ASP.NET MVCでビューをPDFに変換する方法
ビューは、ASP.NETフレームワーク内で使用されるコンポーネントであり、WebアプリケーションでHTMLマークアップを生成するためのものです。 これは、ASP.NET MVC および ASP.NET Core MVC アプリケーションで一般的に使用される Model-View-Controller (MVC) パターンの一部です。 ビューは、HTMLコンテンツを動的にレンダリングすることで、ユーザーにデータを提示する役割を担っています。
ASP.NET Webアプリケーション (.NET Framework) MVCは、Microsoftが提供するWebアプリケーションフレームワークです。 それは、ウェブアプリケーションの開発を整理し、効率化するために、モデル・ビュー・コントローラー(MVC)として知られる構造化アーキテクチャパターンに従います。
- モデル: データ、ビジネスロジック、およびデータの整合性を管理します。
- ビュー: ユーザーインターフェイスを提示し、情報をレンダリングします。
-
コントローラー: ユーザー入力を処理し、リクエストを処理し、モデルとビューとの間の相互作用を調整します。
IronPDF は、ASP.NET MVC プロジェクト内のビューから PDF ファイルを作成するプロセスを簡素化します。 これにより、ASP.NET MVCでPDF生成が簡単かつ直接的になります。
IronPDF拡張パッケージ
IronPdf.Extensions.Mvc.Framework パッケージは、メインのIronPdfパッケージの拡張です。 ASP.NET MVCでビューをPDFドキュメントにレンダリングするためには、IronPdf.Extensions.Mvc.FrameworkパッケージとIronPdfパッケージの両方が必要です。
PM > Install-Package IronPdf.Extensions.Mvc.Framework
PM > Install-Package IronPdf.Extensions.Mvc.Framework
NuGetでインストール
Install-Package IronPdf.Extensions.Mvc.Framework
ビューをPDFにレンダリング
ビューをPDFファイルに変換するには、ASP.NET Webアプリケーション (.NET Framework) MVCプロジェクトが必要です。
クラスのモデルを追加
- 「Models」フォルダーに移動する
- 新しいC#クラスファイル「Person」を作成してください。このクラスは個々のデータを表すモデルとして機能します。 以下のコードスニペットを使用してください:
:path=/static-assets/pdf/content-code-examples/how-to/cshtml-to-pdf-mvc-framework-model.cs
namespace ViewToPdfMVCSample.Models
{
public class Person
{
public int Id { get; set; }
public string Name { get; set; }
public string Title { get; set; }
public string Description { get; set; }
}
}
Namespace ViewToPdfMVCSample.Models
Public Class Person
Public Property Id() As Integer
Public Property Name() As String
Public Property Title() As String
Public Property Description() As String
End Class
End Namespace
コントローラーを編集
「Controllers」フォルダに移動して、「HomeController」ファイルを開いてください。"Persons"アクションを追加します。 以下のコードを参照してください:
提供されたコードでは、ChromePdfRenderer クラスが最初に作成されます。 RenderView
メソッドを使用するには、HttpContext を提供し、「Persons.cshtml」ファイルへのパスを指定し、必要なデータを含む List を提供する必要があります。 ビューをレンダリングする際、ユーザーはRenderingOptionsを利用して余白をカスタマイズし、カスタムテキストおよびHTMLヘッダーとフッターを追加し、結果として得られるPDFドキュメントにページ番号を適用するオプションがあります。
次の内容にご注意ください。
File(pdf.BinaryData, "application/pdf", "viewToPdfMVC.pdf")
。using IronPdf;
using System.Collections.Generic;
using System.Web.Mvc;
using ViewToPdfMVCSample.Models;
namespace ViewToPdfMVCSample.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
// GET: Person
public ActionResult Persons()
{
var persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
if (HttpContext.Request.HttpMethod == "POST")
{
// Provide the path to your View file
var viewPath = "~/Views/Home/Persons.cshtml";
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render View to PDF document
PdfDocument pdf = renderer.RenderView(this.HttpContext, viewPath, persons);
Response.Headers.Add("Content-Disposition", "inline");
// View the PDF
return File(pdf.BinaryData, "application/pdf");
