How to Draw Text and Bitmap on PDFs
Drawing text and images on a PDF involves adding text and images to an existing document. IronPDF seamlessly enables this feature. By incorporating text and images, users can customize PDFs with watermarks, logos, and annotations, improving the document's visual appearance and branding. Additionally, text and images facilitate the presentation of information, data visualization, and the creation of interactive forms.
Get started with IronPDF
Start using IronPDF in your project today with a free trial.
How to Draw Text and Image on PDFs in C#
- Download the C# library for IronPDF to draw text and images on PDFs
- Import the targeted PDF document
- Use the
DrawText
method to add text with the desired font to the imported PDF - Add an image to the PDF using the
DrawBitmap
method - Export the edited PDF document
Draw Text on PDF Example
By utilizing the DrawText
method available for the PdfDocument object, you can add text to an existing PDF without altering its original content.
:path=/static-assets/pdf/content-code-examples/how-to/draw-text-and-bitmap-draw-text.cs
using IronPdf;
using IronSoftware.Drawing;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>testing</h1>");
// Draw text on PDF
pdf.DrawText("Some text", FontTypes.TimesNewRoman.Name, FontSize: 12, PageIndex: 0, X: 100, Y: 100, Color.Black, Rotation: 0);
pdf.SaveAs("drawText.pdf");
Available Fonts in FontTypes Class
The DrawText
method currently supports all Standard Fonts in IronPDF, including Courier, Arial (or Helvetica), Times New Roman, Symbol, and ZapfDingbats. Visit the 'Standard Fonts in IronPDF' section in the Manage Fonts article for italic, bold, and oblique variants of these font types.
The ZapfDingbats font, in particular, can be used to display symbols such as ✖❄▲❪ ❫. For a comprehensive list of supported symbols, you can visit Wikipedia on Zapf Dingbats.
Output fonts sample on PDF
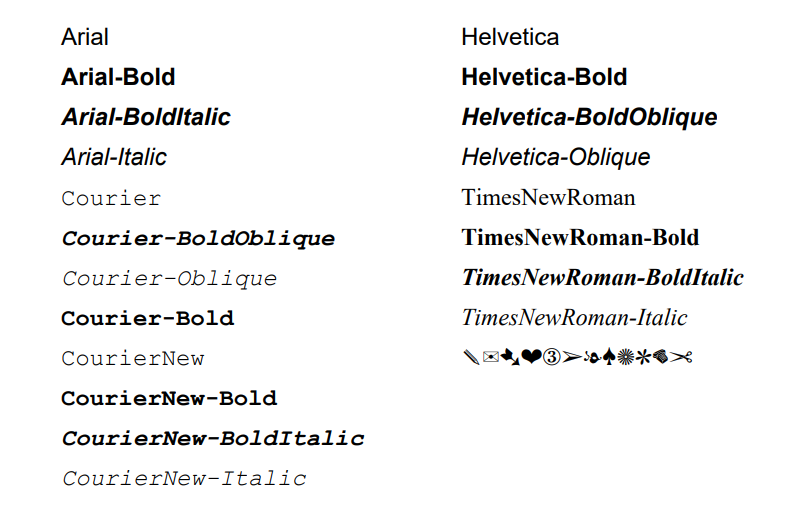
Draw Text with Newline
The draw text action supports newline characters, allowing you to render text with built-in newlines for better formatting and visual clarity.
To achieve this, add newline characters (\n)
to the text string. Using the example above, we can draw Some text\nSecond line
.
Use Custom Font
We also support using custom fonts with the DrawText
method; below is an example with the Pixelify Sans Font added for the text.
