ASP.NET Core MVCでビューをPDFに変換する方法
ビューは、ASP.NETフレームワーク内で使用されるコンポーネントであり、WebアプリケーションでHTMLマークアップを生成するためのものです。 これは、ASP.NET MVC および ASP.NET Core MVC アプリケーションで一般的に使用される Model-View-Controller (MVC) パターンの一部です。 ビューは、HTMLコンテンツを動的にレンダリングすることで、ユーザーにデータを提示する役割を担っています。
ASP.NET Core Web App MVC (Model-View-Controller) は、ASP.NET Core を使用してウェブアプリケーションを構築するために Microsoft が提供するウェブアプリケーションです。
- モデル: モデルはデータとビジネスロジックを表し、データのやり取りを管理し、データソースと通信します。
- ビュー: ビューはユーザーインターフェースを提示し、データの表示に集中してユーザーに情報をレンダリングします。
-
コントローラー(Controller):コントローラーはユーザー入力を処理し、リクエストに応答し、モデル(Model)と通信し、モデルとビュー(View)の間の相互作用を統括します。
IronPDFはASP.NET Core MVCプロジェクト内のビューからPDFファイルを作成するプロセスを簡素化します。 これにより、ASP.NET Core MVCでのPDF生成が簡単かつ直接的になります。
IronPDF拡張パッケージ
IronPdf.Extensions.Mvc.Core パッケージは、主要なIronPdfパッケージの拡張です。 IronPdf.Extensions.Mvc.Core と IronPdfパッケージは、ASP.NET Core MVCでPDFドキュメントにビューをレンダリングするために必要です。
Install-Package IronPdf.Extensions.Mvc.Core
NuGetでインストール
Install-Package IronPdf.Extensions.Mvc.Core
ビューをPDFにレンダリング
ビューをPDFファイルに変換するには、ASP.NET Core Web App(モデル-ビュー-コントローラー)プロジェクトが必要です。
クラスのモデルを追加
- 「Models」フォルダーに移動する
- 新しいC#クラスファイルを「Person」と名付けて作成します。このクラスは、個々のデータを表すモデルとして機能します。 以下のコードスニペットを使用してください:
:path=/static-assets/pdf/content-code-examples/how-to/cshtml-to-pdf-mvc-core-model.cs
namespace ViewToPdfMVCCoreSample.Models
{
public class Person
{
public int Id { get; set; }
public string Name { get; set; }
public string Title { get; set; }
public string Description { get; set; }
}
}
コントローラーを編集
"Controllers" フォルダーに移動し、「HomeController」ファイルを開いてください。「HomeController」にのみ変更を加え、「Persons」アクションを追加します。 以下のコードを参照してください:
以下のコードは、最初にChromePdfRendererクラスをインスタンス化し、IRazorViewRenderer、"Persons.cshtml"へのパス、必要なデータを含むListをRenderRazorViewToPdf
メソッドに渡します。 ユーザーはRenderingOptionsを利用して、結果として得られるPDFにカスタムのテキスト、HTMLヘッダーおよびフッターを含める、カスタムの余白を定義する、ページ番号を適用するなど、さまざまな機能にアクセスできます。
[{i:(PDFドキュメントは次のコードを使用してブラウザで表示できます:File(pdf.BinaryData, "application/pdf")
。 しかし、ブラウザで表示した後にPDFをダウンロードすると、破損したPDFドキュメントが生成されます。
using IronPdf.Extensions.Mvc.Core;
using Microsoft.AspNetCore.Mvc;
using System.Diagnostics;
using ViewToPdfMVCCoreSample.Models;
namespace ViewToPdfMVCCoreSample.Controllers
{
public class HomeController : Controller
{
private readonly ILogger<HomeController> _logger;
private readonly IRazorViewRenderer _viewRenderService;
private readonly IHttpContextAccessor _httpContextAccessor;
public HomeController(ILogger<HomeController> logger, IRazorViewRenderer viewRenderService, IHttpContextAccessor httpContextAccessor)
{
_logger = logger;
_viewRenderService = viewRenderService;
_httpContextAccessor = httpContextAccessor;
}
public IActionResult Index()
{
return View();
}
public async Task<IActionResult> Persons()
{
var persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
if (_httpContextAccessor.HttpContext.Request.Method == HttpMethod.Post.Method)
{
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render View to PDF document
