C# 打印 PDF 文档
您可以在 .NET 应用程序中借助 Visual Basic 或 C# 代码轻松打印 PDF 文件。 本教程将指导您如何使用C#打印PDF的功能来进行编程打印。
如何用 C# 打印 PDF 文件
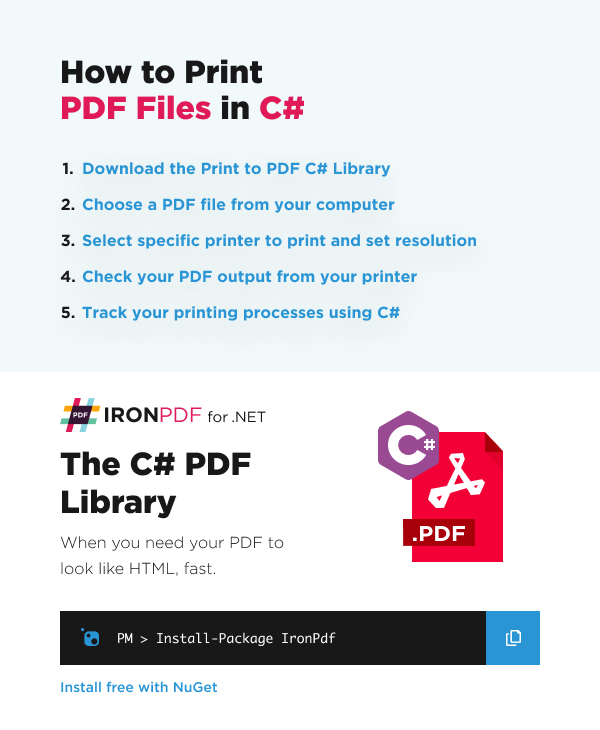
- 下载打印为 PDF 的 C# 库
- 从电脑中选择 PDF 文件
- 选择要打印的特定打印机并设置分辨率
- 检查打印机的 PDF 输出结果
- 使用 C# 跟踪您的印刷流程
立即在您的项目中开始使用IronPDF,并享受免费试用。
创建 PDF 并打印
您可以将 PDF 文档直接静默发送到打印机或创建一个System.Drawing.Printing.PrintDocument对象,可以进行操作并发送到GUI打印对话框。
以下代码可用于两个选项:
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-create-and-print-pdf.cs
using IronPdf;
using System.Threading.Tasks;
// Create a new PDF and print it
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf");
// Print PDF from default printer
await pdf.Print();
// For advanced silent real-world printing options, use PdfDocument.GetPrintDocument
// Remember to add an assembly reference to System.Drawing.dll
System.Drawing.Printing.PrintDocument PrintDocYouCanWorkWith = pdf.GetPrintDocument();
Imports IronPdf
Imports System.Threading.Tasks
' Create a new PDF and print it
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf")
' Print PDF from default printer
Await pdf.Print()
' For advanced silent real-world printing options, use PdfDocument.GetPrintDocument
' Remember to add an assembly reference to System.Drawing.dll
Dim PrintDocYouCanWorkWith As System.Drawing.Printing.PrintDocument = pdf.GetPrintDocument()
高级打印
IronPDF非常擅长处理高级打印功能,例如查找打印机名称或设置打印机以及设置打印机分辨率。
指定打印机名称
要指定打印机名称,您需要做的就是获取当前的打印文档对象。(使用PDF 文档的 GetPrintDocument 方法PDF 文档)然后使用 PrinterSettings.PrinterName 属性,如下所示:
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-specify-printer-name.cs
using IronPdf;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
// Get PrintDocument object
var printDocument = pdf.GetPrintDocument();
// Assign the printer name
printDocument.PrinterSettings.PrinterName = "Microsoft Print to PDF";
// Print document
printDocument.Print();
Imports IronPdf
Private pdf As PdfDocument = PdfDocument.FromFile("sample.pdf")
' Get PrintDocument object
Private printDocument = pdf.GetPrintDocument()
' Assign the printer name
printDocument.PrinterSettings.PrinterName = "Microsoft Print to PDF"
' Print document
printDocument.Print()
设置打印机分辨率
分辨率是指被打印或显示的像素数量,具体取决于您的输出。 您可以使用默认页面设置.打印机分辨率 "属性的 PDF 文档。 这是一个非常快速的演示:
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-specify-printer-resolution.cs
using IronPdf;
using System.Drawing.Printing;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
// Get PrintDocument object
var printDocument = pdf.GetPrintDocument();
// Set printer resolution
printDocument.DefaultPageSettings.PrinterResolution = new PrinterResolution
{
Kind = PrinterResolutionKind.Custom,
X = 1200,
Y = 1200
};
// Print document
printDocument.Print();
Imports IronPdf
Imports System.Drawing.Printing
Private pdf As PdfDocument = PdfDocument.FromFile("sample.pdf")
' Get PrintDocument object
Private printDocument = pdf.GetPrintDocument()
' Set printer resolution
printDocument.DefaultPageSettings.PrinterResolution = New PrinterResolution With {
.Kind = PrinterResolutionKind.Custom,
.X = 1200,
.Y = 1200
}
' Print document
printDocument.Print()
如您所见,我已将分辨率设置为自定义级别:纵向 1200,横向 1200。
PrintToFile 方法
PdfDocument.PrintToFile
方法允许您将 PDF 打印到文件。您只需提供输出文件路径并指定是否希望看到预览。 下面的代码将打印到指定文件,但不包括预览:
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-printtofile.cs
using IronPdf;
using System.Threading.Tasks;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
await pdf.PrintToFile("PathToFile", false);
Imports IronPdf
Imports System.Threading.Tasks
Private pdf As PdfDocument = PdfDocument.FromFile("sample.pdf")
Await pdf.PrintToFile("PathToFile", False)
使用 C# 跟踪打印过程
C# 与 IronPDF 结合的妙处在于,当涉及到跟踪打印页面或任何与打印相关的内容时,其实非常简单。 在下一个示例中,我将演示如何更改打印机的名称、分辨率以及如何计算打印的页数。
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-trace-printing-process.cs
using IronPdf;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
// Get PrintDocument object
var printDocument = pdf.GetPrintDocument();
// Subscribe to the PrintPage event
var printedPages = 0;
printDocument.PrintPage += (sender, args) => printedPages++;
// Print document
printDocument.Print();
Imports IronPdf
Private pdf As PdfDocument = PdfDocument.FromFile("sample.pdf")
' Get PrintDocument object
Private printDocument = pdf.GetPrintDocument()
' Subscribe to the PrintPage event
Private printedPages = 0
Private printDocument.PrintPage += Sub(sender, args) printedPages++
' Print document
printDocument.Print()