:path=/static-assets/pdf/content-code-examples/how-to/draw-text-and-bitmap-draw-custom-font.cs
using IronPdf;
using IronSoftware.Drawing;
using System.IO;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>testing</h1>");
// Add custom font to the PDF
byte[] fontByte = File.ReadAllBytes(@".\PixelifySans-VariableFont_wght.ttf");
var addedFont = pdf.Fonts.Add(fontByte);
// Draw text on PDF
pdf.DrawText("Iron Software", addedFont.Name, FontSize: 12, PageIndex: 0, X: 100, Y: 600, Color.Black, Rotation: 0);
pdf.SaveAs("drawCustomFont.pdf");
Draw Image Example
With IronPDF's DrawBitmap
method, you can easily add bitmaps to an existing PDF document. This method functions similarly to the Image Stamper feature, allowing you to stamp images onto an existing PDF.
Please note
DrawBitmap
method works best with large images. When attempting to use smaller resolution images, you may encounter the following exception: IronPdf.Exceptions.IronPdfNativeException: 'Error while drawing image: data length (567000) is less than expected (756000)'. To overcome this issue, you can use the Image Stamper, which seamlessly handles images of all sizes.Sample image
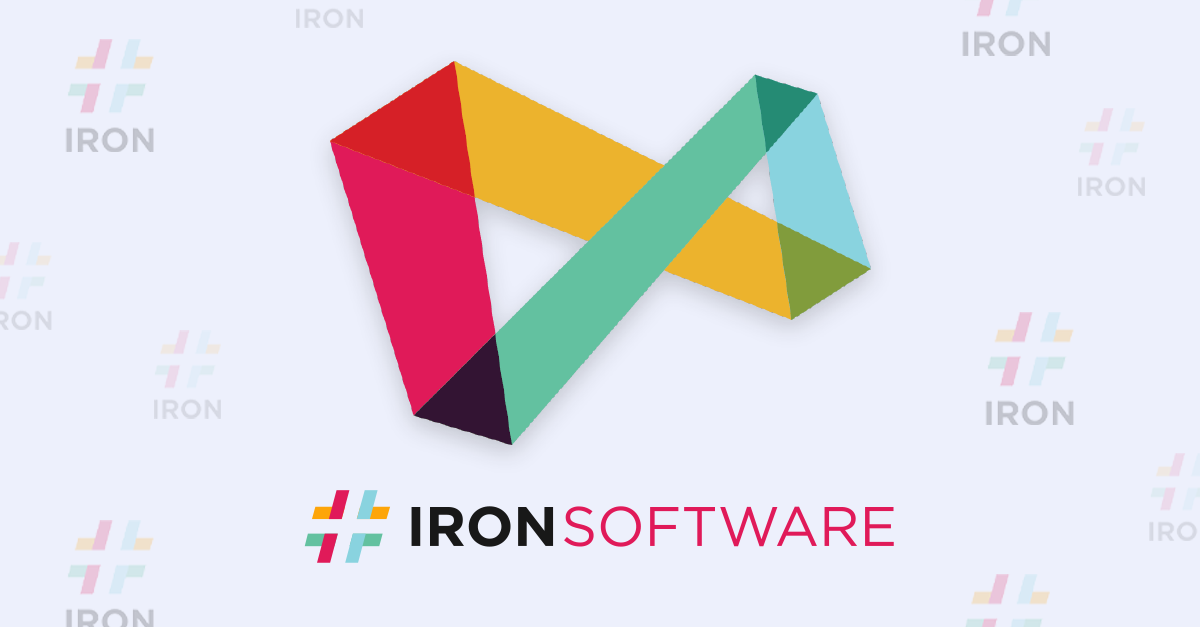
Code
:path=/static-assets/pdf/content-code-examples/how-to/draw-text-and-bitmap-draw-bitmap.cs
using IronPdf;
using IronSoftware.Drawing;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>testing</h1>");
// Open the image from file
AnyBitmap bitmap = AnyBitmap.FromFile("ironSoftware.png");
// Draw the bitmp on PDF
pdf.DrawBitmap(bitmap, 0, 50, 250, 500, 300);
pdf.SaveAs("drawImage.pdf");