如何在 ASP .NET Core 中将 HTML 转换为 PDF
.NET Core PDF生成器
创建 .NET Core PDF文件是一项繁琐的任务。 在ASP.NET MVC项目中处理PDF文件,以及将MVC视图、HTML文件和在线网页转换为PDF可能具有挑战性。 本教程使用IronPDF工具来解决这些问题,为您的许多PDF .NET需求提供指导性指南。
IronPDF 还支持使用 Chrome 对您的 HTML 进行调试,以实现像素完美的 PDF。了解更多。
如何在 .NET Core 中将 HTML 转换为 PDF
- 下载将HTML转换为PDF的C#库
- 使用
RenderUrlAsPdf
将网页URL转换为PDF - 使用
RenderHtmlAsPdf
将HTML标记字符串转换为PDF - 通过配置 Model 和 Services 类将 MVC 视图转换为 PDF
- 修改HTML页面以使用模型,并调用方法将HTML传递给
RenderHtmlAsPdf
概述
完成本教程后,您将能够:
- 从不同的来源(如URL、HTML、MVC视图)转换为PDF
- 使用用于不同输出 PDF 设置的高级选项进行交互
- 将您的项目部署到 Linux 和 Windows 上
- 使用 PDF 文档操作功能
- 添加页眉和页脚、合并文件、添加印章
-
使用Docker
这种广泛的.NET Core HTML转PDF功能将有助于满足各种项目需求。
开始使用IronPDF
立即在您的项目中开始使用IronPDF,并享受免费试用。
步骤 1
1. 免费安装 IronPDF 库
IronPDF可以安装并用于所有.NET项目类型,如Windows应用程序、ASP.NET MVC和.NET Core应用程序。
要将 IronPDF 库添加到我们的项目中有两种方式,一种是通过 Visual Studio 编辑器使用 NuGet 进行安装,另一种是使用包管理控制台通过命令行进行安装。
使用 NuGet 安装
要使用NuGet将IronPDF库添加到我们的项目中,我们可以使用可视化界面(NuGet包管理器)或通过命令使用包管理器控制台:
1.1.1 使用 NuGet 软件包管理器
1- Right click on project name -> Select Manage NuGet Package
2- From browser tab -> search for IronPdf -> Install
3- Click Ok
4- Done!
1.1.2 使用 NuGet 软件包控制台管理器
1- From Tools -> NuGet Package Manager -> Package Manager Console
2- Run command -> Install-Package IronPdf
教程
2.将网站转换为 PDF
样本ConvertUrlToPdf 控制台应用程序
按照以下步骤创建一个新的 Asp.NET MVC 项目
1- Open Visual Studio
2- Choose Create new project
3- 选择控制台应用程序 (.NET Core)
4- Give our sample name “ConvertUrlToPdf” and click create
5- Now we have a console application created
6- Add IronPdf => click install
7- 添加我们的第一行代码,将一个维基百科网站主页渲染为PDF
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-1.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderUrlAsPdf("https://www.wikipedia.org/");
pdf.SaveAs("wiki.pdf");
8- Run and check created file wiki.pdf
3.将 .NET Core HTML 转换为 PDF
Sample: ConvertHTMLToPdf Console application
To render HTML to PDF we have two ways:
1- Write HTML into string then render it
2- Write HTML into file and pass it path to IronPDF to render it
Rendering the HTML string sample code will look like this.
