C# Imprimir Documentos PDF
Puede imprimir fácilmente un PDF en aplicaciones .NET con la ayuda de código Visual Basic o C#. Este tutorial le mostrará cómo utilizar las funciones de impresión de PDF de C# para imprimir mediante programación.
Cómo imprimir archivos PDF en C#
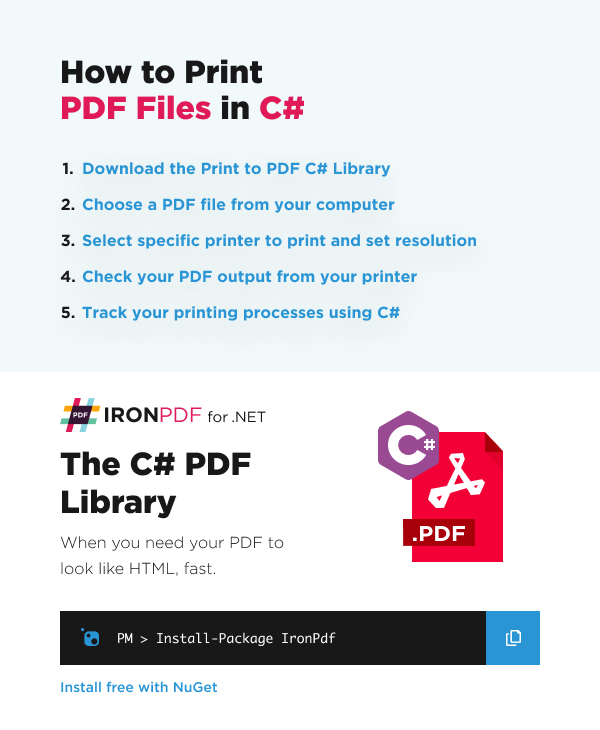
- Descargar la biblioteca Print to PDF C# Library
- Elija un archivo PDF de su ordenador
- Seleccionar una impresora específica para imprimir y establecer la resolución
- Compruebe la salida PDF de su impresora
- Seguimiento de los procesos de impresión con C#
Comience a usar IronPDF en su proyecto hoy con una prueba gratuita.
Crear un PDF e imprimir
Puede enviar un documento PDF directamente a una impresora de manera silenciosa o crear un objeto System.Drawing.Printing.PrintDocument, con el cual se puede trabajar y enviar a los cuadros de diálogo de impresión de la GUI.
El siguiente código puede utilizarse para ambas opciones:
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-create-and-print-pdf.cs
using IronPdf;
using System.Threading.Tasks;
// Create a new PDF and print it
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf");
// Print PDF from default printer
await pdf.Print();
// For advanced silent real-world printing options, use PdfDocument.GetPrintDocument
// Remember to add an assembly reference to System.Drawing.dll
System.Drawing.Printing.PrintDocument PrintDocYouCanWorkWith = pdf.GetPrintDocument();
Impresión avanzada
IronPDF es bastante capaz de lidiar con características avanzadas de Impresión como encontrar el nombre de la impresora o configurarlo y establecer la resolución de la Impresora.
Especifique el nombre de la impresora
Para especificar el nombre de la impresora, todo lo que necesitas hacer es obtener el objeto del documento de impresión actual (usando el método GetPrintDocument para documentos PDF del documento PDF), y luego usar la propiedad PrinterSettings.PrinterName, como sigue:
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-specify-printer-name.cs
using IronPdf;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
// Get PrintDocument object
var printDocument = pdf.GetPrintDocument();
// Assign the printer name
printDocument.PrinterSettings.PrinterName = "Microsoft Print to PDF";
// Print document
printDocument.Print();
Configurar la resolución de la impresora
La resolución se refiere al número de píxeles que se imprimen, o se muestran, dependiendo de la salida. Puedes establecer la resolución de tu impresión utilizando la propiedad DefaultPageSettings.PrinterResolution
del documento PDF. He aquí una demostración muy rápida:
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-specify-printer-resolution.cs
using IronPdf;
using System.Drawing.Printing;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
// Get PrintDocument object
var printDocument = pdf.GetPrintDocument();
// Set printer resolution
printDocument.DefaultPageSettings.PrinterResolution = new PrinterResolution
{
Kind = PrinterResolutionKind.Custom,
X = 1200,
Y = 1200
};
// Print document
printDocument.Print();
Como puedes ver, he ajustado la resolución a un nivel personalizado: 1200 vertical y 1200 horizontal.
Método PrintToFile
El método PdfDocument.PrintToFile
le permite imprimir el PDF en un archivo. Simplemente proporciona la ruta de salida y especifica si desea ver una vista previa. El código siguiente se imprimirá en el archivo especificado sin incluir una vista previa:
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-printtofile.cs
using IronPdf;
using System.Threading.Tasks;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
await pdf.PrintToFile("PathToFile", false);
Seguimiento de los procesos de impresión con C#
Lo bueno de C# junto con IronPDF es que, cuando se trata de realizar un seguimiento de las páginas impresas o de cualquier cosa relacionada con la impresión, en realidad es bastante sencillo. En el siguiente ejemplo, voy a demostrar cómo cambiar el nombre de la impresora, la resolución, así como la forma de obtener un recuento de las páginas que se imprimieron.
:path=/static-assets/pdf/content-code-examples/how-to/csharp-print-pdf-trace-printing-process.cs
using IronPdf;
PdfDocument pdf = PdfDocument.FromFile("sample.pdf");
// Get PrintDocument object
var printDocument = pdf.GetPrintDocument();
// Subscribe to the PrintPage event
var printedPages = 0;
printDocument.PrintPage += (sender, args) => printedPages++;
// Print document
printDocument.Print();