C#でPDFを編集する方法
イントロダクション
Iron Softwareは、IronPDFライブラリに多くの異なるPDF編集機能を簡単に読みやすく理解しやすいメソッドに簡略化しました。 署名の追加、HTMLフッターの追加、ウォーターマークのスタンプ、または注釈の追加など。 IronPDFは、お客様のためのツールであり、読みやすいコード、プログラムによるPDF生成、簡単なデバッグ、およびサポートされているあらゆる環境やプラットフォームへの容易なデプロイを可能にします。
IronPDFには、PDFの編集に関して無数の機能があります。 このチュートリアル記事では、主要なもののいくつかをコード例と説明を交えながら解説します。
この記事を読むことで、C#でIronPDFを使用してPDFを編集する方法について十分に理解できるようになります。
目次
-
ドキュメント構造の編集
- PDFのマージと分割
-
ドキュメントプロパティの編集
- PDFファイルを圧縮する
-
PDFコンテンツを編集する
- 背景と前景を追加
-
スタンピングとウォーターマーキング
もちろん、提供された内容は空白ですので、翻訳するテキストはありません。翻訳したい具体的なコンテンツを更新してください。その際に正確に翻訳させていただきます。スタンパークラスのプロパティ
もちろん、提供された内容は空白ですので、翻訳するテキストはありません。翻訳したい具体的なコンテンツを更新してください。その際に正確に翻訳させていただきます。PDFにテキストをスタンプする
もちろん、提供された内容は空白ですので、翻訳するテキストはありません。翻訳したい具体的なコンテンツを更新してください。その際に正確に翻訳させていただきます。PDFに画像をスタンプする
もちろん、提供された内容は空白ですので、翻訳するテキストはありません。翻訳したい具体的なコンテンツを更新してください。その際に正確に翻訳させていただきます。HTMLをPDFにスタンプする
もちろん、提供された内容は空白ですので、翻訳するテキストはありません。翻訳したい具体的なコンテンツを更新してください。その際に正確に翻訳させていただきます。PDFにバーコードをスタンプする
もちろん、提供された内容は空白ですので、翻訳するテキストはありません。翻訳したい具体的なコンテンツを更新してください。その際に正確に翻訳させていただきます。PDFにQRコードをスタンプする
もちろん、提供された内容は空白ですので、翻訳するテキストはありません。翻訳したい具体的なコンテンツを更新してください。その際に正確に翻訳させていただきます。PDFにウォーターマークを追加する
もちろん、提供された内容は空白ですので、翻訳するテキストはありません。翻訳したい具体的なコンテンツを更新してください。その際に正確に翻訳させていただきます。長さクラスのプロパティ
もちろん、提供された内容は空白ですので、翻訳するテキストはありません。翻訳したい具体的なコンテンツを更新してください。その際に正確に翻訳させていただきます。コード例の長さ
-
PDFでフォームの使用
今日から無料トライアルでIronPDFをあなたのプロジェクトで使い始めましょう。
ドキュメント構造の編集
ページを操作
特定のインデックスでPDFを追加したり、ページを範囲または1ページずつコピーしたり、任意のPDFからページを削除したりすることは、IronPDFを使用すれば非常に簡単です。IronPDFはバックグラウンドでそれらすべてを処理します。
ページを追加
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-1.cs
var pdf = new PdfDocument("report.pdf");
var renderer = new ChromePdfRenderer();
var coverPagePdf = renderer.RenderHtmlAsPdf("<h1>Cover Page</h1><hr>");
pdf.PrependPdf(coverPagePdf);
pdf.SaveAs("report_with_cover.pdf");
Dim pdf = New PdfDocument("report.pdf")
Dim renderer = New ChromePdfRenderer()
Dim coverPagePdf = renderer.RenderHtmlAsPdf("<h1>Cover Page</h1><hr>")
pdf.PrependPdf(coverPagePdf)
pdf.SaveAs("report_with_cover.pdf")
ページをコピー
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-2.cs
var pdf = new PdfDocument("report.pdf");
// Copy pages 5 to 7 and save them as a new document.
pdf.CopyPages(4, 6).SaveAs("report_highlight.pdf");
Dim pdf = New PdfDocument("report.pdf")
' Copy pages 5 to 7 and save them as a new document.
pdf.CopyPages(4, 6).SaveAs("report_highlight.pdf")
ページを削除
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-3.cs
var pdf = new PdfDocument("report.pdf");
// Remove the last page from the PDF and save again
pdf.RemovePage(pdf.PageCount - 1);
pdf.SaveAs("report_minus_one_page.pdf");
Dim pdf = New PdfDocument("report.pdf")
' Remove the last page from the PDF and save again
pdf.RemovePage(pdf.PageCount - 1)
pdf.SaveAs("report_minus_one_page.pdf")
PDFのマージと分割
PDFを1つのPDFに結合したり、既存のPDFを分割したりすることは、IronPDFの直感的なAPIを使用すれば簡単に達成できます。
複数の既存のPDFを1つのPDFドキュメントに結合
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-4.cs
var pdfs = new List<PdfDocument>
{
PdfDocument.FromFile("A.pdf"),
PdfDocument.FromFile("B.pdf"),
PdfDocument.FromFile("C.pdf")
};
PdfDocument mergedPdf = PdfDocument.Merge(pdfs);
mergedPdf.SaveAs("merged.pdf");
foreach (var pdf in pdfs)
{
pdf.Dispose();
}
Dim pdfs = New List(Of PdfDocument) From {PdfDocument.FromFile("A.pdf"), PdfDocument.FromFile("B.pdf"), PdfDocument.FromFile("C.pdf")}
Dim mergedPdf As PdfDocument = PdfDocument.Merge(pdfs)
mergedPdf.SaveAs("merged.pdf")
For Each pdf In pdfs
pdf.Dispose()
Next pdf
コードサンプルページで2つ以上のPDFを結合する方法を確認するには、こちらをご覧ください:これ.