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-2.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Hello IronPdf</h1>");
pdf.SaveAs("HtmlString.pdf");
生成的PDF将如下所示。
4. 将MVC视图转换为PDF
示例:TicketsApps .NET Core MVC 应用程序
让我们实施一个现实生活中的例子。 我选择了一个在线购票网站。打开网站,导航至“预订票务”页面,填写所需信息,然后将您的票据下载为PDF文件。
我们将遵循以下步骤:
创建项目
-
选择“ASP.NET Core Web 应用程序(模型-视图-控制器)”项目。
-
将项目命名为 "TicketsApps"。
-
让我们使用启用了 Linux Docker 的 .NET 8。 在Dockerfile中,将“USER app”更改为“USER root”。 这将确保库获得足够的权限。
- 现在可以了。
添加客户端模型
-
右键单击“Models”文件夹并添加类。
-
将模型命名为 "ClientModel",然后点击添加。
- 将属性'name'、'phone'和'email'添加到ClientModel类中。 通过在每一个上添加“Required”属性,使它们全部成为必填项,如下所示:
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-3.cs
public class ClientModel
{
[Required]
public string Name { get; set; }
[Required]
public string Phone { get; set; }
[Required]
public string Email { get; set; }
}
添加客户服务
-
创建一个文件夹并将其命名为“services”。
-
添加一个名为“ClientServices”的类。
-
添加一个类型为“ClientModel”的静态对象,用作存储库。
- 添加两个功能:一个用于将客户保存到仓库中,另一个用于检索已保存的客户。
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-4.cs
public class ClientServices
{
private static ClientModel _clientModel;
public static void AddClient(ClientModel clientModel)
{
_clientModel = clientModel;
}
public static ClientModel GetClient()
{
return _clientModel;
}
}
设计订票页面
-
在解决方案资源管理器中,右键单击“Controllers”文件夹并添加一个控制器。
-
将其命名为 "BookTicketController"。
-
右键单击 index 函数(或我们称之为 action),然后选择“添加视图”。
-
添加名为 "索引 "的视图。
- 更新 HTML 如下
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-5.cs
@model IronPdfMVCHelloWorld.Models.ClientModel
@{
ViewBag.Title = "Book Ticket";
}
<h2>Index</h2>
@using (Html.BeginForm())
{
<div class="form-horizontal">
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
<div class="form-group">
@Html.LabelFor(model => model.Name, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Name, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Name, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Phone, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Phone, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Phone, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Email, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Email, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Email, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-10 pull-right">
<button type="submit" value="Save" class="btn btn-sm">
<i class="fa fa-plus"></i>
<span>
Save
</span>
</button>
</div>
</div>
</div>
}
- 添加一个导航链接,以便我们的网站访客能够导航到我们的新预定页面。 可以通过更新现有路径中的布局来完成此操作(Views -> Shared -> _Layout.cshtml)。 添加以下代码:
<li class="nav-item">
<a
class="nav-link text-dark"
asp-area=""
asp-controller="BookTicket"
asp-action="Index"
>Book Ticket</a
>
</li>
<li class="nav-item">
<a
class="nav-link text-dark"
asp-area=""
asp-controller="BookTicket"
asp-action="Index"
>Book Ticket</a
>
</li>
-
结果应该是这样的
- 导航至“预订票务”页面。 您应该会发现它看起来是这样的:
验证并保存预订信息
- 添加另一个具有 [HttpPost] 属性的索引操作,以告知 MVC 引擎此操作用于提交数据。 验证发送的模型,如果有效,代码将重定向访问者到TicketView页面。 如果无效,访客将在屏幕上收到错误验证消息。
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-7.cs
[HttpPost]
public ActionResult Index(ClientModel model)
{
if (ModelState.IsValid)
{
ClientServices.AddClient(model);
return RedirectToAction("TicketView");
}
return View(model);
}
错误信息示例
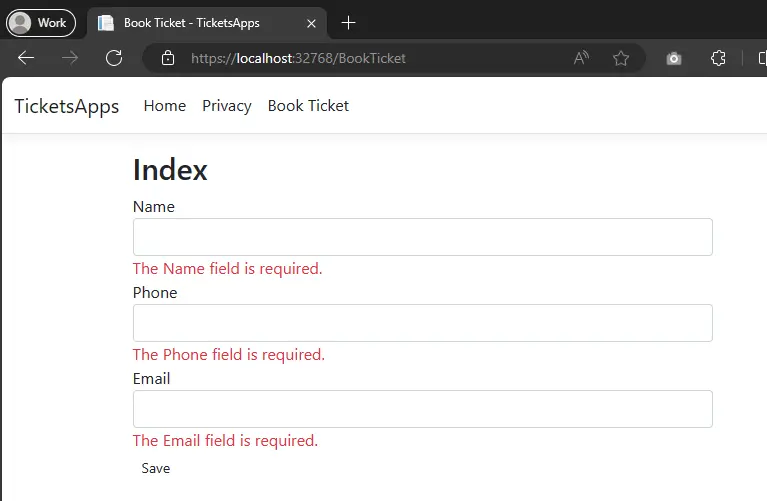
- 在 "模型 "文件中创建一个票据模型,并添加如下代码
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-9.cs
public class TicketModel : ClientModel
{
public int TicketNumber { get; set; }
public DateTime TicketDate { get; set; }
}
- 将TicketView添加到我们的票证显示。 此视图将托管一个Ticket局部视图,该视图负责显示票据,并将用于稍后打印票据。
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-8.cs