}
return View(persons);
}
public ActionResult About()
{
ViewBag.Message = "Your application description page.";
return View();
}
public ActionResult Contact()
{
ViewBag.Message = "Your contact page.";
return View();
}
}
}
using IronPdf;
using System.Collections.Generic;
using System.Web.Mvc;
using ViewToPdfMVCSample.Models;
namespace ViewToPdfMVCSample.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
// GET: Person
public ActionResult Persons()
{
var persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
if (HttpContext.Request.HttpMethod == "POST")
{
// Provide the path to your View file
var viewPath = "~/Views/Home/Persons.cshtml";
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render View to PDF document
PdfDocument pdf = renderer.RenderView(this.HttpContext, viewPath, persons);
Response.Headers.Add("Content-Disposition", "inline");
// View the PDF
return File(pdf.BinaryData, "application/pdf");
}
return View(persons);
}
public ActionResult About()
{
ViewBag.Message = "Your application description page.";
return View();
}
public ActionResult Contact()
{
ViewBag.Message = "Your contact page.";
return View();
}
}
}
Imports IronPdf
Imports System.Collections.Generic
Imports System.Web.Mvc
Imports ViewToPdfMVCSample.Models
Namespace ViewToPdfMVCSample.Controllers
Public Class HomeController
Inherits Controller
Public Function Index() As ActionResult
Return View()
End Function
' GET: Person
Public Function Persons() As ActionResult
'INSTANT VB NOTE: The local variable persons was renamed since Visual Basic will not allow local variables with the same name as their enclosing function or property:
Dim persons_Conflict = New List(Of Person) From {
New Person With {
.Name = "Alice",
.Title = "Mrs.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Bob",
.Title = "Mr.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Charlie",
.Title = "Mr.",
.Description = "Software Engineer"
}
}
If HttpContext.Request.HttpMethod = "POST" Then
' Provide the path to your View file
Dim viewPath = "~/Views/Home/Persons.cshtml"
Dim renderer As New ChromePdfRenderer()
' Render View to PDF document
Dim pdf As PdfDocument = renderer.RenderView(Me.HttpContext, viewPath, persons_Conflict)
Response.Headers.Add("Content-Disposition", "inline")
' View the PDF
Return File(pdf.BinaryData, "application/pdf")
End If
Return View(persons_Conflict)
End Function
Public Function About() As ActionResult
ViewBag.Message = "Your application description page."
Return View()
End Function
Public Function Contact() As ActionResult
ViewBag.Message = "Your contact page."
Return View()
End Function
End Class
End Namespace
一旦RenderView
メソッドを通じてPdfDocumentオブジェクトを取得したら、それにさまざまな改善や調整を加えることができます。 PDFをPDFAまたはPDFUA形式に変換したり、作成したPDFにデジタル署名を追加したり、必要に応じてPDFドキュメントを結合および分割したりできます。 さらに、このライブラリを使用すると、ページを回転させたり、注釈やブックマークを挿入したり、PDF ファイルに個別の透かしを適用したりできます。
