如何在 C&num 中编辑 PDF;
简介
在 IronPDF 库中,Iron Software 已将许多不同的 PDF 编辑功能简化为易于阅读和理解的方法。无论是添加签名、添加 HTML 页脚、加盖水印还是添加注释。IronPDF 是一款适合您的工具,它能让您拥有可读代码、编程式 PDF 生成、轻松调试以及轻松部署到任何支持的环境或平台。
在编辑 PDF 方面,IronPDF 拥有数不胜数的功能。在本教程文章中,我们将通过代码示例和一些解释来介绍其中的一些主要功能。
通过本文,您将对如何使用 IronPDF 用 C# 编辑 PDF 有一个很好的了解。
目录
编辑文档结构
- 合并和拆分 PDF 文件
编辑文档属性
- 压缩PDF
编辑 PDF 内容
- 添加背景和前景
印章和水印
- 长度代码示例
在 PDF 文件中使用表格
<
编辑文档结构
操作页面
使用 IronPDF,在特定索引中添加 PDF、将页面作为一个范围或逐个复制出来,以及从任何 PDF 中删除页面都轻而易举。
添加页面
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-1.cs
var pdf = new PdfDocument("report.pdf");
var renderer = new ChromePdfRenderer();
var coverPagePdf = renderer.RenderHtmlAsPdf("<h1>Cover Page</h1><hr>");
pdf.PrependPdf(coverPagePdf);
pdf.SaveAs("report_with_cover.pdf");
Dim pdf = New PdfDocument("report.pdf")
Dim renderer = New ChromePdfRenderer()
Dim coverPagePdf = renderer.RenderHtmlAsPdf("<h1>Cover Page</h1><hr>")
pdf.PrependPdf(coverPagePdf)
pdf.SaveAs("report_with_cover.pdf")
复制页
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-2.cs
var pdf = new PdfDocument("report.pdf");
// Copy pages 5 to 7 and save them as a new document.
pdf.CopyPages(4, 6).SaveAs("report_highlight.pdf");
Dim pdf = New PdfDocument("report.pdf")
' Copy pages 5 to 7 and save them as a new document.
pdf.CopyPages(4, 6).SaveAs("report_highlight.pdf")
删除页面
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-3.cs
var pdf = new PdfDocument("report.pdf");
// Remove the last page from the PDF and save again
pdf.RemovePage(pdf.PageCount - 1);
pdf.SaveAs("report_minus_one_page.pdf");
Dim pdf = New PdfDocument("report.pdf")
' Remove the last page from the PDF and save again
pdf.RemovePage(pdf.PageCount - 1)
pdf.SaveAs("report_minus_one_page.pdf")
合并和拆分 PDF 文件
使用 IronPDF 直观的 API,可轻松实现将 PDF 合并为一个 PDF 或分割现有 PDF。
将多个现有 PDF 文件合并为一个 PDF 文档
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-4.cs
var pdfs = new List<PdfDocument>
{
PdfDocument.FromFile("A.pdf"),
PdfDocument.FromFile("B.pdf"),
PdfDocument.FromFile("C.pdf")
};
PdfDocument mergedPdf = PdfDocument.Merge(pdfs);
mergedPdf.SaveAs("merged.pdf");
foreach (var pdf in pdfs)
{
pdf.Dispose();
}
Dim pdfs = New List(Of PdfDocument) From {PdfDocument.FromFile("A.pdf"), PdfDocument.FromFile("B.pdf"), PdfDocument.FromFile("C.pdf")}
Dim mergedPdf As PdfDocument = PdfDocument.Merge(pdfs)
mergedPdf.SaveAs("merged.pdf")
For Each pdf In pdfs
pdf.Dispose()
Next pdf
要查看代码示例页面上的两个或更多 PDF,请访问 这里.
分割 PDF 文件并提取页面
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-5.cs
var pdf = new PdfDocument("sample.pdf");
// Take the first page
var pdf_page1 = pdf.CopyPage(0);
pdf_page1.SaveAs("Split1.pdf");
// Take the pages 2 & 3
var pdf_page2_3 = pdf.CopyPages(1, 2);
pdf_page2_3.SaveAs("Spli2t.pdf");
Dim pdf = New PdfDocument("sample.pdf")
' Take the first page
Dim pdf_page1 = pdf.CopyPage(0)
pdf_page1.SaveAs("Split1.pdf")
' Take the pages 2 & 3
Dim pdf_page2_3 = pdf.CopyPages(1, 2)
pdf_page2_3.SaveAs("Spli2t.pdf")
要查看代码示例页面中的拆分和提取页面,请访问 这里.