PDFを分割してページを抽出
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-5.cs
var pdf = new PdfDocument("sample.pdf");
// Take the first page
var pdf_page1 = pdf.CopyPage(0);
pdf_page1.SaveAs("Split1.pdf");
// Take the pages 2 & 3
var pdf_page2_3 = pdf.CopyPages(1, 2);
pdf_page2_3.SaveAs("Spli2t.pdf");
Dim pdf = New PdfDocument("sample.pdf")
' Take the first page
Dim pdf_page1 = pdf.CopyPage(0)
pdf_page1.SaveAs("Split1.pdf")
' Take the pages 2 & 3
Dim pdf_page2_3 = pdf.CopyPages(1, 2)
pdf_page2_3.SaveAs("Spli2t.pdf")
コード例ページでページの分割と抽出を見るには、以下をご覧ください:これ.
ドキュメントプロパティの編集
PDFメタデータの追加と使用
IronPDFを使用して、PDFメタデータを簡単に閲覧および編集できます。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-6.cs
// Open an Encrypted File, alternatively create a new PDF from Html
var pdf = PdfDocument.FromFile("encrypted.pdf", "password");
// Edit file metadata
pdf.MetaData.Author = "Satoshi Nakamoto";
pdf.MetaData.Keywords = "SEO, Friendly";
pdf.MetaData.ModifiedDate = System.DateTime.Now;
// Edit file security settings
// The following code makes a PDF read only and will disallow copy & paste and printing
pdf.SecuritySettings.RemovePasswordsAndEncryption();
pdf.SecuritySettings.MakePdfDocumentReadOnly("secret-key");
pdf.SecuritySettings.AllowUserAnnotations = false;
pdf.SecuritySettings.AllowUserCopyPasteContent = false;
pdf.SecuritySettings.AllowUserFormData = false;
pdf.SecuritySettings.AllowUserPrinting = IronPdf.Security.PdfPrintSecurity.FullPrintRights;
// Change or set the document encryption password
pdf.SecuritySettings.OwnerPassword = "top-secret"; // password to edit the pdf
pdf.SecuritySettings.UserPassword = "shareable"; // password to open the pdf
pdf.SaveAs("secured.pdf");
Imports System
' Open an Encrypted File, alternatively create a new PDF from Html
Dim pdf = PdfDocument.FromFile("encrypted.pdf", "password")
' Edit file metadata
pdf.MetaData.Author = "Satoshi Nakamoto"
pdf.MetaData.Keywords = "SEO, Friendly"
pdf.MetaData.ModifiedDate = DateTime.Now
' Edit file security settings
' The following code makes a PDF read only and will disallow copy & paste and printing
pdf.SecuritySettings.RemovePasswordsAndEncryption()
pdf.SecuritySettings.MakePdfDocumentReadOnly("secret-key")
pdf.SecuritySettings.AllowUserAnnotations = False
pdf.SecuritySettings.AllowUserCopyPasteContent = False
pdf.SecuritySettings.AllowUserFormData = False
pdf.SecuritySettings.AllowUserPrinting = IronPdf.Security.PdfPrintSecurity.FullPrintRights
' Change or set the document encryption password
pdf.SecuritySettings.OwnerPassword = "top-secret" ' password to edit the pdf
pdf.SecuritySettings.UserPassword = "shareable" ' password to open the pdf
pdf.SaveAs("secured.pdf")
デジタル署名
IronPDFには、新規または既存のPDFファイルを.pfxおよび.p12 X509Certificate2デジタル証明書を使用してデジタル署名するオプションがあります。
PDFファイルが署名されると、証明書が検証されない限り変更することはできません。 これにより忠実性が保証されます。
無料で署名証明書を生成するために、Adobe Readerを使用してください。詳細はhttps://helpx.adobe.com/acrobat/using/digital-ids.htmlをご覧ください。
暗号署名に加えて、手書き署名画像や会社の印鑑画像もIronPDFを使用して署名するために使用できます。
既存のPDFに1行のコードで暗号署名を追加!
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-7.cs
using IronPdf;
using IronPdf.Signing;
new IronPdf.Signing.PdfSignature("Iron.p12", "123456").SignPdfFile("any.pdf");
Imports IronPdf
Imports IronPdf.Signing
Call (New IronPdf.Signing.PdfSignature("Iron.p12", "123456")).SignPdfFile("any.pdf")
より高度な制御が可能な例:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-8.cs
using IronPdf;
// Step 1. Create a PDF
var renderer = new ChromePdfRenderer();
var doc = renderer.RenderHtmlAsPdf("<h1>Testing 2048 bit digital security</h1>");
// Step 2. Create a Signature.
// You may create a .pfx or .p12 PDF signing certificate using Adobe Acrobat Reader.
// Read: https://helpx.adobe.com/acrobat/using/digital-ids.html
var signature = new IronPdf.Signing.PdfSignature("Iron.pfx", "123456")
{
// Step 3. Optional signing options and a handwritten signature graphic
SigningContact = "support@ironsoftware.com",
SigningLocation = "Chicago, USA",
SigningReason = "To show how to sign a PDF"
};
//Step 4. Sign the PDF with the PdfSignature. Multiple signing certificates may be used
doc.Sign(signature);
//Step 5. The PDF is not signed until saved to file, steam or byte array.
doc.SaveAs("signed.pdf");
Imports IronPdf
' Step 1. Create a PDF
Private renderer = New ChromePdfRenderer()
Private doc = renderer.RenderHtmlAsPdf("<h1>Testing 2048 bit digital security</h1>")
' Step 2. Create a Signature.
