如何在 PDF 文件上印制文字和图像
在 PDF 上盖印文本和图像是指在现有的 PDF 文档上叠加额外的内容。这些内容通常被称为 "图章",可以是文本、图像或两者的组合。图章通常用于在 PDF 上添加信息、标签、水印或注释。
IronPdf 中总共有 4 种图章。本文将介绍TextStamper、ImageStamper、HTMLStamper和BarcodeStamper。HTMLStamper 尤其强大,因为它可以利用所有 HTML 功能和 CSS 样式。
如何在 PDF 文件上印制文字和图像
- 下载 C# 库,为文本和图像盖章
- 创建和配置所需的定型器类别
- 使用
应用印章
方法将印章应用于 PDF - 使用
应用多个邮票
方法 - 指定要应用印章的特定页面
开始在您的项目中使用IronPDF,并立即获取免费试用。
查看 IronPDF 上 Nuget 用于快速安装和部署。它有超过800万次下载,正在使用C#改变PDF。
考虑安装 IronPDF DLL 直接。下载并手动安装到您的项目或GAC表单中: IronPdf.zip
印章文本示例
首先,从 TextStamper 类中创建一个对象。该对象将包含所有配置,用于指定文本戳记的显示方式。将 TextStamper 对象传递给 ApplyStamp
方法。Text 属性将是显示的文本。此外,我们还可以指定字体家族、字体样式以及印章的位置。
:path=/static-assets/pdf/content-code-examples/how-to/stamp-text-image-stamp-text.cs
using IronPdf;
using IronPdf.Editing;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>");
// Create text stamper
TextStamper textStamper = new TextStamper()
{
Text = "Text Stamper!",
FontFamily = "Bungee Spice",
UseGoogleFont = true,
FontSize = 30,
IsBold = true,
IsItalic = true,
VerticalAlignment = VerticalAlignment.Top,
};
// Stamp the text stamper
pdf.ApplyStamp(textStamper);
pdf.SaveAs("stampText.pdf");
Imports IronPdf
Imports IronPdf.Editing
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>")
' Create text stamper
Private textStamper As New TextStamper() With {
.Text = "Text Stamper!",
.FontFamily = "Bungee Spice",
.UseGoogleFont = True,
.FontSize = 30,
.IsBold = True,
.IsItalic = True,
.VerticalAlignment = VerticalAlignment.Top
}
' Stamp the text stamper
pdf.ApplyStamp(textStamper)
pdf.SaveAs("stampText.pdf")
输出 PDF
要在 TextStamper 中实现多行文本,请使用 HTML 中的 <br> 标记。例如,"line 1 <br> line 2 "将在第一行产生 "line 1",在第二行产生 "line 2"。
邮票图像示例
与文本印章类似,我们首先从 ImageStamper 类中创建一个对象,然后使用 ApplyStamp
方法将图像应用到文档中。该方法的第二个参数还接受一个页面索引,可用于将图章应用到单页或多页。在下面的示例中,我们指定在 PDF 的第 1 页加盖图像。
提示
:path=/static-assets/pdf/content-code-examples/how-to/stamp-text-image-stamp-image.cs
using IronPdf;
using IronPdf.Editing;
using System;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>");
// Create image stamper
