How to Convert Razor Pages to PDFs in ASP.NET Core Web App
A Razor Page is a file with a .cshtml extension that combines C# and HTML to generate web content. In ASP.NET Core, Razor Pages are a simpler way to organize code for web applications, making them a good fit for simple pages that are read-only or do simple data input.
An ASP.NET Core Web App is a web application built using ASP.NET Core, a cross-platform framework for developing modern web applications.
IronPDF simplifies the process of creating PDF files from Razor Pages within an ASP.NET Core Web App project. This makes PDF generation easy and direct in ASP.NET Core Web Apps.
How to Convert Razor Pages to PDFs in ASP.NET Core Web App
IronPDF Extension Package
The IronPdf.Extensions.Razor package is an extension of the main IronPdf package. Both the IronPdf.Extensions.Razor and IronPdf packages are needed to render Razor Pages to PDF documents in a ASP.NET Core Web App.
Install-Package IronPdf.Extensions.Razor
Install with NuGet
Install-Package IronPdf.Extensions.Razor
Render Razor Pages to PDFs
You'll need an ASP.NET Core Web App project to convert Razor pages into PDF files.
Create a Model Class
- Create a new folder in the project and name it "Models."
- Add a standard C# class to the folder and name it "Person." This class will serve as a model for individual data. Use the following code snippet:
:path=/static-assets/pdf/content-code-examples/how-to/cshtml-to-pdf-razor-model.cs
namespace RazorPageSample.Models
{
public class Person
{
public int Id { get; set; }
public string Name { get; set; }
public string Title { get; set; }
public string Description { get; set; }
}
}
Namespace RazorPageSample.Models
Public Class Person
Public Property Id() As Integer
Public Property Name() As String
Public Property Title() As String
Public Property Description() As String
End Class
End Namespace
Add a Razor Page
Add an empty Razor Page to the "Pages" folder and name it "persons.cshtml."
- Modify the newly-created "Persons.cshtml" file using the code sample provided below.
The code below is for displaying the information in the browser.
@page
@using RazorPageSample.Models;
@model RazorPageSample.Pages.PersonsModel
@{
}
<table class="table">
<tr>
<th>Name</th>
<th>Title</th>
<th>Description</th>
</tr>
@foreach (var person in ViewData["personList"] as List<Person>)
{
<tr>
<td>@person.Name</td>
<td>@person.Title</td>
<td>@person.Description</td>
</tr>
}
</table>
<form method="post">
<button type="submit">print</button>
</form>
@page
@using RazorPageSample.Models;
@model RazorPageSample.Pages.PersonsModel
@{
}
<table class="table">
<tr>
<th>Name</th>
<th>Title</th>
<th>Description</th>
</tr>
@foreach (var person in ViewData["personList"] as List<Person>)
{
<tr>
<td>@person.Name</td>
<td>@person.Title</td>
<td>@person.Description</td>
</tr>
}
</table>
<form method="post">
<button type="submit">print</button>
</form>
Next, the code below first instantiates the ChromePdfRenderer class. Passing this to the RenderRazorToPdf
method is sufficient to convert this Razor Page to a PDF document.
The user has full access to the features available in RenderingOptions. These features include the ability to apply page numbers to the generated PDF, set custom margins, and add custom text as well as HTML headers and footers.
- Open the dropdown for the "Persons.cshtml" file to see the "Persons.cshtml.cs" file.
- Modify the "Persons.cshtml.cs" with the code below.
