How to use Custom Logging in C#
Custom logging refers to the practice of implementing a logging system tailored to the specific needs and requirements of an application or system. It involves creating and using log files to record information, events, and messages generated by the software during its operation.
Get started with IronPDF
Start using IronPDF in your project today with a free trial.
How to use Custom Logging in C#
- Download IronPDF from NuGet
- Set the LoggingMode property to LoggingModes.Custom
- Assign the CustomLogger property to your custom logger object
- All log messages will be forwarded to the custom logger
- Output the log messages to view the logs
Custom Logging Example
To utilize the custom logging feature, change the LoggingMode property to LoggingModes.Custom. Afterward, assign the CustomLogger property to the custom logger class that you have created.
:path=/static-assets/pdf/content-code-examples/how-to/custom-logging-custom-logging.cs
IronSoftware.Logger.LoggingMode = IronSoftware.Logger.LoggingModes.Custom;
IronSoftware.Logger.CustomLogger = new CustomLoggerClass("logging");
IronSoftware.Logger.LoggingMode = IronSoftware.Logger.LoggingModes.Custom
IronSoftware.Logger.CustomLogger = New CustomLoggerClass("logging")
IronPdf logs will be directed to the custom logger object. The messages will remain identical to those in the IronPdf logger; they will simply be relayed to the custom logger. How the custom logger manages the messages will be determined by the custom logger designer. Let's use the following custom logger class as an example.
:path=/static-assets/pdf/content-code-examples/how-to/custom-logging-custom-logging-class.cs
public class CustomLoggerClass : ILogger
{
private readonly string categoryName;
public CustomLoggerClass(string categoryName)
{
this.categoryName = categoryName;
}
public IDisposable BeginScope<TState>(TState state)
{
return null;
}
public bool IsEnabled(LogLevel logLevel)
{
return true;
}
public void Log<TState>(LogLevel logLevel, EventId eventId, TState state, Exception exception, Func<TState, Exception, string> formatter)
{
if (!IsEnabled(logLevel))
{
return;
}
// Implement your custom logging logic here.
string logMessage = formatter(state, exception);
// You can use 'logLevel', 'eventId', 'categoryName', and 'logMessage' to log the message as needed.
// For example, you can write it to a file, console, or another destination.
// Example: Writing to the console
Console.WriteLine($"[{logLevel}] [{categoryName}] - {logMessage}");
}
}
Public Class CustomLoggerClass
Implements ILogger
Private ReadOnly categoryName As String
Public Sub New(ByVal categoryName As String)
Me.categoryName = categoryName
End Sub
Public Function BeginScope(Of TState)(ByVal state As TState) As IDisposable
Return Nothing
End Function
Public Function IsEnabled(ByVal logLevel As LogLevel) As Boolean
Return True
End Function
Public Sub Log(Of TState)(ByVal logLevel As LogLevel, ByVal eventId As EventId, ByVal state As TState, ByVal exception As Exception, ByVal formatter As Func(Of TState, Exception, String))
If Not IsEnabled(logLevel) Then
Return
End If
' Implement your custom logging logic here.
Dim logMessage As String = formatter(state, exception)
' You can use 'logLevel', 'eventId', 'categoryName', and 'logMessage' to log the message as needed.
' For example, you can write it to a file, console, or another destination.
' Example: Writing to the console
Console.WriteLine($"[{logLevel}] [{categoryName}] - {logMessage}")
End Sub
End Class
In this case, I have prefixed the log messages with additional information.
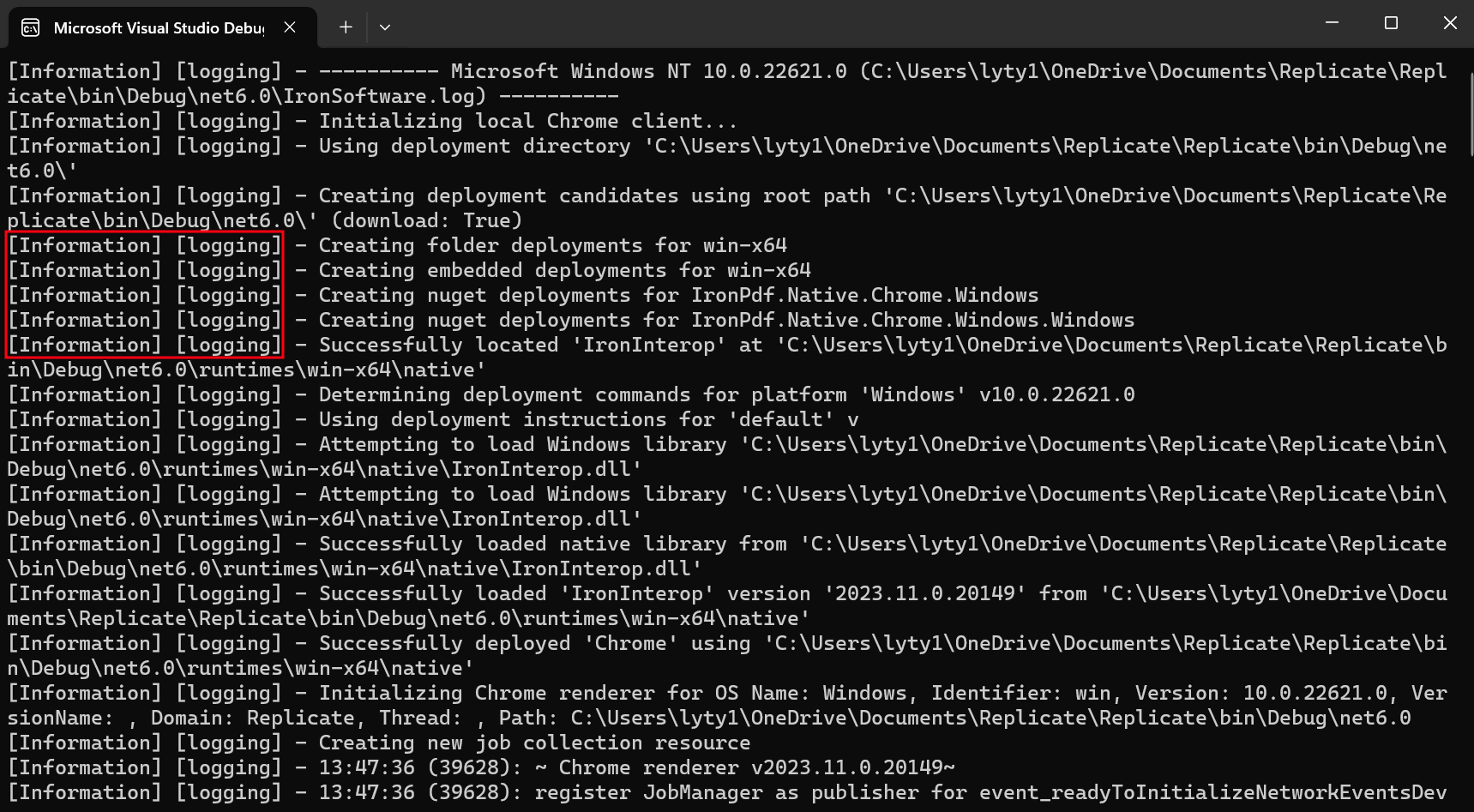