ImageStamper imageStamper = new ImageStamper(new Uri("https://ironpdf.com/img/svgs/iron-pdf-logo.svg"))
{
VerticalAlignment = VerticalAlignment.Top,
};
// Stamp the image stamper
pdf.ApplyStamp(imageStamper, 0);
pdf.SaveAs("stampImage.pdf");
Imports IronPdf
Imports IronPdf.Editing
Imports System
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>")
' Create image stamper
Private imageStamper As New ImageStamper(New Uri("https://ironpdf.com/img/svgs/iron-pdf-logo.svg")) With {.VerticalAlignment = VerticalAlignment.Top}
' Stamp the image stamper
pdf.ApplyStamp(imageStamper, 0)
pdf.SaveAs("stampImage.pdf")
输出 PDF
应用多个印章
使用 ApplyMultipleStamps
方法,通过传递一个印章数组,在文档上应用多个印章。
:path=/static-assets/pdf/content-code-examples/how-to/stamp-text-image-multiple-stamps.cs
using IronPdf;
using IronPdf.Editing;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>");
// Create two text stampers
TextStamper stamper1 = new TextStamper()
{
Text = "Text stamp 1",
VerticalAlignment = VerticalAlignment.Top,
HorizontalAlignment = HorizontalAlignment.Left,
};
TextStamper stamper2 = new TextStamper()
{
Text = "Text stamp 2",
VerticalAlignment = VerticalAlignment.Top,
HorizontalAlignment = HorizontalAlignment.Right,
};
Stamper[] stampersToApply = { stamper1, stamper2 };
// Apply multiple stamps
pdf.ApplyMultipleStamps(stampersToApply);
pdf.SaveAs("multipleStamps.pdf");
Imports IronPdf
Imports IronPdf.Editing
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>")
' Create two text stampers
Private stamper1 As New TextStamper() With {
.Text = "Text stamp 1",
.VerticalAlignment = VerticalAlignment.Top,
.HorizontalAlignment = HorizontalAlignment.Left
}
Private stamper2 As New TextStamper() With {
.Text = "Text stamp 2",
.VerticalAlignment = VerticalAlignment.Top,
.HorizontalAlignment = HorizontalAlignment.Right
}
Private stampersToApply() As Stamper = { stamper1, stamper2 }
' Apply multiple stamps
pdf.ApplyMultipleStamps(stampersToApply)
pdf.SaveAs("multipleStamps.pdf")
输出 PDF
印章位置
