How to Convert Views to PDFs in ASP.NET Core MVC
A View is a component in the ASP.NET framework used for generating HTML markup in web applications. It is a part of the Model-View-Controller (MVC) pattern, commonly used in ASP.NET MVC and ASP.NET Core MVC applications. Views are responsible for presenting data to the user by rendering HTML content dynamically.
ASP.NET Core Web App MVC (Model-View-Controller) is a web application provided by Microsoft for building web applications using ASP.NET Core.
- Model: The Model represents data and business logic, manages data interactions, and communicates with data sources.
- View: The View presents the user interface, focuses on displaying data, and renders information to the user.
- Controller: The Controller handles user input, responds to requests, communicates with the Model, and orchestrates interactions between the Model and the View.
IronPdf simplifies the process of creating PDF files from Views within an ASP.NET Core MVC project. This makes PDF generation easy and direct in ASP.NET Core MVC.
How to Convert Views to PDFs in ASP.NET Core MVC
IronPDF Extension Package
The IronPdf.Extensions.Mvc.Core package is an extension of the main IronPdf package. Both the IronPdf.Extensions.Mvc.Core and IronPdf packages are needed to render Views to PDF documents in a ASP.NET Core MVC.
Install-Package IronPdf.Extensions.Mvc.Core
Install with NuGet
Install-Package IronPdf.Extensions.Mvc.Core
Render Views to PDFs
You'll need an ASP.NET Core Web App (Model-View-Controller) project to convert Views into PDF files.
Add a Model Class
- Navigate to the "Models" folder
- Create a new C# class file named "Person." This class will act as a model to represent individual data. Use the following code snippet:
:path=/static-assets/pdf/content-code-examples/how-to/cshtml-to-pdf-mvc-core-model.cs
namespace ViewToPdfMVCCoreSample.Models
{
public class Person
{
public int Id { get; set; }
public string Name { get; set; }
public string Title { get; set; }
public string Description { get; set; }
}
}
Namespace ViewToPdfMVCCoreSample.Models
Public Class Person
Public Property Id() As Integer
Public Property Name() As String
Public Property Title() As String
Public Property Description() As String
End Class
End Namespace
Edit the Controller
Navigate to the "Controllers" folder and open the "HomeController" file. We are going to make changes only to the HomeController and add the "Persons" action. Please refer to the code below for guidance:
The code below first instantiates the ChromePdfRenderer class, passing an IRazorViewRenderer, the path to our "Persons.cshtml," and the List that contains the required data to the RenderRazorViewToPdf
method. Users can utilize RenderingOptions to access a range of features, such as adding custom text, including HTML headers and footers in the resulting PDF, defining custom margins, and applying page numbers.
Please note
File(pdf.BinaryData, "application/pdf")
. However, downloading the PDF after viewing it in the browser results in a damaged PDF document.using IronPdf.Extensions.Mvc.Core;
using Microsoft.AspNetCore.Mvc;
using System.Diagnostics;
using ViewToPdfMVCCoreSample.Models;
namespace ViewToPdfMVCCoreSample.Controllers
{
public class HomeController : Controller
{
private readonly ILogger<HomeController> _logger;
private readonly IRazorViewRenderer _viewRenderService;
private readonly IHttpContextAccessor _httpContextAccessor;
public HomeController(ILogger<HomeController> logger, IRazorViewRenderer viewRenderService, IHttpContextAccessor httpContextAccessor)
{
_logger = logger;
_viewRenderService = viewRenderService;
_httpContextAccessor = httpContextAccessor;
}
public IActionResult Index()
{
return View();
}
public async Task<IActionResult> Persons()
{
var persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
if (_httpContextAccessor.HttpContext.Request.Method == HttpMethod.Post.Method)
{
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render View to PDF document
PdfDocument pdf = renderer.RenderRazorViewToPdf(_viewRenderService, "Views/Home/Persons.cshtml", persons);
Response.Headers.Add("Content-Disposition", "inline");
// Output PDF document
return File(pdf.BinaryData, "application/pdf", "viewToPdfMVCCore.pdf");
}
return View(persons);
}
public IActionResult Privacy()
{
