如何在C#中編輯PDF
介紹
Iron Software已將IronPDF庫中的許多不同PDF編輯功能簡化為易於閱讀和理解的方法。 無論是添加簽名、添加HTML頁腳、蓋上水印,還是添加註解。 IronPDF 是適合您的工具,它允許您擁有可讀的代碼、程式化的PDF生成、輕鬆的調試以及輕鬆部署到任何支持的環境或平台。
IronPDF 在編輯 PDF 時具有無數的功能。 在本教程文章中,我們將透過一些代碼示例和解釋來介紹幾個主要的主題。
透過本文,您將能夠了解如何在C#中使用IronPDF編輯您的PDF文件。
目錄
編輯文件結構
操作頁面
使用 IronPDF 在特定索引處添加 PDF、按範圍或逐一複製頁面、以及從任何 PDF 刪除頁面都非常簡單,IronPDF 會在幕後處理一切。
添加頁面
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-1.cs
var pdf = new PdfDocument("report.pdf");
var renderer = new ChromePdfRenderer();
var coverPagePdf = renderer.RenderHtmlAsPdf("<h1>Cover Page</h1><hr>");
pdf.PrependPdf(coverPagePdf);
pdf.SaveAs("report_with_cover.pdf");
Dim pdf = New PdfDocument("report.pdf")
Dim renderer = New ChromePdfRenderer()
Dim coverPagePdf = renderer.RenderHtmlAsPdf("<h1>Cover Page</h1><hr>")
pdf.PrependPdf(coverPagePdf)
pdf.SaveAs("report_with_cover.pdf")
複製頁面
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-2.cs
var pdf = new PdfDocument("report.pdf");
// Copy pages 5 to 7 and save them as a new document.
pdf.CopyPages(4, 6).SaveAs("report_highlight.pdf");
Dim pdf = New PdfDocument("report.pdf")
' Copy pages 5 to 7 and save them as a new document.
pdf.CopyPages(4, 6).SaveAs("report_highlight.pdf")
刪除頁面
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-3.cs
var pdf = new PdfDocument("report.pdf");
// Remove the last page from the PDF and save again
pdf.RemovePage(pdf.PageCount - 1);
pdf.SaveAs("report_minus_one_page.pdf");
Dim pdf = New PdfDocument("report.pdf")
' Remove the last page from the PDF and save again
pdf.RemovePage(pdf.PageCount - 1)
pdf.SaveAs("report_minus_one_page.pdf")
合併和拆分PDFs
使用 IronPDF 直观的 API 可轻松地将多个 PDF 合并为一个 PDF 或拆分现有的 PDF。
將多個現有的PDF合併成單一PDF文件
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-4.cs
var pdfs = new List<PdfDocument>
{
PdfDocument.FromFile("A.pdf"),
PdfDocument.FromFile("B.pdf"),
PdfDocument.FromFile("C.pdf")
};
PdfDocument mergedPdf = PdfDocument.Merge(pdfs);
mergedPdf.SaveAs("merged.pdf");
foreach (var pdf in pdfs)
{
pdf.Dispose();
}
Dim pdfs = New List(Of PdfDocument) From {PdfDocument.FromFile("A.pdf"), PdfDocument.FromFile("B.pdf"), PdfDocument.FromFile("C.pdf")}
Dim mergedPdf As PdfDocument = PdfDocument.Merge(pdfs)
mergedPdf.SaveAs("merged.pdf")
For Each pdf In pdfs
pdf.Dispose()
Next pdf
若要查看在我們的代碼範例頁面中合併兩個或多個 PDF,請訪問這裡。
分割PDF並提取頁面
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-5.cs
var pdf = new PdfDocument("sample.pdf");
// Take the first page
var pdf_page1 = pdf.CopyPage(0);
pdf_page1.SaveAs("Split1.pdf");
// Take the pages 2 & 3
var pdf_page2_3 = pdf.CopyPages(1, 2);
pdf_page2_3.SaveAs("Spli2t.pdf");
Dim pdf = New PdfDocument("sample.pdf")
' Take the first page
Dim pdf_page1 = pdf.CopyPage(0)
pdf_page1.SaveAs("Split1.pdf")
' Take the pages 2 & 3
Dim pdf_page2_3 = pdf.CopyPages(1, 2)
pdf_page2_3.SaveAs("Spli2t.pdf")
要在我們的程式碼範例頁面上查看分離和提取頁面,請造訪此處。
編輯文件屬性
添加和使用 PDF 中繼資料
您可以輕鬆使用IronPDF來瀏覽和編輯PDF元數據:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-6.cs
// Open an Encrypted File, alternatively create a new PDF from Html
var pdf = PdfDocument.FromFile("encrypted.pdf", "password");
// Edit file metadata
pdf.MetaData.Author = "Satoshi Nakamoto";
pdf.MetaData.Keywords = "SEO, Friendly";
pdf.MetaData.ModifiedDate = System.DateTime.Now;
// Edit file security settings
// The following code makes a PDF read only and will disallow copy & paste and printing
pdf.SecuritySettings.RemovePasswordsAndEncryption();
pdf.SecuritySettings.MakePdfDocumentReadOnly("secret-key");
pdf.SecuritySettings.AllowUserAnnotations = false;
pdf.SecuritySettings.AllowUserCopyPasteContent = false;