为了确定图章的位置,我们使用了一个 3x3 网格,其中有三列水平线和三行垂直线。您可以选择水平对齐方式:左、中、右,以及垂直对齐方式:上、中、下。为提高精确度,您可以调整每个位置的水平和垂直偏移量。请参考下图,直观了解这一概念。
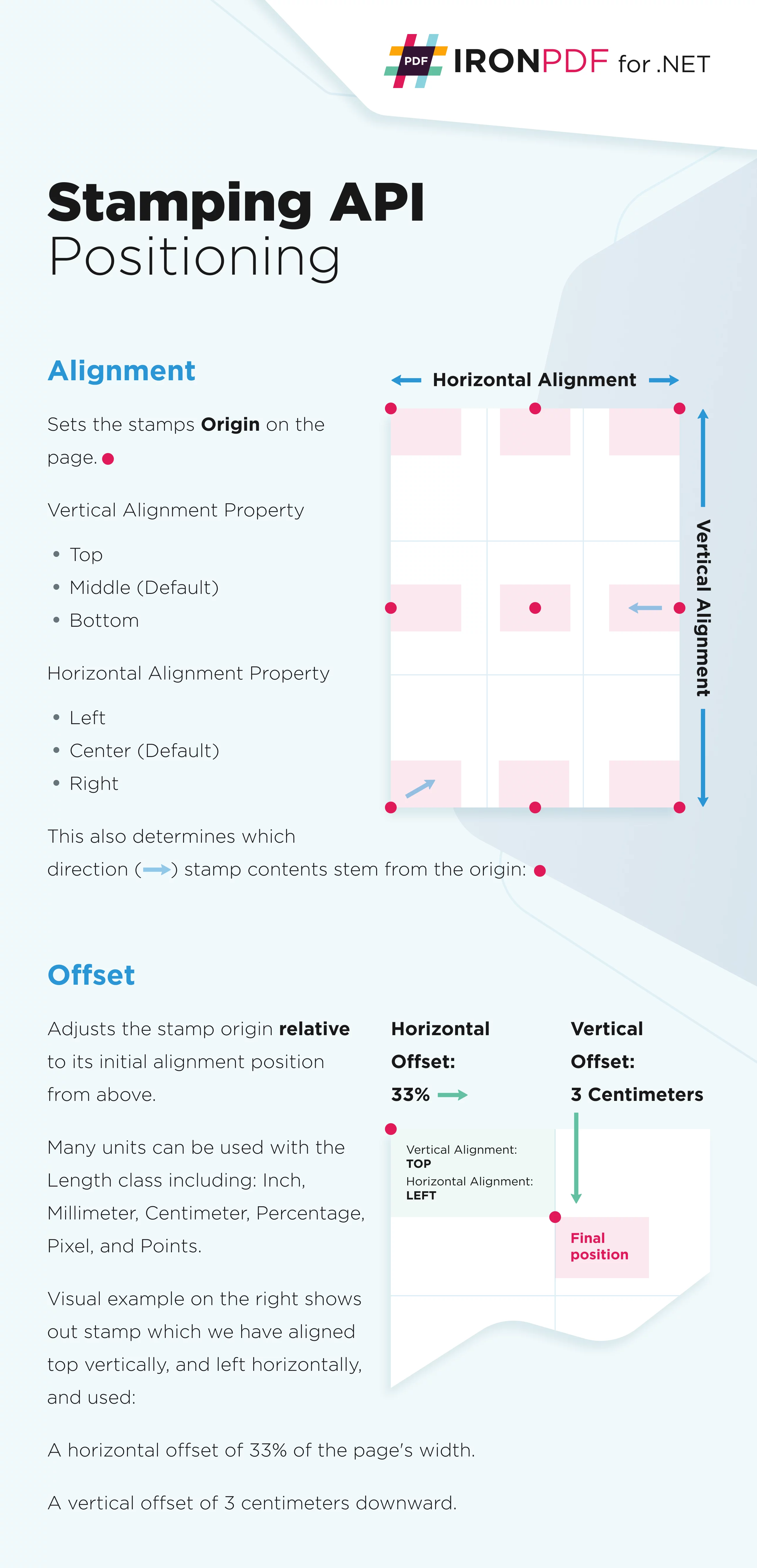
- HorizontalAlignment:邮票相对于页面的水平对齐方式。默认设置为 HorizontalAlignment.Center。
- VerticalAlignment:垂直对齐方式:邮票相对于页面的垂直对齐方式。默认设置为 VerticalAlignmentCenter.Middle。
- HorizontalOffset:水平偏移。默认值为 0,默认单位为 IronPdf.Editing.MeasurementUnit.Percentage。正值表示向右偏移,负值表示向左偏移。
- 垂直偏移:垂直偏移量。默认值为 0,默认单位为 IronPdf.Editing.MeasurementUnit.Percentage。正值表示向下偏移,负值表示向上偏移。
为了指定 HorizontalOffset 和 VerticalOffset 属性,我们将 Length 类实例化。Length 的默认测量单位是百分比,但也可以使用英寸、毫米、厘米、像素和点等测量单位。
代码
:path=/static-assets/pdf/content-code-examples/how-to/stamp-text-image-stamp-location.cs
using IronPdf.Editing;
using System;
// Create text stamper
ImageStamper imageStamper = new ImageStamper(new Uri("https://ironpdf.com/img/svgs/iron-pdf-logo.svg"))
{
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Top,
// Specify offsets
HorizontalOffset = new Length(10),
VerticalOffset = new Length(10),
};
Imports IronPdf.Editing
Imports System
' Create text stamper
Private imageStamper As New ImageStamper(New Uri("https://ironpdf.com/img/svgs/iron-pdf-logo.svg")) With {
.HorizontalAlignment = HorizontalAlignment.Center,
.VerticalAlignment = VerticalAlignment.Top,
.HorizontalOffset = New Length(10),
.VerticalOffset = New Length(10)
}
印章 HTML 示例
我们还可以使用另一种戳记类来戳记文本和图像。HtmlStamper 类可用于渲染带有 CSS 样式的 HTML 设计,然后将其印制到 PDF 文档中。HtmlBaseUrl 属性用于指定 HTML 字符串资产(如 CSS 和图像文件)的基本 URL。
代码
:path=/static-assets/pdf/content-code-examples/how-to/stamp-text-image-stamp-html.cs
using IronPdf;
using IronPdf.Editing;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>");
// Create HTML stamper
HtmlStamper htmlStamper = new HtmlStamper()
{
Html = @"<img src='https://ironpdf.com/img/svgs/iron-pdf-logo.svg'>
<h1>Iron Software</h1>",
VerticalAlignment = VerticalAlignment.Top,
};
// Stamp the HTML stamper
pdf.ApplyStamp(htmlStamper);
pdf.SaveAs("stampHtml.pdf");
Imports IronPdf
Imports IronPdf.Editing
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>")
' Create HTML stamper