return View();
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error()
{
return View(new ErrorViewModel { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });
}
}
}
using IronPdf.Extensions.Mvc.Core;
using Microsoft.AspNetCore.Mvc;
using System.Diagnostics;
using ViewToPdfMVCCoreSample.Models;
namespace ViewToPdfMVCCoreSample.Controllers
{
public class HomeController : Controller
{
private readonly ILogger<HomeController> _logger;
private readonly IRazorViewRenderer _viewRenderService;
private readonly IHttpContextAccessor _httpContextAccessor;
public HomeController(ILogger<HomeController> logger, IRazorViewRenderer viewRenderService, IHttpContextAccessor httpContextAccessor)
{
_logger = logger;
_viewRenderService = viewRenderService;
_httpContextAccessor = httpContextAccessor;
}
public IActionResult Index()
{
return View();
}
public async Task<IActionResult> Persons()
{
var persons = new List<Person>
{
new Person { Name = "Alice", Title = "Mrs.", Description = "Software Engineer" },
new Person { Name = "Bob", Title = "Mr.", Description = "Software Engineer" },
new Person { Name = "Charlie", Title = "Mr.", Description = "Software Engineer" }
};
if (_httpContextAccessor.HttpContext.Request.Method == HttpMethod.Post.Method)
{
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Render View to PDF document
PdfDocument pdf = renderer.RenderRazorViewToPdf(_viewRenderService, "Views/Home/Persons.cshtml", persons);
Response.Headers.Add("Content-Disposition", "inline");
// Output PDF document
return File(pdf.BinaryData, "application/pdf", "viewToPdfMVCCore.pdf");
}
return View(persons);
}
public IActionResult Privacy()
{
return View();
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error()
{
return View(new ErrorViewModel { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });
}
}
}
Imports IronPdf.Extensions.Mvc.Core
Imports Microsoft.AspNetCore.Mvc
Imports System.Diagnostics
Imports ViewToPdfMVCCoreSample.Models
Namespace ViewToPdfMVCCoreSample.Controllers
Public Class HomeController
Inherits Controller
Private ReadOnly _logger As ILogger(Of HomeController)
Private ReadOnly _viewRenderService As IRazorViewRenderer
Private ReadOnly _httpContextAccessor As IHttpContextAccessor
Public Sub New(ByVal logger As ILogger(Of HomeController), ByVal viewRenderService As IRazorViewRenderer, ByVal httpContextAccessor As IHttpContextAccessor)
_logger = logger
_viewRenderService = viewRenderService
_httpContextAccessor = httpContextAccessor
End Sub
Public Function Index() As IActionResult
Return View()
End Function
Public Async Function Persons() As Task(Of IActionResult)
'INSTANT VB NOTE: The local variable persons was renamed since Visual Basic will not allow local variables with the same name as their enclosing function or property:
Dim persons_Conflict = New List(Of Person) From {
New Person With {
.Name = "Alice",
.Title = "Mrs.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Bob",
.Title = "Mr.",
.Description = "Software Engineer"
},
New Person With {
.Name = "Charlie",
.Title = "Mr.",
.Description = "Software Engineer"
}
}
If _httpContextAccessor.HttpContext.Request.Method = HttpMethod.Post.Method Then
Dim renderer As New ChromePdfRenderer()
' Render View to PDF document
Dim pdf As PdfDocument = renderer.RenderRazorViewToPdf(_viewRenderService, "Views/Home/Persons.cshtml", persons_Conflict)
Response.Headers.Add("Content-Disposition", "inline")
' Output PDF document
Return File(pdf.BinaryData, "application/pdf", "viewToPdfMVCCore.pdf")
End If
Return View(persons_Conflict)
End Function
Public Function Privacy() As IActionResult
Return View()
End Function
<ResponseCache(Duration := 0, Location := ResponseCacheLocation.None, NoStore := True)>
Public Function [Error]() As IActionResult
Return View(New ErrorViewModel With {.RequestId = If(Activity.Current?.Id, HttpContext.TraceIdentifier)})
End Function
End Class
End Namespace
After using the RenderRazorToPdf
method, you'll receive a PdfDocument object that's open to further enhancements and modifications. You have the flexibility to convert the PDF to PDFA or PDFUA formats, add your digital signature to the generated PDF, or merge and split PDF documents as needed. Additionally, the library allows you to rotate pages, insert annotations or bookmarks, and imprint unique watermarks onto your PDF files.
