dim viewContext As New ViewContext(controller.ControllerContext, viewResult.View, controller.ViewData, controller.TempData, writer, New HtmlHelperOptions())
Await viewResult.View.RenderAsync(viewContext)
Return writer.GetStringBuilder().ToString()
End Using
End Function
End Module
End Namespace
7. 修改 Program.cs
將以下代碼添加到您的項目中,以確保當保存按鈕被按下時,頁面將導航到正確的URL。
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.MapControllerRoute(name:= "default", pattern:= "{controller=Home}/{action=Index}/{id?}")
8. 示範
- 從Index.cshtml中,當儲存按鈕被按下且asp-action="ExampleView"時,ExampleView方法將被激活。
- ControllerPDF 類的 RenderViewAsync 方法將從 ExampleView 被調用。 此方法將返回由 _layout.cshtml 包裹的 _Example.cshtml 所生成的 HTML。
- 通過將 RenderViewAsync 返回的 HTML 傳遞給 IronPDF 的 RenderHtmlAsPdf 方法來生成 PDF 文件。
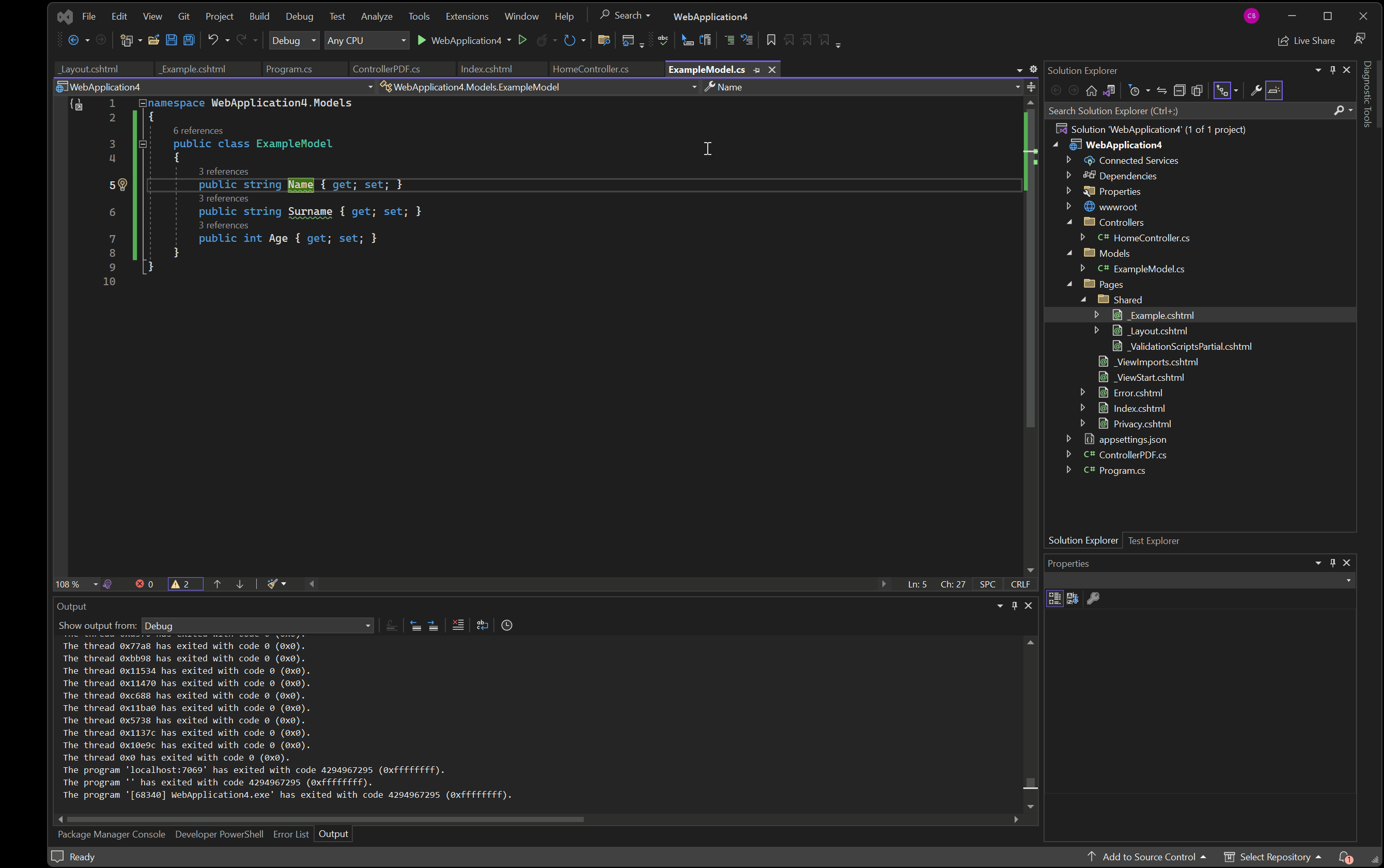