如何在 ASP.NET Core Web 应用程序中将 Razor 页面转换为 PDF
Razor 页面是一个扩展名为 .cshtml 的文件,它结合了 C# 和 HTML 来生成网页内容。在 ASP.NET Core 中,Razor 页面是一种更简单的网络应用程序代码组织方式,非常适合只读页面或简单数据输入页面。
ASP.NET Core Web 应用程序是使用 ASP.NET Core 构建的 Web 应用程序,ASP.NET Core 是一个用于开发现代 Web 应用程序的跨平台框架。
IronPDF 简化了在 ASP.NET Core Web App 项目中从 Razor Pages 创建 PDF 文件的过程。这使得在 ASP.NET Core Web Apps 中生成 PDF 变得简单而直接。
如何在 ASP.NET Core Web 应用程序中将 Razor 页面转换为 PDF
IronPDF 扩展包
IronPdf.Extensions.Razor包是主包IronPdf**的扩展。IronPdf.Extensions.Razor 和 IronPdf 包都是在 ASP.NET Core Web 应用程序中将 Razor 页面呈现为 PDF 文档所必需的。
Install-Package IronPdf.Extensions.Razor
安装使用 NuGet
安装包 IronPdf.Extensions.Razor
将 Razor 页面渲染为 PDF 文件
您需要一个ASP.NET Core Web App项目来将Razor页面转换为PDF文件。
创建一个模型类
- 在项目中新建一个文件夹,命名为 "模型"。
- 在文件夹中添加一个标准 C# 类,命名为 "Person"。该类将作为个人数据的模型。使用以下代码片段:
:path=/static-assets/pdf/content-code-examples/how-to/cshtml-to-pdf-razor-model.cs
namespace RazorPageSample.Models
{
public class Person
{
public int Id { get; set; }
public string Name { get; set; }
public string Title { get; set; }
public string Description { get; set; }
}
}
Namespace RazorPageSample.Models
Public Class Person
Public Property Id() As Integer
Public Property Name() As String
Public Property Title() As String
Public Property Description() As String
End Class
End Namespace
添加一个剃须刀页面
在 "页面 "文件夹下添加一个空的 Razor 页面,并命名为 "persons.cshtml"。
- 使用下面提供的代码示例修改新创建的 "Persons.cshtml "文件。
下面的代码用于在浏览器中显示信息。
@page
@using RazorPageSample.Models;
@model RazorPageSample.Pages.PersonsModel
@{
}
<table class="table">
<tr>
<th>Name</th>
<th>Title</th>
<th>Description</th>
</tr>
@foreach (var person in ViewData ["personList"] as List<Person>)
{
<tr>
<td>@person.Name</td>
<td>@person.Title</td>
<td>@person.Description</td>
</tr>
}
</table>
<form method="post">
<button type="submit">print</button>
</form>
@page
@using RazorPageSample.Models;
@model RazorPageSample.Pages.PersonsModel
@{
}
<table class="table">
<tr>
<th>Name</th>
<th>Title</th>
<th>Description</th>
</tr>
@foreach (var person in ViewData ["personList"] as List<Person>)
{
<tr>
<td>@person.Name</td>
<td>@person.Title</td>
<td>@person.Description</td>
</tr>
}
</table>
<form method="post">
<button type="submit">print</button>
</form>
接下来,下面的代码首先实例化了ChromePdfRenderer类。将this传递给 "RenderRazorToPdf "方法就足以将该Razor页面转换为PDF文档。
用户可以使用渲染选项中的所有功能。这些功能包括 页码 在生成的 PDF 中,设置自定义页边距,并添加自定义 文本以及 HTML 页眉和页脚.