' You may create a .pfx or .p12 PDF signing certificate using Adobe Acrobat Reader.
' Read: https://helpx.adobe.com/acrobat/using/digital-ids.html
Private signature = New IronPdf.Signing.PdfSignature("Iron.pfx", "123456") With {
.SigningContact = "support@ironsoftware.com",
.SigningLocation = "Chicago, USA",
.SigningReason = "To show how to sign a PDF"
}
'Step 4. Sign the PDF with the PdfSignature. Multiple signing certificates may be used
doc.Sign(signature)
'Step 5. The PDF is not signed until saved to file, steam or byte array.
doc.SaveAs("signed.pdf")
PDF添付ファイル
IronPDFは、PDFドキュメントへの添付ファイルの追加および削除を完全にサポートしています。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-9.cs
var Renderer = new ChromePdfRenderer();
var myPdf = Renderer.RenderHtmlFileAsPdf("my-content.html");
// Here we can add an attachment with a name and byte[]
var attachment1 = myPdf.Attachments.AddAttachment("attachment_1", example_attachment);
// And here is an example of removing an attachment
myPdf.Attachments.RemoveAttachment(attachment1);
myPdf.SaveAs("my-content.pdf");
Dim Renderer = New ChromePdfRenderer()
Dim myPdf = Renderer.RenderHtmlFileAsPdf("my-content.html")
' Here we can add an attachment with a name and byte[]
Dim attachment1 = myPdf.Attachments.AddAttachment("attachment_1", example_attachment)
' And here is an example of removing an attachment
myPdf.Attachments.RemoveAttachment(attachment1)
myPdf.SaveAs("my-content.pdf")
PDFファイルを圧縮
IronPDFはPDFの圧縮をサポートしています。 PDFファイルのサイズを削減する一つの方法は、PdfDocument内に埋め込まれた画像のサイズを縮小することです。 IronPDFでは、CompressImages
メソッドを呼び出すことができます。
JPEGのリサイズの仕組みにおいて、100%の品質ではほとんど損失がなく、1%は非常に低品質な出力画像となります。 一般的に、90%以上は高品質とみなされており、80%から90%は中品質、70%から80%は低品質とみなされます。 70%以下に減らすと低品質の画像が生成される可能性がありますが、これによりPdfDocumentのファイルサイズが大幅に削減される可能性があります。
さまざまな値を試していただき、品質とファイルサイズのバランスを最適にするための範囲を把握してください。 画質の低下の明白さは、最終的には入力された画像の種類によります。一部の画像は他の画像よりも著しく鮮明さが低下する場合があります。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-10.cs
var pdf = new PdfDocument("document.pdf");
// Quality parameter can be 1-100, where 100 is 100% of original quality
pdf.CompressImages(60);
pdf.SaveAs("document_compressed.pdf");
Dim pdf = New PdfDocument("document.pdf")
' Quality parameter can be 1-100, where 100 is 100% of original quality
pdf.CompressImages(60)
pdf.SaveAs("document_compressed.pdf")
PDFドキュメント内の目に見えるサイズに応じて画像の解像度を縮小できる、もう1つのオプションパラメーターがあります。 一部の画像構成で歪みが生じる可能性がありますので、ご注意ください。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-11.cs
var pdf = new PdfDocument("document.pdf");
pdf.CompressImages(90, true);
pdf.SaveAs("document_scaled_compressed.pdf");
Dim pdf = New PdfDocument("document.pdf")
pdf.CompressImages(90, True)
pdf.SaveAs("document_scaled_compressed.pdf")
PDFコンテンツの編集
ヘッダーとフッターの追加
PDFに簡単にヘッダーとフッターを追加できます。 IronPDFには、TextHeaderFooter
とHtmlHeaderFooter
という2種類の「HeaderFooters」があります。 TextHeaderFooterは、テキストのみを含むヘッダーおよびフッターに適しており、たとえば `" といったマージフィールドを使用したい場合に最適です。{ページ}以下の内容を日本語に翻訳してください:
{総ページ数}申し訳ありませんが、翻訳するための具体的なテキストが提供されていません。翻訳が必要なテキストを入力してください。 HtmlHeaderFooter は、任意のHTMLに対応し、きれいにフォーマットされる高度なヘッダーおよびフッターです。
HTMLヘッダーとフッター
HTMLヘッダーとフッターは、HTMLのレンダリングされたバージョンを使用して、ピクセルパーフェクトなレイアウトでPDFドキュメントのヘッダーまたはフッターとして使用されます。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-12.cs
var renderer = new ChromePdfRenderer();
// Build a footer using html to style the text
// mergeable fields are:
// {page} {total-pages} {url} {date} {time} {html-title} & {pdf-title}
renderer.RenderingOptions.HtmlFooter = new HtmlHeaderFooter()
{
MaxHeight = 15, //millimeters
HtmlFragment = "<center><i>{page} of {total-pages}<i></center>",
DrawDividerLine = true
};
// Build a header using an image asset
// Note the use of BaseUrl to set a relative path to the assets
renderer.RenderingOptions.HtmlHeader = new HtmlHeaderFooter()
{
MaxHeight = 20, //millimeters
HtmlFragment = "<img src='logo.png'>",
BaseUrl = new System.Uri(@"C:\assets\images").AbsoluteUri
};
Dim renderer = New ChromePdfRenderer()
' Build a footer using html to style the text
' mergeable fields are:
' {page} {total-pages} {url} {date} {time} {html-title} & {pdf-title}
renderer.RenderingOptions.HtmlFooter = New HtmlHeaderFooter() With {
.MaxHeight = 15,
.HtmlFragment = "<center><i>{page} of {total-pages}<i></center>",
.DrawDividerLine = True
}
' Build a header using an image asset
' Note the use of BaseUrl to set a relative path to the assets
renderer.RenderingOptions.HtmlHeader = New HtmlHeaderFooter() With {
.MaxHeight = 20,
.HtmlFragment = "<img src='logo.png'>",
.BaseUrl = (New System.Uri("C:\assets\images")).AbsoluteUri
}
複数のユースケースを含む詳細な例については、以下のチュートリアルをご覧ください:これ.