PdfDocument pdf = renderer.RenderRazorViewToPdf(_viewRenderService, "Views/Home/Persons.cshtml", persons);
Response.Headers.Add("Content-Disposition", "inline");
// Output PDF document
return File(pdf.BinaryData, "application/pdf", "viewToPdfMVCCore.pdf");
}
return View(persons);
}
public IActionResult Privacy()
{
return View();
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error()
{
return View(new ErrorViewModel { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });
}
}
}
using IronPdf.Extensions.Mvc.Core;
using Microsoft.AspNetCore.Mvc;
using System.Diagnostics;
using ViewToPdfMVCCoreSample.Models;
namespace ViewToPdfMVCCoreSample.Controllers
{
public class HomeController : Controller
{
private readonly ILogger<HomeController> _logger;
private readonly IRazorViewRenderer _viewRenderService;
private readonly IHttpContextAccessor _httpContextAccessor;
public HomeController(ILogger<HomeController> logger, IRazorViewRenderer viewRenderService, IHttpContextAccessor httpContextAccessor)
{
_logger = logger;
_viewRenderService = viewRenderService;
_httpContextAccessor = httpContextAccessor;
}
public IActionResult Index()
{
return View();
}
public async Task<IActionResult> Persons()
{
var persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
if (_httpContextAccessor.HttpContext.Request.Method == HttpMethod.Post.Method)
{
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render View to PDF document
PdfDocument pdf = renderer.RenderRazorViewToPdf(_viewRenderService, "Views/Home/Persons.cshtml", persons);
Response.Headers.Add("Content-Disposition", "inline");
// Output PDF document
return File(pdf.BinaryData, "application/pdf", "viewToPdfMVCCore.pdf");
}
return View(persons);
}
public IActionResult Privacy()
{
return View();
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error()
{
return View(new ErrorViewModel { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });
}
}
}
RenderRazorToPdf
メソッドを使用した後、さらなる強化と修正が可能な PdfDocument オブジェクトを受け取ります。 生成されたPDFにPDFAまたはPDFUA形式への変換、デジタル署名の追加、あるいは必要に応じてPDFドキュメントの結合および分割が柔軟に行えます。 さらに、このライブラリを使用すると、ページの回転、注釈 や ブックマーク の挿入、PDFファイルへの独自の透かしの印刷が可能です。
ビューを追加
-
新しく追加されたPersonアクションを右クリックして、「ビューの追加」を選択します。
-
新しいスキャフォールド項目には「Razor View」を選択してください。
-
「リスト」テンプレートと「パーソン」モデルクラスを選択します。
以下のコードは「Persons」という名前の.cshtmlファイルを作成します。
-
「Views」フォルダー -> 「Home」フォルダー -> 「Persons.cshtml」ファイルに移動します。
「Persons」アクションを呼び出すボタンを追加するには、以下のコードを使用します:
@using (Html.BeginForm("Persons", "Home", FormMethod.Post))
{
<input type="submit" value="Print Person" />
}
@using (Html.BeginForm("Persons", "Home", FormMethod.Post))
{
<input type="submit" value="Print Person" />
}
ナビゲーションバーの上部にセクションを追加
-
「Views」フォルダ内の「Shared」フォルダ -> _Layout.cshtmlに移動します。 「Person」ナビゲーション項目を「Home」の後に配置します。
asp-action属性の値がファイル名と完全に一致していることを確認してください。この場合は「Persons」です。
<header>
<nav class="navbar navbar-expand-sm navbar-toggleable-sm navbar-light bg-white border-bottom box-shadow mb-3">