ビューを追加
-
新しく追加されたPersonアクションを右クリックして、「ビューの追加」を選択します。
-
新しくスキャフォールディングされたアイテムには「MVC 5 View」を選択してください。
-
「リスト」テンプレートと「パーソン」モデルクラスを選択します。
以下のコードは「Persons」という名前の.cshtmlファイルを作成します。
-
「Views」フォルダー -> 「Home」フォルダー -> 「Persons.cshtml」ファイルに移動します。
「Persons」アクションを呼び出すボタンを追加するには、以下のコードを使用します:
@using (Html.BeginForm("Persons", "Home", FormMethod.Post))
{
<input type="submit" value="Print Person" />
}
@using (Html.BeginForm("Persons", "Home", FormMethod.Post))
{
<input type="submit" value="Print Person" />
}
ナビゲーションバーの上部にセクションを追加
-
「Views」フォルダで「Shared」フォルダ -> 「_Layout.cshtml」ファイルに移動します。「Home」の後に「Person」ナビゲーションアイテムを配置します。
ActionLinkメソッドの値が、今回の場合「Persons」と一致することを確認してください。
<nav class="navbar navbar-expand-sm navbar-toggleable-sm navbar-dark bg-dark">
<div class="container">
@Html.ActionLink("Application name", "Index", "Home", new { area = "" }, new { @class = "navbar-brand" })
<button type="button" class="navbar-toggler" data-bs-toggle="collapse" data-bs-target=".navbar-collapse" title="Toggle navigation" aria-controls="navbarSupportedContent"
aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse d-sm-inline-flex justify-content-between">
<ul class="navbar-nav flex-grow-1">
<li>@Html.ActionLink("Home", "Index", "Home", new { area = "" }, new { @class = "nav-link" })</li>
<li>@Html.ActionLink("Persons", "Persons", "Home", new { area = "" }, new { @class = "nav-link" })</li>
<li>@Html.ActionLink("About", "About", "Home", new { area = "" }, new { @class = "nav-link" })</li>
<li>@Html.ActionLink("Contact", "Contact", "Home", new { area = "" }, new { @class = "nav-link" })</li>
</ul>
</div>
</div>
</nav>
<nav class="navbar navbar-expand-sm navbar-toggleable-sm navbar-dark bg-dark">
<div class="container">
@Html.ActionLink("Application name", "Index", "Home", new { area = "" }, new { @class = "navbar-brand" })
<button type="button" class="navbar-toggler" data-bs-toggle="collapse" data-bs-target=".navbar-collapse" title="Toggle navigation" aria-controls="navbarSupportedContent"
aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse d-sm-inline-flex justify-content-between">
<ul class="navbar-nav flex-grow-1">
<li>@Html.ActionLink("Home", "Index", "Home", new { area = "" }, new { @class = "nav-link" })</li>
<li>@Html.ActionLink("Persons", "Persons", "Home", new { area = "" }, new { @class = "nav-link" })</li>
<li>@Html.ActionLink("About", "About", "Home", new { area = "" }, new { @class = "nav-link" })</li>
<li>@Html.ActionLink("Contact", "Contact", "Home", new { area = "" }, new { @class = "nav-link" })</li>
</ul>
</div>
</div>
</nav>
プロジェクトを実行
これにより、プロジェクトを実行してPDFドキュメントを生成する方法を示します。
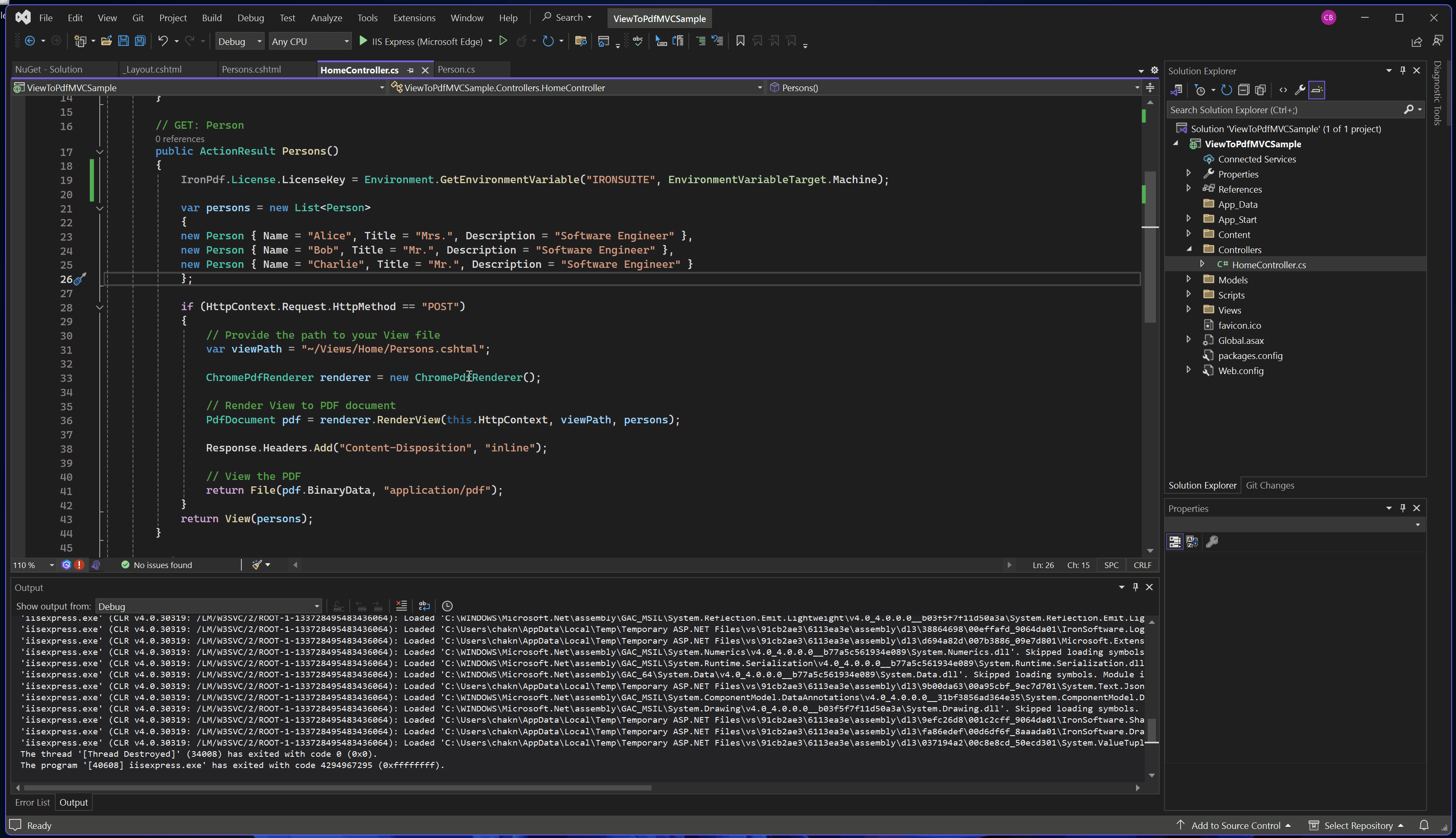
PDFを出力
ASP.NET MVCプロジェクトをダウンロード
このガイドの完全なコードをダウンロードできます。それは、Visual StudioでASP.NET Webアプリケーション (.NET Framework) MVCプロジェクトとして開くことができるZIPファイルとして提供されます。