- 打开 "Persons.cshtml "文件下拉菜单,查看 "Persons.cshtml.cs "文件。
- 用下面的代码修改 "Persons.cshtml.cs"。
请注意
File(pdf.BinaryData、"application/pdf")
.但是,在浏览器中查看 PDF 后再下载,会导致 PDF 文档损坏。using IronPdf.Razor.Pages;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using RazorPageSample.Models;
namespace RazorPageSample.Pages
{
public class PersonsModel : PageModel
{
[BindProperty(SupportsGet = true)]
public List<Person> persons { get; set; }
public void OnGet()
{
persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
ViewData ["personList"] = persons;
}
public IActionResult OnPostAsync()
{
persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
ViewData ["personList"] = persons;
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render Razor Page to PDF document
PdfDocument pdf = renderer.RenderRazorToPdf(this);
Response.Headers.Add("Content-Disposition", "inline");
return File(pdf.BinaryData, "application/pdf", "razorPageToPdf.pdf");
// View output PDF on broswer
return File(pdf.BinaryData, "application/pdf");
}
}
}
using IronPdf.Razor.Pages;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using RazorPageSample.Models;
namespace RazorPageSample.Pages
{
public class PersonsModel : PageModel
{
[BindProperty(SupportsGet = true)]
public List<Person> persons { get; set; }
public void OnGet()
{
persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
ViewData ["personList"] = persons;
}
public IActionResult OnPostAsync()
{
persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
ViewData ["personList"] = persons;
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render Razor Page to PDF document
PdfDocument pdf = renderer.RenderRazorToPdf(this);
Response.Headers.Add("Content-Disposition", "inline");
return File(pdf.BinaryData, "application/pdf", "razorPageToPdf.pdf");
// View output PDF on broswer
return File(pdf.BinaryData, "application/pdf");
}
}
}
Imports IronPdf.Razor.Pages
Imports Microsoft.AspNetCore.Mvc
Imports Microsoft.AspNetCore.Mvc.RazorPages
Imports RazorPageSample.Models
Namespace RazorPageSample.Pages
Public Class PersonsModel
Inherits PageModel
<BindProperty(SupportsGet := True)>
Public Property persons() As List(Of Person)
Public Sub OnGet()
persons = New List(Of Person) From {
New Person With {
.Name = "Alice",
.Title = "Mrs.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Bob",
.Title = "Mr.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Charlie",
.Title = "Mr.",
.Description = "Software Engineer"
}
}
ViewData ("personList") = persons
End Sub
Public Function OnPostAsync() As IActionResult
persons = New List(Of Person) From {