テキストヘッダーとフッター
基本的なヘッダーとフッターはTextHeaderFooterです。以下の例をご覧ください。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-13.cs
var renderer = new ChromePdfRenderer
{
RenderingOptions =
{
FirstPageNumber = 1, // use 2 if a cover-page will be appended
// Add a header to every page easily:
TextHeader =
{
DrawDividerLine = true,
CenterText = "{url}",
Font = IronSoftware.Drawing.FontTypes.Helvetica,
FontSize = 12
},
// Add a footer too:
TextFooter =
{
DrawDividerLine = true,
Font = IronSoftware.Drawing.FontTypes.Arial,
FontSize = 10,
LeftText = "{date} {time}",
RightText = "{page} of {total-pages}"
}
}
};
Dim renderer = New ChromePdfRenderer With {
.RenderingOptions = {
FirstPageNumber = 1, TextHeader = {
DrawDividerLine = True,
CenterText = "{url}",
Font = IronSoftware.Drawing.FontTypes.Helvetica,
FontSize = 12
},
TextFooter = {
DrawDividerLine = True,
Font = IronSoftware.Drawing.FontTypes.Arial,
FontSize = 10,
LeftText = "{date} {time}",
RightText = "{page} of {total-pages}"
}
}
}
また、レンダリング時に値に置き換えられる次のマージフィールドもあります: {ページ}
, {総ページ数}
, {URL}
, {日付}
, {時間}
, {html-タイトル}
, `{pdfタイトル}もちろん、英語のテキストを教えていただけますでしょうか?
PDF内のテキストの検索と置換
PDF 内のプレースホルダを作成し、プログラムで置き換えるか、ReplaceTextOnPage
メソッドを使用してテキストフレーズのすべてのインスタンスを単に置き換えます。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-14.cs
// Using an existing PDF
var pdf = PdfDocument.FromFile("sample.pdf");
// Parameters
int pageIndex = 1;
string oldText = ".NET 6"; // Old text to remove
string newText = ".NET 7"; // New text to add
// Replace Text on Page
pdf.ReplaceTextOnPage(pageIndex, oldText, newText);
// Placeholder Template Example
pdf.ReplaceTextOnPage(pageIndex, "[DATE]", "01/01/2000");
// Save your new PDF
pdf.SaveAs("new_sample.pdf");
' Using an existing PDF
Dim pdf = PdfDocument.FromFile("sample.pdf")
' Parameters
Dim pageIndex As Integer = 1
Dim oldText As String = ".NET 6" ' Old text to remove
Dim newText As String = ".NET 7" ' New text to add
' Replace Text on Page
pdf.ReplaceTextOnPage(pageIndex, oldText, newText)
' Placeholder Template Example
pdf.ReplaceTextOnPage(pageIndex, "[DATE]", "01/01/2000")
' Save your new PDF
pdf.SaveAs("new_sample.pdf")
以下のコード例ページにある「検索と置換のテキスト例」をご覧になるには、こちらをご覧くださいこれ
アウトラインとブックマーク
アウトラインまたは「ブックマーク」は、PDFの重要なページへ移動するための方法を追加します。 Adobe Acrobat Readerではこれらのブックマーク(ネストされることがあります)アプリケーションの左のサイドバーに表示されます。 IronPDFは既存のPDFドキュメントからブックマークを自動的にインポートし、さらにブックマークを追加、編集、およびネストすることができます。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-15.cs
// Create a new PDF or edit an existing document.
PdfDocument pdf = PdfDocument.FromFile("existing.pdf");
// Add bookmark
pdf.Bookmarks.AddBookMarkAtEnd("Author's Note", 2);
pdf.Bookmarks.AddBookMarkAtEnd("Table of Contents", 3);
// Store new bookmark in a variable to add nested bookmarks to
var summaryBookmark = pdf.Bookmarks.AddBookMarkAtEnd("Summary", 17);
// Add a sub-bookmark within the summary
var conclusionBookmark = summaryBookmark.Children.AddBookMarkAtStart("Conclusion", 18);
// Add another bookmark to end of highest-level bookmark list
pdf.Bookmarks.AddBookMarkAtEnd("References", 20);
pdf.SaveAs("existing.pdf");
' Create a new PDF or edit an existing document.
Dim pdf As PdfDocument = PdfDocument.FromFile("existing.pdf")
' Add bookmark
pdf.Bookmarks.AddBookMarkAtEnd("Author's Note", 2)
pdf.Bookmarks.AddBookMarkAtEnd("Table of Contents", 3)
' Store new bookmark in a variable to add nested bookmarks to
Dim summaryBookmark = pdf.Bookmarks.AddBookMarkAtEnd("Summary", 17)
' Add a sub-bookmark within the summary
Dim conclusionBookmark = summaryBookmark.Children.AddBookMarkAtStart("Conclusion", 18)
' Add another bookmark to end of highest-level bookmark list
pdf.Bookmarks.AddBookMarkAtEnd("References", 20)
pdf.SaveAs("existing.pdf")
コード例ページにあるアウトラインとブックマークの例をご覧になるには、こちらをご訪問くださいこれ.