pdf.SecuritySettings.AllowUserFormData = false;
pdf.SecuritySettings.AllowUserPrinting = IronPdf.Security.PdfPrintSecurity.FullPrintRights;
// Change or set the document encryption password
pdf.SecuritySettings.OwnerPassword = "top-secret"; // password to edit the pdf
pdf.SecuritySettings.UserPassword = "shareable"; // password to open the pdf
pdf.SaveAs("secured.pdf");
Imports System
' Open an Encrypted File, alternatively create a new PDF from Html
Dim pdf = PdfDocument.FromFile("encrypted.pdf", "password")
' Edit file metadata
pdf.MetaData.Author = "Satoshi Nakamoto"
pdf.MetaData.Keywords = "SEO, Friendly"
pdf.MetaData.ModifiedDate = DateTime.Now
' Edit file security settings
' The following code makes a PDF read only and will disallow copy & paste and printing
pdf.SecuritySettings.RemovePasswordsAndEncryption()
pdf.SecuritySettings.MakePdfDocumentReadOnly("secret-key")
pdf.SecuritySettings.AllowUserAnnotations = False
pdf.SecuritySettings.AllowUserCopyPasteContent = False
pdf.SecuritySettings.AllowUserFormData = False
pdf.SecuritySettings.AllowUserPrinting = IronPdf.Security.PdfPrintSecurity.FullPrintRights
' Change or set the document encryption password
pdf.SecuritySettings.OwnerPassword = "top-secret" ' password to edit the pdf
pdf.SecuritySettings.UserPassword = "shareable" ' password to open the pdf
pdf.SaveAs("secured.pdf")
數位簽名
IronPDF支持使用.pfx和.p12 X509Certificate2數字證書對新的或現有的PDF文件進行數字簽名。
PDF 檔案簽名後,未經證書驗證便無法修改。 這確保了保真度。
要使用 Adobe Reader 免費生成簽名證書,請閱讀 https://helpx.adobe.com/acrobat/using/digital-ids.html。
除了加密簽名之外,也可以使用手寫簽名圖像或公司印章圖像來進行 IronPDF 簽名。
只需一行程式碼即可加密簽署現有的 PDF!
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-7.cs
using IronPdf;
using IronPdf.Signing;
new IronPdf.Signing.PdfSignature("Iron.p12", "123456").SignPdfFile("any.pdf");
Imports IronPdf
Imports IronPdf.Signing
Call (New IronPdf.Signing.PdfSignature("Iron.p12", "123456")).SignPdfFile("any.pdf")
更高級的範例,具有更多的控制:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-8.cs
using IronPdf;
// Step 1. Create a PDF
var renderer = new ChromePdfRenderer();
var doc = renderer.RenderHtmlAsPdf("<h1>Testing 2048 bit digital security</h1>");
// Step 2. Create a Signature.
// You may create a .pfx or .p12 PDF signing certificate using Adobe Acrobat Reader.
// Read: https://helpx.adobe.com/acrobat/using/digital-ids.html
var signature = new IronPdf.Signing.PdfSignature("Iron.pfx", "123456")
{
// Step 3. Optional signing options and a handwritten signature graphic
SigningContact = "support@ironsoftware.com",
SigningLocation = "Chicago, USA",
SigningReason = "To show how to sign a PDF"
};
//Step 4. Sign the PDF with the PdfSignature. Multiple signing certificates may be used
doc.Sign(signature);
//Step 5. The PDF is not signed until saved to file, steam or byte array.
doc.SaveAs("signed.pdf");
Imports IronPdf
' Step 1. Create a PDF
Private renderer = New ChromePdfRenderer()
Private doc = renderer.RenderHtmlAsPdf("<h1>Testing 2048 bit digital security</h1>")
' Step 2. Create a Signature.
' You may create a .pfx or .p12 PDF signing certificate using Adobe Acrobat Reader.
' Read: https://helpx.adobe.com/acrobat/using/digital-ids.html
Private signature = New IronPdf.Signing.PdfSignature("Iron.pfx", "123456") With {
.SigningContact = "support@ironsoftware.com",
.SigningLocation = "Chicago, USA",
.SigningReason = "To show how to sign a PDF"
}
'Step 4. Sign the PDF with the PdfSignature. Multiple signing certificates may be used
doc.Sign(signature)
'Step 5. The PDF is not signed until saved to file, steam or byte array.