public ActionResult TicketView()
{
var rand = new Random();
var client = ClientServices.GetClient();
var ticket = new TicketModel()
{
TicketNumber = rand.Next(100000, 999999),
TicketDate = DateTime.Now,
Email = client.Email,
Name = client.Name,
Phone = client.Phone
};
return View(ticket);
}
- 右键单击 TicketView 函数,选择“添加视图”,并将其命名为“TicketView”。 添加以下代码:
@model TicketsApps.Models.TicketModel @{ ViewData["Title"] = "TicketView"; }
@Html.Partial("_TicketPdf", Model) @using (Html.BeginForm()) { @Html.HiddenFor(c
=> c.Email) @Html.HiddenFor(c => c.Name) @Html.HiddenFor(c => c.Phone)
@Html.HiddenFor(c => c.TicketDate) @Html.HiddenFor(c => c.TicketNumber)
<div class="form-group">
<div class="col-md-10 pull-right">
<button type="submit" value="Save" class="btn btn-sm">
<i class="fa fa-plus"></i>
<span> Download Pdf </span>
</button>
</div>
</div>
}
@model TicketsApps.Models.TicketModel @{ ViewData["Title"] = "TicketView"; }
@Html.Partial("_TicketPdf", Model) @using (Html.BeginForm()) { @Html.HiddenFor(c
=> c.Email) @Html.HiddenFor(c => c.Name) @Html.HiddenFor(c => c.Phone)
@Html.HiddenFor(c => c.TicketDate) @Html.HiddenFor(c => c.TicketNumber)
<div class="form-group">
<div class="col-md-10 pull-right">
<button type="submit" value="Save" class="btn btn-sm">
<i class="fa fa-plus"></i>
<span> Download Pdf </span>
</button>
</div>
</div>
}
- 右键单击 BookTicket 文件,添加另一个视图并命名为"_TicketPdf"。添加以下代码
@model TicketsApps.Models.TicketModel @{ Layout = null; }
<link href="../css/ticket.css" rel="stylesheet" />
<div class="ticket">
<div class="stub">
<div class="top">
<span class="admit">VIP</span>
<span class="line"></span>
<span class="num">
@Model.TicketNumber
<span> Ticket</span>
</span>
</div>
<div class="number">1</div>
<div class="invite">Room Number</div>
</div>
<div class="check">
<div class="big">
Your <br />
Ticket
</div>
<div class="number">VIP</div>
<div class="info">
<section>
<div class="title">Date</div>
<div>@Model.TicketDate.ToShortDateString()</div>
</section>
<section>
<div class="title">Issued By</div>
<div>Admin</div>
</section>
<section>
<div class="title">Invite Number</div>
<div>@Model.TicketNumber</div>
</section>
</div>
</div>
</div>
@model TicketsApps.Models.TicketModel @{ Layout = null; }
<link href="../css/ticket.css" rel="stylesheet" />
<div class="ticket">
<div class="stub">
<div class="top">
<span class="admit">VIP</span>
<span class="line"></span>
<span class="num">
@Model.TicketNumber
<span> Ticket</span>
</span>
</div>
<div class="number">1</div>
<div class="invite">Room Number</div>
</div>
<div class="check">
<div class="big">
Your <br />
Ticket
</div>
<div class="number">VIP</div>
<div class="info">
<section>
<div class="title">Date</div>
<div>@Model.TicketDate.ToShortDateString()</div>
</section>
<section>
<div class="title">Issued By</div>
<div>Admin</div>
</section>
<section>
<div class="title">Invite Number</div>
<div>@Model.TicketNumber</div>
</section>
</div>
</div>
</div>
-
在 "wwwroot/css" 文件中添加以下 "ticket.css" 文件。
-
将 IronPDF 添加到项目中并同意许可协议。
- 添加将处理下载按钮的 TicketView post 方法。
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-10.cs
[HttpPost]
public ActionResult TicketView(TicketModel model)
{
IronPdf.Installation.TempFolderPath = $@"{Directory.GetParent}/irontemp/";
IronPdf.Installation.LinuxAndDockerDependenciesAutoConfig = true;
var html = this.RenderViewAsync("_TicketPdf", model);
var renderer = new IronPdf.ChromePdfRenderer();
using var pdf = renderer.RenderHtmlAsPdf(html.Result, @"wwwroot/css");
return File(pdf.Stream.ToArray(), "application/pdf");