编辑文档属性
添加和使用 PDF 元数据
您可以轻松使用 IronPDF 浏览和编辑 PDF 元数据:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-6.cs
// Open an Encrypted File, alternatively create a new PDF from Html
var pdf = PdfDocument.FromFile("encrypted.pdf", "password");
// Edit file metadata
pdf.MetaData.Author = "Satoshi Nakamoto";
pdf.MetaData.Keywords = "SEO, Friendly";
pdf.MetaData.ModifiedDate = System.DateTime.Now;
// Edit file security settings
// The following code makes a PDF read only and will disallow copy & paste and printing
pdf.SecuritySettings.RemovePasswordsAndEncryption();
pdf.SecuritySettings.MakePdfDocumentReadOnly("secret-key");
pdf.SecuritySettings.AllowUserAnnotations = false;
pdf.SecuritySettings.AllowUserCopyPasteContent = false;
pdf.SecuritySettings.AllowUserFormData = false;
pdf.SecuritySettings.AllowUserPrinting = IronPdf.Security.PdfPrintSecurity.FullPrintRights;
// Change or set the document encryption password
pdf.SecuritySettings.OwnerPassword = "top-secret"; // password to edit the pdf
pdf.SecuritySettings.UserPassword = "shareable"; // password to open the pdf
pdf.SaveAs("secured.pdf");
Imports System
' Open an Encrypted File, alternatively create a new PDF from Html
Dim pdf = PdfDocument.FromFile("encrypted.pdf", "password")
' Edit file metadata
pdf.MetaData.Author = "Satoshi Nakamoto"
pdf.MetaData.Keywords = "SEO, Friendly"
pdf.MetaData.ModifiedDate = DateTime.Now
' Edit file security settings
' The following code makes a PDF read only and will disallow copy & paste and printing
pdf.SecuritySettings.RemovePasswordsAndEncryption()
pdf.SecuritySettings.MakePdfDocumentReadOnly("secret-key")
pdf.SecuritySettings.AllowUserAnnotations = False
pdf.SecuritySettings.AllowUserCopyPasteContent = False
pdf.SecuritySettings.AllowUserFormData = False
pdf.SecuritySettings.AllowUserPrinting = IronPdf.Security.PdfPrintSecurity.FullPrintRights
' Change or set the document encryption password
pdf.SecuritySettings.OwnerPassword = "top-secret" ' password to edit the pdf
pdf.SecuritySettings.UserPassword = "shareable" ' password to open the pdf
pdf.SaveAs("secured.pdf")
数字签名
IronPDF 具有使用 .pfx 和 .p12 X509Certificate2 数字证书对新的或现有 PDF 文件进行数字签名的选项。
一旦对 PDF 文件进行了签名,在未验证证书的情况下就不能对其进行修改。这就确保了真实性。
要使用 Adobe Reader 免费生成签名证书,请阅读 https://helpx.adobe.com/acrobat/using/digital-ids.html 。
除加密签名外,手写签名图像或公司印章图像也可用于使用 IronPDF 签名。
只需一行代码即可对现有 PDF 进行加密签名!
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-7.cs
using IronPdf;
using IronPdf.Signing;
new IronPdf.Signing.PdfSignature("Iron.p12", "123456").SignPdfFile("any.pdf");
Imports IronPdf
Imports IronPdf.Signing
Call (New IronPdf.Signing.PdfSignature("Iron.p12", "123456")).SignPdfFile("any.pdf")
控制能力更强的高级示例:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-8.cs
using IronPdf;
// Step 1. Create a PDF
var renderer = new ChromePdfRenderer();
var doc = renderer.RenderHtmlAsPdf("<h1>Testing 2048 bit digital security</h1>");
// Step 2. Create a Signature.
// You may create a .pfx or .p12 PDF signing certificate using Adobe Acrobat Reader.
// Read: https://helpx.adobe.com/acrobat/using/digital-ids.html
var signature = new IronPdf.Signing.PdfSignature("Iron.pfx", "123456")
{
// Step 3. Optional signing options and a handwritten signature graphic
SigningContact = "support@ironsoftware.com",
SigningLocation = "Chicago, USA",
SigningReason = "To show how to sign a PDF"
};
//Step 4. Sign the PDF with the PdfSignature. Multiple signing certificates may be used
doc.Sign(signature);
//Step 5. The PDF is not signed until saved to file, steam or byte array.
doc.SaveAs("signed.pdf");
Imports IronPdf
' Step 1. Create a PDF
Private renderer = New ChromePdfRenderer()
Private doc = renderer.RenderHtmlAsPdf("<h1>Testing 2048 bit digital security</h1>")
' Step 2. Create a Signature.
' You may create a .pfx or .p12 PDF signing certificate using Adobe Acrobat Reader.
' Read: https://helpx.adobe.com/acrobat/using/digital-ids.html
Private signature = New IronPdf.Signing.PdfSignature("Iron.pfx", "123456") With {
.SigningContact = "support@ironsoftware.com",
.SigningLocation = "Chicago, USA",
.SigningReason = "To show how to sign a PDF"
}
'Step 4. Sign the PDF with the PdfSignature. Multiple signing certificates may be used
doc.Sign(signature)
'Step 5. The PDF is not signed until saved to file, steam or byte array.