New Person With {
.Name = "Alice",
.Title = "Mrs.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Bob",
.Title = "Mr.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Charlie",
.Title = "Mr.",
.Description = "Software Engineer"
}
}
ViewData ("personList") = persons
Dim renderer As New ChromePdfRenderer()
' Render Razor Page to PDF document
Dim pdf As PdfDocument = renderer.RenderRazorToPdf(Me)
Response.Headers.Add("Content-Disposition", "inline")
Return File(pdf.BinaryData, "application/pdf", "razorPageToPdf.pdf")
' View output PDF on broswer
Return File(pdf.BinaryData, "application/pdf")
End Function
End Class
End Namespace
RenderRazorToPdf "方法返回一个PDFDocument对象,该对象可以进行其他处理和编辑。您可以将 PDF 导出为 PDF/A 或 PDFUA应用 数字签名 或 合并和拆分 PDF 文档。该方法还可以旋转页面,添加 注释 或 书签和 加盖自定义水印 到您的 PDF 上。
在顶部导航栏添加一个部分
- 导航至页面文件夹 -> 共享文件夹 -> _Layout.cshtml。将 "人员 "导航项放在 "主页 "之后。
确保 asp-page 属性的值与我们的文件名(本例中为 "Persons")完全匹配。
<header>
<nav class="navbar navbar-expand-sm navbar-toggleable-sm navbar-light bg-white border-bottom box-shadow mb-3">
<div class="container">
<a class="navbar-brand" asp-area="" asp-page="/Index">RazorPageSample</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target=".navbar-collapse" aria-controls="navbarSupportedContent"
aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="navbar-collapse collapse d-sm-inline-flex justify-content-between">
<ul class="navbar-nav flex-grow-1">
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Index">Home</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Persons">Person</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Privacy">Privacy</a>
</li>
</ul>
</div>
</div>
</nav>
</header>
<header>
<nav class="navbar navbar-expand-sm navbar-toggleable-sm navbar-light bg-white border-bottom box-shadow mb-3">
<div class="container">
<a class="navbar-brand" asp-area="" asp-page="/Index">RazorPageSample</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target=".navbar-collapse" aria-controls="navbarSupportedContent"
aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="navbar-collapse collapse d-sm-inline-flex justify-content-between">
<ul class="navbar-nav flex-grow-1">
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Index">Home</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Persons">Person</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Privacy">Privacy</a>
</li>
</ul>
</div>
</div>
</nav>
</header>
运行项目
向您演示如何运行项目并生成 PDF 文档。
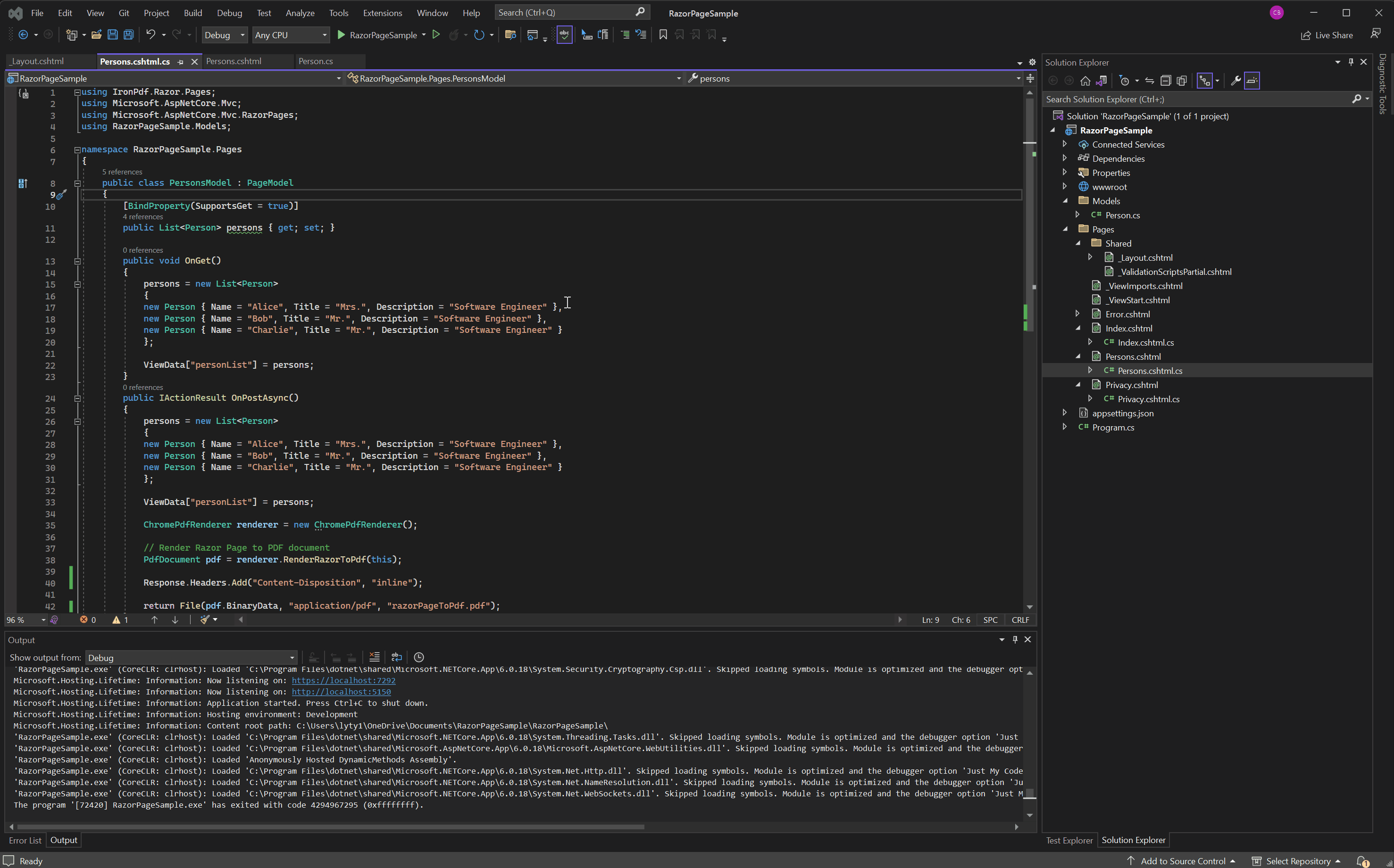
下载 ASP.NET Core Web 应用程序项目
您可以下载本指南的完整代码。它是一个压缩文件,你可以在 Visual Studio 中作为 ASP.NET Core Web App 项目打开。