How to Rasterize a PDF to Images
Rasterizing a PDF file involves converting it into a pixel-based image format like JPEG or PNG. This process transforms each page of the PDF into a static image, where the content is represented by pixels. Rasterization offers several advantages, including the ability to display PDF content, generate thumbnails, perform image processing, and facilitate secure document sharing.
With IronPDF, you can easily and programmatically convert PDFs to images. Whether you need to incorporate PDF rendering into your application, generate image previews, perform image-based operations, or enhance document security, IronPDF has you covered.
Get started with IronPDF
Start using IronPDF in your project today with a free trial.
How to Rasterize a PDF to Images in C#
- Download the C# library for rasterizing PDF to images
- Load an existing PDF or create a PDF from a file, HTML, or URL
- Invoke the
RasterizeToImageFiles
method to export images from the PDF document - Specify the DPI to improve clarity
- Specify custom output image dimensions according to your requirements
Rasterize a PDF to Images Example
The RasterizeToImageFiles
method is utilized to export images from a PDF document. This method is available on the PdfDocument object, whether you are importing a PDF document file locally or rendering it from an HTML file to PDF conversion guide, HTML string to PDF conversion guide, or URL to PDF conversion guide.
Please note
Tips
:path=/static-assets/pdf/content-code-examples/how-to/rasterize-pdf-to-images-rasterize.cs
using IronPdf;
// Instantiate Renderer
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render PDF from web URL
PdfDocument pdf = renderer.RenderUrlAsPdf("https://en.wikipedia.org/wiki/Main_Page");
// Export images from PDF
pdf.RasterizeToImageFiles("wikipage_*.png");
Output Folder
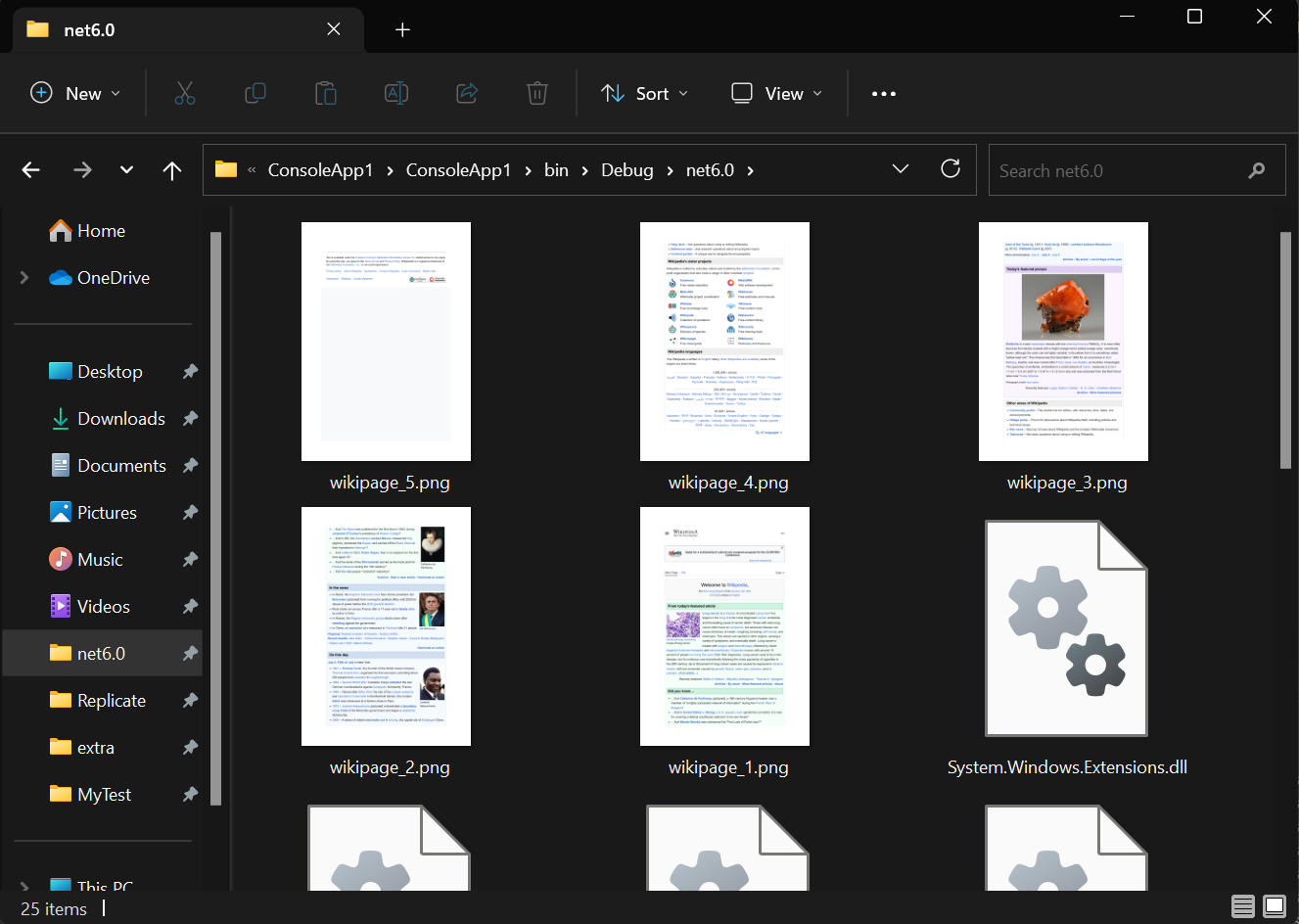
If the form fields' values are intended to be visible in the output images, please flatten the PDF before converting it to an image or pass true to the Flatten parameter of the method. Forms will not be detectable after using the Flatten
method.
