Azure上で.NETを使用してHTMLからPDFを実行する方法は?
はい。 IronPDFは、Azure上でPDFドキュメントを生成、操作、読み取るために使用できます。 IronPDFは、多くのAzureプラットフォーム上で、MVCウェブサイトやAzure Functionsなどを含めて徹底的にテストされています。
Docker 上の Azure Functions
Docker コンテナ内で Azure Functions を実行している場合は、以下をご参照ください。このAzure Docker Linuxチュートリアルその代わりに
Azure FunctionでPDFジェネレータを作成する方法
- AzureでPDFを生成するためのC#ライブラリをインストール
- Azure Basic B1 以上のホスティング層を選択してください
- チェックを外してください
パッケージファイルから実行
公開時のオプション - 推奨される設定手順に従ってください。
- コード例を使用して、Azureを使用してPDFジェネレーターを作成してください。
チュートリアルの方法
プロジェクトの設定
IronPDFをインストールして始めましょう
最初のステップは、NuGetを使用してIronPDFをインストールすることです。
- WindowsベースのAzure Functionsでは、
IronPdf
パッケージを使用してください。Windows用NuGet IronPdfパッケージ - LinuxベースのAzure Functionsでは、
IronPdf.Linux
パッケージを使用してください。Linux用NuGet IronPdfパッケージ
Install-Package IronPdf
または、.dll ファイルを手動でインストールするには、Azure用IronPDF直接ダウンロードパッケージリンク.
正しいAzureオプションを選択
Azureレベルのホスティングレベルを選択する
Azure Basic B1 は、エンドユーザーのレンダリングニーズに必要な最低ホスティングレベルです。 高スループットシステムを作成している場合、これをアップグレードする必要があるかもしれません。
続行する前に
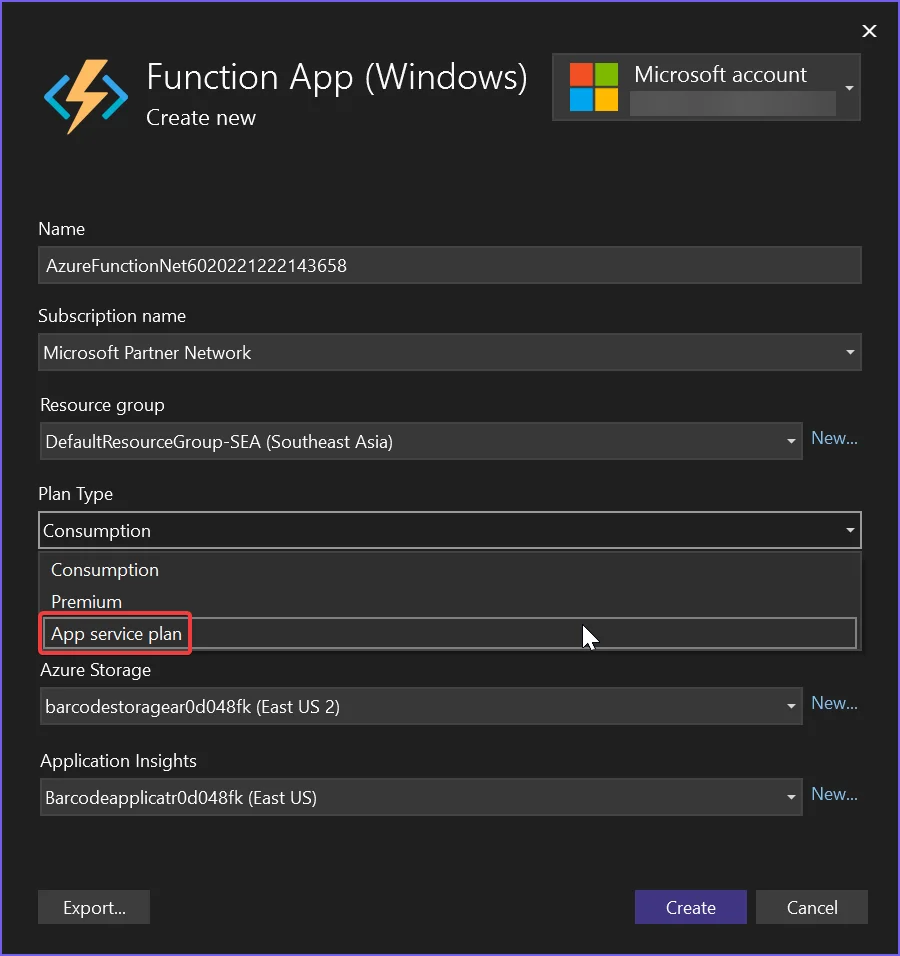
「パッケージファイルから実行」チェックボックス
Azure Functionsアプリケーションを公開するときは、Run from package file
がNOT選択されていることを確認してください。
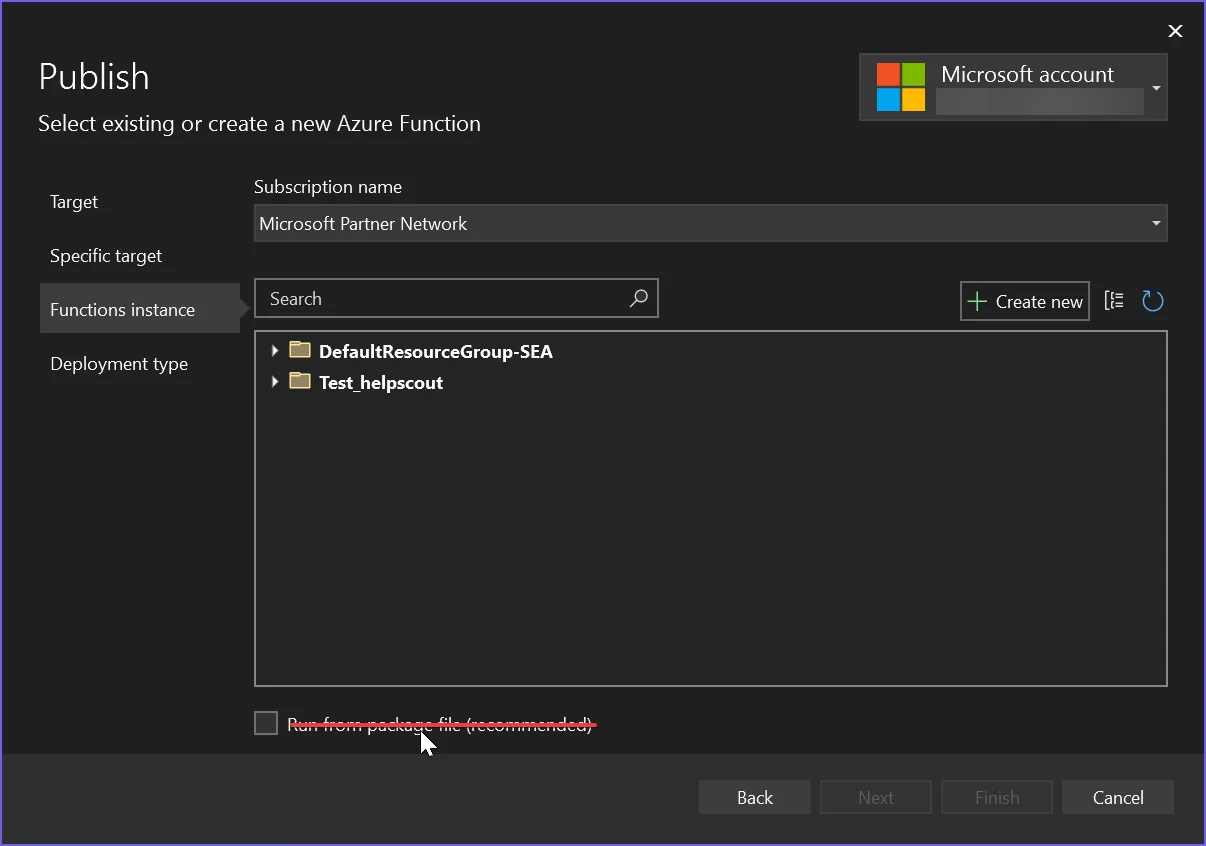
.NET 6 の構成
マイクロソフトは最近、イメージングライブラリを.NET 6+から削除し、多くのレガシーAPIを破壊しました。そのため、これらのレガシーAPI呼び出しを引き続き許可するようにプロジェクトを設定する必要があります。
-
Linuxでは、マシンに
libgdiplus
をインストールするために、Installation.LinuxAndDockerDependenciesAutoConfig=true;
を設定してください。 -
以下を .NET 6 プロジェクトの .csproj ファイルに追加してください:
True もちろん、英語のテキストを教えていただけますでしょうか? - プロジェクト内に
runtimeconfig.template.json
というファイルを作成し、次の内容を記入してください:
{
"configProperties": {
"System.Drawing.EnableUnixSupport": true
}
}
{
"configProperties": {
"System.Drawing.EnableUnixSupport": true
}
}
If True Then
"configProperties":
If True Then
"System.Drawing.EnableUnixSupport": True
End If
End If
- 最後に、プログラムの先頭に次の行を追加します:
System.AppContext.SetSwitch(「System.Drawing.EnableUnixSupport」、true);
AzureでDockerを使用する
Azureでパフォーマンスを制御し、SVGフォントへのアクセスを得る一つの方法として、Dockerコンテナ内からIronPDFアプリケーションとFunctionsを使用することが挙げられます。
私たちは包括的なIronPDF Azure Docker チュートリアルLinuxとWindowsのインスタンスに対応しており、読むことを推奨します。
Azure Function コード例