注釈の追加および編集
IronPDFはPDFの注釈に関する多くのカスタマイズオプションを提供します。 以下にその機能を示す例をご参照ください:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-16.cs
// create a new PDF or load and edit an existing document.
var pdf = PdfDocument.FromFile("existing.pdf");
// Create a PDF annotation object
var textAnnotation = new IronPdf.Annotations.TextAnnotation(PageIndex: 0)
{
Title = "This is the major title",
Contents = "This is the long 'sticky note' comment content...",
Subject = "This is a subtitle",
Opacity = 0.9,
Printable = false,
Hidden = false,
OpenByDefault = true,
ReadOnly = false,
Rotatable = true,
};
// Add the annotation "sticky note" to a specific page and location within any new or existing PDF.
pdf.Annotations.Add(textAnnotation);
pdf.SaveAs("existing.pdf");
' create a new PDF or load and edit an existing document.
Dim pdf = PdfDocument.FromFile("existing.pdf")
' Create a PDF annotation object
Dim textAnnotation = New IronPdf.Annotations.TextAnnotation(PageIndex:= 0) With {
.Title = "This is the major title",
.Contents = "This is the long 'sticky note' comment content...",
.Subject = "This is a subtitle",
.Opacity = 0.9,
.Printable = False,
.Hidden = False,
.OpenByDefault = True,
.ReadOnly = False,
.Rotatable = True
}
' Add the annotation "sticky note" to a specific page and location within any new or existing PDF.
pdf.Annotations.Add(textAnnotation)
pdf.SaveAs("existing.pdf")
PDFの注釈を使用すると、「付箋」ライクなコメントをPDFページに追加することができます。 TextAnnotation
クラスを使用すると、プログラムによって注釈を追加することができます。 対応する高度なテキストアノテーション機能には、サイズ調整、不透明度、アイコン、編集が含まれます。
背景と前景を追加
IronPDFを使用すると、2つのPDFファイルを簡単に結合し、1つを背景または前景として使用することができます。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-17.cs
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf");
pdf.AddBackgroundPdf(@"MyBackground.pdf");
pdf.AddForegroundOverlayPdfToPage(0, @"MyForeground.pdf", 0);
pdf.SaveAs(@"C:\Path\To\Complete.pdf");
Dim renderer = New ChromePdfRenderer()
Dim pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf")
pdf.AddBackgroundPdf("MyBackground.pdf")
pdf.AddForegroundOverlayPdfToPage(0, "MyForeground.pdf", 0)
pdf.SaveAs("C:\Path\To\Complete.pdf")
スタンプと透かし
スタンピングとウォーターマークの機能は、あらゆるPDFエディターの基本的な機能です。 IronPDFには、画像スタンプやHTMLスタンプなど、さまざまなスタンプを作成するための素晴らしいAPIがあります。 以下のように、アライメントとオフセットを使用して高度にカスタマイズ可能な配置を行うことができます。
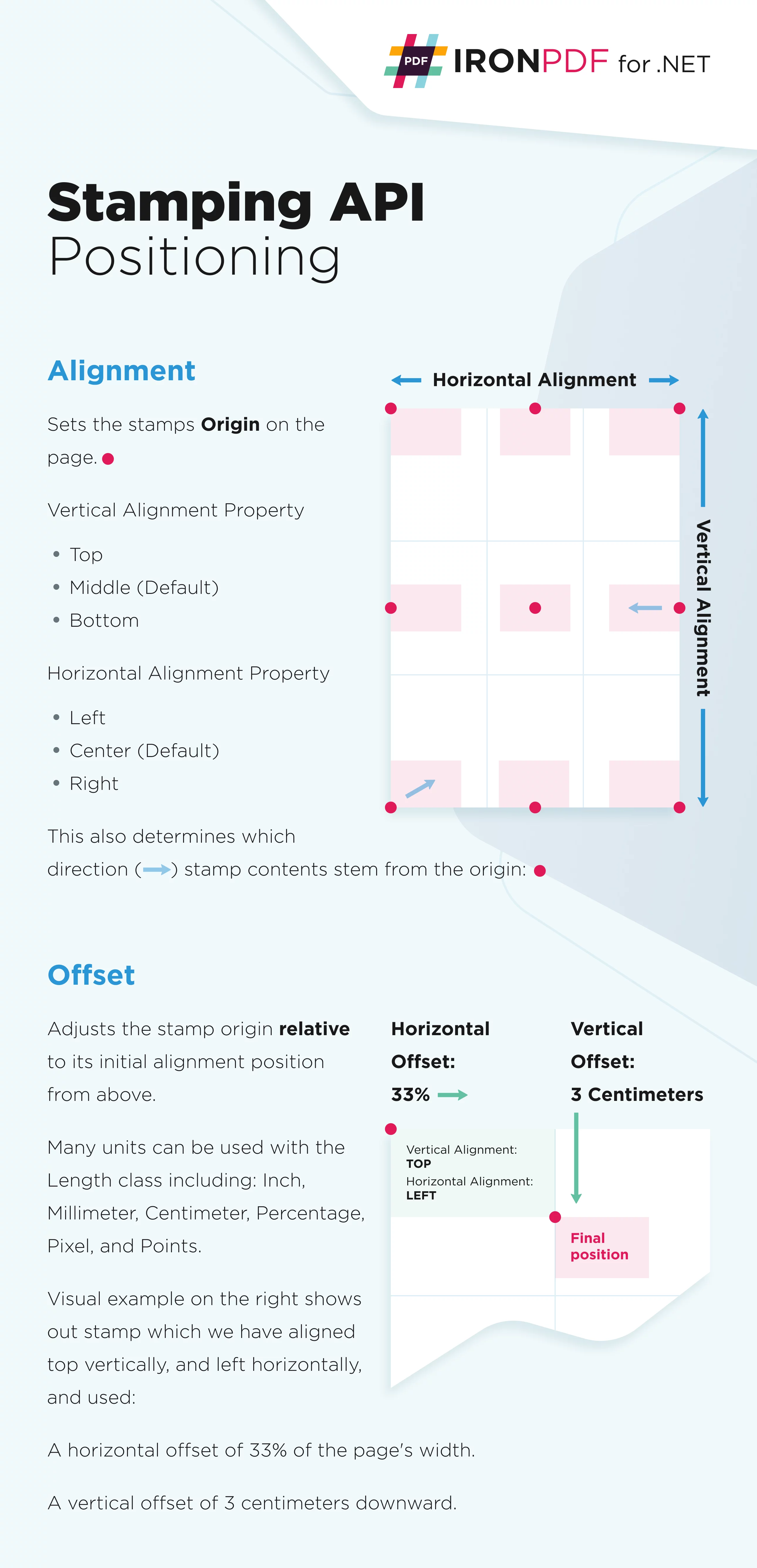
スタンパー抽象クラス
IronPDFのすべてのスタンピングメソッドのパラメータとしてStamper
抽象クラスが使用されます。
それぞれのユースケースに対応する多くのクラスがあります。
- テキストをスタンプする
TextStamper
-テキスト例 - 画像にスタンプを押すための
ImageStamper
画像の例 - HTMLをスタンプするための
HtmlStamper
HTMLの例 - バーコードをスタンプするための
BarcodeStamper
バーコードの例 -
QRコードをスタンプするための
BarcodeStamper
QRコードの例- 透かしのために、参照してください[Here is the translation of the given text into Japanese:
これ](#anchor-add-a-watermark-to-a-pdf).
これらのいずれかを適用するには、ApplyStamp
の一つを使用してください。()メソッド 参照:このチュートリアルの「Apply Stamp」セクション.
スタンパークラスのプロパティ
abstract class Stamper
└─── int : Opacity
└─── int : Rotation
└─── double : Scale
└─── Length : HorizontalOffset
└─── Length : VerticalOffset
└─── Length : MinWidth
└─── Length : MaxWidth
└─── Length : MinHeight
└─── Length : MaxHeight
└─── string : Hyperlink
└─── bool : IsStampBehindContent (default : false)
└─── HorizontalAlignment : HorizontalAlignment
│ │ Left
│ │ Center (default)
│ │ Right
│
└─── VerticalAlignment : VerticalAlignment
│ Top
│ Middle (default)
│ Bottom
スタンプの例
以下では、Stamper のすべてのサブクラスをコード例とともに紹介します。
PDFにテキストをスタンプする
2つの異なるテキストスタンパーをそれぞれ異なる方法で作成し、両方を適用する:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-18.cs
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>");
TextStamper stamper1 = new TextStamper
{
Text = "Hello World! Stamp One Here!",
FontFamily = "Bungee Spice",
UseGoogleFont = true,
FontSize = 100,
IsBold = true,
IsItalic = true,
VerticalAlignment = VerticalAlignment.Top
};
TextStamper stamper2 = new TextStamper()
{
Text = "Hello World! Stamp Two Here!",
FontFamily = "Bungee Spice",