Private htmlStamper As New HtmlStamper() With {
.Html = "<img src='https://ironpdf.com/img/svgs/iron-pdf-logo.svg'>
<h1>Iron Software</h1>",
.VerticalAlignment = VerticalAlignment.Top
}
' Stamp the HTML stamper
pdf.ApplyStamp(htmlStamper)
pdf.SaveAs("stampHtml.pdf")
- Html:要印制到 PDF 上的 HTML 片段。所有对 JavaScript、CSS 和图片文件的外部引用都将相对于 Stamper 类的 HtmlBaseUrl 属性。
HtmlBaseUrl:HTML 基本 URL,外部 CSS、Javascript 和图像文件的引用将相对于该 URL。
- CSSMediaType:启用 Media="screen" CSS 样式和样式表。通过设置 AllowScreenCss=false,IronPdf 将使用 CSS 从 HTML 渲染媒体="打印 "的 Stamp,就像在浏览器打印对话框中打印网页一样。默认值为 PdfCssMediaType.Screen。
邮票条形码示例
BarcodeStamper 类可用于在现有 PDF 文档上直接加印条形码。条码标记器支持的条码类型包括 QRCode、Code128 和 Code39。
代码
:path=/static-assets/pdf/content-code-examples/how-to/stamp-text-image-stamp-barcode.cs
using IronPdf;
using IronPdf.Editing;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>");
// Create barcode stamper
BarcodeStamper barcodeStamper = new BarcodeStamper("IronPdf!!", BarcodeEncoding.Code39)
{
VerticalAlignment = VerticalAlignment.Top,
};
// Stamp the barcode stamper
pdf.ApplyStamp(barcodeStamper);
pdf.SaveAs("stampBarcode.pdf");
Imports IronPdf
Imports IronPdf.Editing
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Example HTML Document!</h1>")
' Create barcode stamper
Private barcodeStamper As New BarcodeStamper("IronPdf!!", BarcodeEncoding.Code39) With {.VerticalAlignment = VerticalAlignment.Top}
' Stamp the barcode stamper
pdf.ApplyStamp(barcodeStamper)
pdf.SaveAs("stampBarcode.pdf")
- 值:条形码的字符串值。
- BarcodeType:条形码的编码类型,支持的类型包括 QRCode、Code128 和 Code39。默认为 QRCode。
- 宽度:渲染条形码的宽度(像素)。默认值为 250px。
- **高度***:渲染条形码的高度(像素)。默认值为 250px。
探索印章选项
除了上面提到和解释的选项外,下面还有更多选项可供印章类使用。
- 透明度:允许印章透明。0 表示完全不可见,100 表示完全不透明。
- 旋转:按指定的顺时针方向将图章旋转 0 至 360 度。
- 最大宽度:输出印章的最大宽度。
- MaxHeight:输出印章的最大高度。
- MinWidth:输出印章的最小宽度。
- MinHeight:输出印章的最小高度。
- 超链接:使该印章器的印章元素具有单击超链接。注意:通过链接(a) 标签不通过戳记保留。
- 比例:对图章应用百分比比例,使其变大或变小。默认值为 100 (百分比)没有任何效果。
- IsStampBehindContent:设置为 "true "可在内容后面应用图章。如果内容不透明,图章可能不可见。
WaitFor:一个方便的包装器,用于等待各种事件或等待一定时间。
- 超时:渲染超时,单位为秒。默认值为 60。