この例では、ログエントリを自動的に組み込みのAzureロガーに出力します。(ILogger log
を参照してください):
[FunctionName("PrintPdf")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Anonymous, "get", "post", Route = null)] HttpRequest req,
ILogger log, ExecutionContext context)
{
log.LogInformation("Entered PrintPdf API function...");
// Apply license key
IronPdf.License.LicenseKey = "IRONPDF-MYLICENSE-KEY-1EF01";
// Enable log
IronPdf.Logging.Logger.LoggingMode = IronPdf.Logging.Logger.LoggingModes.Custom;
IronPdf.Logging.Logger.CustomLogger = log;
IronPdf.Logging.Logger.EnableDebugging = false;
// Configure IronPdf
Installation.LinuxAndDockerDependenciesAutoConfig = false;
Installation.ChromeGpuMode = IronPdf.Engines.Chrome.ChromeGpuModes.Disabled;
try
{
log.LogInformation("About to render pdf...");
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render PDF
var pdf = renderer.RenderUrlAsPdf("https://www.google.com/");
log.LogInformation("finished rendering pdf...");
return new FileContentResult(pdf.BinaryData, "application/pdf") { FileDownloadName = "google.pdf" };
}
catch (Exception e)
{
log.LogError(e, "Error while rendering pdf", e);
}
return new OkObjectResult("OK");
}
[FunctionName("PrintPdf")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Anonymous, "get", "post", Route = null)] HttpRequest req,
ILogger log, ExecutionContext context)
{
log.LogInformation("Entered PrintPdf API function...");
// Apply license key
IronPdf.License.LicenseKey = "IRONPDF-MYLICENSE-KEY-1EF01";
// Enable log
IronPdf.Logging.Logger.LoggingMode = IronPdf.Logging.Logger.LoggingModes.Custom;
IronPdf.Logging.Logger.CustomLogger = log;
IronPdf.Logging.Logger.EnableDebugging = false;
// Configure IronPdf
Installation.LinuxAndDockerDependenciesAutoConfig = false;
Installation.ChromeGpuMode = IronPdf.Engines.Chrome.ChromeGpuModes.Disabled;
try
{
log.LogInformation("About to render pdf...");
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render PDF
var pdf = renderer.RenderUrlAsPdf("https://www.google.com/");
log.LogInformation("finished rendering pdf...");