doc.SaveAs("signed.pdf")
PDF 附件
IronPDF 完全支持在 PDF 文档中添加和删除附件。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-9.cs
var Renderer = new ChromePdfRenderer();
var myPdf = Renderer.RenderHtmlFileAsPdf("my-content.html");
// Here we can add an attachment with a name and byte[]
var attachment1 = myPdf.Attachments.AddAttachment("attachment_1", example_attachment);
// And here is an example of removing an attachment
myPdf.Attachments.RemoveAttachment(attachment1);
myPdf.SaveAs("my-content.pdf");
Dim Renderer = New ChromePdfRenderer()
Dim myPdf = Renderer.RenderHtmlFileAsPdf("my-content.html")
' Here we can add an attachment with a name and byte[]
Dim attachment1 = myPdf.Attachments.AddAttachment("attachment_1", example_attachment)
' And here is an example of removing an attachment
myPdf.Attachments.RemoveAttachment(attachment1)
myPdf.SaveAs("my-content.pdf")
压缩 PDF
IronPDF 支持压缩 PDF。减少PDF文件大小的方法之一是减少PdfDocument中嵌入图像的大小。在 IronPDF 中,我们可以调用 CompressImages
方法。
按照调整 JPEG 大小的工作原理,100% 的质量几乎没有损失,而 1% 的质量则是非常低的输出图像。一般来说,90% 及以上为高质量,80%-90% 为中等质量,70%-80% 为低质量。降低到70%以下会产生低质量的图像,但这样做可能会大大减小PdfDocument的总文件大小。
请尝试使用不同的值来感受质量与文件大小的范围,以获得满足您要求的最佳平衡。质量下降的明显程度最终取决于输入图像的类型,有些图像的清晰度可能比其他图像下降得更明显。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-10.cs
var pdf = new PdfDocument("document.pdf");
// Quality parameter can be 1-100, where 100 is 100% of original quality
pdf.CompressImages(60);
pdf.SaveAs("document_compressed.pdf");
Dim pdf = New PdfDocument("document.pdf")
' Quality parameter can be 1-100, where 100 is 100% of original quality
pdf.CompressImages(60)
pdf.SaveAs("document_compressed.pdf")
第二个可选参数可以根据 PDF 文档中的可见尺寸缩减图像分辨率。请注意,这可能会导致某些图像配置失真:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-11.cs
var pdf = new PdfDocument("document.pdf");
pdf.CompressImages(90, true);
pdf.SaveAs("document_scaled_compressed.pdf");
Dim pdf = New PdfDocument("document.pdf")
pdf.CompressImages(90, True)
pdf.SaveAs("document_scaled_compressed.pdf")
编辑 PDF 内容
添加页眉和页脚
您可以轻松地为 PDF 添加页眉和页脚。IronPDF 有两种不同的 "页眉页脚",即 "TextHeaderFooter "和 "HtmlHeaderFooter"。TextHeaderFooter 更适用于只有文本的页眉和页脚,而且可能需要使用合并字段,如""{页码} 的 {总页数}"`.HtmlHeaderFooter 是一款先进的页眉和页脚工具,可在其中使用任何 HTML 并格式化整齐。
HTML 页眉和页脚
HTML 页眉和页脚将使用您的 HTML 渲染版本作为 PDF 文档的页眉或页脚,实现完美的像素布局。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-12.cs
var renderer = new ChromePdfRenderer();
// Build a footer using html to style the text
// mergeable fields are:
// {page} {total-pages} {url} {date} {time} {html-title} & {pdf-title}
renderer.RenderingOptions.HtmlFooter = new HtmlHeaderFooter()
{
MaxHeight = 15, //millimeters
HtmlFragment = "<center><i>{page} of {total-pages}<i></center>",
DrawDividerLine = true
};
// Build a header using an image asset
// Note the use of BaseUrl to set a relative path to the assets
renderer.RenderingOptions.HtmlHeader = new HtmlHeaderFooter()
{
MaxHeight = 20, //millimeters
HtmlFragment = "<img src='logo.png'>",
BaseUrl = new System.Uri(@"C:\assets\images").AbsoluteUri
};
Dim renderer = New ChromePdfRenderer()
' Build a footer using html to style the text
' mergeable fields are:
' {page} {total-pages} {url} {date} {time} {html-title} & {pdf-title}
renderer.RenderingOptions.HtmlFooter = New HtmlHeaderFooter() With {
.MaxHeight = 15,
.HtmlFragment = "<center><i>{page} of {total-pages}<i></center>",
.DrawDividerLine = True
}
' Build a header using an image asset
' Note the use of BaseUrl to set a relative path to the assets
renderer.RenderingOptions.HtmlHeader = New HtmlHeaderFooter() With {
.MaxHeight = 20,
.HtmlFragment = "<img src='logo.png'>",
.BaseUrl = (New System.Uri("C:\assets\images")).AbsoluteUri
}
有关包含多个使用案例的完整深入示例,请参阅以下教程: 这里.
