如何将图像转换为 PDF
将图像转换为 PDF 是一个有用的过程,可将多个图像文件合并在一起 (如 JPG、PNG 或 TIFF) 合并成一个 PDF 文档。这样做通常是为了创建数字作品集、演示文稿或报告,从而更容易以更有条理和普遍可读的格式共享和存储图像集合。
通过 IronPdf,您可以将单张或多张图片转换为 PDF 文件,并使用独特的 图像位置和行为.这些行为包括贴合页面、页面居中和裁剪页面。此外,您还可以添加 文本和 HTML 页眉和页脚, 应用水印设置自定义页面尺寸,并包含背景和前景叠加层。
如何将图像转换为 PDF
开始在您的项目中使用IronPDF,并立即获取免费试用。
查看 IronPDF 上 Nuget 用于快速安装和部署。它有超过800万次下载,正在使用C#改变PDF。
考虑安装 IronPDF DLL 直接。下载并手动安装到您的项目或GAC表单中: IronPdf.zip
将图像转换为 PDF 示例
使用 ImageToPdfConverter 类中的 ImageToPdf
静态方法将图像转换为 PDF 文档。该方法只需提供图像的文件路径,就能将图像转换为具有默认图像位置和行为的 PDF 文档。支持的图像格式包括 .bmp、.jpeg、.jpg、.gif、.png、.svg、.tif、.tiff、.webp、.apng、.avif、.cur、.dib、.ico、.jfif、.jif、.jpe、.pjp 和 .pjpeg。
图片样本
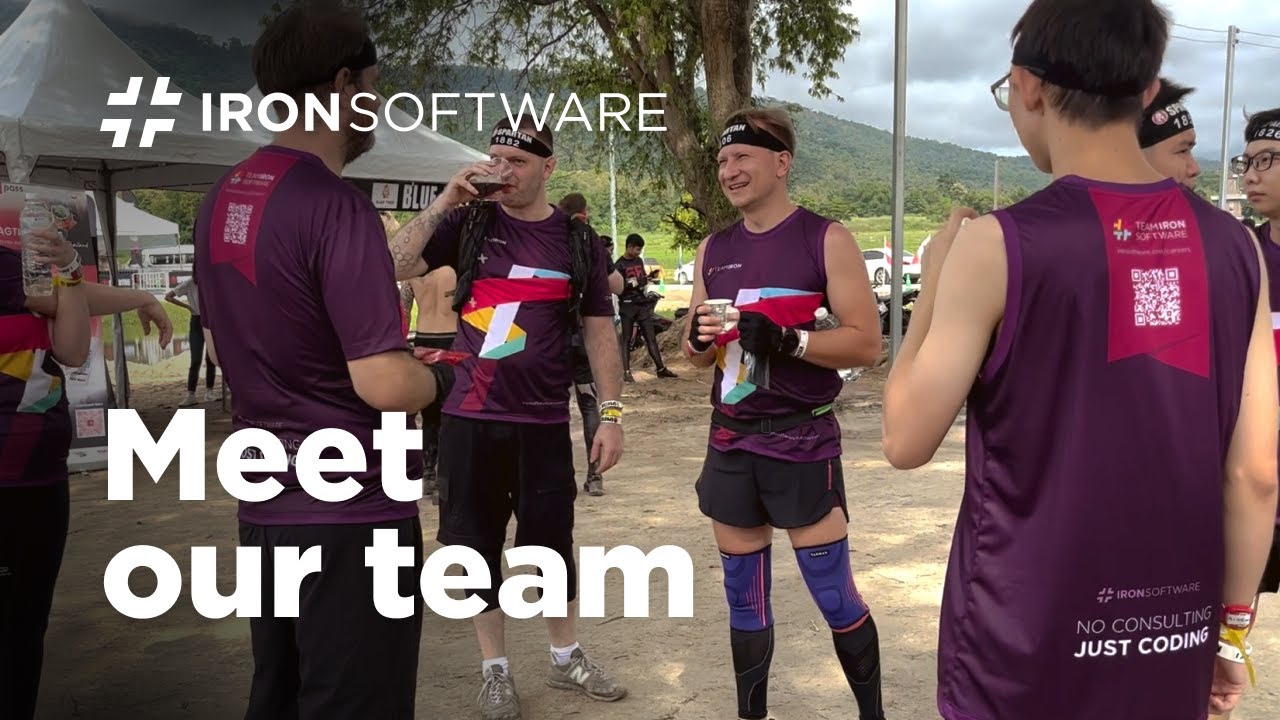
代码
:path=/static-assets/pdf/content-code-examples/how-to/image-to-pdf-convert-one-image.cs
using IronPdf;
string imagePath = "meetOurTeam.jpg";
// Convert an image to a PDF
PdfDocument pdf = ImageToPdfConverter.ImageToPdf(imagePath);
// Export the PDF
pdf.SaveAs("imageToPdf.pdf");
Imports IronPdf
Private imagePath As String = "meetOurTeam.jpg"
' Convert an image to a PDF
Private pdf As PdfDocument = ImageToPdfConverter.ImageToPdf(imagePath)
' Export the PDF
pdf.SaveAs("imageToPdf.pdf")
输出 PDF
将图像转换为 PDF 示例
要将多个图像转换为 PDF 文档,应提供一个包含文件路径的 IEnumerable 对象,而不是单个文件路径,如上例所示。这将再次生成具有默认图像位置和行为的 PDF 文档。
:path=/static-assets/pdf/content-code-examples/how-to/image-to-pdf-convert-multiple-images.cs
using IronPdf;
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
// Retrieve all JPG and JPEG image paths in the 'images' folder.