}
- 在“Controller”文件中创建一个控制器,并将其命名为“ControllerExtensions”。 此控制器将部分视图渲染为字符串。 如下使用扩展代码:
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-11.cs
using System.IO;
using System.Threading.Tasks;
public static class ControllerExtensions
{
public static async Task<string> RenderViewAsync<TModel>(this Controller controller, string viewName, TModel model, bool partial = false)
{
if (string.IsNullOrEmpty(viewName))
{
viewName = controller.ControllerContext.ActionDescriptor.ActionName;
}
controller.ViewData.Model = model;
using (var writer = new StringWriter())
{
IViewEngine viewEngine = controller.HttpContext.RequestServices.GetService(typeof(ICompositeViewEngine)) as ICompositeViewEngine;
ViewEngineResult viewResult = viewEngine.FindView(controller.ControllerContext, viewName, !partial);
if (viewResult.Success == false)
{
return $"A view with the name {viewName} could not be found";
}
ViewContext viewContext = new ViewContext(controller.ControllerContext, viewResult.View, controller.ViewData, controller.TempData, writer, new HtmlHelperOptions());
await viewResult.View.RenderAsync(viewContext);
return writer.GetStringBuilder().ToString();
}
}
}
- 运行申请并填写机票信息,然后点击 "保存"。
- View the generated ticket
下载 PDF 文件
要将票证下载为PDF格式,请点击“下载PDF”。 您将收到一份包含门票的PDF文件。
您可以下载本指南的完整代码。它是一个压缩文件,您可以在Visual Studio中打开。 点击此处下载项目。
5. .NET PDF 渲染选项图表
我们有一些高级选项可以定义PDF呈现选项,例如调整页边距,
纸张方向、纸张尺寸等。
下表展示了多种不同的选项。
Class | ChromePdfRenderer | |
---|---|---|
Description | Used to define PDF print out options, like paper size, DPI, headers and footers | |
Properties / functions | Type | Description |
CustomCookies | Dictionary<string, string> | Custom cookies for the HTML render. Cookies do not persist between renders and must be set each time. |
PaperFit | VirtualPaperLayoutManager | A manager for setting up virtual paper layouts, controlling how content will be laid out on PDF "paper" pages. Includes options for Default Chrome Behavior, Zoomed, Responsive CSS3 Layouts, Scale-To-Page & Continuous Feed style PDF page setups. |
UseMarginsOnHeaderAndFooter | UseMargins | Use margin values from the main document when rendering headers and footers. |
CreatePdfFormsFromHtml | bool | Turns all HTML form elements into editable PDF forms. Default value is true. |
CssMediaType | PdfCssMediaType | Enables Media="screen" CSS Styles and StyleSheets. Default value is PdfCssMediaType.Screen. |
CustomCssUrl | string | Allows a custom CSS style-sheet to be applied to HTML before rendering. May be a local file path or a remote URL. Only applicable when rendering HTML to PDF. |
EnableJavaScript | bool | Enables JavaScript and JSON to be executed before the page is rendered. Ideal for printing from Ajax / Angular Applications. Default value is false. |
EnableMathematicalLaTex | bool | Enables rendering of Mathematical LaTeX Elements. |
Javascript | string | A custom JavaScript string to be executed after all HTML has loaded but before PDF rendering. |
JavascriptMessageListener | StringDelegate | A method callback to be invoked whenever a browser JavaScript console message becomes available. |
FirstPageNumber | int | First page number to be used in PDF Headers and Footers. Default value is 1. |
TableOfContents | TableOfContentsTypes | Generates a table of contents at the location in the HTML document where an element is found with id "ironpdf-toc". |
GrayScale | bool | Outputs a black-and-white PDF. Default value is false. |
TextHeader | ITextHeaderFooter | Sets the footer content for every PDF page as text, supporting 'mail-merge' and automatically turning URLs into hyperlinks. |