Please note
File(pdf.BinaryData, "application/pdf")
. However, downloading the PDF after viewing it in the browser results in a damaged PDF document.using IronPdf.Razor.Pages;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using RazorPageSample.Models;
namespace RazorPageSample.Pages
{
public class PersonsModel : PageModel
{
[BindProperty(SupportsGet = true)]
public List<Person> persons { get; set; }
public void OnGet()
{
persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
ViewData["personList"] = persons;
}
public IActionResult OnPostAsync()
{
persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
ViewData["personList"] = persons;
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render Razor Page to PDF document
PdfDocument pdf = renderer.RenderRazorToPdf(this);
Response.Headers.Add("Content-Disposition", "inline");
return File(pdf.BinaryData, "application/pdf", "razorPageToPdf.pdf");
// View output PDF on broswer
return File(pdf.BinaryData, "application/pdf");
}
}
}
using IronPdf.Razor.Pages;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using RazorPageSample.Models;
namespace RazorPageSample.Pages
{
public class PersonsModel : PageModel
{
[BindProperty(SupportsGet = true)]
public List<Person> persons { get; set; }
public void OnGet()
{
persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
ViewData["personList"] = persons;
}
public IActionResult OnPostAsync()
{
persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
ViewData["personList"] = persons;
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render Razor Page to PDF document
PdfDocument pdf = renderer.RenderRazorToPdf(this);
Response.Headers.Add("Content-Disposition", "inline");
return File(pdf.BinaryData, "application/pdf", "razorPageToPdf.pdf");
// View output PDF on broswer
return File(pdf.BinaryData, "application/pdf");
}
}
}
Imports IronPdf.Razor.Pages
Imports Microsoft.AspNetCore.Mvc
Imports Microsoft.AspNetCore.Mvc.RazorPages
Imports RazorPageSample.Models
Namespace RazorPageSample.Pages
Public Class PersonsModel
Inherits PageModel
<BindProperty(SupportsGet := True)>
Public Property persons() As List(Of Person)
Public Sub OnGet()
persons = New List(Of Person) From {
New Person With {
.Name = "Alice",
.Title = "Mrs.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Bob",
.Title = "Mr.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Charlie",
.Title = "Mr.",
.Description = "Software Engineer"
}
}
ViewData("personList") = persons
End Sub
Public Function OnPostAsync() As IActionResult
persons = New List(Of Person) From {
New Person With {
.Name = "Alice",
.Title = "Mrs.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Bob",
.Title = "Mr.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Charlie",
.Title = "Mr.",
.Description = "Software Engineer"
}
}
ViewData("personList") = persons
Dim renderer As New ChromePdfRenderer()
' Render Razor Page to PDF document
Dim pdf As PdfDocument = renderer.RenderRazorToPdf(Me)
Response.Headers.Add("Content-Disposition", "inline")
Return File(pdf.BinaryData, "application/pdf", "razorPageToPdf.pdf")
' View output PDF on broswer
Return File(pdf.BinaryData, "application/pdf")
End Function
End Class
End Namespace
The RenderRazorToPdf
method returns a PdfDocument object that can undergo additional processing and editing. You can export the PDF as PDFA or PDFUA, apply a digital signature to the rendered PDF document, or merge and split PDF documents. The method also allows you to rotate pages, add annotations or bookmarks, and stamp custom watermarks onto your PDF.
Add a Section to the Top Navigation Bar
- Navigate to the Pages folder -> Shared folder -> _Layout.cshtml. Place the "Person" navigation item after "Home".
Make sure the value for the asp-page attribute matches exactly with our file name, which in this case is "Persons".
<header>
<nav class="navbar navbar-expand-sm navbar-toggleable-sm navbar-light bg-white border-bottom box-shadow mb-3">
<div class="container">
<a class="navbar-brand" asp-area="" asp-page="/Index">RazorPageSample</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target=".navbar-collapse" aria-controls="navbarSupportedContent"
aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="navbar-collapse collapse d-sm-inline-flex justify-content-between">
<ul class="navbar-nav flex-grow-1">
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Index">Home</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Persons">Person</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Privacy">Privacy</a>
</li>
</ul>
</div>
</div>
</nav>
</header>
<header>
<nav class="navbar navbar-expand-sm navbar-toggleable-sm navbar-light bg-white border-bottom box-shadow mb-3">
<div class="container">
<a class="navbar-brand" asp-area="" asp-page="/Index">RazorPageSample</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target=".navbar-collapse" aria-controls="navbarSupportedContent"
aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="navbar-collapse collapse d-sm-inline-flex justify-content-between">
<ul class="navbar-nav flex-grow-1">
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Index">Home</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Persons">Person</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-page="/Privacy">Privacy</a>
</li>
</ul>
</div>
</div>
</nav>
</header>
Run the Project
This will show you how to run the project and generate a PDF document.