文本页眉和页脚
基本的页眉和页脚是 TextHeaderFooter,请参见下面的示例。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-13.cs
var renderer = new ChromePdfRenderer
{
RenderingOptions =
{
FirstPageNumber = 1, // use 2 if a cover-page will be appended
// Add a header to every page easily:
TextHeader =
{
DrawDividerLine = true,
CenterText = "{url}",
Font = IronSoftware.Drawing.FontTypes.Helvetica,
FontSize = 12
},
// Add a footer too:
TextFooter =
{
DrawDividerLine = true,
Font = IronSoftware.Drawing.FontTypes.Arial,
FontSize = 10,
LeftText = "{date} {time}",
RightText = "{page} of {total-pages}"
}
}
};
Dim renderer = New ChromePdfRenderer With {
.RenderingOptions = {
FirstPageNumber = 1, TextHeader = {
DrawDividerLine = True,
CenterText = "{url}",
Font = IronSoftware.Drawing.FontTypes.Helvetica,
FontSize = 12
},
TextFooter = {
DrawDividerLine = True,
Font = IronSoftware.Drawing.FontTypes.Arial,
FontSize = 10,
LeftText = "{date} {time}",
RightText = "{page} of {total-pages}"
}
}
}
我们还有以下合并字段,这些字段的值将在渲染时被替换: .{页码}
, {总页数}
, {网址}
, {日期}
, {时间}
, {html-title}
, {pdf标题}
查找并替换 PDF 中的文本
在 PDF 文件中创建占位符,并以编程方式替换它们,或者使用我们的 ReplaceTextOnPage
方法替换文本短语的所有实例。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-14.cs
// Using an existing PDF
var pdf = PdfDocument.FromFile("sample.pdf");
// Parameters
int pageIndex = 1;
string oldText = ".NET 6"; // Old text to remove
string newText = ".NET 7"; // New text to add
// Replace Text on Page
pdf.ReplaceTextOnPage(pageIndex, oldText, newText);
// Placeholder Template Example
pdf.ReplaceTextOnPage(pageIndex, "[DATE]", "01/01/2000");
// Save your new PDF
pdf.SaveAs("new_sample.pdf");
' Using an existing PDF
Dim pdf = PdfDocument.FromFile("sample.pdf")
' Parameters
Dim pageIndex As Integer = 1
Dim oldText As String = ".NET 6" ' Old text to remove
Dim newText As String = ".NET 7" ' New text to add
' Replace Text on Page
pdf.ReplaceTextOnPage(pageIndex, oldText, newText)
' Placeholder Template Example
pdf.ReplaceTextOnPage(pageIndex, "[DATE]", "01/01/2000")
' Save your new PDF
pdf.SaveAs("new_sample.pdf")
要查看代码示例页面上的查找和替换文本示例,请访问 这里
大纲和书签
大纲或 "书签 "为浏览 PDF 关键页面提供了一种方法。在 Adobe Acrobat Reader 中,这些书签 (可嵌套) 显示在应用程序的左侧边栏中。IronPDF 会自动从 PDF 文档中导入现有书签,并允许添加、编辑和嵌套更多书签。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-15.cs
// Create a new PDF or edit an existing document.
PdfDocument pdf = PdfDocument.FromFile("existing.pdf");
// Add bookmark
pdf.Bookmarks.AddBookMarkAtEnd("Author's Note", 2);
pdf.Bookmarks.AddBookMarkAtEnd("Table of Contents", 3);
// Store new bookmark in a variable to add nested bookmarks to
var summaryBookmark = pdf.Bookmarks.AddBookMarkAtEnd("Summary", 17);
// Add a sub-bookmark within the summary
var conclusionBookmark = summaryBookmark.Children.AddBookMarkAtStart("Conclusion", 18);
// Add another bookmark to end of highest-level bookmark list
pdf.Bookmarks.AddBookMarkAtEnd("References", 20);
pdf.SaveAs("existing.pdf");
' Create a new PDF or edit an existing document.
Dim pdf As PdfDocument = PdfDocument.FromFile("existing.pdf")
' Add bookmark
pdf.Bookmarks.AddBookMarkAtEnd("Author's Note", 2)
pdf.Bookmarks.AddBookMarkAtEnd("Table of Contents", 3)
' Store new bookmark in a variable to add nested bookmarks to
Dim summaryBookmark = pdf.Bookmarks.AddBookMarkAtEnd("Summary", 17)
' Add a sub-bookmark within the summary
Dim conclusionBookmark = summaryBookmark.Children.AddBookMarkAtStart("Conclusion", 18)
' Add another bookmark to end of highest-level bookmark list
pdf.Bookmarks.AddBookMarkAtEnd("References", 20)
pdf.SaveAs("existing.pdf")
要查看代码示例页面上的大纲和书签示例,请访问 这里.
添加和编辑注释
IronPDF 拥有大量自定义 PDF 注释的功能。请参阅以下示例,了解其功能:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-16.cs
// create a new PDF or load and edit an existing document.
var pdf = PdfDocument.FromFile("existing.pdf");
// Create a PDF annotation object
var textAnnotation = new IronPdf.Annotations.TextAnnotation(PageIndex: 0)
{
Title = "This is the major title",
Contents = "This is the long 'sticky note' comment content...",
Subject = "This is a subtitle",
Icon = IronPdf.Annotations.TextAnnotation.AnnotationIcon.Help,
Opacity = 0.9,
Printable = false,
Hidden = false,
OpenByDefault = true,
ReadOnly = false,
Rotatable = true,
};
// Add the annotation "sticky note" to a specific page and location within any new or existing PDF.