<div class="container-fluid">
<a class="navbar-brand" asp-area="" asp-controller="Home" asp-action="Index">ViewToPdfMVCCoreSample</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target=".navbar-collapse" aria-controls="navbarSupportedContent"
aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="navbar-collapse collapse d-sm-inline-flex justify-content-between">
<ul class="navbar-nav flex-grow-1">
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-controller="Home" asp-action="Index">Home</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-controller="Home" asp-action="Persons">Person</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-controller="Home" asp-action="Privacy">Privacy</a>
</li>
</ul>
</div>
</div>
</nav>
</header>
<header>
<nav class="navbar navbar-expand-sm navbar-toggleable-sm navbar-light bg-white border-bottom box-shadow mb-3">
<div class="container-fluid">
<a class="navbar-brand" asp-area="" asp-controller="Home" asp-action="Index">ViewToPdfMVCCoreSample</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target=".navbar-collapse" aria-controls="navbarSupportedContent"
aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="navbar-collapse collapse d-sm-inline-flex justify-content-between">
<ul class="navbar-nav flex-grow-1">
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-controller="Home" asp-action="Index">Home</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-controller="Home" asp-action="Persons">Person</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-controller="Home" asp-action="Privacy">Privacy</a>
</li>
</ul>
</div>
</div>
</nav>
</header>
Program.cs ファイルを編集
私たちは、IHttpContextAccessorとIRazorViewRendererインターフェースを依存性注入(DI)コンテナに登録します。 以下のコードを参考にしてください。
using IronPdf.Extensions.Mvc.Core;
using Microsoft.AspNetCore.Mvc.ViewFeatures;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllersWithViews();
builder.Services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
builder.Services.AddSingleton<ITempDataProvider, CookieTempDataProvider>();
// Register IRazorViewRenderer here
builder.Services.AddSingleton<IRazorViewRenderer, RazorViewRenderer>();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
using IronPdf.Extensions.Mvc.Core;
using Microsoft.AspNetCore.Mvc.ViewFeatures;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllersWithViews();
builder.Services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
builder.Services.AddSingleton<ITempDataProvider, CookieTempDataProvider>();
// Register IRazorViewRenderer here
builder.Services.AddSingleton<IRazorViewRenderer, RazorViewRenderer>();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
プロジェクトを実行
これにより、プロジェクトを実行してPDFドキュメントを生成する方法を示します。
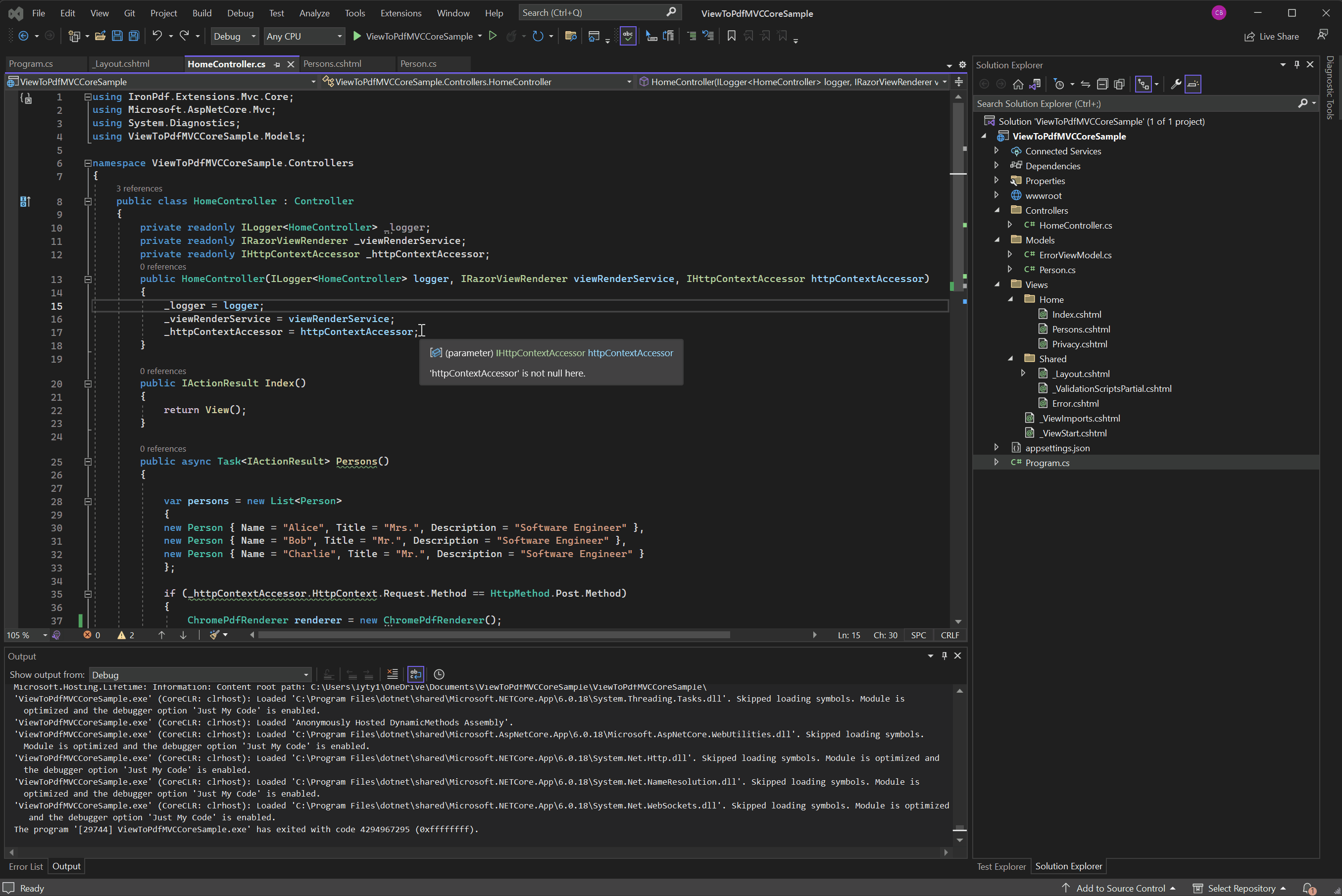
ASP.NET Core MVC プロジェクトをダウンロード
このガイドの完全なコードをダウンロードできます。それは、Visual StudioでASP.NET Core Web App(Model-View-Controller)プロジェクトとして開くことができる圧縮ファイルとして提供されます。