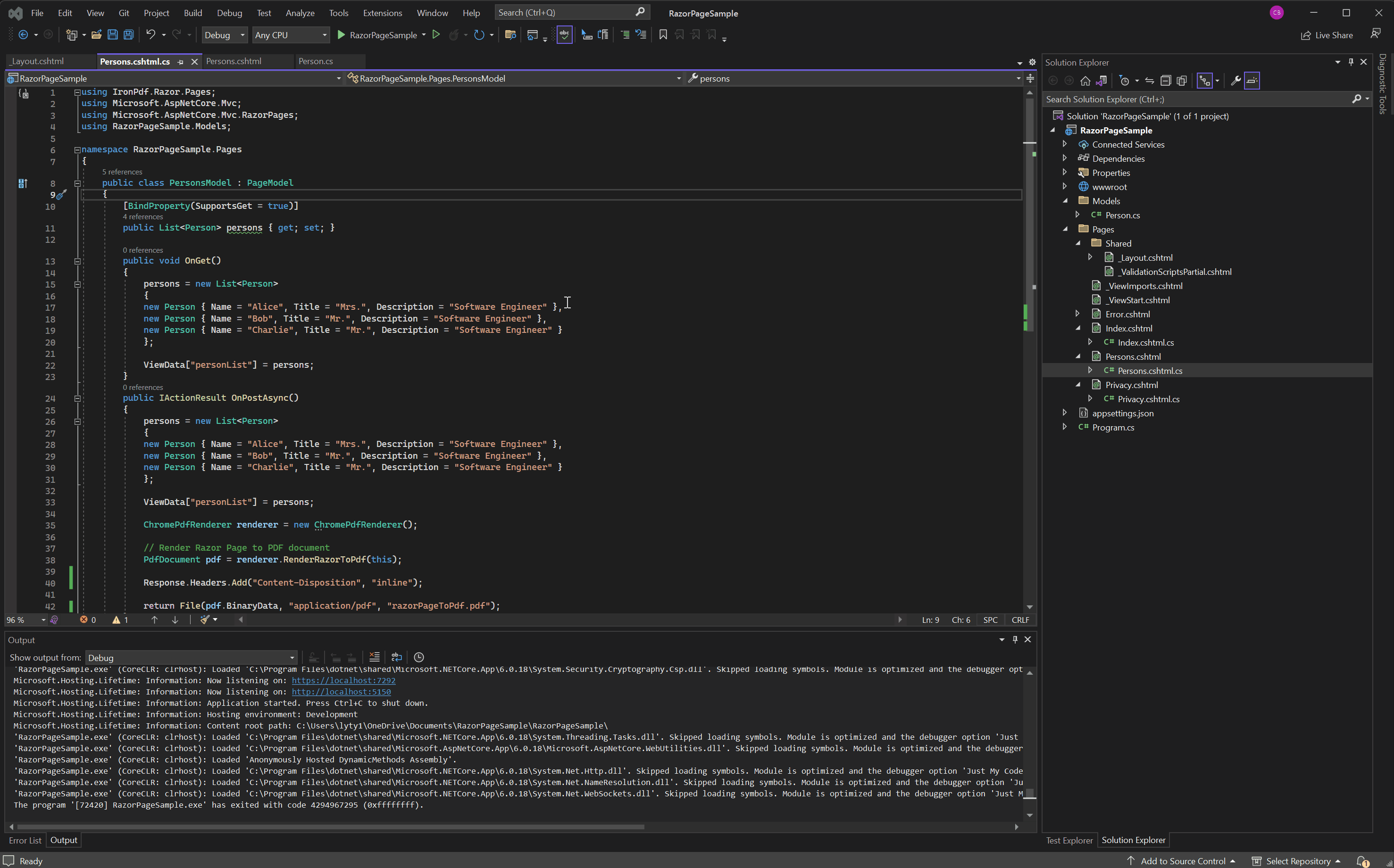
Download ASP.NET Core Web App Project
You can download the complete code for this guide. It comes as a zipped file that you can open in Visual Studio as a ASP.NET Core Web App project.