pdf.Annotations.Add(textAnnotation);
pdf.SaveAs("existing.pdf");
IRON VB CONVERTER ERROR developers@ironsoftware.com
PDF 注释允许在 PDF 页面添加类似 "便笺 "的注释。文本注释 "类允许以编程方式添加注释。支持的高级文本注释功能包括大小、不透明度、图标和编辑。
添加背景和前景
使用 IronPDF,我们可以轻松合并 2 个 PDF 文件,将其中一个作为背景或前景:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-17.cs
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf");
pdf.AddBackgroundPdf(@"MyBackground.pdf");
pdf.AddForegroundOverlayPdfToPage(0, @"MyForeground.pdf", 0);
pdf.SaveAs(@"C:\Path\To\Complete.pdf");
Dim renderer = New ChromePdfRenderer()
Dim pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf")
pdf.AddBackgroundPdf("MyBackground.pdf")
pdf.AddForegroundOverlayPdfToPage(0, "MyForeground.pdf", 0)
pdf.SaveAs("C:\Path\To\Complete.pdf")
冲压和水印
制作图章和水印是任何 PDF 编辑器的基本功能。IronPDF 拥有令人惊叹的 API,可用于创建各种图章,如图像图章和 HTML 图章。所有这些都可以使用对齐和偏移进行高度自定义定位,点击此处即可查看:
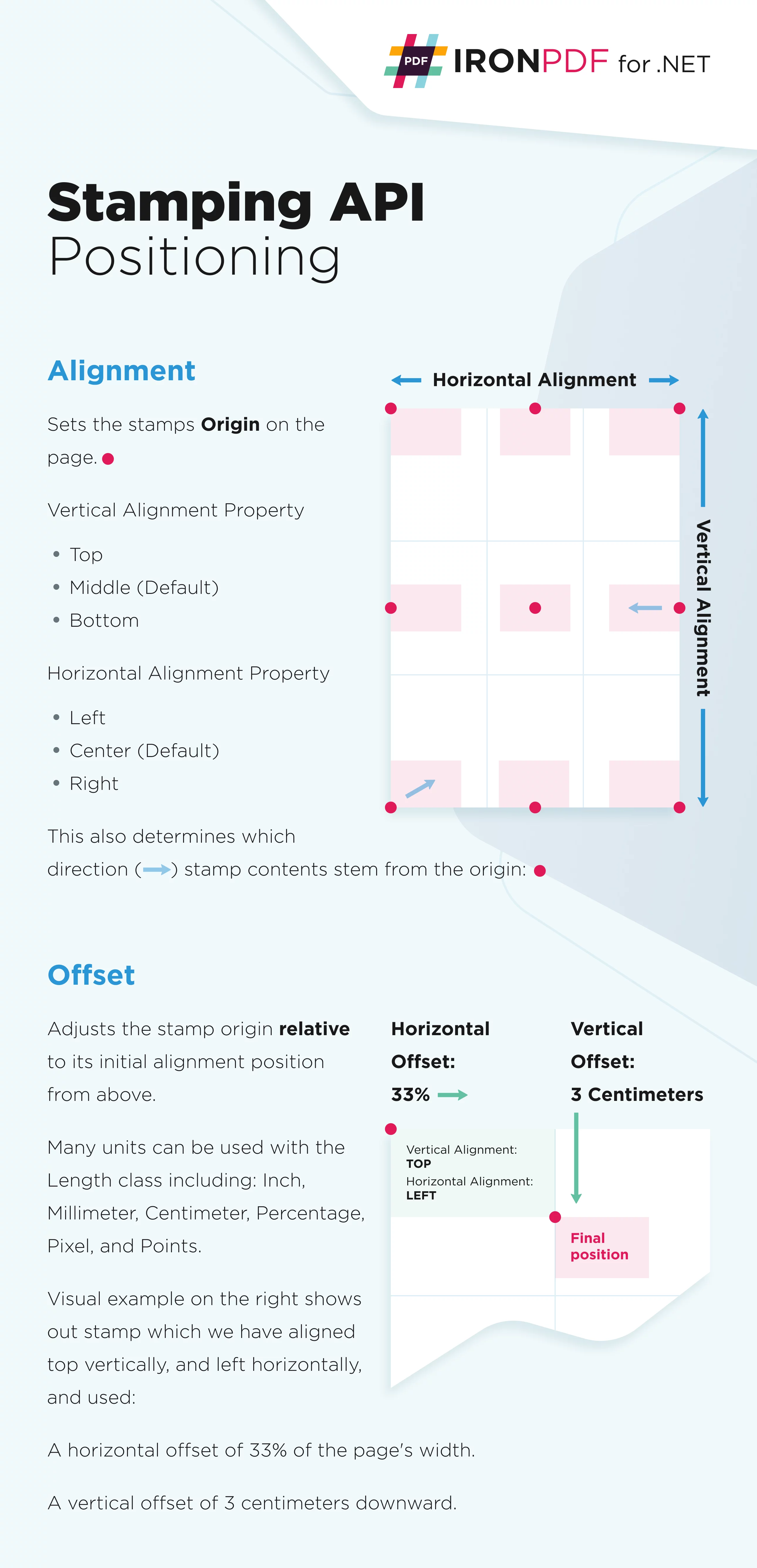
Stamper 抽象类
Stamper "抽象类用作所有 IronPDF 冲压方法的参数。
每个用例都有许多类:
- 为文本盖章的
TextStamper
类 文本示例 - 为图像盖章的 "ImageStamper 图片示例
- HtmlStamper "可对 HTML 进行标记 HTML 示例
- 用于在条形码上加盖戳记的 "BarcodeStamper"(条形码戳记器)。 条形码示例
- 用于在 QR 代码上加盖戳记的 "BarcodeStamper"(条形码戳记器)。 QR 码示例
- 有关水印,请参阅 这里.