doc.SaveAs("signed.pdf")
PDF 附件
IronPDF 完全支持向您的 PDF 文件添加和移除附件。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-9.cs
var Renderer = new ChromePdfRenderer();
var myPdf = Renderer.RenderHtmlFileAsPdf("my-content.html");
// Here we can add an attachment with a name and byte[]
var attachment1 = myPdf.Attachments.AddAttachment("attachment_1", example_attachment);
// And here is an example of removing an attachment
myPdf.Attachments.RemoveAttachment(attachment1);
myPdf.SaveAs("my-content.pdf");
Dim Renderer = New ChromePdfRenderer()
Dim myPdf = Renderer.RenderHtmlFileAsPdf("my-content.html")
' Here we can add an attachment with a name and byte[]
Dim attachment1 = myPdf.Attachments.AddAttachment("attachment_1", example_attachment)
' And here is an example of removing an attachment
myPdf.Attachments.RemoveAttachment(attachment1)
myPdf.SaveAs("my-content.pdf")
壓縮PDF
IronPDF 支援 PDF 壓縮。 PDF 文件大小可以通過減少 PdfDocument 中嵌入圖像的大小來降低。 在 IronPDF 中,我們可以調用 CompressImages
方法。
調整JPEG圖片大小的方式是,100%的品質幾乎沒有損失,而1%則是非常低的輸出圖像品質。 一般來說,90%及以上被認為是高品質,80%-90%被認為是中等品質,70%-80%被認為是低品質。 將品質降低至70%以下將會產生低品質的圖像,但這樣做可能會顯著減少PdfDocument的總檔案大小。
請嘗試使用不同的值,以感受品質與文件大小之間的範圍,並為您的需求找到最佳平衡。 影像質量下降的明顯性最終取決於輸入的圖像類型,某些圖像的清晰度可能會比其他圖像顯著降低。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-10.cs
var pdf = new PdfDocument("document.pdf");
// Quality parameter can be 1-100, where 100 is 100% of original quality
pdf.CompressImages(60);
pdf.SaveAs("document_compressed.pdf");
Dim pdf = New PdfDocument("document.pdf")
' Quality parameter can be 1-100, where 100 is 100% of original quality
pdf.CompressImages(60)
pdf.SaveAs("document_compressed.pdf")
該參數是第二個可選參數,可以根據其在PDF文件中的可見大小來縮小圖像分辨率。 請注意,這可能會導致某些圖像配置的扭曲:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-11.cs
var pdf = new PdfDocument("document.pdf");
pdf.CompressImages(90, true);
pdf.SaveAs("document_scaled_compressed.pdf");
Dim pdf = New PdfDocument("document.pdf")
pdf.CompressImages(90, True)
pdf.SaveAs("document_scaled_compressed.pdf")
編輯PDF內容
添加頁眉和頁腳
您可以輕鬆地為您的PDF添加頁首和頁尾。 IronPDF 有兩種不同類型的「HeaderFooters」,分別是TextHeaderFooter
和 HtmlHeaderFooter
。 TextHeaderFooter適用於僅包含文字且可能需要使用合併欄位(如"{page} of {total-pages}"
)的頁首和頁尾。 HtmlHeaderFooter 是一種進階的頁首和頁尾,可以處理其中的任何 HTML 內容,並且格式整齊。
HTML 標頭和頁尾
HTML頁首和頁尾將使用您的HTML的渲染版本作為PDF文檔的頁首或頁尾,以實現像素完美的佈局。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-12.cs
var renderer = new ChromePdfRenderer();
// Build a footer using html to style the text
// mergeable fields are:
// {page} {total-pages} {url} {date} {time} {html-title} & {pdf-title}
renderer.RenderingOptions.HtmlFooter = new HtmlHeaderFooter()
{
MaxHeight = 15, //millimeters
HtmlFragment = "<center><i>{page} of {total-pages}<i></center>",
DrawDividerLine = true
};
// Build a header using an image asset
// Note the use of BaseUrl to set a relative path to the assets
renderer.RenderingOptions.HtmlHeader = new HtmlHeaderFooter()
{
MaxHeight = 20, //millimeters
HtmlFragment = "<img src='logo.png'>",
BaseUrl = new System.Uri(@"C:\assets\images").AbsoluteUri
};
Dim renderer = New ChromePdfRenderer()
' Build a footer using html to style the text
' mergeable fields are:
' {page} {total-pages} {url} {date} {time} {html-title} & {pdf-title}
renderer.RenderingOptions.HtmlFooter = New HtmlHeaderFooter() With {
.MaxHeight = 15,
.HtmlFragment = "<center><i>{page} of {total-pages}<i></center>",
.DrawDividerLine = True
}
' Build a header using an image asset
' Note the use of BaseUrl to set a relative path to the assets
renderer.RenderingOptions.HtmlHeader = New HtmlHeaderFooter() With {
.MaxHeight = 20,
.HtmlFragment = "<img src='logo.png'>",
.BaseUrl = (New System.Uri("C:\assets\images")).AbsoluteUri
}
如需包含多種使用案例的完整詳細範例,請參閱以下教程:此處。
文本標頭和頁尾
基本的頁首和頁尾是 TextHeaderFooter,請參考下面的例子。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-13.cs
var renderer = new ChromePdfRenderer
{
RenderingOptions =
{
FirstPageNumber = 1, // use 2 if a cover-page will be appended
// Add a header to every page easily:
TextHeader =
{
DrawDividerLine = true,
CenterText = "{url}",