TextFooter | ||
HtmlHeader | HtmlHeaderFooter | Sets the header content for every PDF page as HTML. Supports 'mail-merge'. |
HtmlFooter | ||
InputEncoding | Encoding | The input character encoding as a string. Default value is Encoding.UTF8. |
MarginTop | double | Top PDF "paper" margin in millimeters. Set to zero for border-less and commercial printing applications. Default value is 25. |
MarginRight | double | Right PDF "paper" margin in millimeters. Set to zero for border-less and commercial printing applications. Default value is 25. |
MarginBottom | double | Bottom PDF "paper" margin in millimeters. Set to zero for border-less and commercial printing applications. Default value is 25. |
MarginLeft | double | Left PDF "paper" margin in millimeters. Set to zero for border-less and commercial printing applications. Default value is 25. |
PaperOrientation | PdfPaperOrientation | The PDF paper orientation, such as Portrait or Landscape. Default value is Portrait. |
PaperSize | PdfPaperSize | Sets the paper size |
SetCustomPaperSizeinCentimeters | double | Sets the paper size in centimeters. |
SetCustomPaperSizeInInches | Sets the paper size in inches. | |
SetCustomPaperSizeinMilimeters | Sets the paper size in millimeters. | |
SetCustomPaperSizeinPixelsOrPoints | Sets the paper size in screen pixels or printer points. | |
PrintHtmlBackgrounds | Boolean | Indicates whether to print background-colors and images from HTML. Default value is true. |
RequestContext | RequestContexts | Request context for this render, determining isolation of certain resources such as cookies. |
Timeout | Integer | Render timeout in seconds. Default value is 60. |
Title | String | PDF Document Name and Title metadata, useful for mail-merge and automatic file naming in the IronPdf MVC and Razor extensions. |
ForcePaperSize | Boolean | Force page sizes to be exactly what is specified via IronPdf.ChromePdfRenderOptions.PaperSize by resizing the page after generating a PDF from HTML. Helps correct small errors in page size when rendering HTML to PDF. |
WaitFor | WaitFor | A wrapper object that holds configuration for wait-for mechanism for users to wait for certain events before rendering. By default, it will wait for nothing. |
6. .NET PDF 页眉页脚选项图表
Class | TextHeaderFooter | |
---|---|---|
Description | Used to define text header and footer display options | |
Properties \ functions | Type | Description |
CenterText | string | Set the text in centered/left/right of PDF header or footer. Can also merge metadata using strings placeholders: {page}, {total-pages}, {url}, {date}, {time}, {html-title}, {pdf-title} |
LeftText | ||
RightText | ||
DrawDividerLine | Boolean | Adds a horizontal line divider between the header/footer and the page content on every page of the PDF document. |
DrawDividerLineColor | Color | The color of the divider line specified for IronPdf.TextHeaderFooter.DrawDividerLine. |
Font | PdfFont | Font family used for the PDF document. Default is IronSoftware.Drawing.FontTypes.Helvetica. |
FontSize | Double | Font size in pixels. |
7. 应用PDF打印(渲染)选项
让我们尝试配置我们的PDF渲染选项。
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-12.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Set rendering options
renderer.RenderingOptions.PaperSize = IronPdf.Rendering.PdfPaperSize.A4;
renderer.RenderingOptions.PaperOrientation = IronPdf.Rendering.PdfPaperOrientation.Portrait;
renderer.RenderHtmlFileAsPdf(@"testFile.html").SaveAs("GeneratedFile.pdf");
8.Docker .NET Core 应用程序
8.1. 什么是Docker?
