C#でPDFドキュメントを印刷
.NETアプリケーションで、Visual BasicまたはC#コードを使用して簡単にPDFを印刷できます。 このチュートリアルでは、C#のPDF印刷機能を使用してプログラム的に印刷する方法を説明します。
C#でPDFファイルを印刷する方法
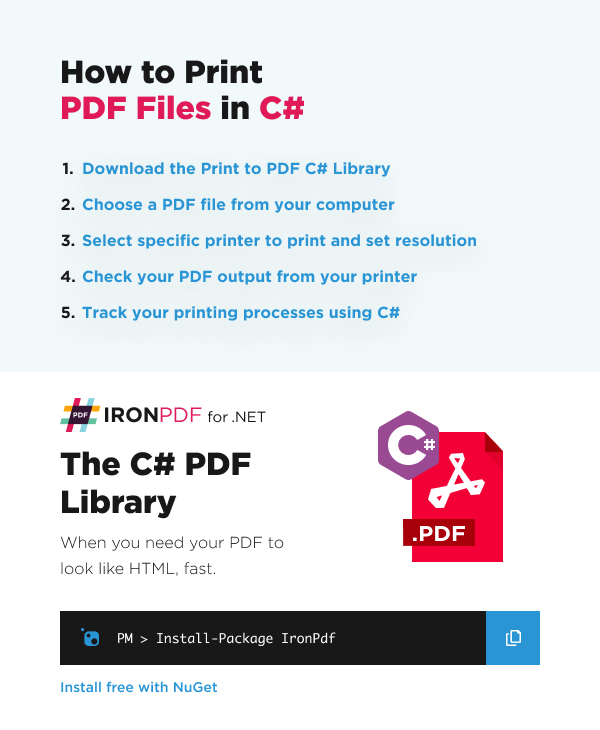
- PDF出力用C#ライブラリをダウンロード
- コンピュータからPDFファイルを選択してください
- 特定のプリンターを選択して解像度を設定する
- プリンタからのPDF出力を確認してください。
- C#を使用して印刷プロセスを追跡する
今日から無料トライアルでIronPDFをあなたのプロジェクトで使い始めましょう。
PDFを作成して印刷する
PDFドキュメントをプリンターにサイレントで直接送信することができます。または、System.Drawing.Printing.PrintDocumentGUI印刷ダイアログに送信して操作できるオブジェクト。
以下のコードは、両方のオプションで使用できます:
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-create-and-print-pdf.cs
using IronPdf;
using System.Threading.Tasks;
// Create a new PDF and print it
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf");
// Print PDF from default printer
await pdf.Print();
// For advanced silent real-world printing options, use PdfDocument.GetPrintDocument
// Remember to add an assembly reference to System.Drawing.dll
System.Drawing.Printing.PrintDocument PrintDocYouCanWorkWith = pdf.GetPrintDocument();
Imports IronPdf
Imports System.Threading.Tasks
' Create a new PDF and print it
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf")
' Print PDF from default printer
Await pdf.Print()
' For advanced silent real-world printing options, use PdfDocument.GetPrintDocument
' Remember to add an assembly reference to System.Drawing.dll
Dim PrintDocYouCanWorkWith As System.Drawing.Printing.PrintDocument = pdf.GetPrintDocument()
高度な印刷
IronPDFは、プリンター名の検索や設定、プリンターの解像度の設定など、高度な印刷機能に十分対応できます。
プリンターの名前を指定する
プリンター名を指定するには、現在の印刷ドキュメントオブジェクトを取得するだけです。(使用してPDFドキュメントのGetPrintDocumentメソッドPDFドキュメントの)次に、PrinterSettings.PrinterName プロパティを使用します。
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-specify-printer-name.cs
using IronPdf;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
// Get PrintDocument object
var printDocument = pdf.GetPrintDocument();
// Assign the printer name
printDocument.PrinterSettings.PrinterName = "Microsoft Print to PDF";
// Print document
printDocument.Print();
Imports IronPdf
Private pdf As PdfDocument = PdfDocument.FromFile("sample.pdf")
' Get PrintDocument object
Private printDocument = pdf.GetPrintDocument()
' Assign the printer name
printDocument.PrinterSettings.PrinterName = "Microsoft Print to PDF"
' Print document
printDocument.Print()
プリンターの解像度を設定する
解像度とは、出力に応じて印刷または表示されるピクセルの数を指します。 印刷の解像度はDefaultPageSettings.PrinterResolution`プロパティPDFドキュメントの こちらは非常に簡単なデモンストレーションです。
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-specify-printer-resolution.cs
using IronPdf;
using System.Drawing.Printing;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
// Get PrintDocument object
var printDocument = pdf.GetPrintDocument();
// Set printer resolution
printDocument.DefaultPageSettings.PrinterResolution = new PrinterResolution
{
Kind = PrinterResolutionKind.Custom,
X = 1200,
Y = 1200
};
// Print document
printDocument.Print();
Imports IronPdf
Imports System.Drawing.Printing
Private pdf As PdfDocument = PdfDocument.FromFile("sample.pdf")
' Get PrintDocument object
Private printDocument = pdf.GetPrintDocument()
' Set printer resolution
printDocument.DefaultPageSettings.PrinterResolution = New PrinterResolution With {
.Kind = PrinterResolutionKind.Custom,
.X = 1200,
.Y = 1200
}
' Print document
printDocument.Print()
ご覧の通り、解像度をカスタムレベルに設定しました:垂直1200および水平1200。
ファイルへの印刷メソッド
PdfDocument.PrintToFile
メソッドを使用すると、PDFをファイルに印刷できます。出力ファイルパスを指定し、プレビューを表示するかどうかを指定するだけです。 以下のコードは、プレビューを含めずに指定されたファイルに印刷されます:
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-printtofile.cs
using IronPdf;
using System.Threading.Tasks;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
await pdf.PrintToFile("PathToFile", false);
Imports IronPdf
Imports System.Threading.Tasks
Private pdf As PdfDocument = PdfDocument.FromFile("sample.pdf")
Await pdf.PrintToFile("PathToFile", False)
C#を使用した印刷プロセスのトレース
C#とIronPDFの組み合わせの素晴らしさは、印刷されたページや印刷に関連するものを追跡することに関しては、実際には非常にシンプルであるということです。 次の例では、プリンタの名前と解像度を変更する方法と、印刷されたページ数を取得する方法を示します。
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-trace-printing-process.cs
using IronPdf;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
// Get PrintDocument object
var printDocument = pdf.GetPrintDocument();
// Subscribe to the PrintPage event
var printedPages = 0;
printDocument.PrintPage += (sender, args) => printedPages++;
// Print document
printDocument.Print();
Imports IronPdf
Private pdf As PdfDocument = PdfDocument.FromFile("sample.pdf")
' Get PrintDocument object
Private printDocument = pdf.GetPrintDocument()
' Subscribe to the PrintPage event
Private printedPages = 0
Private printDocument.PrintPage += Sub(sender, args) printedPages++
' Print document
printDocument.Print()