Font = IronSoftware.Drawing.FontTypes.Helvetica,
FontSize = 12
},
// Add a footer too:
TextFooter =
{
DrawDividerLine = true,
Font = IronSoftware.Drawing.FontTypes.Arial,
FontSize = 10,
LeftText = "{date} {time}",
RightText = "{page} of {total-pages}"
}
}
};
Dim renderer = New ChromePdfRenderer With {
.RenderingOptions = {
FirstPageNumber = 1, TextHeader = {
DrawDividerLine = True,
CenterText = "{url}",
Font = IronSoftware.Drawing.FontTypes.Helvetica,
FontSize = 12
},
TextFooter = {
DrawDividerLine = True,
Font = IronSoftware.Drawing.FontTypes.Arial,
FontSize = 10,
LeftText = "{date} {time}",
RightText = "{page} of {total-pages}"
}
}
}
我們還有以下合併欄位,這些欄位在呈現時將被替換為相應的值:{page}
、{total-pages}
、{url}
、{date}
、{time}
、{html-title}
、{pdf-title}
在 PDF 中尋找並替換文字
在您的 PDF 中製作佔位符並以程式方式替換它們,或使用我們的 ReplaceTextOnPage
方法簡單地替換所有文本短語的實例。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-14.cs
// Using an existing PDF
var pdf = PdfDocument.FromFile("sample.pdf");
// Parameters
int pageIndex = 1;
string oldText = ".NET 6"; // Old text to remove
string newText = ".NET 7"; // New text to add
// Replace Text on Page
pdf.ReplaceTextOnPage(pageIndex, oldText, newText);
// Placeholder Template Example
pdf.ReplaceTextOnPage(pageIndex, "[DATE]", "01/01/2000");
// Save your new PDF
pdf.SaveAs("new_sample.pdf");
' Using an existing PDF
Dim pdf = PdfDocument.FromFile("sample.pdf")
' Parameters
Dim pageIndex As Integer = 1
Dim oldText As String = ".NET 6" ' Old text to remove
Dim newText As String = ".NET 7" ' New text to add
' Replace Text on Page
pdf.ReplaceTextOnPage(pageIndex, oldText, newText)
' Placeholder Template Example
pdf.ReplaceTextOnPage(pageIndex, "[DATE]", "01/01/2000")
' Save your new PDF
pdf.SaveAs("new_sample.pdf")
要查看我們程式碼範例頁面上的查找和替換文字範例,請訪問這裡
大綱和書籤
在PDF中添加大綱或“書籤”可以方便定位到關鍵頁面。 在 Adobe Acrobat Reader 中,這些書籤(可能是嵌套的)顯示在應用程式的左側邊欄。 IronPDF 將自動從 PDF 文件導入現有的書籤,並允許添加、編輯和嵌套更多書籤。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-15.cs
// Create a new PDF or edit an existing document.
PdfDocument pdf = PdfDocument.FromFile("existing.pdf");
// Add bookmark
pdf.Bookmarks.AddBookMarkAtEnd("Author's Note", 2);
pdf.Bookmarks.AddBookMarkAtEnd("Table of Contents", 3);
// Store new bookmark in a variable to add nested bookmarks to
var summaryBookmark = pdf.Bookmarks.AddBookMarkAtEnd("Summary", 17);
// Add a sub-bookmark within the summary
var conclusionBookmark = summaryBookmark.Children.AddBookMarkAtStart("Conclusion", 18);
// Add another bookmark to end of highest-level bookmark list
pdf.Bookmarks.AddBookMarkAtEnd("References", 20);
pdf.SaveAs("existing.pdf");
' Create a new PDF or edit an existing document.
Dim pdf As PdfDocument = PdfDocument.FromFile("existing.pdf")
' Add bookmark
pdf.Bookmarks.AddBookMarkAtEnd("Author's Note", 2)
pdf.Bookmarks.AddBookMarkAtEnd("Table of Contents", 3)
' Store new bookmark in a variable to add nested bookmarks to
Dim summaryBookmark = pdf.Bookmarks.AddBookMarkAtEnd("Summary", 17)
' Add a sub-bookmark within the summary
Dim conclusionBookmark = summaryBookmark.Children.AddBookMarkAtStart("Conclusion", 18)
' Add another bookmark to end of highest-level bookmark list
pdf.Bookmarks.AddBookMarkAtEnd("References", 20)
pdf.SaveAs("existing.pdf")
要查看我們代碼範例頁面上的大綱和書籤範例,請造訪這裡。
添加和編輯註釋
IronPDF具有對PDF註釋進行大量自定義的功能。 請參考以下示例,展示其功能:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-16.cs
// create a new PDF or load and edit an existing document.
var pdf = PdfDocument.FromFile("existing.pdf");
// Create a PDF annotation object
var textAnnotation = new IronPdf.Annotations.TextAnnotation(PageIndex: 0)
{
Title = "This is the major title",
Contents = "This is the long 'sticky note' comment content...",
Subject = "This is a subtitle",
Opacity = 0.9,
Printable = false,
Hidden = false,
OpenByDefault = true,
ReadOnly = false,
Rotatable = true,
};
// Add the annotation "sticky note" to a specific page and location within any new or existing PDF.
pdf.Annotations.Add(textAnnotation);
pdf.SaveAs("existing.pdf");
' create a new PDF or load and edit an existing document.