要应用其中任何一个,请使用我们的 `ApplyStamp()方法。参见: 本教程的 "应用印章 "部分.
Stamper 类属性
abstract class Stamper
└─── int : Opacity
└─── int : Rotation
└─── double : Scale
└─── Length : HorizontalOffset
└─── Length : VerticalOffset
└─── Length : MinWidth
└─── Length : MaxWidth
└─── Length : MinHeight
└─── Length : MaxHeight
└─── string : Hyperlink
└─── bool : IsStampBehindContent (default : false)
└─── HorizontalAlignment : HorizontalAlignment
│ │ Left
│ │ Center (default)
│ │ Right
│
└─── VerticalAlignment : VerticalAlignment
│ Top
│ Middle (default)
│ Bottom
冲压示例
下面我们通过代码示例展示 Stamper 的每个子类。
在 PDF 上加盖文本印记
以不同的方式创建两种不同的文本图章,并同时应用:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-18.cs
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>");
TextStamper stamper1 = new TextStamper
{
Text = "Hello World! Stamp One Here!",
FontFamily = "Bungee Spice",
UseGoogleFont = true,
FontSize = 100,
IsBold = true,
IsItalic = true,
VerticalAlignment = VerticalAlignment.Top
};
TextStamper stamper2 = new TextStamper()
{
Text = "Hello World! Stamp Two Here!",
FontFamily = "Bungee Spice",
UseGoogleFont = true,
FontSize = 30,
VerticalAlignment = VerticalAlignment.Bottom
};
Stamper[] stampersToApply = { stamper1, stamper2 };
pdf.ApplyMultipleStamps(stampersToApply);
pdf.ApplyStamp(stamper2);
Dim renderer = New ChromePdfRenderer()
Dim pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>")
Dim stamper1 As New TextStamper With {
.Text = "Hello World! Stamp One Here!",
.FontFamily = "Bungee Spice",
.UseGoogleFont = True,
.FontSize = 100,
.IsBold = True,
.IsItalic = True,
.VerticalAlignment = VerticalAlignment.Top
}
Dim stamper2 As New TextStamper() With {
.Text = "Hello World! Stamp Two Here!",
.FontFamily = "Bungee Spice",
.UseGoogleFont = True,
.FontSize = 30,
.VerticalAlignment = VerticalAlignment.Bottom
}
Dim stampersToApply() As Stamper = { stamper1, stamper2 }
pdf.ApplyMultipleStamps(stampersToApply)
pdf.ApplyStamp(stamper2)
将图像印到 PDF 上
在现有 PDF 文档的不同页面组合上应用图像印记:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-19.cs
var pdf = new PdfDocument("/attachments/2022_Q1_sales.pdf");
ImageStamper logoImageStamper = new ImageStamper("/assets/logo.png");
// Apply to every page, one page, or some pages
pdf.ApplyStamp(logoImageStamper);
pdf.ApplyStamp(logoImageStamper, 0);
pdf.ApplyStamp(logoImageStamper, new[] { 0, 3, 11 });
Dim pdf = New PdfDocument("/attachments/2022_Q1_sales.pdf")
Dim logoImageStamper As New ImageStamper("/assets/logo.png")
' Apply to every page, one page, or some pages
pdf.ApplyStamp(logoImageStamper)
pdf.ApplyStamp(logoImageStamper, 0)
pdf.ApplyStamp(logoImageStamper, { 0, 3, 11 })
在 PDF 上加印 HTML
编写自己的 HTML 作为图章:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-20.cs
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderHtmlAsPdf("<p>Hello World, example HTML body.</p>");
HtmlStamper stamper = new HtmlStamper($"<p>Example HTML Stamped</p><div style='width:250pt;height:250pt;background-color:red;'></div>")
{
HorizontalOffset = new Length(-3, MeasurementUnit.Inch),
VerticalAlignment = VerticalAlignment.Bottom
};
pdf.ApplyStamp(stamper);
Dim renderer = New ChromePdfRenderer()
Dim pdf = renderer.RenderHtmlAsPdf("<p>Hello World, example HTML body.</p>")
Dim stamper As New HtmlStamper($"<p>Example HTML Stamped</p><div style='width:250pt;height:250pt;background-color:red;'></div>")
If True Then
'INSTANT VB WARNING: An assignment within expression was extracted from the following statement:
'ORIGINAL LINE: HorizontalOffset = new Length(-3, MeasurementUnit.Inch), VerticalAlignment = VerticalAlignment.Bottom
New Length(-3, MeasurementUnit.Inch), VerticalAlignment = VerticalAlignment.Bottom
HorizontalOffset = New Length(-3, MeasurementUnit.Inch), VerticalAlignment
End If
pdf.ApplyStamp(stamper)
在 PDF 上加印条形码
创建和印制条形码的示例:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-21.cs
BarcodeStamper bcStamp = new BarcodeStamper("IronPDF", BarcodeEncoding.Code39);
bcStamp.HorizontalAlignment = HorizontalAlignment.Left;
bcStamp.VerticalAlignment = VerticalAlignment.Bottom;
var pdf = new PdfDocument("example.pdf");
pdf.ApplyStamp(bcStamp);
Dim bcStamp As New BarcodeStamper("IronPDF", BarcodeEncoding.Code39)
bcStamp.HorizontalAlignment = HorizontalAlignment.Left
bcStamp.VerticalAlignment = VerticalAlignment.Bottom