UseGoogleFont = true,
FontSize = 30,
VerticalAlignment = VerticalAlignment.Bottom
};
Stamper[] stampersToApply = { stamper1, stamper2 };
pdf.ApplyMultipleStamps(stampersToApply);
pdf.ApplyStamp(stamper2);
Dim renderer = New ChromePdfRenderer()
Dim pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>")
Dim stamper1 As New TextStamper With {
.Text = "Hello World! Stamp One Here!",
.FontFamily = "Bungee Spice",
.UseGoogleFont = True,
.FontSize = 100,
.IsBold = True,
.IsItalic = True,
.VerticalAlignment = VerticalAlignment.Top
}
Dim stamper2 As New TextStamper() With {
.Text = "Hello World! Stamp Two Here!",
.FontFamily = "Bungee Spice",
.UseGoogleFont = True,
.FontSize = 30,
.VerticalAlignment = VerticalAlignment.Bottom
}
Dim stampersToApply() As Stamper = { stamper1, stamper2 }
pdf.ApplyMultipleStamps(stampersToApply)
pdf.ApplyStamp(stamper2)
PDFに画像をスタンプする
さまざまなページの組み合わせで既存のPDFドキュメントに画像スタンプを適用する:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-19.cs
var pdf = new PdfDocument("/attachments/2022_Q1_sales.pdf");
ImageStamper logoImageStamper = new ImageStamper("/assets/logo.png");
// Apply to every page, one page, or some pages
pdf.ApplyStamp(logoImageStamper);
pdf.ApplyStamp(logoImageStamper, 0);
pdf.ApplyStamp(logoImageStamper, new[] { 0, 3, 11 });
Dim pdf = New PdfDocument("/attachments/2022_Q1_sales.pdf")
Dim logoImageStamper As New ImageStamper("/assets/logo.png")
' Apply to every page, one page, or some pages
pdf.ApplyStamp(logoImageStamper)
pdf.ApplyStamp(logoImageStamper, 0)
pdf.ApplyStamp(logoImageStamper, { 0, 3, 11 })
PDFにHTMLをスタンプする
スタンプとして使用する独自のHTMLを書く:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-20.cs
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderHtmlAsPdf("<p>Hello World, example HTML body.</p>");
HtmlStamper stamper = new HtmlStamper($"<p>Example HTML Stamped</p><div style='width:250pt;height:250pt;background-color:red;'></div>")
{
HorizontalOffset = new Length(-3, MeasurementUnit.Inch),
VerticalAlignment = VerticalAlignment.Bottom
};
pdf.ApplyStamp(stamper);
Dim renderer = New ChromePdfRenderer()
Dim pdf = renderer.RenderHtmlAsPdf("<p>Hello World, example HTML body.</p>")
Dim stamper As New HtmlStamper($"<p>Example HTML Stamped</p><div style='width:250pt;height:250pt;background-color:red;'></div>")
If True Then
'INSTANT VB WARNING: An assignment within expression was extracted from the following statement:
'ORIGINAL LINE: HorizontalOffset = new Length(-3, MeasurementUnit.Inch), VerticalAlignment = VerticalAlignment.Bottom
New Length(-3, MeasurementUnit.Inch), VerticalAlignment = VerticalAlignment.Bottom
HorizontalOffset = New Length(-3, MeasurementUnit.Inch), VerticalAlignment
End If
pdf.ApplyStamp(stamper)
PDFにバーコードをスタンプする
バーコードの作成およびスタンプの例:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-21.cs
BarcodeStamper bcStamp = new BarcodeStamper("IronPDF", BarcodeEncoding.Code39);
bcStamp.HorizontalAlignment = HorizontalAlignment.Left;
bcStamp.VerticalAlignment = VerticalAlignment.Bottom;
var pdf = new PdfDocument("example.pdf");
pdf.ApplyStamp(bcStamp);
Dim bcStamp As New BarcodeStamper("IronPDF", BarcodeEncoding.Code39)
bcStamp.HorizontalAlignment = HorizontalAlignment.Left
bcStamp.VerticalAlignment = VerticalAlignment.Bottom
Dim pdf = New PdfDocument("example.pdf")
pdf.ApplyStamp(bcStamp)
PDFにQRコードをスタンプする
QRコードの作成とスタンプの例:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-22.cs
BarcodeStamper qrStamp = new BarcodeStamper("IronPDF", BarcodeEncoding.QRCode);
qrStamp.Height = 50; // pixels
qrStamp.Width = 50; // pixels
qrStamp.HorizontalAlignment = HorizontalAlignment.Left;
qrStamp.VerticalAlignment = VerticalAlignment.Bottom;
var pdf = new PdfDocument("example.pdf");
pdf.ApplyStamp(qrStamp);
Dim qrStamp As New BarcodeStamper("IronPDF", BarcodeEncoding.QRCode)
qrStamp.Height = 50 ' pixels