Learn how to fill and edit PDF forms programmatically in the following article: "How to Fill and Edit PDF Forms."
Rasterize a PDF to Images Advanced Example
Let's explore the additional parameters available for the RasterizeToImageFiles
method.
Specify image type
Another parameter provided by the method allows you to specify the file types for the output images. We support BMP, JPEG, PNG, GIF, TIFF, and SVG formats. Each type has its corresponding method that can be directly invoked from the PdfDocument object to export the respective image type. Here are the available methods:
ToBitmap
: Rasterizes (renders) the PDF into individual IronSoftware.Drawing.AnyBitmap objects, with one Bitmap for each page.ToJpegImages
: Renders the PDF pages as JPEG files and saves them to disk.ToPngImages
: Renders the PDF pages as PNG (Portable Network Graphic) files and saves them to disk.ToTiffImages
: Renders the PDF pages as single-page TIFF (Tagged Image File Format / Tif) files and saves them to disk.ToMultiPageTiffImage
: Renders the PDF pages as a single multi-page TIFF file and saves it to disk.SaveAsSvg
: Converts the PDF document to an SVG format and saves it to the specified file path.ToSvgString
: Converts a specific page of the PDF document to an SVG format and returns it as a string.
:path=/static-assets/pdf/content-code-examples/how-to/rasterize-pdf-to-images-image-type.cs
using IronPdf;
// Instantiate Renderer
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render PDF from web URL
PdfDocument pdf = renderer.RenderUrlAsPdf("https://en.wikipedia.org/wiki/Main_Page");
// Export images from PDF
pdf.RasterizeToImageFiles("wikipage_*.png", IronPdf.Imaging.ImageType.Png);
Specify DPI
When using the default DPI of 96, the output images may appear blurry. To mitigate this phenomenon, it is important to specify a higher DPI value.
:path=/static-assets/pdf/content-code-examples/how-to/rasterize-pdf-to-images-dpi.cs
using IronPdf;
// Instantiate Renderer
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render PDF from web URL
PdfDocument pdf = renderer.RenderUrlAsPdf("https://en.wikipedia.org/wiki/Main_Page");
// Export images from PDF with DPI 150
pdf.RasterizeToImageFiles("wikipage_*.png", DPI: 150);
Specify pages index
It is also possible to specify the pages of the PDF document that you want to rasterize into image(s). In the example below, images of PDF document pages 1-3 will be generated as output.
:path=/static-assets/pdf/content-code-examples/how-to/rasterize-pdf-to-images-page-indexes.cs
using IronPdf;
using System.Linq;
// Instantiate Renderer
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render PDF from web URL
PdfDocument pdf = renderer.RenderUrlAsPdf("https://en.wikipedia.org/wiki/Main_Page");
// Export images from PDF page 1_3
pdf.RasterizeToImageFiles("wikipage_*.png", Enumerable.Range(1, 3));
Specify Image Dimensions
When converting PDF documents to images, you have the flexibility to customize the height and width of the output images. The provided height and width values represent the maximum dimensions, while ensuring that the aspect ratio of the original document is preserved. For instance, in the case of a portrait PDF document, the specified height value will be exact, while the width value may be adjusted to maintain the correct aspect ratio.
:path=/static-assets/pdf/content-code-examples/how-to/rasterize-pdf-to-images-image-dimensions.cs
using IronPdf;
// Instantiate Renderer
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render PDF from web URL
PdfDocument pdf = renderer.RenderUrlAsPdf("https://en.wikipedia.org/wiki/Main_Page");
// Export images from PDF
pdf.RasterizeToImageFiles("wikipage_*.png", 500, 500);
Specifications for Output Images
The dimensions for the output images are specified using the width by height format, denoted as width x height.
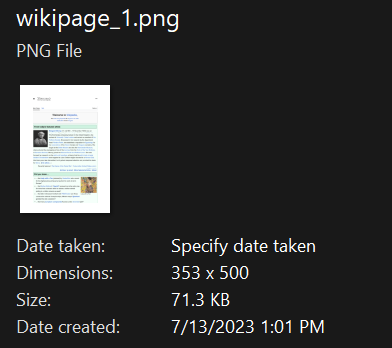
Portrait
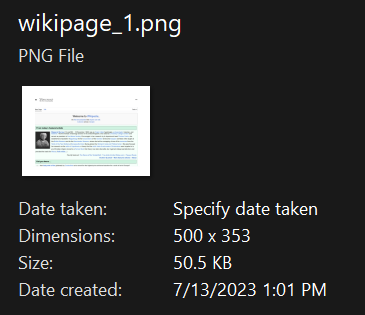
Landscape