Docker 是一套平台即服务产品,它使用操作系统级虚拟化技术来交付称为容器的软件包。 容器相互隔离,并打包它们自己的软件、库和配置文件; 他们可以通过明确的渠道相互沟通。
您可以在Docker 和 ASP.NET Core 应用程序了解更多信息。
我们将直接进入使用 Docker 的部分,但如果您想了解更多,可以查看这里的 .NET 和 Docker 简介。 还有更多关于如何为 .NET Core 应用程序构建容器的内容。
让我们一起开始使用Docker吧。
8.2. 安装 Docker
访问 Docker 网站,安装 Docker。
点击开始。
点击下载 Mac 和 Windows 版。
免费注册,然后登录。
下载 Windows 版 Docker
开始安装 Docker
它需要重新启动。 重新启动计算机后,登录到 Docker。
现在您可以通过打开Windows命令行或PowerShell脚本运行Docker“hello world”,然后输入:
Docker运行hello-world
这是一份最重要的命令行列表,可以帮助您:
- Docker 镜像 => 列出此机器上的所有可用镜像
- Docker ps => 列出所有运行中的容器
- Docker ps –a => 列出所有容器
8.3.运行到 Linux 容器中
9.处理现有 PDF 文档
9.1. 打开现有PDF
由于您可以从 URL 和 HTML(文本或文件)创建 PDF,因此您也可以处理现有的 PDF 文档。
以下是一个示例,用于打开普通PDF或带密码的加密PDF。
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-13.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
// Open an unencrypted pdf
PdfDocument unencryptedPdf = PdfDocument.FromFile("testFile.pdf");
// Open an encrypted pdf
PdfDocument encryptedPdf = PdfDocument.FromFile("testFile2.pdf", "MyPassword");
9.2. 合并多个PDF文件
您可以按以下方式将多个PDF合并为一个PDF:
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-14.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
List<PdfDocument> PDFs = new List<PdfDocument>();
PDFs.Add(PdfDocument.FromFile("1.pdf"));
PDFs.Add(PdfDocument.FromFile("2.pdf"));
PDFs.Add(PdfDocument.FromFile("3.pdf"));
using PdfDocument PDF = PdfDocument.Merge(PDFs);
PDF.SaveAs("mergedFile.pdf");
foreach (PdfDocument pdf in PDFs)
{
pdf.Dispose();
}
在当前 PDF 的末尾添加另一个 PDF,如下所示:
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-15.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
PdfDocument pdf = PdfDocument.FromFile("1.pdf");
PdfDocument pdf2 = PdfDocument.FromFile("2.pdf");
pdf.AppendPdf(pdf2);
pdf.SaveAs("appendedFile.pdf");
从给定的索引开始,将 PDF 插入另一个 PDF:
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-16.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
PdfDocument pdf = PdfDocument.FromFile("1.pdf");
PdfDocument pdf2 = PdfDocument.FromFile("2.pdf");
pdf.InsertPdf(pdf2, 0);
pdf.SaveAs("InsertIntoSpecificIndex.pdf");
9.3 添加页眉或页脚
您可以在现有的PDF中添加页眉和页脚,或者在从HTML或URL渲染PDF时添加。
可以使用两个类在PDF中添加页眉或页脚:
- TextHeaderFooter:在页眉或页脚添加简单文本。
-
HtmlHeaderFooter:添加包含丰富HTML内容和图片的页眉或页脚。
现在让我们看两个例子,展示如何使用这两个类为现有的pdf添加页眉/页脚或在渲染时添加页眉/页脚。
9.3.1 向现有PDF添加页眉
下面是一个示例,展示如何加载现有的PDF,然后使用
AddTextHeaders
和AddHtmlFooters
方法添加页眉和页脚。
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-17.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
PdfDocument pdf = PdfDocument.FromFile("testFile.pdf");
TextHeaderFooter header = new TextHeaderFooter()
{
CenterText = "Pdf Header",
LeftText = "{date} {time}",
RightText = "{page} of {total-pages}",
DrawDividerLine = true,
FontSize = 10
};
pdf.AddTextHeaders(header);
pdf.SaveAs("withHeader.pdf");
HtmlHeaderFooter Footer = new HtmlHeaderFooter()
{
HtmlFragment = "<span style='text-align:right'> page {page} of {totalpages}</span>",
DrawDividerLine = true,
MaxHeight = 10 //mm
};
pdf.AddHtmlFooters(Footer);
pdf.SaveAs("withHeaderAndFooters.pdf");
9.3.2 向新 PDF 添加页眉和页脚
以下是使用渲染选项从HTML文件创建PDF并为其添加页眉和页脚的示例。
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-18.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
ChromePdfRenderer renderer = new ChromePdfRenderer();
renderer.RenderingOptions.TextHeader = new TextHeaderFooter()
{
CenterText = "Pdf Header",
LeftText = "{date} {time}",
RightText = "{page} of {total-pages}",
DrawDividerLine = true,
FontSize = 10
};
renderer.RenderingOptions.HtmlFooter = new HtmlHeaderFooter()
{
HtmlFragment = "<span style='text-align:right'> page {page} of {totalpages}</span>",
DrawDividerLine = true,
MaxHeight = 10
};
PdfDocument pdf = renderer.RenderHtmlFileAsPdf("test.html");
pdf.SaveAs("generatedFile.pdf");
10. 添加PDF密码和安全性