Add a View
- Right-click on the newly added Person action and select "Add View."
- Choose "Razor View" for the new Scaffolded item.
- Select the "List" template and the "Person" model class.
This will create a .cshtml file named "Persons."
- Navigate to the "Views" folder -> "Home" folder -> "Persons.cshtml" file.
To add a button that invokes the "Persons" action, use the code below:
@using (Html.BeginForm("Persons", "Home", FormMethod.Post))
{
<input type="submit" value="Print Person" />
}
@using (Html.BeginForm("Persons", "Home", FormMethod.Post))
{
<input type="submit" value="Print Person" />
}
Add a Section to the Top Navigation Bar
- In the same "Views" folder navigate to the "Shared" folder -> _Layout.cshtml. Place the "Person" navigation item after "Home".
Make sure the value for the asp-action attribute matches exactly with our file name, which in this case is "Persons".
<header>
<nav class="navbar navbar-expand-sm navbar-toggleable-sm navbar-light bg-white border-bottom box-shadow mb-3">
<div class="container-fluid">
<a class="navbar-brand" asp-area="" asp-controller="Home" asp-action="Index">ViewToPdfMVCCoreSample</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target=".navbar-collapse" aria-controls="navbarSupportedContent"
aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="navbar-collapse collapse d-sm-inline-flex justify-content-between">
<ul class="navbar-nav flex-grow-1">
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-controller="Home" asp-action="Index">Home</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-controller="Home" asp-action="Persons">Person</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-controller="Home" asp-action="Privacy">Privacy</a>
</li>
</ul>
</div>
</div>
</nav>
</header>
<header>
<nav class="navbar navbar-expand-sm navbar-toggleable-sm navbar-light bg-white border-bottom box-shadow mb-3">
<div class="container-fluid">
<a class="navbar-brand" asp-area="" asp-controller="Home" asp-action="Index">ViewToPdfMVCCoreSample</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target=".navbar-collapse" aria-controls="navbarSupportedContent"
aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="navbar-collapse collapse d-sm-inline-flex justify-content-between">
<ul class="navbar-nav flex-grow-1">
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-controller="Home" asp-action="Index">Home</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-controller="Home" asp-action="Persons">Person</a>
</li>
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-controller="Home" asp-action="Privacy">Privacy</a>
</li>
</ul>
</div>
</div>
</nav>
</header>
Edit Program.cs File
We will be registering the IHttpContextAccessor and IRazorViewRenderer interface to the dependecy injection (DI) container. Please check the code below for your reference.
using IronPdf.Extensions.Mvc.Core;
using Microsoft.AspNetCore.Mvc.ViewFeatures;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllersWithViews();
builder.Services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
builder.Services.AddSingleton<ITempDataProvider, CookieTempDataProvider>();
// Register IRazorViewRenderer here
builder.Services.AddSingleton<IRazorViewRenderer, RazorViewRenderer>();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
using IronPdf.Extensions.Mvc.Core;
using Microsoft.AspNetCore.Mvc.ViewFeatures;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllersWithViews();
builder.Services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
builder.Services.AddSingleton<ITempDataProvider, CookieTempDataProvider>();
// Register IRazorViewRenderer here
builder.Services.AddSingleton<IRazorViewRenderer, RazorViewRenderer>();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
Imports IronPdf.Extensions.Mvc.Core
Imports Microsoft.AspNetCore.Mvc.ViewFeatures
Private builder = WebApplication.CreateBuilder(args)
' Add services to the container.
builder.Services.AddControllersWithViews()
builder.Services.AddSingleton(Of IHttpContextAccessor, HttpContextAccessor)()
builder.Services.AddSingleton(Of ITempDataProvider, CookieTempDataProvider)()
' Register IRazorViewRenderer here
builder.Services.AddSingleton(Of IRazorViewRenderer, RazorViewRenderer)()
Dim app = builder.Build()
' Configure the HTTP request pipeline.
If Not app.Environment.IsDevelopment() Then
app.UseExceptionHandler("/Home/Error")
' The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts()
End If
app.UseHttpsRedirection()
app.UseStaticFiles()
app.UseRouting()
app.UseAuthorization()
app.MapControllerRoute(name:= "default", pattern:= "{controller=Home}/{action=Index}/{id?}")
app.Run()
Run the Project
This will show you how to run the project and generate a PDF document.