Dim pdf = PdfDocument.FromFile("existing.pdf")
' Create a PDF annotation object
Dim textAnnotation = New IronPdf.Annotations.TextAnnotation(PageIndex:= 0) With {
.Title = "This is the major title",
.Contents = "This is the long 'sticky note' comment content...",
.Subject = "This is a subtitle",
.Opacity = 0.9,
.Printable = False,
.Hidden = False,
.OpenByDefault = True,
.ReadOnly = False,
.Rotatable = True
}
' Add the annotation "sticky note" to a specific page and location within any new or existing PDF.
pdf.Annotations.Add(textAnnotation)
pdf.SaveAs("existing.pdf")
PDF註釋允許在PDF頁面上添加類似“便利貼”的評論。 TextAnnotation
類別允許以程式方式添加註解。 支持的高級文本註釋功能包括尺寸調整、透明度、圖標和編輯功能。
添加背景和前景
使用IronPDF,我們可以輕鬆地合併2個PDF文件,使用其中一個作為背景或前景。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-17.cs
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf");
pdf.AddBackgroundPdf(@"MyBackground.pdf");
pdf.AddForegroundOverlayPdfToPage(0, @"MyForeground.pdf", 0);
pdf.SaveAs(@"C:\Path\To\Complete.pdf");
Dim renderer = New ChromePdfRenderer()
Dim pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf")
pdf.AddBackgroundPdf("MyBackground.pdf")
pdf.AddForegroundOverlayPdfToPage(0, "MyForeground.pdf", 0)
pdf.SaveAs("C:\Path\To\Complete.pdf")
學印和浮水印
任何 PDF 編輯器的基本功能包括添加印章和浮水印的能力。 IronPDF 提供了一個驚人的 API,用於創建各種印章,如圖片印章和 HTML 印章。 這些都具有高度可自訂的位置,使用對齊和偏移可以在此處看到:
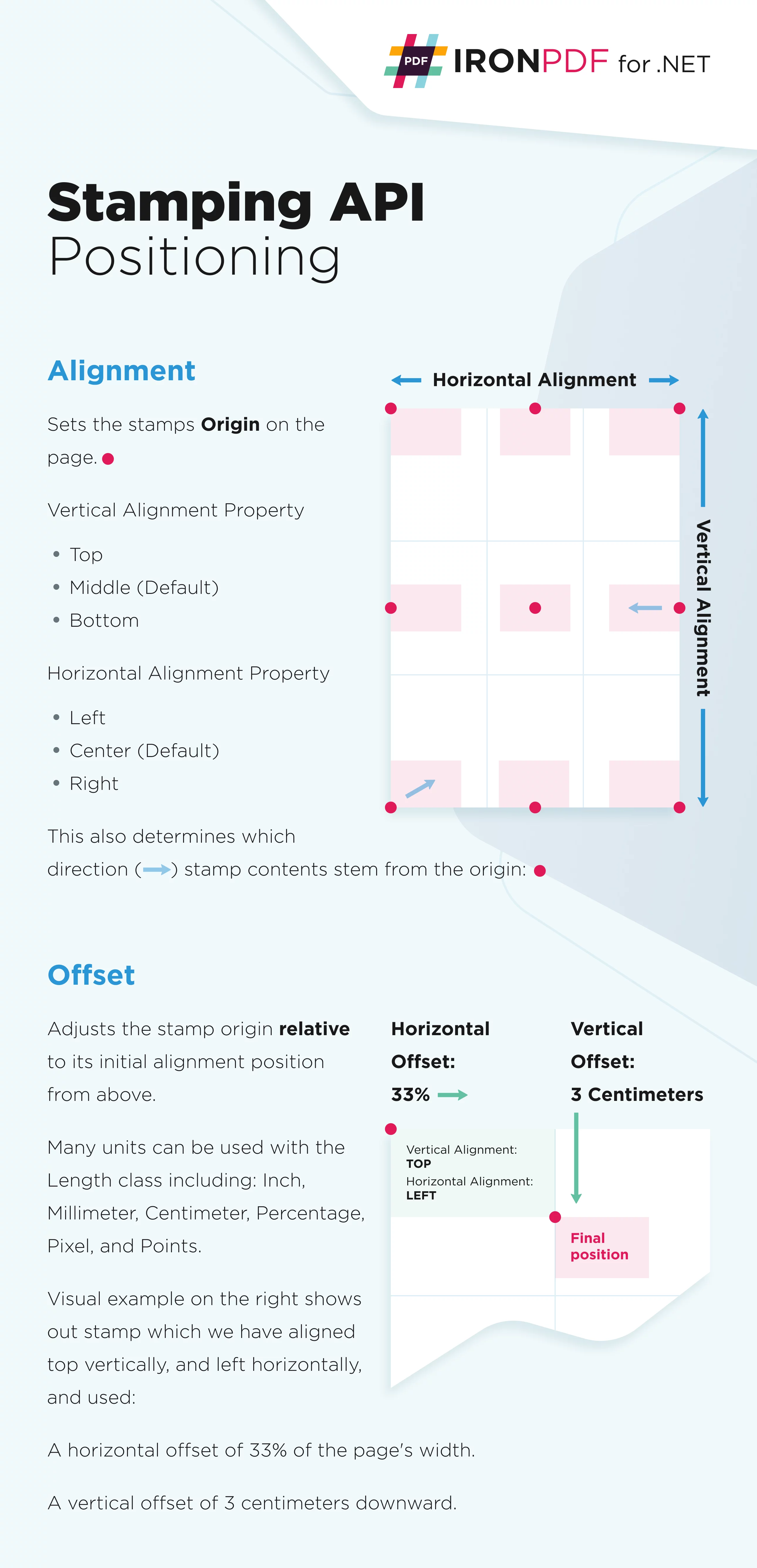
Stamper 抽象類別
Stamper
抽象類別用作 IronPDF 所有蓋章方法的參數。
每個用例都有許多類別:
TextStamper
用於加蓋文字 - 文字範例ImageStamper
用於在圖像上加蓋印章 - 圖像範例HtmlStamper
用於在 HTML 上蓋章 - HTML 示例BarcodeStamper
用於在條碼上蓋章 - 條碼範例BarcodeStamper
用於蓋上 QR 代碼 - QR 代碼範例有關浮水印,請參閱此處。
要應用其中任何一個,請使用我們的
ApplyStamp()
方法之一。 參見:本教程的應用印章部分。
Stamper 類別屬性
abstract class Stamper
└─── int : Opacity
└─── int : Rotation
└─── double : Scale
└─── Length : HorizontalOffset
└─── Length : VerticalOffset
└─── Length : MinWidth
└─── Length : MaxWidth
└─── Length : MinHeight
└─── Length : MaxHeight
└─── string : Hyperlink
└─── bool : IsStampBehindContent (default : false)
└─── HorizontalAlignment : HorizontalAlignment
│ │ Left
│ │ Center (default)
│ │ Right
│
└─── VerticalAlignment : VerticalAlignment
│ Top
│ Middle (default)
│ Bottom
蓋章範例
在下面我們展示了 Stamper 的每一個子類別以及一個程式碼範例。
將文字戳記到 PDF 上
以不同方式創建兩個不同的文字印章並同時應用它們:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-18.cs
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>");
TextStamper stamper1 = new TextStamper
{
Text = "Hello World! Stamp One Here!",
FontFamily = "Bungee Spice",
UseGoogleFont = true,
FontSize = 100,
IsBold = true,
IsItalic = true,
VerticalAlignment = VerticalAlignment.Top
};
TextStamper stamper2 = new TextStamper()
{
Text = "Hello World! Stamp Two Here!",
FontFamily = "Bungee Spice",
UseGoogleFont = true,
FontSize = 30,
VerticalAlignment = VerticalAlignment.Bottom
};
Stamper[] stampersToApply = { stamper1, stamper2 };
pdf.ApplyMultipleStamps(stampersToApply);
pdf.ApplyStamp(stamper2);
Dim renderer = New ChromePdfRenderer()
Dim pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>")
Dim stamper1 As New TextStamper With {
.Text = "Hello World! Stamp One Here!",
.FontFamily = "Bungee Spice",
.UseGoogleFont = True,
.FontSize = 100,
.IsBold = True,
.IsItalic = True,
.VerticalAlignment = VerticalAlignment.Top
}
Dim stamper2 As New TextStamper() With {
.Text = "Hello World! Stamp Two Here!",
.FontFamily = "Bungee Spice",
.UseGoogleFont = True,
.FontSize = 30,
.VerticalAlignment = VerticalAlignment.Bottom