return new FileContentResult(pdf.BinaryData, "application/pdf") { FileDownloadName = "google.pdf" };
}
catch (Exception e)
{
log.LogError(e, "Error while rendering pdf", e);
}
return new OkObjectResult("OK");
}
<FunctionName("PrintPdf")>
Public Shared Async Function Run(<HttpTrigger(AuthorizationLevel.Anonymous, "get", "post", Route := Nothing)> ByVal req As HttpRequest, ByVal log As ILogger, ByVal context As ExecutionContext) As Task(Of IActionResult)
log.LogInformation("Entered PrintPdf API function...")
' Apply license key
IronPdf.License.LicenseKey = "IRONPDF-MYLICENSE-KEY-1EF01"
' Enable log
IronPdf.Logging.Logger.LoggingMode = IronPdf.Logging.Logger.LoggingModes.Custom
IronPdf.Logging.Logger.CustomLogger = log
IronPdf.Logging.Logger.EnableDebugging = False
' Configure IronPdf
Installation.LinuxAndDockerDependenciesAutoConfig = False
Installation.ChromeGpuMode = IronPdf.Engines.Chrome.ChromeGpuModes.Disabled
Try
log.LogInformation("About to render pdf...")
Dim renderer As New ChromePdfRenderer()
' Render PDF
Dim pdf = renderer.RenderUrlAsPdf("https://www.google.com/")
log.LogInformation("finished rendering pdf...")
Return New FileContentResult(pdf.BinaryData, "application/pdf") With {.FileDownloadName = "google.pdf"}
Catch e As Exception
log.LogError(e, "Error while rendering pdf", e)
End Try
Return New OkObjectResult("OK")
End Function
既知の問題
共有ホスティングプランではSVGフォントのレンダリングは利用できません。
見つかった制限の一つはAzure ホスティングプラットフォームの概要安価な共有ウェブアプリティアでは、サーバーがGoogleフォントなどのSVGフォントを読み込むことをサポートしていません。 なぜなら、これらの共有ホスティング・プラットフォームは、セキュリティ上の理由からWindows GDI+グラフィックス・オブジェクトへのアクセスが許可されていないからです。
以下の内容を日本語に翻訳してください:
使用をお勧めしますIronPDF用WindowsまたはLinux Dockerコンテナ・ガイドこの問題を解決するために、最適なフォントレンダリングが必要な場合、Azure上のVPSを利用するのも一つの方法です。
Azureの無料ティアホスティングは遅いです。
Azureの無料および共有レベル、または従量課金プランは、PDFレンダリングには適していません。 私たちは、自分たち自身で使用しているAzure B1ホスティング/プレミアムプランを推奨します。 HTML から PDF への変換プロセスは、あらゆるコンピューターにとって重要な「仕事」です。これは、あなた自身のマシンでウェブページを開いてレンダリングすることに似ています。実際のブラウザエンジンが使用されるため、それに応じてプロビジョニングする必要があり、同様の性能を持つデスクトップマシンのレンダリング時間と同程度の時間がかかると予想されます。
技術サポートリクエストチケットの作成
リクエストチケットを作成するにはIronPDFのエンジニアリングサポートリクエストの作成方法ガイド