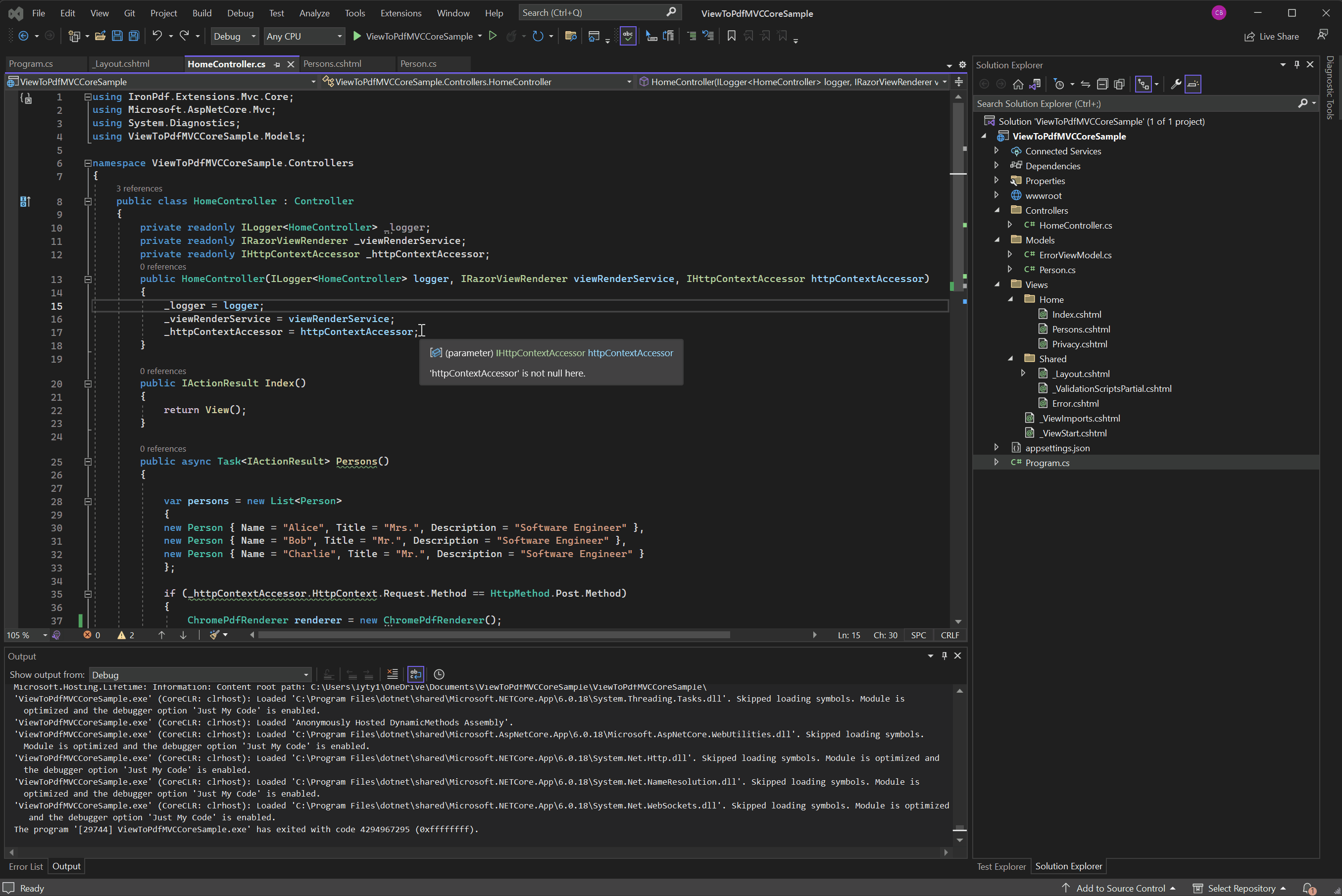
Download ASP.NET Core MVC Project
You can download the complete code for this guide. It comes as a zipped file that you can open in Visual Studio as a ASP.NET Core Web App (Model-View-Controller) project.