}
Dim stampersToApply() As Stamper = { stamper1, stamper2 }
pdf.ApplyMultipleStamps(stampersToApply)
pdf.ApplyStamp(stamper2)
在 PDF 上蓋上圖片
將圖像印章應用於現有PDF文件的各種頁面組合:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-19.cs
var pdf = new PdfDocument("/attachments/2022_Q1_sales.pdf");
ImageStamper logoImageStamper = new ImageStamper("/assets/logo.png");
// Apply to every page, one page, or some pages
pdf.ApplyStamp(logoImageStamper);
pdf.ApplyStamp(logoImageStamper, 0);
pdf.ApplyStamp(logoImageStamper, new[] { 0, 3, 11 });
Dim pdf = New PdfDocument("/attachments/2022_Q1_sales.pdf")
Dim logoImageStamper As New ImageStamper("/assets/logo.png")
' Apply to every page, one page, or some pages
pdf.ApplyStamp(logoImageStamper)
pdf.ApplyStamp(logoImageStamper, 0)
pdf.ApplyStamp(logoImageStamper, { 0, 3, 11 })
將 HTML 標記到 PDF
撰寫自己的HTML以用作印章:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-20.cs
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderHtmlAsPdf("<p>Hello World, example HTML body.</p>");
HtmlStamper stamper = new HtmlStamper($"<p>Example HTML Stamped</p><div style='width:250pt;height:250pt;background-color:red;'></div>")
{
HorizontalOffset = new Length(-3, MeasurementUnit.Inch),
VerticalAlignment = VerticalAlignment.Bottom
};
pdf.ApplyStamp(stamper);
Dim renderer = New ChromePdfRenderer()
Dim pdf = renderer.RenderHtmlAsPdf("<p>Hello World, example HTML body.</p>")
Dim stamper As New HtmlStamper($"<p>Example HTML Stamped</p><div style='width:250pt;height:250pt;background-color:red;'></div>")
If True Then
'INSTANT VB WARNING: An assignment within expression was extracted from the following statement:
'ORIGINAL LINE: HorizontalOffset = new Length(-3, MeasurementUnit.Inch), VerticalAlignment = VerticalAlignment.Bottom
New Length(-3, MeasurementUnit.Inch), VerticalAlignment = VerticalAlignment.Bottom
HorizontalOffset = New Length(-3, MeasurementUnit.Inch), VerticalAlignment
End If
pdf.ApplyStamp(stamper)
將條碼加印在 PDF 上
示例:創建和蓋章條碼:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-21.cs
BarcodeStamper bcStamp = new BarcodeStamper("IronPDF", BarcodeEncoding.Code39);
bcStamp.HorizontalAlignment = HorizontalAlignment.Left;
bcStamp.VerticalAlignment = VerticalAlignment.Bottom;
var pdf = new PdfDocument("example.pdf");
pdf.ApplyStamp(bcStamp);
Dim bcStamp As New BarcodeStamper("IronPDF", BarcodeEncoding.Code39)
bcStamp.HorizontalAlignment = HorizontalAlignment.Left
bcStamp.VerticalAlignment = VerticalAlignment.Bottom
Dim pdf = New PdfDocument("example.pdf")
pdf.ApplyStamp(bcStamp)
將 QR 碼蓋章到 PDF 上
創建和蓋章 QR Code 的示例:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-22.cs
BarcodeStamper qrStamp = new BarcodeStamper("IronPDF", BarcodeEncoding.QRCode);
qrStamp.Height = 50; // pixels
qrStamp.Width = 50; // pixels
qrStamp.HorizontalAlignment = HorizontalAlignment.Left;
qrStamp.VerticalAlignment = VerticalAlignment.Bottom;
var pdf = new PdfDocument("example.pdf");
pdf.ApplyStamp(qrStamp);
Dim qrStamp As New BarcodeStamper("IronPDF", BarcodeEncoding.QRCode)
qrStamp.Height = 50 ' pixels
qrStamp.Width = 50 ' pixels
qrStamp.HorizontalAlignment = HorizontalAlignment.Left
qrStamp.VerticalAlignment = VerticalAlignment.Bottom
Dim pdf = New PdfDocument("example.pdf")
pdf.ApplyStamp(qrStamp)
在 PDF 上添加浮水印
水印是一種用於每個頁面的標記,可以輕鬆使用ApplyWatermark
方法應用:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-23.cs