qrStamp.Width = 50 ' pixels
qrStamp.HorizontalAlignment = HorizontalAlignment.Left
qrStamp.VerticalAlignment = VerticalAlignment.Bottom
Dim pdf = New PdfDocument("example.pdf")
pdf.ApplyStamp(qrStamp)
PDFにウォーターマークを追加する
ウォーターマークは、ApplyWatermark
メソッドを使用して簡単に適用できる各ページのスタンプの一種です。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-23.cs
var pdf = new PdfDocument("/attachments/design.pdf");
string html = "<h1> Example Title <h1/>";
int rotation = 0;
int watermarkOpacity = 30;
pdf.ApplyWatermark(html, rotation, watermarkOpacity);
Dim pdf = New PdfDocument("/attachments/design.pdf")
Dim html As String = "<h1> Example Title <h1/>"
Dim rotation As Integer = 0
Dim watermarkOpacity As Integer = 30
pdf.ApplyWatermark(html, rotation, watermarkOpacity)
ウォーターマーキングの例をコード例のページでご覧になるには、次のリンクを訪問してください。これ.
PDFにスタンプを適用する
スタンプをPDFに適用するために使用できるApplyStampメソッドにはいくつかのオーバーロードがあります。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-24.cs
var pdf = new PdfDocument("/assets/example.pdf");
// Apply one stamp to all pages
pdf.ApplyStamp(myStamper);
// Apply one stamp to a specific page
pdf.ApplyStamp(myStamper, 0);
// Apply one stamp to specific pages
pdf.ApplyStamp(myStamper, new[] { 0, 3, 5 });
// Apply a stamp array to all pages
pdf.ApplyMultipleStamps(stampArray);
// Apply a stamp array to a specific page
pdf.ApplyMultipleStamps(stampArray, 0);
// Apply a stamp array to specific pages
pdf.ApplyMultipleStamps(stampArray, new[] { 0, 3, 5 });
// And some Async versions of the above
await pdf.ApplyStampAsync(myStamper, 4);
await pdf.ApplyMultipleStampsAsync(stampArray);
// Additional Watermark apply method
string html = "<h1> Example Title <h1/>";
int rotation = 0;
int watermarkOpacity = 30;
pdf.ApplyWatermark(html, rotation, watermarkOpacity);
Dim pdf = New PdfDocument("/assets/example.pdf")
' Apply one stamp to all pages
pdf.ApplyStamp(myStamper)
' Apply one stamp to a specific page
pdf.ApplyStamp(myStamper, 0)
' Apply one stamp to specific pages
pdf.ApplyStamp(myStamper, { 0, 3, 5 })
' Apply a stamp array to all pages
pdf.ApplyMultipleStamps(stampArray)
' Apply a stamp array to a specific page
pdf.ApplyMultipleStamps(stampArray, 0)
' Apply a stamp array to specific pages
pdf.ApplyMultipleStamps(stampArray, { 0, 3, 5 })
' And some Async versions of the above
Await pdf.ApplyStampAsync(myStamper, 4)
Await pdf.ApplyMultipleStampsAsync(stampArray)
' Additional Watermark apply method
Dim html As String = "<h1> Example Title <h1/>"
Dim rotation As Integer = 0
Dim watermarkOpacity As Integer = 30
pdf.ApplyWatermark(html, rotation, watermarkOpacity)
長さクラス
Length クラスには、Unit
と Value
という二つのプロパティがあります。 MeasurementUnit
列挙型から使用するユニットを決定したら(デフォルトはページのPercentage
です)次に、Value
を選択してベース単位の倍数として使用する長さの量を決定します。
長さクラスのプロパティ
class Length
└─── double : Value (default : 0)
└─── MeasurementUnit : Unit
Inch
Millimeter
Centimeter
Percentage (default)
Pixel
Points
長さの例
長さの作成
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-25.cs
new Length(value: 5, unit: MeasurementUnit.Inch); // 5 inches
new Length(value: 25, unit: MeasurementUnit.Pixel);// 25px
new Length(); // 0% of the page dimension because value is defaulted to zero and unit is defaulted to percentage
new Length(value: 20); // 20% of the page dimension
Dim tempVar As New Length(value:= 5, unit:= MeasurementUnit.Inch) ' 5 inches
Dim tempVar2 As New Length(value:= 25, unit:= MeasurementUnit.Pixel) ' 25px
Dim tempVar3 As New Length() ' 0% of the page dimension because value is defaulted to zero and unit is defaulted to percentage
Dim tempVar4 As New Length(value:= 20) ' 20% of the page dimension
長さをパラメーターとして使用する
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-26.cs
HtmlStamper logoStamper = new HtmlStamper
{
VerticalOffset = new Length(15, MeasurementUnit.Percentage),
HorizontalOffset = new Length(1, MeasurementUnit.Inch)
// set other properties...