Dim pdf = New PdfDocument("example.pdf")
pdf.ApplyStamp(bcStamp)
在 PDF 上加印 QR 码
创建并加印 QR 码的示例:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-22.cs
BarcodeStamper qrStamp = new BarcodeStamper("IronPDF", BarcodeEncoding.QRCode);
qrStamp.Height = 50; // pixels
qrStamp.Width = 50; // pixels
qrStamp.HorizontalAlignment = HorizontalAlignment.Left;
qrStamp.VerticalAlignment = VerticalAlignment.Bottom;
var pdf = new PdfDocument("example.pdf");
pdf.ApplyStamp(qrStamp);
Dim qrStamp As New BarcodeStamper("IronPDF", BarcodeEncoding.QRCode)
qrStamp.Height = 50 ' pixels
qrStamp.Width = 50 ' pixels
qrStamp.HorizontalAlignment = HorizontalAlignment.Left
qrStamp.VerticalAlignment = VerticalAlignment.Bottom
Dim pdf = New PdfDocument("example.pdf")
pdf.ApplyStamp(qrStamp)
为 PDF 添加水印
水印是每一页的一种印记,可使用 "ApplyWatermark "方法轻松应用:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-23.cs
var pdf = new PdfDocument("/attachments/design.pdf");
string html = "<h1> Example Title <h1/>";
int rotation = 0;
int watermarkOpacity = 30;
pdf.ApplyWatermark(html, rotation, watermarkOpacity);
Dim pdf = New PdfDocument("/attachments/design.pdf")
Dim html As String = "<h1> Example Title <h1/>"
Dim rotation As Integer = 0
Dim watermarkOpacity As Integer = 30
pdf.ApplyWatermark(html, rotation, watermarkOpacity)
要查看代码示例页面上的水印示例,请访问 这里.
在 PDF 上应用印章
ApplyStamp 方法有几种重载,可用于在 PDF 上应用印章。
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-24.cs
var pdf = new PdfDocument("/assets/example.pdf");
// Apply one stamp to all pages
pdf.ApplyStamp(myStamper);
// Apply one stamp to a specific page
pdf.ApplyStamp(myStamper, 0);
// Apply one stamp to specific pages
pdf.ApplyStamp(myStamper, new[] { 0, 3, 5 });
// Apply a stamp array to all pages
pdf.ApplyMultipleStamps(stampArray);
// Apply a stamp array to a specific page
pdf.ApplyMultipleStamps(stampArray, 0);
// Apply a stamp array to specific pages
pdf.ApplyMultipleStamps(stampArray, new[] { 0, 3, 5 });
// And some Async versions of the above
await pdf.ApplyStampAsync(myStamper, 4);
await pdf.ApplyMultipleStampsAsync(stampArray);
// Additional Watermark apply method
string html = "<h1> Example Title <h1/>";
int rotation = 0;
int watermarkOpacity = 30;
pdf.ApplyWatermark(html, rotation, watermarkOpacity);
Dim pdf = New PdfDocument("/assets/example.pdf")
' Apply one stamp to all pages
pdf.ApplyStamp(myStamper)
' Apply one stamp to a specific page
pdf.ApplyStamp(myStamper, 0)
' Apply one stamp to specific pages
pdf.ApplyStamp(myStamper, { 0, 3, 5 })
' Apply a stamp array to all pages
pdf.ApplyMultipleStamps(stampArray)
' Apply a stamp array to a specific page
pdf.ApplyMultipleStamps(stampArray, 0)
' Apply a stamp array to specific pages
pdf.ApplyMultipleStamps(stampArray, { 0, 3, 5 })
' And some Async versions of the above
Await pdf.ApplyStampAsync(myStamper, 4)
Await pdf.ApplyMultipleStampsAsync(stampArray)
' Additional Watermark apply method
Dim html As String = "<h1> Example Title <h1/>"
Dim rotation As Integer = 0
Dim watermarkOpacity As Integer = 30
pdf.ApplyWatermark(html, rotation, watermarkOpacity)
长度等级
Length 类有两个属性:单位 "和 "值"。从 MeasurementUnit
枚举中决定使用哪个单位后 (默认为页面的 `百分比)然后选择 "值",以决定使用基本单位倍数的长度量。
长度类属性
class Length
└─── double : Value (default : 0)
└─── MeasurementUnit : Unit
Inch
Millimeter
Centimeter
Percentage (default)
Pixel
Points
长度示例
创建长度
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-25.cs
new Length(value: 5, unit: MeasurementUnit.Inch); // 5 inches
new Length(value: 25, unit: MeasurementUnit.Pixel);// 25px
new Length(); // 0% of the page dimension because value is defaulted to zero and unit is defaulted to percentage
new Length(value: 20); // 20% of the page dimension
Dim tempVar As New Length(value:= 5, unit:= MeasurementUnit.Inch) ' 5 inches
Dim tempVar2 As New Length(value:= 25, unit:= MeasurementUnit.Pixel) ' 25px
Dim tempVar3 As New Length() ' 0% of the page dimension because value is defaulted to zero and unit is defaulted to percentage
Dim tempVar4 As New Length(value:= 20) ' 20% of the page dimension
使用长度作为参数
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-26.cs
HtmlStamper logoStamper = new HtmlStamper
{
VerticalOffset = new Length(15, MeasurementUnit.Percentage),
HorizontalOffset = new Length(1, MeasurementUnit.Inch)
// set other properties...