var pdf = new PdfDocument("/attachments/design.pdf");
string html = "<h1> Example Title <h1/>";
int rotation = 0;
int watermarkOpacity = 30;
pdf.ApplyWatermark(html, rotation, watermarkOpacity);
Dim pdf = New PdfDocument("/attachments/design.pdf")
Dim html As String = "<h1> Example Title <h1/>"
Dim rotation As Integer = 0
Dim watermarkOpacity As Integer = 30
pdf.ApplyWatermark(html, rotation, watermarkOpacity)
如需查看我們程式碼範例頁面上的浮水印範例,請造訪此處。
將印章應用到 PDF
有幾個 ApplyStamp 方法的重載可用於將您的 Stamper 應用於 PDF。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-24.cs
var pdf = new PdfDocument("/assets/example.pdf");
// Apply one stamp to all pages
pdf.ApplyStamp(myStamper);
// Apply one stamp to a specific page
pdf.ApplyStamp(myStamper, 0);
// Apply one stamp to specific pages
pdf.ApplyStamp(myStamper, new[] { 0, 3, 5 });
// Apply a stamp array to all pages
pdf.ApplyMultipleStamps(stampArray);
// Apply a stamp array to a specific page
pdf.ApplyMultipleStamps(stampArray, 0);
// Apply a stamp array to specific pages
pdf.ApplyMultipleStamps(stampArray, new[] { 0, 3, 5 });
// And some Async versions of the above
await pdf.ApplyStampAsync(myStamper, 4);
await pdf.ApplyMultipleStampsAsync(stampArray);
// Additional Watermark apply method
string html = "<h1> Example Title <h1/>";
int rotation = 0;
int watermarkOpacity = 30;
pdf.ApplyWatermark(html, rotation, watermarkOpacity);
Dim pdf = New PdfDocument("/assets/example.pdf")
' Apply one stamp to all pages
pdf.ApplyStamp(myStamper)
' Apply one stamp to a specific page
pdf.ApplyStamp(myStamper, 0)
' Apply one stamp to specific pages
pdf.ApplyStamp(myStamper, { 0, 3, 5 })
' Apply a stamp array to all pages
pdf.ApplyMultipleStamps(stampArray)
' Apply a stamp array to a specific page
pdf.ApplyMultipleStamps(stampArray, 0)
' Apply a stamp array to specific pages
pdf.ApplyMultipleStamps(stampArray, { 0, 3, 5 })
' And some Async versions of the above
Await pdf.ApplyStampAsync(myStamper, 4)
Await pdf.ApplyMultipleStampsAsync(stampArray)
' Additional Watermark apply method
Dim html As String = "<h1> Example Title <h1/>"
Dim rotation As Integer = 0
Dim watermarkOpacity As Integer = 30
pdf.ApplyWatermark(html, rotation, watermarkOpacity)
長度等級
Length 類別有兩個屬性:Unit
和 Value
。 一旦你決定從MeasurementUnit
列舉類型中選擇使用哪個單位(默認為頁面的Percentage
),接著選擇Value
來決定使用多少倍基準單位的長度。
長度類屬性
class Length
└─── double : Value (default : 0)
└─── MeasurementUnit : Unit
Inch
Millimeter
Centimeter
Percentage (default)
Pixel
Points
長度範例
創建長度
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-25.cs
new Length(value: 5, unit: MeasurementUnit.Inch); // 5 inches
new Length(value: 25, unit: MeasurementUnit.Pixel);// 25px
new Length(); // 0% of the page dimension because value is defaulted to zero and unit is defaulted to percentage
new Length(value: 20); // 20% of the page dimension
Dim tempVar As New Length(value:= 5, unit:= MeasurementUnit.Inch) ' 5 inches
Dim tempVar2 As New Length(value:= 25, unit:= MeasurementUnit.Pixel) ' 25px
Dim tempVar3 As New Length() ' 0% of the page dimension because value is defaulted to zero and unit is defaulted to percentage
Dim tempVar4 As New Length(value:= 20) ' 20% of the page dimension
使用長度作為參數
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-26.cs
HtmlStamper logoStamper = new HtmlStamper
{
VerticalOffset = new Length(15, MeasurementUnit.Percentage),
HorizontalOffset = new Length(1, MeasurementUnit.Inch)
// set other properties...