};
Dim logoStamper As New HtmlStamper With {
.VerticalOffset = New Length(15, MeasurementUnit.Percentage),
.HorizontalOffset = New Length(1, MeasurementUnit.Inch)
}
PDFでフォームを使用する
フォームを作成および編集
IronPDFを使用して埋め込みフォームフィールド付きのPDFを作成する:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-27.cs
// Step 1. Creating a PDF with editable forms from HTML using form and input tags
const string formHtml = @"
<html>
<body>
<h2>Editable PDF Form</h2>
<form>
First name: <br> <input type='text' name='firstname' value=''> <br>
Last name: <br> <input type='text' name='lastname' value=''>
</form>
</body>
</html>";
// Instantiate Renderer
var renderer = new ChromePdfRenderer();
renderer.RenderingOptions.CreatePdfFormsFromHtml = true;
renderer.RenderHtmlAsPdf(formHtml).SaveAs("BasicForm.pdf");
// Step 2. Reading and Writing PDF form values.
var formDocument = PdfDocument.FromFile("BasicForm.pdf");
// Read the value of the "firstname" field
var firstNameField = formDocument.Form.FindFormField("firstname");
// Read the value of the "lastname" field
var lastNameField = formDocument.Form.FindFormField("lastname");
' Step 1. Creating a PDF with editable forms from HTML using form and input tags
Const formHtml As String = "
<html>
<body>
<h2>Editable PDF Form</h2>
<form>
First name: <br> <input type='text' name='firstname' value=''> <br>
Last name: <br> <input type='text' name='lastname' value=''>
</form>
</body>
</html>"
' Instantiate Renderer
Dim renderer = New ChromePdfRenderer()
renderer.RenderingOptions.CreatePdfFormsFromHtml = True
renderer.RenderHtmlAsPdf(formHtml).SaveAs("BasicForm.pdf")
' Step 2. Reading and Writing PDF form values.
Dim formDocument = PdfDocument.FromFile("BasicForm.pdf")
' Read the value of the "firstname" field
Dim firstNameField = formDocument.Form.FindFormField("firstname")
' Read the value of the "lastname" field
Dim lastNameField = formDocument.Form.FindFormField("lastname")
コード例ページでPDF フォームのサンプルを見るには、以下のリンクをご覧ください。これ.
既存のフォームを記入
IronPDFを使用すると、既存のすべてのフォームフィールドに簡単にアクセスして、それらを記入し再保存することができます。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-28.cs
var formDocument = PdfDocument.FromFile("BasicForm.pdf");
// Set and Read the value of the "firstname" field
var firstNameField = formDocument.Form.FindFormField("firstname");
firstNameField.Value = "Minnie";
Console.WriteLine("FirstNameField value: {0}", firstNameField.Value);
// Set and Read the value of the "lastname" field
var lastNameField = formDocument.Form.FindFormField("lastname");
lastNameField.Value = "Mouse";
Console.WriteLine("LastNameField value: {0}", lastNameField.Value);
formDocument.SaveAs("FilledForm.pdf");
Dim formDocument = PdfDocument.FromFile("BasicForm.pdf")
' Set and Read the value of the "firstname" field
Dim firstNameField = formDocument.Form.FindFormField("firstname")
firstNameField.Value = "Minnie"
Console.WriteLine("FirstNameField value: {0}", firstNameField.Value)
' Set and Read the value of the "lastname" field
Dim lastNameField = formDocument.Form.FindFormField("lastname")
lastNameField.Value = "Mouse"
Console.WriteLine("LastNameField value: {0}", lastNameField.Value)
formDocument.SaveAs("FilledForm.pdf")
コード例ページでPDF フォームのサンプルを見るには、以下のリンクをご覧ください。これ.
結論
上記の例のリストは、IronPDFがPDFの編集に関してすぐに使える重要な機能を持っていることを示しています。
以下の内容を日本語に翻訳してください:
新機能のリクエストをしたい場合や、IronPDFやライセンスに関する一般的な質問がある場合は、どうぞサポートチームに連絡する. 喜んでお手伝いいたします。