IEnumerable<String> imagePaths = Directory.EnumerateFiles("images").Where(f => f.EndsWith(".jpg") || f.EndsWith(".jpeg"));
// Convert images to a PDF
PdfDocument pdf = ImageToPdfConverter.ImageToPdf(imagePaths);
// Export the PDF
pdf.SaveAs("imagesToPdf.pdf");
Imports IronPdf
Imports System
Imports System.Collections.Generic
Imports System.IO
Imports System.Linq
' Retrieve all JPG and JPEG image paths in the 'images' folder.
Private imagePaths As IEnumerable(Of String) = Directory.EnumerateFiles("images").Where(Function(f) f.EndsWith(".jpg") OrElse f.EndsWith(".jpeg"))
' Convert images to a PDF
Private pdf As PdfDocument = ImageToPdfConverter.ImageToPdf(imagePaths)
' Export the PDF
pdf.SaveAs("imagesToPdf.pdf")
输出 PDF
图像位置和行为
为了方便使用,我们提供了一系列有用的图片位置和行为选项。例如,您可以将图片居中放置在页面上,或者在保持图片纵横比的情况下将其调整到页面大小。所有可用的图片位置和行为如下:
- TopLeftCornerOfPage: 图像置于页面左上角。
- 页面右上角: 图像位于页面右上角。
- CenteredOnPage: 图像位于页面中心。
- FitToPageAndMaintainAspectRatio: 图像适合页面并保持其原始宽高比。
- BottomLeftCornerOfPage: 图像位于页面的左下角。
- BottomRightCornerOfPage(页面右下角): 图像位于页面右下角。
- 适合页面: 图像适合页面。
- 裁剪页面: 调整页面以适合图像。
:path=/static-assets/pdf/content-code-examples/how-to/image-to-pdf-convert-one-image-image-behavior.cs
using IronPdf;
using IronPdf.Imaging;
string imagePath = "meetOurTeam.jpg";
// Convert an image to a PDF with image behavior of centered on page
PdfDocument pdf = ImageToPdfConverter.ImageToPdf(imagePath, ImageBehavior.CenteredOnPage);
// Export the PDF
pdf.SaveAs("imageToPdf.pdf");
Imports IronPdf
Imports IronPdf.Imaging
Private imagePath As String = "meetOurTeam.jpg"
' Convert an image to a PDF with image behavior of centered on page
Private pdf As PdfDocument = ImageToPdfConverter.ImageToPdf(imagePath, ImageBehavior.CenteredOnPage)
' Export the PDF
pdf.SaveAs("imageToPdf.pdf")
图像行为比较
![]() 页面左上角 | ![]() 页面右上角 |
![]() 以页面为中心 | ![]() 适合页面并保持宽高比 |
![]() 页面左下角 | ![]() 页面右下角 |
![]() FitToPage | ![]() 作物页面 |
应用渲染选项
在 ImageToPdf
静态方法的引擎盖下,将各种类型的图像转换为 PDF 文档的关键在于将图像作为 HTML img 标签导入,然后将 HTML 转换为 PDF。这也是我们可以将ChromePdfRenderOptions对象作为 "ImageToPdf "方法的第三个参数来直接自定义渲染过程的原因。
:path=/static-assets/pdf/content-code-examples/how-to/image-to-pdf-convert-one-image-rendering-options.cs
using IronPdf;
string imagePath = "meetOurTeam.jpg";
ChromePdfRenderOptions options = new ChromePdfRenderOptions()
{
HtmlHeader = new HtmlHeaderFooter()
{
HtmlFragment = "<h1 style='color: #2a95d5;'>Content Header</h1>",
DrawDividerLine = true,
},
};
// Convert an image to a PDF with custom header
PdfDocument pdf = ImageToPdfConverter.ImageToPdf(imagePath, options: options);
// Export the PDF
pdf.SaveAs("imageToPdfWithHeader.pdf");
Imports IronPdf
Private imagePath As String = "meetOurTeam.jpg"
Private options As New ChromePdfRenderOptions() With {
.HtmlHeader = New HtmlHeaderFooter() With {
.HtmlFragment = "<h1 style='color: #2a95d5;'>Content Header</h1>",
.DrawDividerLine = True
}
}
' Convert an image to a PDF with custom header
Private pdf As PdfDocument = ImageToPdfConverter.ImageToPdf(imagePath, options:= options)
' Export the PDF
pdf.SaveAs("imageToPdfWithHeader.pdf")
输出 PDF
如果您想将 PDF 文档转换或光栅化为图像,请参阅我们的 如何将PDF光栅化为图像 文章