您可以为您的PDF文件设置密码并编辑文件安全设置,如防止复制和打印。
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-19.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
PdfDocument pdf = PdfDocument.FromFile("testFile.pdf");
// Edit file metadata
pdf.MetaData.Author = "john smith";
pdf.MetaData.Keywords = "SEO, Friendly";
pdf.MetaData.ModifiedDate = DateTime.Now;
// Edit file security settings
// The following code makes a PDF read only and will disallow copy & paste and printing
pdf.SecuritySettings.RemovePasswordsAndEncryption();
pdf.SecuritySettings.MakePdfDocumentReadOnly("secret-key"); //secret-key is a owner password
pdf.SecuritySettings.AllowUserAnnotations = false;
pdf.SecuritySettings.AllowUserCopyPasteContent = false;
pdf.SecuritySettings.AllowUserFormData = false;
pdf.SecuritySettings.AllowUserPrinting = IronPdf.Security.PdfPrintSecurity.FullPrintRights;
// Change or set the document ecrpytion password
pdf.Password = "123";
pdf.SaveAs("secured.pdf");
11. 数字签名 PDFs
您也可以按如下方式对PDF文件进行数字签名:
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-20.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
PdfDocument pdf = PdfDocument.FromFile("testFile.pdf");
pdf.Sign(new PdfSignature("cert123.pfx", "password"));
pdf.SaveAs("signed.pdf");
高级示例可实现更多控制:
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-21.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
PdfDocument pdf = PdfDocument.FromFile("testFile.pdf");
IronPdf.Signing.PdfSignature signature = new IronPdf.Signing.PdfSignature("cert123.pfx", "123");
// Optional signing options
signature.SigningContact = "support@ironsoftware.com";
signature.SigningLocation = "Chicago, USA";
signature.SigningReason = "To show how to sign a PDF";
// Sign the PDF with the PdfSignature. Multiple signing certificates may be used
pdf.Sign(signature);
12.从 PDF 中提取文本和图像
提取文本和图像 使用 IronPdf,您可以从 PDF 中提取文本和图像,具体方法如下:
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-22.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
PdfDocument pdf = PdfDocument.FromFile("testFile.pdf");
pdf.ExtractAllText(); // Extract all text in the pdf
pdf.ExtractTextFromPage(0); // Read text from specific page
// Extract all images in the pdf
var AllImages = pdf.ExtractAllImages();
// Extract images from specific page
var ImagesOfAPage = pdf.ExtractImagesFromPage(0);
12.1. 将PDF光栅化为图像
您也可以按以下方式将PDF页面转换为图像:
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-23.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
PdfDocument pdf = PdfDocument.FromFile("testFile.pdf");
List<int> pageList = new List<int>() { 1, 2 };
pdf.RasterizeToImageFiles("*.png", pageList);
13. 添加PDF水印
以下是如何为PDF页面添加水印的示例。
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-24.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf");
// Apply watermark
pdf.ApplyWatermark("<h2 style='color:red'>SAMPLE</h2>", 30, IronPdf.Editing.VerticalAlignment.Middle, IronPdf.Editing.HorizontalAlignment.Center);
pdf.SaveAs("Watermarked.pdf");
水印有一套受限的选项和功能。 为了获得更大的控制权,您可以使用HTMLStamper类。
:path=/static-assets/pdf/content-code-examples/tutorials/dotnet-core-pdf-generating-25.cs
IronPdf.License.LicenseKey = "YourLicenseKey";
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<div>test text </div>");
// Configure HTML stamper
HtmlStamper backgroundStamp = new HtmlStamper()
{
Html = "<h2 style='color:red'>copyright 2018 ironpdf.com",
MaxWidth = new Length(20),
MaxHeight = new Length(20),
Opacity = 50,
Rotation = -45,
IsStampBehindContent = true,
VerticalAlignment = VerticalAlignment.Middle
};
pdf.ApplyStamp(backgroundStamp);
pdf.SaveAs("stamped.pdf");
教程快速访问
保留 PDF CSharp Cheat Sheet
在您的 .NET 应用程序中使用我们的方便参考文档开发 PDF。 该文档提供了快速访问常用功能和生成与编辑 PDF 的 C# 和 VB.NET 示例的功能,这一可共享工具能够帮助您节省时间和精力,让您在项目中使用 IronPDF 和常见的 PDF 需求快速入门。
保存速查表