如何在 Blazor 服务器中将 Razor 转换为 PDF
Razor 组件是一种用户界面元素,例如页面、对话框或数据输入表单,使用 C# 和 Razor 语法构建。 它作为可重复使用的UI组件。
Blazor Server 是一个网页框架,允许您使用 C# 而不是 JavaScript 来构建交互式网页用户界面。 在此框架中,组件的逻辑在服务器上运行。
IronPDF允许您在Blazor Server项目或应用程序中从Razor组件生成PDF文档。 这使得在Blazor Server中创建PDF文件/页面变得简单直接。
如何将 Razor 组件转换为 PDF
IronPDF 扩展包
IronPdf.Extensions.Blazor 包是主要 IronPdf 包的一个扩展。 在Blazor Server应用程序中将Razor组件渲染为PDF文档需要使用IronPdf.Extensions.Blazor和IronPdf包。
PM > Install-Package IronPdf.Extensions.Blazor
安装使用 NuGet
安装包 IronPdf.Extensions.Blazor
将 Razor 组件渲染为 PDFs
需要一个Blazor Server App项目来将Razor组件转换为PDF。
添加一个模型类
添加一个标准的C#类,并将其命名为 PersonInfo。 此类将用作存储个人信息的模型。 插入以下代码:
:path=/static-assets/pdf/content-code-examples/how-to/razor-to-pdf-blazor-server-model.cs
namespace BlazorSample.Data
{
public class PersonInfo
{
public int Id { get; set; }
public string Name { get; set; }
public string Title { get; set; }
public string Description { get; set; }
}
}
Namespace BlazorSample.Data
Public Class PersonInfo
Public Property Id() As Integer
Public Property Name() As String
Public Property Title() As String
Public Property Description() As String
End Class
End Namespace
添加一个 Razor 组件
使用RenderRazorComponentToPdf
方法将Razor组件转换为PDFs。 通过实例化 ChromePdfRenderer 类来访问这个方法。 该方法返回一个PdfDocument对象,您可以使用它来导出PDF文档或进一步修改它。
返回的 PdfDocument 可以进行额外的修改,例如转换为PDF/A或PDF/UA格式。 您还可以分合旋转 PDF 文档页面,并添加注释或书签. 自定义水印也可以盖在您的PDF上。
添加一个 Razor 组件并命名为 Person。 输入以下代码:
@page "/Person"
@using BlazorSample.Data;
@using IronPdf;
@using IronPdf.Extensions.Blazor;
<h3>Person</h3>
@code {
[Parameter]
public IEnumerable<PersonInfo> persons { get; set; }
public Dictionary<string, object> Parameters { get; set; } = new Dictionary<string, object>();
protected override async Task OnInitializedAsync()
{
persons = new List<PersonInfo>
{
new PersonInfo { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new PersonInfo { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new PersonInfo { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
}
private async void PrintToPdf()
{
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Apply text footer
renderer.RenderingOptions.TextFooter = new TextHeaderFooter()
{
LeftText = "{date} - {time}",
DrawDividerLine = true,
RightText = "Page {page} of {total-pages}",
Font = IronSoftware.Drawing.FontTypes.Arial,
FontSize = 11
};
Parameters.Add("persons", persons);
// Render razor component to PDF
PdfDocument pdf = renderer.RenderRazorComponentToPdf<Person>(Parameters);
File.WriteAllBytes("razorComponentToPdf.pdf", pdf.BinaryData);
}
}
<table class="table">
<tr>
<th>Name</th>
<th>Title</th>
<th>Description</th>
</tr>