};
Dim logoStamper As New HtmlStamper With {
.VerticalOffset = New Length(15, MeasurementUnit.Percentage),
.HorizontalOffset = New Length(1, MeasurementUnit.Inch)
}
在 PDF 中使用表單
建立與編輯表單
使用 IronPDF 創建一個包含嵌入式表單字段的 PDF:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-27.cs
// Step 1. Creating a PDF with editable forms from HTML using form and input tags
const string formHtml = @"
<html>
<body>
<h2>Editable PDF Form</h2>
<form>
First name: <br> <input type='text' name='firstname' value=''> <br>
Last name: <br> <input type='text' name='lastname' value=''>
</form>
</body>
</html>";
// Instantiate Renderer
var renderer = new ChromePdfRenderer();
renderer.RenderingOptions.CreatePdfFormsFromHtml = true;
renderer.RenderHtmlAsPdf(formHtml).SaveAs("BasicForm.pdf");
// Step 2. Reading and Writing PDF form values.
var formDocument = PdfDocument.FromFile("BasicForm.pdf");
// Read the value of the "firstname" field
var firstNameField = formDocument.Form.FindFormField("firstname");
// Read the value of the "lastname" field
var lastNameField = formDocument.Form.FindFormField("lastname");
' Step 1. Creating a PDF with editable forms from HTML using form and input tags
Const formHtml As String = "
<html>
<body>
<h2>Editable PDF Form</h2>
<form>
First name: <br> <input type='text' name='firstname' value=''> <br>
Last name: <br> <input type='text' name='lastname' value=''>
</form>
</body>
</html>"
' Instantiate Renderer
Dim renderer = New ChromePdfRenderer()
renderer.RenderingOptions.CreatePdfFormsFromHtml = True
renderer.RenderHtmlAsPdf(formHtml).SaveAs("BasicForm.pdf")
' Step 2. Reading and Writing PDF form values.
Dim formDocument = PdfDocument.FromFile("BasicForm.pdf")
' Read the value of the "firstname" field
Dim firstNameField = formDocument.Form.FindFormField("firstname")
' Read the value of the "lastname" field
Dim lastNameField = formDocument.Form.FindFormField("lastname")
如需查看我們程式碼範例頁面上的 PDF 表單範例,請造訪這裡。
填寫現有表單
使用IronPDF,您可以輕鬆訪問PDF中的所有現有表單字段並填寫它們以便重新保存:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-28.cs
var formDocument = PdfDocument.FromFile("BasicForm.pdf");
// Set and Read the value of the "firstname" field
var firstNameField = formDocument.Form.FindFormField("firstname");
firstNameField.Value = "Minnie";
Console.WriteLine("FirstNameField value: {0}", firstNameField.Value);
// Set and Read the value of the "lastname" field
var lastNameField = formDocument.Form.FindFormField("lastname");
lastNameField.Value = "Mouse";
Console.WriteLine("LastNameField value: {0}", lastNameField.Value);
formDocument.SaveAs("FilledForm.pdf");
Dim formDocument = PdfDocument.FromFile("BasicForm.pdf")
' Set and Read the value of the "firstname" field
Dim firstNameField = formDocument.Form.FindFormField("firstname")
firstNameField.Value = "Minnie"
Console.WriteLine("FirstNameField value: {0}", firstNameField.Value)
' Set and Read the value of the "lastname" field
Dim lastNameField = formDocument.Form.FindFormField("lastname")
lastNameField.Value = "Mouse"
Console.WriteLine("LastNameField value: {0}", lastNameField.Value)
formDocument.SaveAs("FilledForm.pdf")
如需查看我們程式碼範例頁面上的 PDF 表單範例,請造訪這裡。
結論
上述示例列表表明,IronPDF 在編輯 PDF 時具有即開即用的關鍵功能。
如果您想提出功能請求或對IronPDF或授權有任何一般問題,請聯繫我們的支持團隊。 我们将非常乐意协助您。