};
Dim logoStamper As New HtmlStamper With {
.VerticalOffset = New Length(15, MeasurementUnit.Percentage),
.HorizontalOffset = New Length(1, MeasurementUnit.Inch)
}
在 PDF 文件中使用表单
创建和编辑表格
使用 IronPDF 创建内嵌表单字段的 PDF:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-27.cs
// Step 1. Creating a PDF with editable forms from HTML using form and input tags
const string formHtml = @"
<html>
<body>
<h2>Editable PDF Form</h2>
<form>
First name: <br> <input type='text' name='firstname' value=''> <br>
Last name: <br> <input type='text' name='lastname' value=''>
</form>
</body>
</html>";
// Instantiate Renderer
var renderer = new ChromePdfRenderer();
renderer.RenderingOptions.CreatePdfFormsFromHtml = true;
renderer.RenderHtmlAsPdf(formHtml).SaveAs("BasicForm.pdf");
// Step 2. Reading and Writing PDF form values.
var formDocument = PdfDocument.FromFile("BasicForm.pdf");
// Read the value of the "firstname" field
var firstNameField = formDocument.Form.FindFormField("firstname");
// Read the value of the "lastname" field
var lastNameField = formDocument.Form.FindFormField("lastname");
' Step 1. Creating a PDF with editable forms from HTML using form and input tags
Const formHtml As String = "
<html>
<body>
<h2>Editable PDF Form</h2>
<form>
First name: <br> <input type='text' name='firstname' value=''> <br>
Last name: <br> <input type='text' name='lastname' value=''>
</form>
</body>
</html>"
' Instantiate Renderer
Dim renderer = New ChromePdfRenderer()
renderer.RenderingOptions.CreatePdfFormsFromHtml = True
renderer.RenderHtmlAsPdf(formHtml).SaveAs("BasicForm.pdf")
' Step 2. Reading and Writing PDF form values.
Dim formDocument = PdfDocument.FromFile("BasicForm.pdf")
' Read the value of the "firstname" field
Dim firstNameField = formDocument.Form.FindFormField("firstname")
' Read the value of the "lastname" field
Dim lastNameField = formDocument.Form.FindFormField("lastname")
要查看代码示例页面上的 PDF 表单示例,请访问 这里.
填写现有表格
使用 IronPDF,您可以轻松访问 PDF 中的所有现有表单字段,并在重新保存时填写它们:
:path=/static-assets/pdf/content-code-examples/tutorials/csharp-edit-pdf-complete-28.cs
var formDocument = PdfDocument.FromFile("BasicForm.pdf");
// Set and Read the value of the "firstname" field
var firstNameField = formDocument.Form.FindFormField("firstname");
firstNameField.Value = "Minnie";
Console.WriteLine("FirstNameField value: {0}", firstNameField.Value);
// Set and Read the value of the "lastname" field
var lastNameField = formDocument.Form.FindFormField("lastname");
lastNameField.Value = "Mouse";
Console.WriteLine("LastNameField value: {0}", lastNameField.Value);
formDocument.SaveAs("FilledForm.pdf");
Dim formDocument = PdfDocument.FromFile("BasicForm.pdf")
' Set and Read the value of the "firstname" field
Dim firstNameField = formDocument.Form.FindFormField("firstname")
firstNameField.Value = "Minnie"
Console.WriteLine("FirstNameField value: {0}", firstNameField.Value)
' Set and Read the value of the "lastname" field
Dim lastNameField = formDocument.Form.FindFormField("lastname")
lastNameField.Value = "Mouse"
Console.WriteLine("LastNameField value: {0}", lastNameField.Value)
formDocument.SaveAs("FilledForm.pdf")
要查看代码示例页面上的 PDF 表单示例,请访问 这里.
结论
上述例子表明,IronPDF 在编辑 PDF 时具有开箱即用的关键功能。
如果您想提出功能请求,或有任何关于 IronPDF 或许可的一般问题,请 联系我们的支持团队.我们将非常乐意为您提供帮助。