@foreach (var person in persons)
{
<tr>
<td>@person.Name</td>
<td>@person.Title</td>
<td>@person.Description</td>
</tr>
}
</table>
<button class="btn btn-primary" @onclick="PrintToPdf">Print to Pdf</button>
@page "/Person"
@using BlazorSample.Data;
@using IronPdf;
@using IronPdf.Extensions.Blazor;
<h3>Person</h3>
@code {
[Parameter]
public IEnumerable<PersonInfo> persons { get; set; }
public Dictionary<string, object> Parameters { get; set; } = new Dictionary<string, object>();
protected override async Task OnInitializedAsync()
{
persons = new List<PersonInfo>
{
new PersonInfo { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new PersonInfo { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new PersonInfo { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
}
private async void PrintToPdf()
{
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Apply text footer
renderer.RenderingOptions.TextFooter = new TextHeaderFooter()
{
LeftText = "{date} - {time}",
DrawDividerLine = true,
RightText = "Page {page} of {total-pages}",
Font = IronSoftware.Drawing.FontTypes.Arial,
FontSize = 11
};
Parameters.Add("persons", persons);
// Render razor component to PDF
PdfDocument pdf = renderer.RenderRazorComponentToPdf<Person>(Parameters);
File.WriteAllBytes("razorComponentToPdf.pdf", pdf.BinaryData);
}
}
<table class="table">
<tr>
<th>Name</th>
<th>Title</th>
<th>Description</th>
</tr>
@foreach (var person in persons)
{
<tr>
<td>@person.Name</td>
<td>@person.Title</td>
<td>@person.Description</td>
</tr>
}
</table>
<button class="btn btn-primary" @onclick="PrintToPdf">Print to Pdf</button>
Private page "/Person" [using] BlazorSample.Data
Private IronPdf As [using]
Private IronPdf As [using]
'INSTANT VB TODO TASK: The following line could not be converted:
(Of h3) Person</h3> code
If True Then
<Parameter>
public IEnumerable(Of PersonInfo) persons {get;set;}
public Dictionary(Of String, Object) Parameters {get;set;} = New Dictionary(Of String, Object)()
'INSTANT VB TODO TASK: Local functions are not converted by Instant VB:
' protected override async Task OnInitializedAsync()
' {
' persons = New List<PersonInfo> { New PersonInfo { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" }, New PersonInfo { Name = "Bob", Title = "Mr.", Description = "Software Engineer" }, New PersonInfo { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" } };
' }
'INSTANT VB TODO TASK: Local functions are not converted by Instant VB:
' private async void PrintToPdf()
' {
' ChromePdfRenderer renderer = New ChromePdfRenderer();
'
' ' Apply text footer
' renderer.RenderingOptions.TextFooter = New TextHeaderFooter() { LeftText = "{date} - {time}", DrawDividerLine = True, RightText = "Page {page} of {total-pages}", Font = IronSoftware.Drawing.FontTypes.Arial, FontSize = 11 };
'
' Parameters.Add("persons", persons);
'
' ' Render razor component to PDF
' PdfDocument pdf = renderer.RenderRazorComponentToPdf<Person>(Parameters);
'
' File.WriteAllBytes("razorComponentToPdf.pdf", pdf.BinaryData);
' }
End If
<table class="table"> (Of tr) (Of th) Name</th> (Of th) Title</th> (Of th) Description</th> </tr> foreach(var person in persons)
If True Then
(Of tr) (Of td) person.Name</td> (Of td) person.Title</td> (Of td) person.Description</td> </tr>
End If
'INSTANT VB TODO TASK: The following line uses invalid syntax:
'</table> <button class="btn btn-primary" @onclick="PrintToPdf"> Print @to Pdf</button>
此外,使用这种方法生成 PDF,您可以完全访问 RenderingOptions 中的所有功能。 这包括插入的能力文本以及 HTML 页眉和页脚. 此外,您可以添加页码并根据自己的喜好调整页面尺寸和布局。
向左侧菜单添加一个部分
- 导航到“共享文件夹”,并打开 NavMenu.razor。
- 在我们的Razor组件中添加一个打开Person部分的代码。 我们的Person组件将是第二个选项。
<div class="@NavMenuCssClass" @onclick="ToggleNavMenu">
<nav class="flex-column">
<div class="nav-item px-3">
<NavLink class="nav-link" href="" Match="NavLinkMatch.All">
<span class="oi oi-home" aria-hidden="true"></span> Home
</NavLink>
</div>
<div class="nav-item px-3">
<NavLink class="nav-link" href="Person">
<span class="oi oi-list-rich" aria-hidden="true"></span> Person
</NavLink>
</div>
<div class="nav-item px-3">
<NavLink class="nav-link" href="counter">
<span class="oi oi-plus" aria-hidden="true"></span> Counter
</NavLink>
</div>
<div class="nav-item px-3">
<NavLink class="nav-link" href="fetchdata">
<span class="oi oi-list-rich" aria-hidden="true"></span> Fetch data
</NavLink>
</div>
</nav>
</div>
<div class="@NavMenuCssClass" @onclick="ToggleNavMenu">
<nav class="flex-column">
<div class="nav-item px-3">
<NavLink class="nav-link" href="" Match="NavLinkMatch.All">
<span class="oi oi-home" aria-hidden="true"></span> Home
</NavLink>
</div>
<div class="nav-item px-3">
<NavLink class="nav-link" href="Person">
<span class="oi oi-list-rich" aria-hidden="true"></span> Person
</NavLink>
</div>
<div class="nav-item px-3">
<NavLink class="nav-link" href="counter">
<span class="oi oi-plus" aria-hidden="true"></span> Counter
</NavLink>
</div>
<div class="nav-item px-3">
<NavLink class="nav-link" href="fetchdata">
<span class="oi oi-list-rich" aria-hidden="true"></span> Fetch data
</NavLink>
</div>
</nav>
</div>
运行项目
这将向您展示如何运行项目并生成PDF文档。
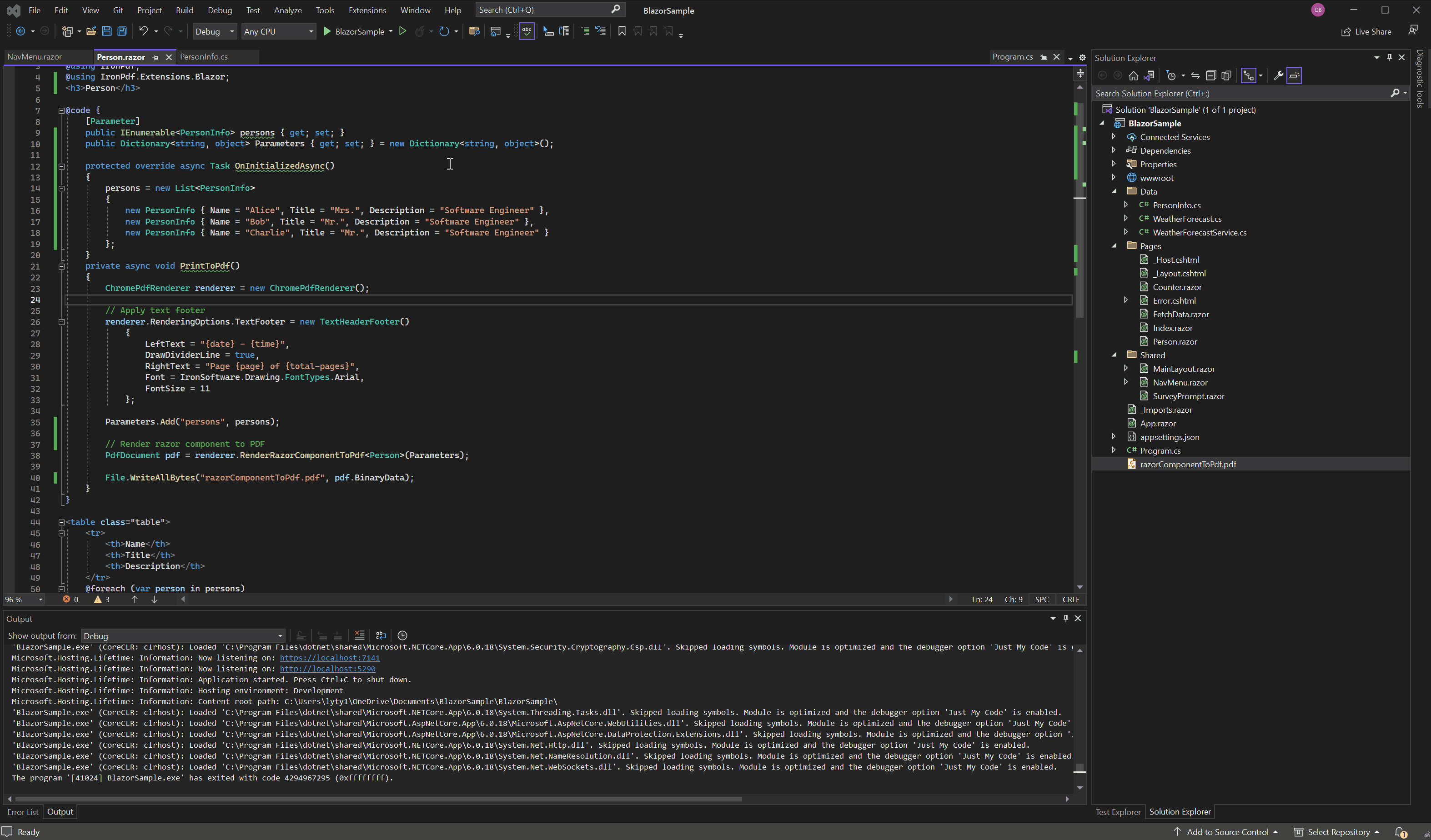
下载 Blazor 服务器应用项目
您可以下载本指南的完整代码。它以压缩文件的形式提供,您可以在Visual Studio中将其打开为Blazor Server App项目。